The PWM DC motor speed regulation simulation schematic diagram is as follows.
The PWM DC motor speed regulation schematic diagram and PCB diagram drawn by Altium Designer are as follows:
The PWM DC motor speed control microcontroller program is as follows
#include #include #include "LCD1602.h" #define uchar unsigned char #define uint unsigned int /**********Port Assignment***********************************/ sbit add_key=P1^0; //duty cycle increase sbit dec_key=P1^1; //duty cycle reduction sbit z_key=P1^2; //Forward key sbit f_key=P1^3; //Reverse key sbit run_key=P1^4; //Start/Stop key sbit beep=P3^2; //Motor drive related sbit ENA=P3^5; sbit IN1=P3^7; sbit IN2=P3^4; /**********Variable definitions*******************************/ //Motor related definitions bit Rotation_f=0; //Stepper motor rotation status flag is 0 forward and 1 reverse bit power_flag=0; //Master switch flag #define PWM_cnt_LEN 100 //Count full length #define PWM_ON 1 //On #define PWM_OFF 0 //Off uint PWM_cnt; //PWM count uchar PWM_duty; //PWM duty cycle uint speed_cnt=0; //speed uint speed_value; bit dis_speed_flag=0; uint delay_cnt; uchar dis_buf[6]; uchar dis_cnt; uchar dis_buf1[4]; uchar dis_cnt1; /************Define 1ms delay subroutine****************************/ void delay1ms(uint t) //1ms delay subroutine { uint k,j; for(k=0;k } /**********************************************************/ /**********Buzzer alarm**********************/ void beep_alarm() { beep=0; delay1ms(100); beep=1; } /***********************************************/ /**********Motor stops************************/ void motor_stop() { IN1=1; IN2=1; } /*******************************************/ /*******Motor rotates forward************************/ void motor_foreward() { IN1=1; IN2=0; } /****************************************/ /********Motor reverse ****************************/ void motor_reversal() { IN1=0; IN2=1; } /************************************************/ /************Display speed*********************/ void dis_speed() { dis_cnt=0; if(speed_value/10000!=0) { dis_buf[dis_cnt]=speed_value/10000+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%10000/1000+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%1000/100+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%100/10+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%10+0x30; dis_cnt++; } else if(speed_value%10000/1000!=0) { dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=speed_value%10000/1000+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%1000/100+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%100/10+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%10+0x30; dis_cnt++; } else if(speed_value%1000/100!=0) { dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=speed_value%1000/100+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%100/10+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%10+0x30; dis_cnt++; } else if(speed_value%100/10!=0) { dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=speed_value%100/10+0x30; dis_cnt++; dis_buf[dis_cnt]=speed_value%10+0x30; dis_cnt++; } else { dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=' '; dis_cnt++; dis_buf[dis_cnt]=speed_value%10+0x30; dis_cnt++; } dis_buf[dis_cnt]=''; LCD1602_Print(2,0,dis_buf); //Display speed } /************************************************/ /**********Display duty cycle*********************/ void dis_duty() { dis_cnt1=0; if(PWM_duty/100!=0) { dis_buf1[dis_cnt1]=PWM_duty/100+0x30; dis_cnt1++; dis_buf1[dis_cnt1]=PWM_duty%100/10+0x30; dis_cnt1++; dis_buf1[dis_cnt1]=PWM_duty%10+0x30; dis_cnt1++; } else if(PWM_duty%100/10!=0) { dis_buf1[dis_cnt1]=' '; dis_cnt1++; dis_buf1[dis_cnt1]=PWM_duty%100/10+0x30; dis_cnt1++; dis_buf1[dis_cnt1]=PWM_duty%10+0x30; dis_cnt1++; } else { dis_buf1[dis_cnt1]=' '; dis_cnt1++; dis_buf1[dis_cnt1]=' '; dis_cnt1++; dis_buf1[dis_cnt1]=PWM_duty%10+0x30; dis_cnt1++; } dis_buf1[dis_cnt1]=''; LCD1602_Print(9,1,dis_buf1); //Display duty cycle } /************************************************/ /*************Timer initialization procedure***********************/ void timer_init(void) { TMOD=0x11; //Set timer 0, 1 to 16-bit mode TH0=0xD8; //10ms timing initial value 10ms D8F0 TL0=0xF0; ET0=1; //Timer 0 is disconnected TR0=1; //Start timer 0 TH1=0xFF; //100us timing initial value TL1=0x9C; ET1=1; //Timer 1 disconnected TR1=1; //Start timer 1 EA=1; // total disconnect } /********************************************************/ /***********Timer 0 interrupt service routine*******************/ void timer0(void) interrupt 1 { TH0=0xD8; //10ms timing initial value 10ms D8F0 TL0=0xF0; delay_cnt++; if(delay_cnt>=200) //2S timing { delay_cnt=0; speed_value=speed_cnt*30; //30 Multiply the count in two seconds by 30 to get the revolutions per minute (also divided by 2 because there are two magnets) 30/2=15 speed_cnt=0; dis_speed_flag=1; } } /********************************************************/ /**********External interrupt 1 service routine**************/ void int1() interrupt 2 { speed_cnt++; //speed } /************************************************/ /***********Timer 1 interrupt service routine***********************/ void timer1(void) interrupt 3 { TH1=0xFF; //100us timing initial value TL1=0x9C; if(++PWM_cnt==PWM_cnt_LEN) { PWM_cnt=0; if(power_flag==1) ENA=PWM_ON; else ENA=PWM_OFF; } if(PWM_cnt==PWM_duty) { ENA=PWM_OFF; } } /********************************************************/ /*******Key processing procedure**********************************/ void key_check() { if(add_key==0) //duty cycle increase { delay1ms(50); if(add_key==0) { beep_alarm(); //Buzzer alarm PWM_duty++; if(PWM_duty>100) PWM_duty=100; dis_duty(); //Display duty cycle } } if(dec_key==0) //duty cycle reduction { delay1ms(50); if(dec_key==0) { beep_alarm(); //Buzzer alarm PWM_duty--; if(PWM_duty>100) PWM_duty=0; dis_duty(); //Display duty cycle } } if(z_key==0) //Forward key { delay1ms(50); if(z_key==0) { beep_alarm(); //Buzzer alarm Rotation_f=0; //Stepper motor rotation status flag is 0 forward and 1 reverse LCD1602_Print(12,0,"pros"); motor_stop(); //motor stops delay1ms(1); motor_foreward(); //Motor forward } } if(f_key==0) //Reverse key { delay1ms(50); if(f_key==0) { beep_alarm(); //Buzzer alarm Rotation_f=1; //Stepper motor rotation status flag is 0 forward and 1 reverse LCD1602_Print(12,0,"cons"); motor_stop(); //motor stops delay1ms(1); motor_reversal(); //Motor reversal } } if(run_key==0) //Start/Stop key { delay1ms(50); if(run_key==0) { beep_alarm(); //Buzzer alarm power_flag=~power_flag; //Master switch flag if(power_flag==1) { if(Rotation_f==0) //Stepper motor rotation status flag is 0 forward and 1 reverse { motor_stop(); //motor stops delay1ms(1); motor_foreward(); //Motor forward } else { motor_stop(); //motor stops delay1ms(1); motor_reversal(); //Motor reversal } } else motor_stop(); //motor stops
Previous article:4*4 matrix button "row and column inversion method" scans and displays 0-f in sequence
Next article:Tracking and obstacle avoidance remote control car based on STC15 single chip microcomputer
Recommended ReadingLatest update time:2024-11-16 13:31
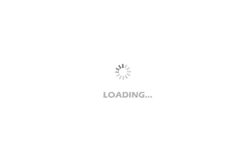
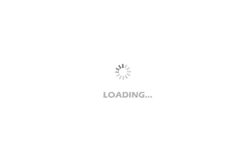
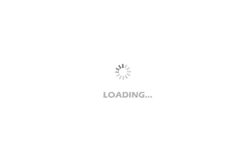
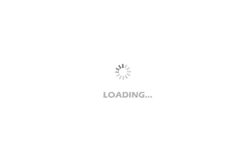
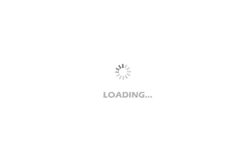
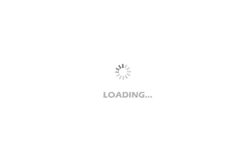
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- #idlemarket# MM32 development board exchange open source development board
- Forum Prize: Texas Instruments TI-36X Pro Scientific Calculator Disassembly and Analysis
- Why does Kalman filter need to do the prediction step?
- Misiqi's project cooperation based on ESP32 development board IOT Bluetooth WIFI
- Is this a circuit that can prevent the MCU from hanging?
- The principle and method of generating multiple PWM waveforms using one timer
- The impact of 5G on positioning technology
- Why is the output waveform of Quartus 13.0 always at a low level?
- A comprehensive look at the entire family of MSP430 microcontrollers
- CircuitPython upgraded to 5.2.0