In the single-chip microcomputer, there is a problem of identifying buttons. The commonly used independent button circuit is as follows:
Since mechanical contact switches are flexible and will vibrate, they need to be debounced. There are two ways to debounce: hardware debounce and software debounce.
Hardware debounce circuit:
Software debouncing often uses delayed debouncing: when a key is pressed, when a low level is detected, there is a delay of a period of time, usually 5 to 10ms, and then the port is detected again. If it is still a low level, it means that the key is detected to be pressed, and the same applies when the key is released.
There are generally two methods for detecting independent buttons: interrupt detection and query detection.
The button is connected to the P3^2 pin to detect the number of times the button is pressed and released.
1. Query detection: You can directly loop the detection in the main program, or you can use a timer to detect once every 20ms. The following is a direct detection in the loop.
Main function:
#include
#include
#define uint unsigned int
sbit in0 = P3^2; //input signal pin
uint Press_flag = 1; //button pressed flag
uint count = 0 ; //Number of key presses
void delay_ms(uint ms)
{
uint i , j ;
for(i = 0 ; i < ms ; i++)
{
for(j = 0 ; j < 333 ; j++)
{
_nop_() ;
}
}
}
void key()
{
if(in0 == 0) //0 is detected
{
delay_ms(10); // Delay debounce
if(in0 == 0) // detect 0 again
{
Press_flag = 0; //Indicates that the button is pressed
}
}
if(Press_flag == 0) //If the key is pressed, detect if the key pops up
{
if(in0 == 1)
{
delay_ms(10) ;
if(in0 == 1)
{
count++; //Indicates the number of times the key is pressed and released
Press_flag = 1; // reset button pressed flag
}
}
}
}
void main(void)
{
while(1)
{
key() ;
}
}
Signal function:
signal void test(unsigned int cc)
{
unsigned int i , j ;
for(i = 0 ; i < cc ; i++)
{
for(j = 0 ; j < 2 ; j++)
{
port3 &= ~(1<<2) ;
swatch(0.002) ;
port3 |= 1<<2 ;
swatch(0.002);
}
port3 &= ~(1<<2) ;
swatch(0.002) ;
swatch(0.01) ;
for(j = 0 ; j < 2 ; j++)
{
port3 |= 1<<2 ;
swatch(0.002) ;
port3 &= ~(1<<2) ;
swatch(0.002) ;
}
port3 |= 1<<2 ;
swatch(0.02) ;
swatch(0.1) ;
}
_break_ = 1 ;
}
Signal function waveform:
You can see that the value of count is 4
2. Interruption detection
Main program:
#include
#include
#define uint unsigned int
sbit in0 = P3^2 ;
uint count = 0 ;
uint press_flag = 1 ;
void delay_ms(uint ms)
{
uint i , j ;
for(i = 0 ; i < ms ; i++)
{
for(j = 0 ; j < 333 ; j++)
{
_nop_() ;
}
}
}
void Init_INT(void)
{
EA = 1; // Enable general interrupt
EX0 = 1; //Open external interrupt 0
IT0 = 1; // Falling edge triggers interrupt
}
void Int0 (void) interrupt 0
{
EX0 = 0; //Disable external interrupt 0 to prevent frequent interrupt triggering
delay_ms(10) ;
if(in0 == 0)
{
press_flag = 0 ; //button pressed flag
}
if(press_flag == 0 && in0 == 1) // detect button pop-up
{
delay_ms(10) ;
if(in0 == 1)
{
count++ ;
press_flag = 1 ;
}
}
EX0 = 1; // Enable external interrupt
}
void main(void)
{
Init_INT(); //Interrupt initialization
while(1)
{
}
}
Previous article:Summary of the key points of single-chip C51 programming
Next article:STC89C52 MCU RAM Mode
Recommended ReadingLatest update time:2024-11-16 23:35
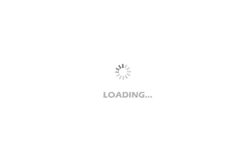
- Popular Resources
- Popular amplifiers
-
A review of learning-based camera and lidar simulation methods for autonomous driving systems
-
Simulation and Modeling of Chemical Sensors Volume 5 Electro-Optical Sensors Part 1 Photocopy
-
ADS2008 RF Circuit Design and Simulation Examples (Edited by Xu Xingfu)
-
CVPR 2023 Paper Summary: Embodied Vision: Active Agents, Simulation
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Live broadcast at 10 am today [Renesas Electronics Secure IoT Suite provides you with secure cloud connection solutions
- Xun developed Qt for Android for i.MX6ULL Terminator QT application
- Applications of RF Transformers
- RF FilterRF knowledge classics to understand
- 【NXP Rapid IoT Review】+Hello world!
- 5G miniaturized terminal and base station antenna technology
- DIY retro game console based on Raspberry Pi Zero
- Transistor static operating point
- The History of DSP and Why No One Mentions DSP Anymore
- RISC-V MCU IDE MRS (MounRiver Studio) development: Add standard math library reference