ATMEGA16 written by AVR stdio controls the forward and reverse rotation and speed of the stepper motor. It is free for everyone to refer to and criticize.
It is suitable for beginners. There are seven buttons in total. Three buttons control three speeds of forward rotation, three buttons control three speeds of reverse rotation, and one button controls stop.
This program controls the stepper motor and is connected to the stepper motor driver, so there is only one pulse. If you want to connect the stepper motor directly, you only need to make a slight change.
Main program preview:
#define F_CPU 800000UL
#include #include #define INT8U unsigned int #define INT16U unsigned int const INT8U FFW[]={0x01,0x00,0x01,0x00,0x01,0x00,0x01,0x00,0x01,0x00,0x01,0x00,0x01,0x00,0x01,0x00,0x01,0x00}; // output 8 pulses from port p0 in one beat //Button definition #define K1_DOWN()((PIND &_BV(PD0)) == 0x00) //K1: forward speed 1 #define K2_DOWN()((PIND &_BV(PD1)) == 0x00) //K2: forward speed 2 #define K3_DOWN()((PIND &_BV(PD2)) == 0x00) //K3: forward speed 3 #define K4_DOWN()((PIND &_BV(PD3)) == 0x00) //K4: stop #define K5_DOWN()((PIND &_BV(PD4)) == 0x00) //K5: reverse speed 1 #define K6_DOWN()((PIND &_BV(PD5)) == 0x00) //K6: reverse speed 2 #define K7_DOWN()((PIND &_BV(PD6)) == 0x00) //K7: reverse speed 3 void STEP_MOTOR_FWRUN_1(INT8U n) { INT8U i,j; for(i=0;i for(j=0;j<16;j++)//loop output 16*8 beats { //if (K1_DOWN())break; if (K2_DOWN())break; if (K3_DOWN())break; if (K4_DOWN())return; //When K4 is pressed midway, the motor stops rotating if (K5_DOWN())break; if (K6_DOWN())break; if (K7_DOWN())break; PORTB=FFW[j]; _delay_us(50); } PORTB=0x01; //After the last circle, the output is 0x01 and the motor returns to the starting point } } void STEP_MOTOR_FWRUN_2(INT8U n) { INT8U i,j; for(i=0;i for(j=0;j<16;j++) { if (K1_DOWN())break; // if (K2_DOWN())break; if (K3_DOWN())break; if (K4_DOWN())return; //When K4 is pressed midway, the motor stops rotating if (K5_DOWN())break; if (K6_DOWN())break; if (K7_DOWN())break; PORTB=FFW[j]; _delay_us(150); } PORTB=0x01; //After the last circle, the output is 0x01 and the motor returns to the starting point } } void STEP_MOTOR_FWRUN_3(INT8U n) { INT8U i,j; for(i=0;i for(j=0;j<16;j++) { if (K1_DOWN())break; if (K2_DOWN())break; // if (K3_DOWN())break; if (K4_DOWN())return; //When K4 is pressed midway, the motor stops rotating if (K5_DOWN())break; if (K6_DOWN())break; if (K7_DOWN())break; PORTB=FFW[j]; _delay_us(300); } PORTB=0x01; //After the last circle, the output is 0x01 and the motor returns to the starting point } } void STEP_MOTOR_CWRUN_5(INT8U n) { INT8U i,j; for(i=0;i for(j=0;j<16;j++) { if (K1_DOWN())break; if (K2_DOWN())break; if (K3_DOWN())break; if (K4_DOWN())return; //When K4 is pressed midway, the motor stops rotating // if (K5_DOWN())break; if (K6_DOWN())break; if (K7_DOWN())break; PORTB=FFW[j]; _delay_us(300); } PORTB=0x01; //After the last circle, the output is 0x01 and the motor returns to the starting point } } void STEP_MOTOR_CWRUN_6(INT8U n) { INT8U i,j; for(i=0;i for(j=0;j<16;j++) { if (K1_DOWN())break; if (K2_DOWN())break; if (K3_DOWN())break; if (K4_DOWN())return; //When K4 is pressed midway, the motor stops rotating if (K5_DOWN())break; //if (K6_DOWN())break; if (K7_DOWN())break; PORTB=FFW[j]; //Forward when direction is 0 _delay_us(150); } PORTB=0x01; //After the last circle, the output is 0x01 and the motor returns to the starting point } } void STEP_MOTOR_CWRUN_7(INT8U n) { INT8U i,j; for(i=0;i for(j=0;j<16;j++) { if (K1_DOWN())break; if (K2_DOWN())break; if (K3_DOWN())break; if (K4_DOWN())return; //When K4 is pressed midway, the motor stops rotating if (K5_DOWN())break; if (K6_DOWN())break; // if (K7_DOWN())break; PORTB=FFW[j]; _delay_us(50); } PORTB=0x01; //After the last circle, the output is 0x01 and the motor returns to the starting point } } void beep() { PORTC=0x40; _delay_ms(120); PORTC=0X00; } void initonbeep() { PORTC=0x40; _delay_ms(120); PORTC=0X00; _delay_ms(120); PORTC=0x40; _delay_ms(120); PORTC=0X00; _delay_ms(120); PORTC=0x40; _delay_ms(120); PORTC=0X00; _delay_ms(120); PORTC=0x40; _delay_ms(120); PORTC=0X00; } int main() { INT8U r=65535; DDRB=0xFF;PORTB=FFW[0]; //control output DDRD=0X00;PORTD=0xFF; //Key input DDRA=0X00;PORTA=0xFF; //Direction signal DDRC=0XFF;PORTC=0x00; //Buzzer initonbeep(); while(1) { if(K1_DOWN()) { _delay_us(200); if(K1_DOWN()) { beep(); //while(K1_DOWN()); //Wait for K1 to be released PORTA=0x00; //direction signal is 0 STEP_MOTOR_FWRUN_1(r); //Click to rotate forward r circles } } if(K2_DOWN()) { _delay_us(200); if(K2_DOWN()) { beep(); //while(K1_DOWN()); //Wait for K1 to be released PORTA=0x00; //direction signal is 0 STEP_MOTOR_FWRUN_2(r); //Click to rotate forward r circles } } if(K3_DOWN()) { _delay_us(200); if(K3_DOWN()) { beep(); //while(K1_DOWN()); //Wait for K1 to be released PORTA=0x00; //direction signal is 0 STEP_MOTOR_FWRUN_3(r); //Click to rotate forward r circles } } if(K5_DOWN()) { _delay_us(200); if(K5_DOWN()) { beep(); //while(K2_DOWN()); //Wait for K2 to be released PORTA=0x01; //direction signal is 1 STEP_MOTOR_CWRUN_5(r); //Motor reverses r turns } } if(K6_DOWN()) { _delay_us(200); if(K6_DOWN()) { beep(); //while(K2_DOWN()); //Wait for K2 to be released PORTA=0x01; //direction signal is 1 STEP_MOTOR_CWRUN_6(r); //Motor reverses r turns } } if(K7_DOWN()) { _delay_us(200); if(K7_DOWN()) { beep(); //while(K2_DOWN()); //Wait for K2 to be released PORTA=0x01; //direction signal is 1 STEP_MOTOR_CWRUN_7(r); //Motor reverses r turns } } } }
Previous article:Mega128 waveform generator simulation and source program LCD display
Next article:Infrared control motor system source code based on ATmega128 microcontroller
Recommended ReadingLatest update time:2024-11-16 08:59
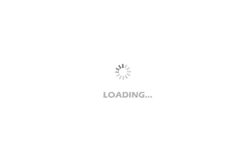
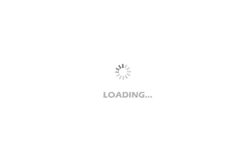
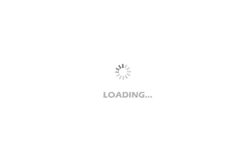
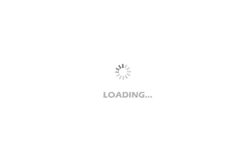
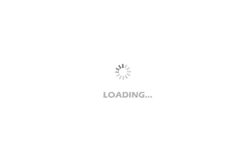
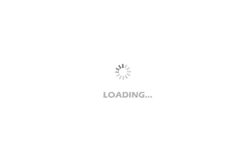
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Port the STM32F407 keil version program to IAR for ARM
- Reimagining Antenna Design Solutions for Next-Generation Mobile Devices
- Electronic skin
- IEEE 802.11 common technical standards
- Better than seeing! See how 77GHz millimeter-wave radar protects ADAS
- How to improve the power of voltage doubler rectifier circuit
- What do you think about the answers to the exercises?
- FAQ: Security Resilience of Platform Firmware | Microchip Security Solutions Seminar Series 8
- DAP Miniwiggler emulator usage issues
- Selection and application of transmitters in several special working conditions