What is a Serial Port
UART: Universal Asynchronous Receiver/Transmitter
USART: Universal Synchronous Asynchronous Receiver/Transmitter
One is the most commonly used and simplest serial data transmission protocol. Only two data lines are needed to achieve full-duplex.
Tx: Send data line
Rx: Receive data line
A B
TX -----------> Rx
Rx <------------Tx
Full-duplex: Two devices can send and receive at the same time
Serial data: Only one wire is used for transmission and only one bit can be sent at a time. One bit is sent and received one bit after another.
Module communication: upper computer lower computer
Communication generally requires two devices, which we call the upper computer and the lower computer.
Host computer: A machine with strong processing performance is called host computer. Most of the data processing is done on the host computer.
Lower computer: The terminal for data collection, a machine with single processing performance, is called the lower computer.
The serial port has only one data transmission line. If A wants to send a character data 10101010 to B
A -------- ---------
-------- ------- ...
How long is the high level period? Even if no data is sent, there is a level state on the Tx line.
How does it know you are sending? ....
How is UART data transmitted?
UART protocol serial port protocol.
The serial port sends and receives data in frames.
1 frame = 1 start bit + 5-9 bits of data + 0/1 bit of parity + stop bits (0.5, 1,1,5,2)
Start bit: one cycle of low level
Data bits: 5-9 bits. The exact number of bits needs to be negotiated by both parties. The transmission is LSB (least significant bit) first…MSB
Check Digit:
0 bit: no check bit
1 bit: check bit. "Lai Zi" X
D0 D1 D2 … Dn X
Odd parity: ensure that the number of transmitted 1s is an odd number
D0 D1 D2 … Dn X Make sure the number of 1s in this string of data is an odd number
1 0 1 0 1 0 1 0 X(1)
1 0 1 0 1 0 1 1 X(0)
Even parity: To save the transmitted 1 as an even number
Stop bit: High level
0.5 stop bit. High level for half a cycle
1 stop bit
1.5 stop bits
2 stop bits
Baudrate: Transmission rate.
Determine the time period.
115200 bps: bits per second
the physical layer standards
TTL level UART: TTL level serial port
RS-232:
RS-422:
RS-485:
TTL level UART:
Logic low (0) 0V
Logic High(1) 5V/3.3V
RS-232: Suitable for longer distance transmission
Logic low level (0) +3v~+15V
Logic high level (1) -3v~-15v
TTL UART RS-232 RS-422 RS-485
Level 1 3.3V/5V 1 -5V ~ -15V +/- 2v +/- 1.5v
0 0V 0 5V ~ +15V
Signal Single-ended signal Single-ended signal Differential signal Differential signal
Transmission length < 2m <15m <1200m <1200m
Different serial port standards have different pins. TX/Rx must exist.
TTL
RX
TX
GND
VCC
STM32F4xx UART controllers
TX
RX
Hardware flow control:
RTS: Request To Send
The terminal tells the other party that it can transmit data to me.
CTS: Clear To Send Clear to Send signal
The other party tells the terminal, I want to send you data
RTS -------> CTS (other party)
CTS <------- RTS (other party)
Flags:
TXE: Transmit data Register Empty
The transmit data register is empty.
It does not mean that the last data has been sent, because the data may still be in the shifter.
But now you can write data to TDR.
TC : Transmit Complete
Sending completed.
The data in the transmit shift register has been sent to the Tx pin.
RXNE: Read Data register Not Empty
The receive data register is not empty, which means you can read data.
STM32F4xx serial port code flow
(1) GPIO port configuration
The TX/RX pins of the serial port are multiplexed by the GPIO port.
a. Enable the clock of the GPIO group
RCC_AHB1PeriphClockCmd
b. Configure the GPIO port function GPIO_Init
c. Select specific reuse functions
GPIO_PinAFConfig
(2) usart configuration
a. Enable usart clock
b. USART_Init
USART_Init(USART_TypeDef * USARTx, USART_InitTypeDef * USART_InitStruct);
typedef struct
{
uint32_t USART_BaudRate; // baud rate
uint16_t USART_WordLength; //Transmission word length, choose one of the following two:
USART_WordLength_8b
USART_WordLength_9b
In STM32: Transmission word length = number of data bits + number of check bits
uint16_t USART_StopBits; //Stop bit number, as follows:
USART_StopBits_1 1bit stop bit
USART_StopBits_1_5 1.5bit stop bit
USART_StopBits_2 2 bits stop bit
USART_StopBits_0_5 0.5bit stop bit
uint16_t USART_Parity; //Verification method, as follows:
USART_Parity_No No parity
USART_Parity_Odd odd parity
USART_Parity_Even Even parity
uint16_t USART_Mode; //Serial port mode, as follows: can be combined
USART_Mode_Tx Transmit mode
USART_Mode_Rx Receive mode
USART_Mode_Tx | USART_Mode_Rx Transmit and Receive modes
uint16_t USART_HardwareFlowControl; //Hardware flow control
USART_HardwareFlowControl_None No hardware flow control
USART_HardwareFlowControl_RTS RTS Request to send. You can receive data from the other party.
USART_HardwareFlowControl_CTS CTS Clear to send, you can send data to the other party.
USART_HardwareFlowControl_RTS_CTS RTS_CTS Use flow control for both sending and receiving
} USART_InitTypeDef;
(3) Interrupt configuration
USART_ITConfig <- configure serial interrupt
In STM32, one USART corresponds to only one interrupt channel, but the interrupt that causes the serial port
There are many events, such as:
TXE -> The transmit register is empty, which can cause a serial port interrupt
TC -> Sending completed, can cause serial port interruption
RXNE -> The receiving register is not empty, which can cause a serial port interrupt
…
However, these events require "interrupt control bit enable"
USART_ITConfig(USART1, USART_IT_RXNE,ENABLE);
USART_ITConfig is used to configure a serial port's XX event to cause a serial port interrupt.
In the serial port interrupt function, you need to determine which serial port event caused the interrupt!!!
NVIC_Init()
(4) Enable the serial port
USART_Cmd
Receive (interrupt function)
USART1_IRQHanlder()
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
//There is data to read
data = USART_ReceiveData(USART1); //Read the received data
}
// Clear the interrupt flag of USART1
USART_GetITStatus
}
USART_GetITStatus is used to obtain the xx event flag of the serial port
send data
USART_SendData(USART1, 0x55);
while (USART_GetFlagStatus(USRAT1, USART_FLAG_TXE) == RESET); //Wait for sending to end
Previous article:STM32F4 USART1 TX RX FIFO
Next article:Data exchange between STM32F103 serial port 1 and serial port 2 at different baud rates
Recommended ReadingLatest update time:2024-11-16 13:54
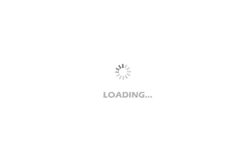
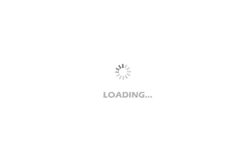
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [TI star products limited time sale] + Buy, buy, buy!
- [Xianji HPM6750 Review] CoreMark running test
- [Runhe Neptune Review] Six-tone LED light
- Huawei Electromagnetic Compatibility Structural Design Specification V2.0
- TMS320F28035 timer interrupt routine
- [TI mmWave Radar Evaluation] 5+AWR1443 EVM Board Sensitivity (Minimum Distance) Test and Summary
- 【ESP32-S2-Kaluga-1 Review】Getting weather information via https
- LE audio, Bluetooth positioning, Bluetooth mesh... Where will the next technological explosion point of Bluetooth be? ?
- RAM is equivalent to the computer's running memory. Can the memory stick also be expanded?
- LPS25HB Barometric Pressure Sensor PCB Package and Code