6.1 ADC structure and register description
The main function of the analog-to-digital converter (ADC) is to convert analog signals into digital signals for data processing by the microcontroller.
ADC is divided into successive comparison type, double integration type and ∑-∆ type according to the conversion principle.
The successive approximation ADC converts analog signals into digital signals through successive comparisons. It has a fast conversion speed but low accuracy and is the most commonly used ADC.
The dual-integral ADC converts analog signals into digital signals by integrating twice. It has high accuracy and strong anti-interference ability, but it is slow. It is mainly used in measuring instruments such as multimeters.
∑-∆ ADC has the dual advantages of successive approximation and dual integration, and is gradually being widely used.
The STM32ADC is a 12-bit successive comparator with up to 18 channels. It can measure 16 external and 2 internal signal sources. The conversion of each channel can be performed in word, continuous, scan or discontinuous mode. The conversion result can be stored in a 16-bit data register in left-aligned or right-aligned mode.
The STM32ADC analog watchdog feature allows the application to detect if the input voltage exceeds the user-defined high/low thresholds
The input clock must not exceed 14MHz and is generated by dividing PCLK2
The STM32ADC is mainly composed of analog multiplexers, analog-to-digital converters, data registers, and trigger selection.
The conversion channels are divided into two groups: regular channels and injection channels.
Regular channels consist of up to 16 channels, converted in sequence
The injection channel consists of up to 4 channels, which can be inserted into the conversion
GPIO pins used by ADC
Channel 16 of ADC1 is internally connected to the temperature sensor
Channel 17 is internally connected to the reference power supply VREFINT
The ADC operates through 20 registers
6.2 ADC Design Example
6.2.1 Using ADC1 regular channel to realize analog-to-digital conversion of external analog signals
Registers and their contents related to regular channels
ADC regular channel initialization subroutine
//ADC1 initialization subroutine
void Adc1_Init(void)
{
RCC->APB2ENR |= 1<<3; //Turn on GPIOB clock
RCC->APB2ENR |= 1<<9; //Turn on ADC1 clock
GPIOB->CRL &=0xffffff00; //PB.01 (IN9), PB.00 (IN8) analog input
ADC1->CR1 |= 1<<8; //Scan mode
ADC1->CR2 |= 1<<20; //Regular channel external trigger: TIM1_CH1
ADC1->SQR1 |= 1<<20; //Regular channel sequence length: 2
ADC1->SQR3 |= 9; //1st conversion channel: IN9
ADC1->SQR3 |= 8<<5; //2nd conversion channel: IN8
ADC1->CR2 |= 1; //Turn on ADC
ADC1->CR2 |= 1<<2; //Calibrate ADC
while(ADC1->CR2 & 1<<2); //Wait for calibration to complete
}
ADC regular channel processing subroutine
//ADC1 processing subroutine
void Adc1_Proc(void)
{
if(ADC1->SR & 2) //EOC=1 (conversion ends)
{
adc1_dat[adc1_num] = ADC1->DR; //Read conversion value
if(++adc1_num == 2)
adc1_num = 0;
}
}
6.2.2 Using ADC1 injection channel to measure the temperature of the internal temperature sensor
There is a temperature sensor in STM32, which is connected to channel 16 of ADC1 and can be used to measure the temperature of the chip
The maximum sampling time of the temperature sensor is 17.1ms, the temperature range is -40~125°C, and the temperature calculation formula is as follows:
Where: V is the voltage value of the temperature sensor, N is the digital value after analog-to-digital conversion
Injection channel related registers and their contents
ADC injection channel initialization subroutine
//ADC1 initialization subroutine
void Adc1_Init(void)
{
RCC->APB2ENR |= 1<<3; //Turn on GPIOB clock
RCC->APB2ENR |= 1<<9; //Turn on ADC1 clock
GPIOB->CRL &=0xffffff00; //PB.01 (IN9), PB.00 (IN8) analog input
ADC1->CR1 |= 1<<8; //Scan mode
ADC1->CR2 |= 1<<20; //Regular channel external trigger: TIM1_CH1
ADC1->SQR1 |= 1<<20; //Regular channel sequence length: 2
ADC1->SQR3 |= 9; //1st conversion channel: IN9
ADC1->SQR3 |= 8<<5; //2nd conversion channel: IN8
ADC1->CR2 |= 1<<10; //Injection channel automatic conversion
ADC1->CR2 |= 1<<23; //Turn on the temperature sensor
ADC1->SMPR1 |= 5<<18; //SMP16[2:0] =5(55.5)
// (55.5+12.5)/4MHz=17s
ADC1->JSQR |= 0x10<<15; //JSRR[4:0]=0x10 (channel 16)
ADC1->CR2 |= 1; //Turn on ADC
ADC1->CR2 |= 1<<2; //Calibrate ADC
while(ADC1->CR2 & 1<<2); //Wait for calibration to complete
}
ADC injection channel processing subroutine
//ADC1 processing subroutine
void Adc1_Proc(void)
{
if(ADC1->SR & 2) //EOC=1 (conversion ends)
{
adc1_dat[adc1_num] = ADC1->DR; //Read conversion value
if(++adc1_num == 2)
adc1_num = 0;
}
if(ADC1->SR & 4) //JEOC=1 (injection channel conversion ends)
{
ADC1->SR &= ~6; //Clear JEOC and EOC
adc1_jdat = ADC1->JDR1; //Read conversion value
}
}
Previous article:51 MCU: Use ADC0832 to do analog-to-digital conversion and measure voltage
Next article:51 MCU Notes
Recommended ReadingLatest update time:2024-11-22 20:30
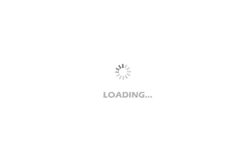
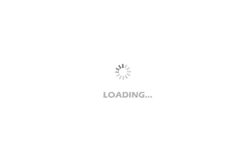
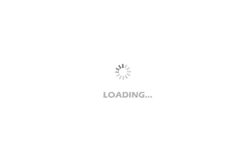
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Another technical solution for power-type plug-in hybrid: A brief discussion on Volvo T8 plug-in hybrid technology
- Share a video explanation by a senior hardware engineer - 3C certification surge and voltage drop test standards - the key is free
- MM32F031 Development Board Review 2: Unboxing Photos and STLINK Download
- [Qinheng Trial] CH559EVT Trial 3: Flowing Water Light
- ST MEMS Device Resource Library - State Machine (FSM) and Machine Learning (MLC) Functions [LSM6DSOX]
- Today's live broadcast: Typical applications of ADI switch/multiplexer series products
- [RVB2601 Creative Application Development] A record of the development board repair process
- [Analog Electronics Course Selection Test] + Basic Knowledge of Operational Amplifiers
- ARM9 programming STM32F030 microcontroller problem
- [Repost] Useful information: One article teaches you how to understand inductors
- Research and Design of SDTVHDTV Conversion Based on FPGA