1. Download multiple versions of EWstm8
IAR EWSTM8 series tutorial 01_IAR introduction, download, installation and registration - Zhihu https://zhuanlan.zhihu.com/p/42499895
Download address: https://pan.baidu.com/s/1slF5kYx#list/path=%2F https://pan.baidu.com/s/1slF5kYx#list/path=%2F
2. Download the standard library
stm8s standard firmware library (STSW-STM8069) download, http://www.st.com/web/en/catalog/tools/PF258009
3. Getting started
stm8 development environment configuration and testing - Xiaocao Guangming and Embedded - CSDN blog https://blog.csdn.net/xiaocaoguangming/article/details/9327977
STM8 Self-study Notes-002 STM8 Initial Use and Development Environment Establishment - weixin_43572492's Blog - CSDN Blog https://blog.csdn.net/weixin_43572492/article/details/85068875
Establish IAR project based on STM8 official standard library
1. Go to the ST official website or forum to download the STM8 standard peripheral library
2. Unzip the downloaded compressed package
3. Create an STM8 project template folder and copy related files
Create four files in the folder (this depends on personal preference). I like to create a project folder Project to store project files, a Library file to store library files, App to store user programs, and Doc to store documentation.
Copy the contents of the STM8S_StdPeriph_Driver file in the official library file Libraries to the newly created Library file, and copy main.c stm8s_conf.h stm8s_it.c and stm8s_it.h in the Template folder under the official Project file to the App folder.
4. Add groups and files to the project
Open IAR and select Project->Create New Project->ok, save the file to the Project folder; right-click the project and select Add Group, then add the App group and the Libraries group in turn.
Add files in App and Libraries folder. Add all *.c files in the src folder of the standard library in Libraries.
5. Project Settings
When choosing the specific model of STM8 microcontroller to be used, pay attention to the options of Code and Data. Take STM8S103K as an example.
Set the path of the compiler, which is the path to search for *.h. PROJDIR PROJ_DIRPROJ
DIR refers to the save path of the project file workspace, that is, the path of the *.eew file. …refers to the parent path, that is, the parent directory.
After understanding the above two points, include the inc path of the STM8 library file.
//Use relative path so that you don't have to reset the library function path when you move the program to another computer
$PROJ_DIR$..
$PROJ_DIR$..App
$PROJ_DIR$..Libraryinc
Then set the debugger mode to ST-Link. Now the settings are complete and you can compile the project.
Then you will see an error! ?
The error message is that the chip type is not set in the header file stm8s.h. Then go to set it - open stm8s.h to make changes and define the chip model. We choose stm8s103. Here you should choose according to the actual chip type.
Then compile again. Will you find that the error will still be reported! ? ?
This time the error message is that ADC2, CAN, etc. cannot be defined. Why does this happen? Let's continue to look at the stm8s.h file, which contains the instructions.
Here it says that after we define STM8S103, we will only define ADC1, not ADC2! ? In fact, because there is no ADC2 peripheral on the 8S103 chip, we can just remove the .c files that do not define ADC2, CAN, etc. from the Libraries group (the source files of all STM8S peripherals are added, but it depends on which peripherals the target MCU supports).
After removing it, there will be no error when compiling again. You can see a warning. That is because an official ST library function has export parameters but no return statement.
This is the end. Next, you can perform programming and debugging according to your needs.
4. 12 modes of GPIO in STM8
Original text: https://blog.csdn.net/weibo1230123/article/details/81204776
(1) GPIO_Mode_In_FL_No_IT: floating input without interrupt
(2) GPIO_Mode_In_PU_No_IT: Pull-up input without interrupt
(3) GPIO_Mode_In_FL_IT: Floating input has interrupt
(4) GPIO_Mode_In_PU_IT: Pull-up input has interrupt
(5) GPIO_Mode_Out_OD_Low_Fast: Output open-drain, low level, 10MHz
(6) GPIO_Mode_Out_PP_Low_Fast: Output push-pull, low level, 10MHz
(7) GPIO_Mode_Out_OD_Low_Slow: Output open-drain, low level, 2MHz
(8) GPIO_Mode_Out_PP_Low_Slow: Output push-pull, low level, 2MHz
(9) GPIO_Mode_Out_OD_HiZ_Fast: Output open-drain, high-impedance level, 10MHz
(10) GPIO_Mode_Out_PP_High_Fast: Output push-pull, high level, 10MHz
(11) GPIO_Mode_Out_OD_HiZ_Slow: Output open-drain, high-impedance level, 2MHz
(12) GPIO_Mode_Out_PP_High_Slow: Output push-pull, high level, 2MHz
---------------------
Channel 1 and channel 2 of tim2 of stm8 timer output PWM. Do the corresponding GPIOs need to be configured? How to configure them?
No configuration is required. You only need to set the PWM related registers and configure it to input PWM. It will automatically output PWM.
TIM1 and TIM2 of stm8 can output PWM. The output pin is the channel of TIM. You can use the library function to set
First configure the PWM frequency, then configure the PWM type, channel, and then enable the timer. If you need to use interrupts, you can also turn on interrupts.
/* Time base configuration */
TIM2_TimeBaseInit(TIM2_PRESCALER_1, 999); //2kHz
/* PWM1 Mode configuration: Channel1 */
TIM2_OC1Init(TIM2_OCMODE_PWM1, TIM2_OUTPUTSTATE_ENABLE,CCR1_Val, TIM2_OCPOLARITY_HIGH);
TIM2_OC1PreloadConfig(ENABLE);
/* TIM2 enable counter */
TIM2_Cmd(ENABLE);
5. If you need to output pwm, you also need to set the option byte
STM8S---IO multiplexing of option byte write operation - FreeApe - CSDN blog https://blog.csdn.net/FreeApe/article/details/47008033
/* MAIN.C file
Functons: Operate the option byte, set IO multiplexing, and modify the PWM output pin PA3 or PD2 of the CH3 channel of TIM2
Date : July 22, 2015
Author : yicm
Notes :
*/
#include void CLK_init(void) { CLK_ICKR |= 0X01; //Enable internal high-speed clock HSI CLK_CKDIVR = 0x08; //16M internal RC is divided by 2 and the system clock is 8M while(!(CLK_ICKR&0x02)); //HSI is ready CLK_SWR=0xe1; //HSI is the main clock source } void Init_GPIO(void) { /*Set to push-pull output, PD2 is connected to LED light*/ PD_DDR |= 0X04; //Set PD2 port to output mode PD_CR1 |= 0X04; //Set PD2 port to push-pull output mode PD_CR2 &= 0XFD; PA_DDR |= 0X08; //Set PA3 port to output mode PA_CR1 |= 0X08; //Set PA3 port to push-pull output mode PA_CR2 |= 0XF7; } void Init_Tim2(void) { TIM2_CCMR3 |= 0X70; //Set the output comparison mode of timer 2 three channels (PD2) TIM2_CCMR3 |= 0X04; //Output compare 3 preload enable TIM2_CCER2 |= 0x03; //Channel 3 is enabled, low level is valid, and it is configured as output // Initialize the clock divider to 1, that is, the clock frequency of the counter is Fmaster=8M/64=0.125MHZ TIM2_PSCR = 0X07; // Initialize the auto-load register to determine the frequency of the PWM square wave, Fpwm=0.125M/62500=2HZ TIM2_ARRH = 62500/256; TIM2_ARRL = 62500%256; // Initialize the comparison register to determine the duty cycle of the PWM square wave: 5000/10000 = 50% TIM2_CCR3H = 31250/256; TIM2_CCR3L = 31250%256; //Start counting; Update interrupt disable TIM2_CR1 |= 0x81; //TIM2_IER |= 0x00; } void Write_Option_Byte(void) { unsigned char opt[6] = {0,0,0x00,0,0,0}; /*Unlock Flash*/ do { FLASH_DUKR = 0xAE; FLASH_DUKR = 0x56; } while(!(FLASH_IAPSR & 0X08)); /*Enable write operation to option bytes*/ FLASH_CR2 = 0X80; /*Complementary control register*/ FLASH_NCR2 = 0X7F; /*Write operation, 0x02: PD2. 0x00: PA3*/ *((unsigned char *)0x4800) = opt[0]; *((unsigned char *)0x4801) = opt[1]; *((unsigned char *)0x4802) = ~opt[1]; *((unsigned char *)0x4803) = opt[2]; *((unsigned char *)0x4804) = ~opt[2]; *((unsigned char *)0x4805) = opt[3]; *((unsigned char *)0x4806) = ~opt[3]; *((unsigned char *)0x4807) = opt[4]; *((unsigned char *)0x4808) = ~opt[0]; *((unsigned char *)0x4809) = opt[5]; *((unsigned char *)0x480A) = ~opt[5]; /*Wait for writing to complete*/ while(!(FLASH_IAPSR & 0x04)); } main() { int i; Write_Option_Byte(); //When running the program, shield for(i=0;i<10000;++i); //Delay effect. Sometimes adding a delay can make the erase and application program work without blocking at the same time. CLK_init(); //Shield during erase, otherwise an error will occur during the next stlink simulation Init_GPIO(); //Shield during erase, otherwise an error will occur during the next stlink simulation Init_Tim2(); //Shield during erase, otherwise an error will occur during the next stlink simulation while (1); } 6. Modify option bytes through IAR STM8 Option Byte Configuration in IAR - STM8 - Forum - STMicroelectronics STM32/STM8 Technology Community http://www.stmcu.org.cn/module/forum/thread-607140-1-1.html 1. Select your debugging tool in the project Options... Debugger. After selecting, the corresponding debugging tool menu will appear in the IAR menu bar. As shown in the figure below, I have selected ST_LINK here. 2. Select the Option Bytes option in the ST_LINK menu shown in the figure above to enter the setting interface shown in the figure below. 3. Modify the options to be set by right-clicking, save and remember the saved path. 4. Open the project Options... dialog box again as shown in the figure below, and select the debugging tool option you are using. I use ST-LINK. Check Use option byte configuration and add the Option Bytes settings file you just saved. You can use an absolute path or a relative path when adding a settings file. The absolute path shown in the figure is recommended to use a relative path such as $PROJ_DIR$settingsobcf.obc. 5. Enter the program simulation debugging, select the OPTION option in the Memory viewing window, and you can see the option bytes configuration results as shown in the figure below.
Previous article:STM8 firmware library + IAR --CLK
Next article:Detailed explanation of STM32 firmware library
Recommended ReadingLatest update time:2024-11-16 13:49
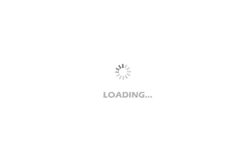
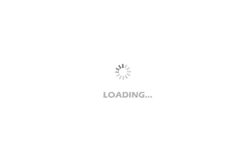
- Popular Resources
- Popular amplifiers
-
STM8 C language programming (1) - basic program and startup code analysis
-
Description of the BLDC back-EMF sampling method based on the STM8 official library
-
CVPR 2023 Paper Summary: Robotics
-
STM32 MCU project example: Smart watch design based on TouchGFX (8) Associating the underlying driver with the UI
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- I called Knowles today and it was hilarious.
- Is there any sensor that can detect whether there is someone in front through the tinted glass?
- Should the filter capacitor and bleeder resistor in the circuit be placed before or after the anti-reverse polarity diode?
- Which teacher can explain this circuit?
- [2022 Digi-Key Innovation Design Competition] 1. STM32F7508-DK Unboxing
- Some predictions about the national competition questions - about power supply questions
- RFID low frequency and high frequency antenna technology
- First look at Texas Instruments' C2000 series
- What is UWB and why is it in my phone? Ultra-wideband technology, explained
- Can the TF card that comes with SensorTile.box be used?