GNU Inline Assembler
Inline assembly is to use assembly statements directly in C for programming, so that the program can implement some tasks that cannot be completed by C language in C program. For example, inline assembly or embedded assembly must be used in the following situations
Using Saturating Arithmetic in Programs
The program needs to operate the coprocessor
Complete the operation of the program status register in the C program
__asm__ __volatile__("asm code":output:input:changed registers);
Note:
Using __asm__ and __volatile__ means that the compiler will not check the following content, but will hand it directly to the assembler.
If you want to optimize the transformer, __volatile__ can be omitted
There is no asm code and "" cannot be omitted
There is no preceding or intermediate part, and the corresponding parts cannot be omitted:
There is no changed part and it must be omitted accordingly:
The final ; cannot be omitted. For C language, this is a statement.
The assembly code must be placed in a string, and you cannot press Enter to wrap the line in the middle of the string. You can write multiple strings. Note that there cannot be any symbols in the middle, which will merge the two strings into one.
There must be a line break between instructions, and you can also use t to keep the instructions neat in the assembly.
asm code
"mov r0, r0nt"
"mov r1,r1nt"
"mov r2,r2"
output(asm->C)
:"constraint" (variable)
"Constraint" is used to define the storage location of the variable:
r means use any available register
m indicates the memory address of the variable to be used
+Readable and writable
=Write Only
& means that the output operand cannot use the register used by the input part, and can only be used in the form of "+&" or "=&"
input(C->asm)
:"constraint" (variable/immediate)
"Constraint" is used to define the storage location of the variable:
r means use any available register (immediate values and variables are both OK)
m indicates the memory address of the variable to be used
i means using an immediate value
example
int a=100,b=200;
int result;
__asm__ __volatile__(
"mov %0,%3nt" //%0 is a placeholder, indicating result, and the same applies to the following
"ldr r0,%1nt"
"ldr r1,%2nt"
"str r0,%2nt"
"str %1,%1nt"
:"=r"(result),"+m"(a),"+m"(b)
:"i"(123)
);
ATPCS
Parameters are passed between subroutines through registers R0~R3. If there are more than four parameters, the extra parameters are passed using the stack. The called subroutine does not need to restore the contents of registers R0~R3 before returning.
In a subroutine, registers R4 to R11 are used to save local variables. If some registers in R4 to R11 are used in a subroutine, the values of these registers must be saved when the subroutine is entered, and the values of these registers must be restored before returning. For registers not used in the subroutine, these operations do not need to be performed. In a Thumb program, usually only registers R4 to R7 can be used to save local variables.
R12 is used as the scrtach register between subroutines (used to save SP, and used to pop the stack when the function returns), denoted as ip
R13 is used as the data stack pointer, denoted as sp
R14 is used as a link register, denoted as lr
R15 is recorded as the program register, denoted as pc
Mutual calls
When calling between C and assembly, special attention should be paid to complying with the corresponding ATPCS rules
C calling assembly
//.c
#include extern void strcopy(char* des, const char* src); int main(){ const char* srcstr = "src string"; char desstr[]="des string"; strcopy(desstr, srcstr); return 0; } ;.asm .global strcopy strcopy: ;R0 points to the destination string ;R1 points to the source string LDRB R2, [R1], #1 ; Load bytes and update source string pointer address STRB R2, [R0], #1 ;Store the season and update the destination string pointer address CMP R2, #0 ; Check if it is the end of the string BNE strcopy ;If not, the program jumps to strcopy to continue the loop MOV pc, ir ; program returns Assembly calls C //.c int fcn(int a, int b, int c, int d, int e){ return a+b+c+d+e; } ;.asm ; Assume that when the program enters f, the value in R0 is i ;int f(int i){return fcn(i, 2*i, 3*i, 4*i, 5*i);} .text .global _start _start: STR lr, [sp, #-4]! ;Save the return address lr ADD R1, R0, R0; calculate 2*i (the second parameter) ADD R2, R1, R0; calculate 3*i (the third parameter) ADD R3, R1, R2 ; calculate 5*i STR R3, [SP, #-4]! ; The fifth parameter is passed through the stack ADD R3, R1, R1; calculate 4*i (the 4th parameter) BL fcn ;Call C program ADD sp, sp, #4 ; remove the fifth parameter from the stack .end
Previous article:ARM assembler structure
Next article:ARM register analysis
Recommended ReadingLatest update time:2024-11-16 11:45
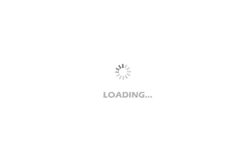
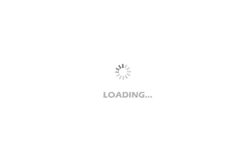
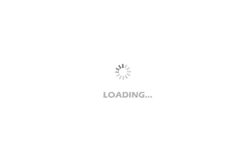
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- ADI Award-winning Live Broadcast: Things about Inertial MEMS Applications
- MM32F103 BUG reminder to avoid pitfalls
- Topology Selection of Bidirectional DCDC Converter
- [Second Batch of Shortlist] 2022 Digi-Key Innovation Design Competition
- Will MakeCode support Python?
- What are the specific differences between triodes and MOS tubes?
- Teach you how to design an accurate and thermally efficient wearable body temperature detection system?
- SimpleLink Ultra-Low-Power Wireless MCU for Bluetooth Smart Applications
- CC2540 Bluetooth Low Energy USB Dongle Reference Design
- Bode plot