Development board: FL2440
Core chip: S3C2440 (ARM9)
Ultrasonic module: HC-SR04
Working principle of ultrasonic module: There are four pins in total, VCC is connected to 5V, GND is connected to ground, Trig pin is connected to the high level transmitted by the chip through IO (duration is not less than 10 microseconds), and then the Echo pin outputs a high level for a period of time. The duration of the high level is the interval time from the ultrasonic wave is emitted to the echo is received.
The main purpose is to use the watchdog timer as a timer to calculate the duration of the high level returned by the Echo pin. The reset enable and interrupt enable should be turned off, and then the "dog" is given the maximum value. After a period of time, the current value is subtracted from the maximum value to get the consumed value, and then multiplied by the time interval of each subtraction of the "dog" (determined by setting the pre-division and division factors), which is the consumed time.
An important error occurred during the experiment: After the program was automatically run, the elapsed time was always 0, but the correct value could be obtained by using AXD single-step execution - Solution: Increase the time interval for each decrease of "dog".
Here is the procedure:
2440init.s
1 ;=========================================
2 ; NAME: 2440INIT.S
3 ; DESC: C start up codes
4; Configure memory, ISR, stacks
5 ; Initialize C-variables
6 ; HISTORY:
7 ; 2002.02.25:kwtark: ver 0.0
8 ; 2002.03.20:purnnamu: Add some functions for testing STOP,Sleep mode
9 ; 2003.03.14:DonGo: Modified for 2440.
10 ;=========================================
11
12 GET option.inc
13 GET memcfg.inc
14 GET 2440addr.inc
15
16 BIT_SELFREFRESH EQU (1<<22)
17
18 ;Pre-defined constants
19 USERMODE EQU 0x10
20 FIQMODE EQU 0x11
21 IRQMODE EQU 0x12
22 SVCMODE EQU 0x13
23 ABORTMODE EQU 0x17
24 UNDEFMODE EQU 0x1b
25 MODEMASK EQU 0x1f
26 NOINT EQU 0xc0
27
28;The location of stacks
29 UserStack EQU (_STACK_BASEADDRESS-0x3800) ;0x33ff4800 ~
30 SVCStack EQU (_STACK_BASEADDRESS-0x2800) ;0x33ff5800 ~
31 UndefStack EQU (_STACK_BASEADDRESS-0x2400) ;0x33ff5c00 ~
32 AbortStack EQU (_STACK_BASEADDRESS-0x2000) ;0x33ff6000 ~
33 IRQStack EQU (_STACK_BASEADDRESS-0x1000) ;0x33ff7000 ~
34 FIQStack EQU (_STACK_BASEADDRESS-0x0) ;0x33ff8000 ~
35
36 ;Check if tasm.exe(armasm -16 ...@ADS 1.0) is used.
37 GBLL THUMBCODE
38 [{CONFIG} = 16
39 THUMBCODE SETL {TRUE}
40 CODE32
41 |
42 THUMBCODE SETL {FALSE}
43 ]
44
45 MACRO
46 MOV_PC_LR
47 [ THUMBCODE
48 bx lr
49 |
50 mov pc,lr
51 ]
52 MEND
53
54 MACRO
55 MOVEQ_PC_LR
56 [ THUMBCODE
57 bxeq lr
58 |
59 moveq pc,lr
60 ]
61 MEND
62
63
64 MACRO
65 $HandlerLabel HANDLER $HandleLabel
66
67 $HandlerLabel
68 sub sp,sp,#4 ;decrement sp(to store jump address)
69 stmfd sp!,{r0} ;PUSH the work register to stack (lr does not push because it returns to original address)
70 ldr r0,=$HandleLabel;load the address of HandleXXX to r0
71 ldr r0,[r0] ;load the contents(service routine start address) of HandleXXX
72 str r0,[sp,#4] ;store the contents(ISR) of HandleXXX to stack
73 ldmfd sp!,{r0,pc} ;POP the work register and pc(jump to ISR)
74 MEND
75
76
77
78
79 IMPORT Main ; The main entry of mon program
80
81 AREA Init, CODE, READONLY
82
83 ENTRY
84
85 EXPORT __ENTRY
86 __ENTRY
87 ResetEntry
88 ;1)The code, which converts to Big-endian, should be in little endian code.
89 ;2)The following little endian code will be compiled in Big-Endian mode.
90; The code byte order should be changed as the memory bus width.
91 ;3)The pseudo instruction,DCD can not be used here because the linker generates error.
92 ASSERT :DEF:ENDIAN_CHANGE
93 [ ENDIAN_CHANGE
94 ASSERT :DEF:ENTRY_BUS_WIDTH
95
96
97 [ENTRY_BUS_WIDTH=16
98 andeq r14,r7,r0,lsl #20 ;DCD 0x0007ea00
99 ]
100
101 [ENTRY_BUS_WIDTH=8
102 streq r0,[r0,-r10,ror #1] ;DCD 0x070000ea
103 ]
104 |
105 b ResetHandler
106 ]
107 b HandlerUndef ;handler for Undefined mode
108 b HandlerSWI ;handler for SWI interrupt
109 b HandlerPabort ; handler for PAbort
110 b HandlerDabort ; handler for DAbort
111 b . ;reserved
112 b HandlerIRQ ;handler for IRQ interrupt
113 b HandlerFIQ ;handler for FIQ interrupt
114
115 ;@0x20
116 ; b EnterPWDN ; Must be @0x20.
117
118
119 HandlerFIQ HANDLER HandleFIQ
120 HandlerIRQ HANDLER HandleIRQ
121 HandlerUndef HANDLER HandleUndef
122 HandlerSWI HANDLER HandleSWI
123 HandlerDabort HANDLER HandleDabort
124 HandlerPabort HANDLER HandlePabort
125
126 IsrIRQ
127 sub sp,sp,#4 ;reserved for PC
128 stmfd sp!,{r8-r9}
129
130 ldr r9,=INTOFFSET
131 ldr r9,[r9]
132 ldr r8,=HandleEINT0
133 add r8,r8,r9,lsl #2
134 ldr r8,[r8]
135 str r8,[sp,#8]
136 ldmfd sp!,{r8-r9,pc}
137
138
139 LTORG
140
141 ;=======
142 ; ENTRY
143 ;=======
144 ResetHandler
145 ldr r0,=WTCON ;watch dog disable
146 ldr r1,=0x0
147 str r1,[r0]
148
149 ldr r0,=INTMSK
150 ldr r1,=0xffffffff ;all interrupt disable
151 str r1,[r0]
152
153 ldr r0,=INTSUBMSK
154 ldr r1,=0x7fff ;all sub interrupt disable
155 str r1,[r0]
156
157
158
159 ;To reduce PLL lock time, adjust the LOCKTIME register.
160 ldr r0,=LOCKTIME
161 ldr r1,=0xffffff
162 str r1,[r0]
163
164 [PLL_ON_START
165 ; Added for confirm clock divide. for 2440.
166 ; Setting value Fclk:Hclk:Pclk
167 ldr r0,=CLKDIVN
168 ldr r1,=CLKDIV_VAL ; 0=1:1:1, 1=1:1:2, 2=1:2:2, 3=1:2:4, 4=1:4:4, 5=1:4:8, 6=1:3:3, 7=1:3:6.
169 str r1,[r0]
170
171 ;program has not been copied, so use these directly,
172 [CLKDIV_VAL>1; means Fclk:Hclk is not 1:1.
173 mrc p15,0,r0,c1,c0,0
174 orr r0,r0,#0xc0000000;R1_nF:OR:R1_iA
175 mcr p15,0,r0,c1,c0,0
176 |
177 mrc p15,0,r0,c1,c0,0
178 bic r0,r0,#0xc0000000;R1_iA:OR:R1_nF
179 mcr p15,0,r0,c1,c0,0
180 ]
181
182 ;Configure UPLL
183 ldr r0,=UPLLCON
184 ldr r1,=((U_MDIV<<12)+(U_PDIV<<4)+U_SDIV)
185 str r1,[r0]
186 nop ; Caution: After UPLL setting, at least 7-clocks delay must be inserted for setting hardware be completed.
187 nop
188 nop
189 nop
190 nop
191 nop
192 nop
193 ;Configure MPLL
194 ldr r0,=MPLLCON
195 ldr r1,=((M_MDIV<<12)+(M_PDIV<<4)+M_SDIV) ;Fin=16.9344MHz
196 str r1,[r0]
197 ]
198
199 ;Check if the boot is caused by the wake-up from SLEEP mode.
200
201
202
203
204; ...
205 ;;;;;;;;;;;;; When EINT0 is pressed, Clear SDRAM
206; ...
207 ; check if EIN0 button is pressed
208
209
210 1 bl InitStacks
211
212
213
214
215 ;===========================================================
216 ; Setup IRQ handler
217 ldr r0,=HandleIRQ ;This routine is needed
218 ldr r1,=IsrIRQ ;if there is not 'subs pc,lr,#4' at 0x18, 0x1c
219 str r1,[r0]
220
221
222
223
224 [ :LNOT:THUMBCODE
225 bl Main ;Do not use main() because ......
226 b .
227 ]
228
229 [THUMBCODE ;for start-up code for Thumb mode
230 orr lr,pc,#1
231 bx lr
232 CODE16
233 bl Main ;Do not use main() because ......
234 b .
235 CODE32
236 ]
237
238
239 ;function initializing stacks
240 InitStacks
241; Do not use DRAM, such as stmfd, ldmfd......
242 ;SVCstack is initialized before
243 ;Under toolkit ver 2.5, 'msr cpsr,r1' can be used instead of 'msr cpsr_cxsf,r1'
244 mrs r0,cpsr
245 bic r0,r0,#MODEMASK
246 orr r1,r0,#UNDEFMODE|NOINT
247 msr cpsr_cxsf,r1 ;UndefMode
248 ldr sp,=UndefStack ; UndefStack=0x33FF_5C00
249
250 orr r1,r0,#ABORTMODE|NOINT
251 msr cpsr_cxsf,r1 ;AbortMode
252 ldr sp,=AbortStack ; AbortStack=0x33FF_6000
253
254 orr r1,r0,#IRQMODE|NOINT
255 msr cpsr_cxsf,r1 ;IRQMode
256 ldr sp,=IRQStack ; IRQStack=0x33FF_7000
257
258 orr r1,r0,#FIQMODE|NOINT
259 msr cpsr_cxsf,r1 ;FIQMode
260 ldr sp,=FIQStack ; FIQStack=0x33FF_8000
261
262 bic r0,r0,#MODEMASK|NOINT
263 orr r1,r0,#SVCMODE
264 msr cpsr_cxsf,r1 ;SVCMode
265 ldr sp,=SVCStack ; SVCStack=0x33FF_5800
266
267; USER mode has not been initialized.
268
269 mov pc,lr
270;The LR register will not be valid if the current mode is not SVC mode.
271
272 ;===========================================================
273
274
275
276
277
278
279
280
281 ;=====================================================================
282 ; Clock division test
283 ; Assemble code, because VSYNC time is very short
284 ;=====================================================================
285
286
287
288 ALIGN
289
290 AREA RamData, DATA, READWRITE
291
292 ^ _ISR_STARTADDRESS ; _ISR_STARTADDRESS = 0x33FF_FF00
293 HandleReset # 4
294 HandleUndef # 4
295 HandleSWI # 4
296 HandlePabort # 4
297 HandleDabort # 4
298 HandleReserved # 4
299 HandleIRQ # 4
300 HandleFIQ # 4
301
302 ;Do not use the label 'IntVectorTable',
303 ;The value of IntVectorTable is different with the address you think it may be.
304 ;IntVectorTable
305 ;@0x33FF_FF20
306 HandleEINT0 # 4
307 HandleEINT1 # 4
308 HandleEINT2 # 4
309 HandleEINT3 # 4
310 HandleEINT4_7 # 4
311 HandleEINT8_23 # 4
312 HandleCAM # 4 ; Added for 2440.
313 HandleBATFLT # 4
314 HandleTICK # 4
315 HandleWDT # 4
316 HandleTIMER0 #4
317 HandleTIMER1 #4
318 HandleTIMER2 #4
319 HandleTIMER3 # 4
320 HandleTIMER4 #4
321 HandleUART2 # 4
322 ;@0x33FF_FF60
323 HandleLCD #4
324 HandleDMA0 # 4
325 HandleDMA1 # 4
326 HandleDMA2 # 4
327 HandleDMA3 # 4
328 HandleMMC # 4
329 HandleSPI0 # 4
330 HandleUART1 #4
331 HandleNFCON # 4 ; Added for 2440.
332 HandleUSBD # 4
333 HandleUSBH # 4
334 HandleIIC # 4
335 HandleUART0 #4
336 HandleSPI1 #4
337 HandleRTC # 4
338 HandleADC # 4
339 ;@0x33FF_FFA0
340 END
2440addr.h
1 //ISR
2
3 //_ISR_STARTADDRESS EQU 0x33ffff00
4 #define _IRQ_BASEADDRESS 0x33ffff00
5 /*#define pISR_RESET (*(unsigned *)(_IRQ_BASEADDRESS+0x0))
6 #define pISR_UNDEF (*(unsigned *)(_IRQ_BASEADDRESS+0x4))
7 #define pISR_SWI (*(unsigned *)(_IRQ_BASEADDRESS+0x8))
8 #define pISR_PABORT (*(unsigned *)(_IRQ_BASEADDRESS+0xc))
9 #define pISR_DABORT (*(unsigned *)(_IRQ_BASEADDRESS+0x10))
10 #define pISR_RESERVED (*(unsigned *)(_IRQ_BASEADDRESS+0x14))
11 #define pISR_IRQ (*(unsigned *)(_IRQ_BASEADDRESS+0x18))
12 #define pISR_FIQ (*(unsigned *)(_IRQ_BASEADDRESS+0x1c))*/
13 #define pISR_WDT (*(unsigned *)(_IRQ_BASEADDRESS+0x44))
14
15
16 //UART0
17 #define rUTRSTAT0 (*(volatile unsigned *)0x50000010) //UART 0 Tx/Rx status
18 #define rULCON0 (*(volatile unsigned *)0x50000000) //UART 0 Line control
19 #define rUCON0 (*(volatile unsigned *)0x50000004) //UART 0 Control
Previous article:Serial port control FL2440 (S3C2440) LED
Next article:Use WinCE's precise timing function to output pwm signals to control the servo
Recommended ReadingLatest update time:2024-11-16 12:51
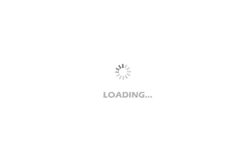
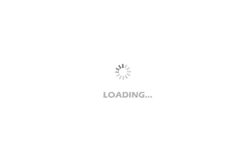
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Things to note when using peelable adhesive
- Last day! TI Live Broadcast with Prizes | Application of Precision ADC in Transmitters
- Renesas CPK-RA6M4 development board evaluation + SHT20 sensor reading data
- Share 2018 Electronics Competition Paper - [B- Fire Extinguishing Aircraft] Fujian Province Ti Cup Special Prize / Xiamen University / Changmen University Team
- Constant voltage and constant current power supply design
- About the difference between CC1312R LAUNCHPAD versions
- About the signal input mode of the power amplifier
- My Journey of MCU Development (Part 1)
- How to understand the accuracy parameter (1.5% + 3) of the Fluke F17B+ multimeter?
- Inverting proportional operational amplifier circuit