Example description:
1. This example uses the PWM input capture mode of the STM8 microcontroller timer TIM1 to measure the infrared remote control code.
2. The infrared remote control code format is NEC infrared code. #define uchar unsigned char uchar IrRecStep = 0; //Receiving step //--------Boot code low level range------------------ //Delay program //Initialize infrared receiving port //Use TIM1's PWM input capture TIM1_CCER1 &= 0xee; //Disable input capture 1, 2 //Infrared receiving program while (!(CLK_SWCR & 0x08)); } //Main program
3. The infrared receiving signal input is connected to PC1 (TIM1 input channel 1).
4. Use external crystal oscillator 16M.
5. Use IAR FOR STM8 development environment.
6. The program code is as follows:
//--------------------------------------------------------------------
#include
#define uint unsigned int
uchar IrIndex = 0; //Receiving bit number
uchar IrRecFlag = 0; //Receiving completed flag
uchar IrRecBuff[4]; //Receiving buffer
uchar IrRecAddr1; //Infrared address code
uchar IrRecAddr2;
uchar IrRecData1; //Infrared data code
uchar IrRecData2;
uint IrCycle = 0; //Infrared cycle
uint IrHigh = 0; //Duty cycle
const uchar IrCode[8]={0x01,0x02,0x04,0x08,0x10,0x20,0x40,0x80};
#define IrHeadLow_Max 2300 //2300*4 = 9200us
#define IrHeadLow_Min 2100 //2100*4 = 8400us
//--------Boot code high level range------------------
#define IrHeadHigh_Max 1250 //1250*4 = 5000us
#define IrHeadHigh_Min 1050 //1050*4 = 4200us
//--------Data 0 period range-------------------
#define IrData00_Max 300 //300*4 = 1200us
#define IrData00_Min 260 //260*4 = 1040us
//--------Data 1 period range-------------------
#define IrData10_Max 580 //580*4 = 2320us
#define IrData1_Min 400 //400*4 = 1600us
//--------Data 1 duty cycle (high level) range---------
#define IrData1_Max 50 //50*4 = 1760us
#define IrData1_Min 200 //200*4 = 1600us
//--------Data 2 duty cycle (high level) range---------
#define IrData1_Max 440 //440*4 = 1760us
#define IrData1_Min 300 //300*4 = 1600us
//----------------------------------------------------------
#define REMOTE_CLK_DIV 63 //63+1=64----16Mh/64=4us
#define REMOTE_ICF_FILTER 0x30
void Delay_ms( uint ms )
{
uint i,j;
for( j=0; j {
for( i=0; i<1000; i++ )
{;}
}
}
void Ir_Init(void)
{
PC_DDR_DDR1 = 0; //PC1 is input
PC_CR1_C11 = 1;
PC_CR2_C21 = 0;
}
void Ir_PWM_Init(void)
{
TIM1_PSCRH = 0;
TIM1_PSCRL = REMOTE_CLK_DIV;
TIM1_CCMR1 |= (REMOTE_ICF_FILTER + 0x01); // ch1 to ti1fp1
TIM1_CCER1 &= 0xec; //ch1 rising edge trigger
TIM1_CCMR2 |= (REMOTE_ICF_FILTER + 0x02); // ch2 selects ti1fp2
TIM1_CCER1 |= 0x20; //ch1 falling edge triggers ic2
TIM1_SMCR |= 0x54; //Select source trigger source and trigger mode reset
TIM1_CCER1 |= 0x11; //Enable input capture 1, 2
TIM1_CR1 |= 0x05;
}
void Ir_Receive(void)
{
switch(IrRecStep)
{
case 0:
IrIndex = 0;
if ((TIM1_SR1_CC1IF == 1)&&(TIM1_SR1_CC2IF == 1))
{
IrHigh = (uint)(TIM1_CCR2H);
IrHigh = (IrHigh << 8) + TIM1_CCR2L; //IrHigh duty cycle
IrCycle = (uint)(TIM1_CCR1H);
IrCycle = (IrCycle << 8) + TIM1_CCR1L; //IrCycle period
//Is it within the low level range of the boot code
if (((IrCycle - IrHigh) < IrHeadLow_Max)&&((IrCycle - IrHigh) > IrHeadLow_Min))
{
IrRecStep = 1;
TIM1_SR1_CC1IF = 0;
TIM1_SR1_CC2IF = 0;
TIM1_SR2_CC1OF = 0;
TIM1_SR2_CC2OF = 0; TIM1_SR1_UIF =
0;
IrRecBuff[0] = 0;
IrRecBuff[1] = 0;
IrRecBuff[2] = 0;
IrRecBuff[3] = 0;
}
}
break;
case 1:
if ((TIM1_SR2_CC1OF==1) || (TIM1_SR2_CC2OF==1) || (TIM1_SR1_UIF==1))
{
IrRecStep = 0;
}
else
{
if ((TIM1_SR1_CC1IF == 1)&&(TIM1_SR1_CC2IF == 1))
{
IrHigh = (uint)(TIM1_CCR2H);
IrHigh = (IrHigh << 8) + TIM1_CCR2L;
IrCycle = (uint)(TIM1_CCR1H);
IrCycle = (IrCycle << 8) + TIM1_CCR1L;
//Is it within the boot code high level range
if ((IrHigh < IrHeadHigh_Max)&&(IrHigh > IrHeadHigh_Min))
{
IrRecStep = 2;
}
}
}
break;
case 2:
if ((TIM1_SR2_CC1OF==1) || (TIM1_SR2_CC2OF==1) || (TIM1_SR1_UIF==1))
{
IrRecStep = 0;
}
else
{
if ((TIM1_SR1_CC1IF == 1)&&(TIM1_SR1_CC2IF == 1))
{
IrHigh = (uint)(TIM1_CCR2H);
IrHigh = (IrHigh << 8) + TIM1_CCR2L;
IrCycle = (uint)(TIM1_CCR1H);
IrCycle = (IrCycle << 8) + TIM1_CCR1L;
//Is the cycle within the range of data 0
if ((IrCycle > IrData00_Min)&&(IrCycle < IrData00_Max))
{
//Is the high level within the range of data 0
if ((IrHigh > IrData0_Min)&&(IrHigh < IrData0_Max))
{
IrIndex++;
}
else
{
IrRecStep = 0;
}
}
//Is the cycle within the range of data 1
else if ((IrCycle > IrData10_Min)&&(IrCycle < IrData10_Max))
{
//Is the high level within the range of data 1
if ((IrHigh > IrData1_Min)&&(IrHigh < IrData1_Max))
{
IrRecBuff[IrIndex >> 3] |= IrCode[IrIndex & 0x07];
IrIndex++;
}
else
{
IrRecStep = 0;
}
}
else
{
IrRecStep = 0;
}
if(IrIndex >= 32)
{
IrRecStep = 0;
IrRecFlag = 1;
}
}
}
break;
}
}
//Clock initialization
void CLK_Init(void)
{
CLK_ECKR=0x03; //External clock register external clock is ready, external clock is on
CLK_SWCR=0x02; //Switch control register enables switching mechanism
CLK_SWR=0xB4; //Master clock switch register selects HSE as master clock source*/
CLK_CSSR=0x01; //Clock security system register
}
//Initialization
void Devices_Init(void)
{
Delay_ms(200);
CLK_Init();
Ir_Init();
Ir_PWM_Init();
void main( void )
{
Devices_Init();
while(1)
{
Ir_Receive();
if(IrRecFlag == 1)
{
IrRecAddr1 = IrRecBuff[0];
IrRecAddr2 = IrRecBuff[1];
IrRecData1 = IrRecBuff[2];
IrRecData2 = IrRecBuff[3];
IrRecFlag = 0;
}
}
}
Previous article:stm8s development (nine) use of EEPROM: use EEPROM to store data!
Next article:LED intelligent lighting control system based on IOT
Recommended ReadingLatest update time:2024-11-16 16:19
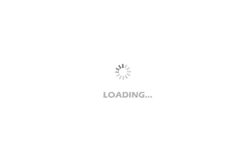
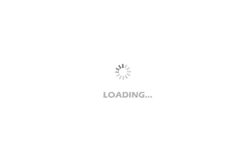
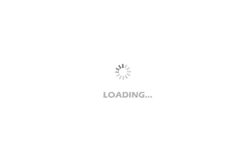
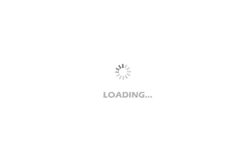
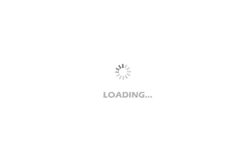
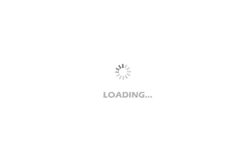
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
-
Intelligent Control Technology of Permanent Magnet Synchronous Motor (Written by Wang Jun)
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- The pitfalls of first contact with sensortile.box
- Award-winning live broadcast: Hidden costs of isolation system design
- UA level constant current source output chip
- Issues with changing MAC when batch flashing blueNRG-1 with BlueNRG-X Flasher Utility
- EEWORLD University Hall ---- Lao Wu's MCU Practice_NO.1 Project Practice
- [NXP Rapid IoT Review] + Rapid IoT Studio online IDE
- I'm studying BQ76940 recently and want to develop a BMS. I've been looking for information and encountered some questions during the process.
- [Sipeed LicheeRV 86 Panel Review] 4. Building a cross-compilation environment
- 【GD32E231 DIY Contest】4. Achieve 60-second timing
- 【DIY Creative LED V2】V2 version