Based on the DHT11 temperature and humidity alarm system LCD1602 display operation instructions
This design system is a DHT11 temperature and humidity alarm system LCD1602 display, which can set the high temperature alarm value and low temperature alarm value of temperature, the high humidity alarm value and low temperature alarm value of humidity. When there is an alarm, the buzzer will emit a dripping alarm sound, and the green light-emitting diode will flash at the same time. The alarm information can also be seen very intuitively on the LCD1602 liquid crystal. It also has a power-off save function. The data is saved in the EEPOM inside the microcontroller. After entering the setting interface, if no key is pressed, the system will automatically exit the setting interface after 30 seconds. The humanized key setting, the key also has the function of continuous addition and subtraction. When the key is pressed, there is a key sound, that is, the buzzer calls. The key sound can be set in the menu, and the key sound can be set to turn on or off. The system also has a function to cancel the alarm return difference value to increase the anti-interference of the system.
Alarm mode: sound and light alarm
buzzer drips alarm sound, light-emitting diode flashes.
DHT11 temperature and humidity detection
microcontroller circuit schematic diagram:
Buzzer Buzzer B1
10uF capacitor C1
20pF capacitor C2, C3
D indicator D1, D2, D3, D4,
LCD1602 LCD1
9012 transistor Q1
10K resistor R3, R4
2K resistor R1
2K resistor R2, R5
SW-PB button S1, S2, S3, S4, S5
sw-gray power switch SW1
U1 MCU U1
DHT11 temperature and humidity sensor U2
12M crystal oscillator Y1
MCU source program is as follows:
#include #define uchar unsigned char //unsigned character macro definition variable range 0~255 #define uint unsigned int //Unsigned integer macro definition variable range 0~65535 sbit beep = P1^4; //Buzzer int flag_300ms; // Definition of IO variables for buttons uchar key_can; //Key value variable sbit wh_led = P2^2; // Temperature alarm indicator IO port definition sbit wl_led = P2^3; // Temperature alarm indicator IO port definition sbit sh_led = P2^1; //Humidity alarm indicator IO port definition sbit sl_led = P2^0; //Humidity alarm indicator IO port definition uchar flag_en = 1; uchar menu_1; //Menu design variables uint t_high = 35, t_low = 10; //temperature alarm parameters uint s_high = 80,s_low = 10; //Humidity alarm parameters /******************************************************************* * Name: delay_1ms() * Function: Delay 1ms function * Input: q * Output: None ********************************************************************/ void delay_1ms(uint q) { uint i,j; for(i=0;i } sbit dht11=P1^5; //Temperature sensor IO port definition uchar table_dht11[5]={1,2,3,4,5}; uchar code table_num[]="0123456789abcdefg"; sbit rs=P1^0; //Register selection signal H: data register L: instruction register sbit rw=P1^1; //Register selection signal H: data register L: instruction register sbit e =P1^2; //Chip select signal falling edge trigger /******************************************************************** * Name: delay_uint() * Function: small delay. * Input: None * Output: None ***************************************************************************/ void delay_uint(uint q) { while(q--); } /******************************************************************** * Name: write_com(uchar com) * Function: 1602 command function * Input: Input command value * Output: None ***************************************************************************/ void write_com(uchar com) { e=0; rs=0; rw=0; P0=com; delay_uint(25); e=1; delay_uint(100); e=0; } /******************************************************************** * Name: write_data(uchar dat) * Function: 1602 write data function * Input: Data to be written to 1602 * Output: None ***************************************************************************/ void write_data(uchar dat) { e=0; rs=1; rw=0; P0=dat; delay_uint(25); e=1; delay_uint(100); e=0; } /***********************LCD1602 displays specific characters****0XDF************************/ void write_zifu(uchar hang,uchar add,uchar date) { if(hang==1) write_com(0x80+add); else write_com(0x80+0x40+add); write_data(date); } /***********************LCD1602 displays two decimal numbers************************/ void write_sfm2(uchar hang,uchar add,uint date) { if(hang==1) write_com(0x80+add); else write_com(0x80+0x40+add); write_data(0x30+date/10%10); write_data(0x30+date%10); } /**************************LCD1602 displays this character function****************************/ void write_string(uchar hang,uchar add,uchar *p) { if(hang==1) write_com(0x80+add); else write_com(0x80+0x40+add); while(1) { if(*p == '') break; write_data(*p); p++; } } /***********************lcd1602 initialization settings****************************/ void init_1602() //lcd1602 initialization { write_com(0x38); write_com(0x0c); write_com(0x06); delay_uint(1000); write_string(1,0,"Wd:00 H00 L00 "); write_string(2,0,"Sd:00% H00% L00% "); write_zifu(1,5,0xdf); //display degree write_zifu(1,10,0xdf); //display degree write_zifu(1,15,0xdf); //display degree write_sfm2(1,3,table_dht11[2]); //Display temperature write_sfm2(2,3,table_dht11[0]); //Display humidity write_sfm2(1,7,t_high); //Display temperature write_sfm2(2,7,s_high); //Display humidity write_sfm2(1,10,t_low); //Display temperature write_sfm2(2,10,s_low); //Display humidity } /************************Independent key program*****************/ uchar key_can; //key value void key() //Independent key program { static uchar key_new; key_can = 20; //key value restoration P3 |= 0xf0; if((P3 & 0xf0) != 0xf0) //button pressed { delay_1ms(1); //key debounce if(((P3 & 0xf0) != 0xf0) && (key_new == 1)) { //Confirm that the button was pressed key_new = 0; switch(P3 & 0xf0) { case 0xd0: key_can = 1; break; //Get the k1 key value case 0xb0: key_can = 2; break; //Get the K2 key value case 0x70: key_can = 3; break; //Get the k3 key value } } } else key_new = 1; } /********************Button display function***************/ void key_with() { if(key_can == 1) //Set key { menu_1++; if(menu_1 > 2) { menu_1 = 0; init_1602(); //lcd1602 initialization } } if(menu_1 == 1) //Set high temperature alarm { if(key_can == 2) { t_high ++; //Set the high temperature value plus 1 if(t_high > 99) t_high = 99; } if(key_can == 3) { t_high -- ; //Set the high temperature value minus 1 if(t_high <= t_low) t_high = t_low + 1; } write_sfm2(1,8,t_high); //Display temperature write_com(0x80+8); //Move the cursor to the second position write_com(0x0f); //Display the cursor and blink } if(menu_1 == 2) //Set low temperature alarm { if(key_can == 2) { t_low ++; //Set the low temperature value plus 1 if(t_low >= t_high) t_low = t_high - 1; } if(key_can == 3) //Set the high temperature value minus 1 { t_low -- ; if(t_low <= 1) t_low = 1; } write_sfm2(1,13,t_low); //Display humidity write_com(0x80+13); //Move the cursor to the second position write_com(0x0f); //Display the cursor and blink } if(menu_1 == 3) //Set high humidity alarm { if(key_can == 2) { s_high ++ ; //Set the high humidity value plus 1 if(s_high > 99) s_high = 99; } if(key_can == 3) { s_high -- ; //Set the high humidity value minus 1 if(s_high <= s_low) s_high = s_low + 1; } write_sfm2(2,8,s_high); //Display humidity write_com(0x80+0x40+8); //Move the cursor to the second position write_com(0x0f); //Display the cursor and blink } if(menu_1 == 4) //Set low humidity alarm { if(key_can == 2) { s_low ++; //Set the low humidity value plus 1 for(j=0;j<120;j++);
Previous article:Single chip LCD12864 unlimited expansion multi-level menu source code
Next article:51 MCU CS1237 electronic scale source program with detailed comments
Recommended ReadingLatest update time:2024-11-16 16:49
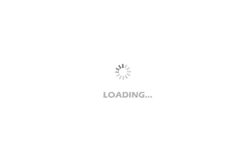
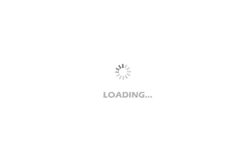
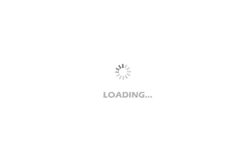
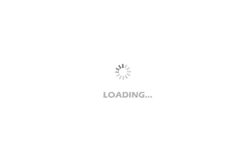
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- About C2000 program serial port upgrade
- Moderators and those who want to be moderators, please accept this "Moderator Strategy"
- What are the functions of the three buttons and three indicator lights?
- Teacher He Lei from Zhixin Technology explains the state machine
- Keysight Technologies Live Review: Oscilloscope Basics Training
- Remote control jog problem
- How to solve the problem of field effect tube heating when used as a switch
- How to configure slave device registers via SPI in tms57ls
- [RVB2601 Creative Application Development] 9~11 Game End Restart and Optimize Code, etc.
- Evaluation of domestic FPGA Gaoyun GW1N-4 series development board - stepper motor control (first edition)