1. Timer Classification
In the STM32F1 series, in addition to the interconnected products, there are a total of 8 timers, which are divided into basic timers, general timers and advanced timers. The basic timers TIM6 and TIM7 are 16-bit timers that can only count up, can only be timed, and have no external IO. The general timer TIM2/3/4/5 is a 16-bit timer that can count up/down, can be timed, can output comparison, can input capture, and each timer has four external IOs. The advanced timer TIM1/8 is a 16-bit timer that can count up/down, can be timed, can output comparison, can input capture, and can also have three-phase motor complementary output signals. Each timer has 8 external IOs.
2. Functional Block Diagram Analysis
The core of the basic timer is the timebase, which is also present in the general timer and advanced timer.
1. Clock source
The timer clock TIMxCLK, that is, the internal clock CK_INT, is provided after being divided by the APB1 prescaler. If the APB1 prescaler coefficient is equal to 1, the frequency remains unchanged, otherwise the frequency is multiplied by 2. The APB1 prescaler coefficient in the library function is 2, that is, PCLK1=36M, so the timer clock TIMxCLK=36*2=72M.
2. Counter clock
After the timer clock passes through the PSC prescaler, CK_CNT, it is used to drive the counter to count. PSC is a 16-bit prescaler that can divide the timer clock TIMxCLK by any number between 1 and 65536.
The specific calculation method is: CK_CNT=TIMxCLK/(PSC+1).
3. Counter
Counter CNT is a 16-bit counter that can only count up, and the maximum count value is 65535. When the count reaches the auto-reload register, an update event is generated, and the register is cleared to zero and starts counting from the beginning.
4. Automatic reload register
The auto-reload register ARR is a 16-bit register that contains the maximum value that the counter can count. When the count reaches this value, if the interrupt is enabled, the timer will generate an overflow interrupt.
5. Calculation of timing time
The timing time of the timer is equal to the interrupt cycle of the counter multiplied by the number of interrupts. When the counter is driven by CK_CNT, the time it takes to count a number is the reciprocal of CK_CLK, which is equal to: 1/(TIMxCLK/(PSC+1)). The time it takes to generate an interrupt is equal to: 1/(CK_CLK * ARR). If a variable time is set in the interrupt service program to record the number of interrupts, then we can calculate that the timing time we need is equal to: 1/CK_CLK *(ARR+1)*time.
3. Detailed explanation of timer initialization structure
In the standard library function header file stm32f10x_tim.h, four initialization structures are established for the timer peripheral. The basic timer only uses one of them, namely TIM_TimeBaseInitTypeDef, and the other three are explained in the advanced timer chapter.
typedef struct {
uint16_t TIM_Prescaler; // Prescaler
uint16_t TIM_CounterMode; // Counting mode
uint32_t TIM_Period; // timer period
uint16_t TIM_ClockDivision; // Clock division
uint8_t TIM_RepetitionCounter; //Repetition counter
} TIM_TimeBaseInitTypeDef;
(1) TIM_Prescaler: Timer prescaler setting. The clock source passes through this prescaler to become the timer clock. It sets the value of the TIMx_PSC register. The setting range is 0 to 65535, achieving 1 to 65536 division.
(2) TIM_CounterMode: Timer counting mode, which can be up-counting, down-counting and three center-aligned modes. The basic timer can only count up, that is, TIMx_CNT can only increase from 0 and does not need to be initialized.
(3) TIM_Period: Timer period, which is actually the value of the auto-reload register, which is updated to the shadow register when an event is generated. The range is 0 to 65535.
(4) TIM_ClockDivision: Clock division, set the division ratio between the timer clock CK_INT frequency and the digital filter sampling clock frequency. The basic timer does not have this function and does not need to be set.
(5) TIM_RepetitionCounter: repetition counter, which belongs to the special register bit of the advanced control register. It can be used to easily control the number of output PWM. No need to set it here.
Although the timer basic initialization structure has 5 members, only two of them need to be set for the basic timer.
4. Basic timer experiment
This experiment uses the basic timer TIM6/7 to set the timing for 1s, and the LED flips once after 1s. The basic timer is an internal resource of the microcontroller, has no external IO, and does not need to be connected to an external circuit. Now only an LED is needed.
software design
Write two timer driver files, bsp_TiMbase.h and bsp_TiMbase.h, to configure the timer interrupt priority and initialize the timer.
1. Programming points
(1) Turn on the timer clock TIMx_CLK, x[6,7];
(2) Initialize the time base initialization structure;
(3) Enable TIMx, x[6,7] update interrupt;
(4) Turn on the timer;
(5) Write an interrupt service routine
The timing programming points of general timer and advanced timer are similar to those of basic timer, except that the counting mode of the counter needs to be selected, whether it is up or down. Because the basic timer can only count up, and there is no register configured for the counting mode, the default is up.
2. Software Analysis
Basic timer macro definition
1 /********************Basic timer TIM parameter definition, only limited to TIM6, 7************/
2 #define BASIC_TIM6 // If you use TIM7, just comment out this macro
3
4 #ifdef BASIC_TIM6 // Use basic timer TIM6
5 #define BASIC_TIM TIM6
6 #define BASIC_TIM_APBxClock_FUN RCC_APB1PeriphClockCmd
7 #define BASIC_TIM_CLK RCC_APB1Periph_TIM6
8 #define BASIC_TIM_IRQ TIM6_IRQn
9 #define BASIC_TIM_IRQHandler TIM6_IRQHandler
10
11 #else // Use basic timer TIM7
12 #define BASIC_TIM TIM7
13 #define BASIC_TIM_APBxClock_FUN RCC_APB1PeriphClockCmd
14 #define BASIC_TIM_CLK RCC_APB1Periph_TIM7
15 #define BASIC_TIM_IRQ TIM7_IRQn
16 #define BASIC_TIM_IRQHandler TIM7_IRQHandler
17
18 #endif
The basic timers are TIM6 and TIM7, which we can use selectively. In order to improve the portability of the code, we define the code that needs to be modified when the timer needs to be modified as a macro. The default timer is timer 6. If you want to change it to timer 7, just comment out the macro BASIC_TIM6.
Basic timer settings
void BASIC_TIM_Config(void)
2 {
3 TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
4
5 // Turn on the timer clock, that is, the internal clock CK_INT=72M
6 BASIC_TIM_APBxClock_FUN(BASIC_TIM_CLK, ENABLE);
7
8 // Automatically reload the value of the register week (count value)
9 TIM_TimeBaseStructure.TIM_Period=1000;
10
11 // Generate an update or interrupt after accumulating TIM_Period frequencies
12 // The clock pre-division number is 71, then the clock driving the counter CK_CNT = CK_INT / (71+1) = 1M
13 TIM_TimeBaseStructure.TIM_Prescaler= 71;
14
15 // Clock division factor, basic timer does not have it, so don't worry about it
16 //TIM_TimeBaseStructure.TIM_ClockDivision=TIM_CKD_DIV1;
17
18 //Counter counting mode, the basic timer can only count upwards, there is no counting mode setting
19 //TIM_TimeBaseStructure.TIM_CounterMode=TIM_CounterMode_Up;
20
21 // Repeat the counter value. The basic timer does not have it, so don't worry about it.
22 //TIM_TimeBaseStructure.TIM_RepetitionCounter=0;
twenty three
24 // Initialize the timer
25 TIM_TimeBaseInit(BASIC_TIM, &TIM_TimeBaseStructure);
26
27 // Clear the counter interrupt flag
28 TIM_ClearFlag(BASIC_TIM, TIM_FLAG_Update);
29
30 // Enable counter interrupt
31 TIM_ITConfig(BASIC_TIM,TIM_IT_Update,ENABLE);
32
33 // Enable counter
34 TIM_Cmd(BASIC_TIM, ENABLE);
35
36 // Temporarily turn off the timer clock and wait for use
37 BASIC_TIM_APBxClock_FUN(BASIC_TIM_CLK, DISABLE)
38 }
We set the timer auto-reload register ARR value to 1000, set the clock prescaler to 71, then the clock driving the counter: CK_CNT = CK_INT / (71+1)=1M, then the time for the counter to count once is equal to: 1/CK_CNT=1us, when the counter counts to the ARR value of 1000, an interrupt is generated, and the interrupt time is: 1/CK_CNT*ARR=1ms.
When initializing the timer, we define a structure: TIM_TimeBaseInitTypeDef. There are 5 members in the TIM_TimeBaseInitTypeDef structure. The registers of TIM6 and TIM7 only have TIM_Prescaler and TIM_Period. The other three members are not available in the basic timer. Therefore, when using TIM6 and TIM7, only these two members need to be initialized. The other three members are only available in the general timer and advanced timer. The specific descriptions are as follows:
1 typedef struct {
2 TIM_Prescaler // both
3 TIM_CounterMode // TIMx,x[6,7] not available, others available
4 TIM_Period // both
5 TIM_ClockDivision // TIMx,x[6,7] not available, others available
6 TIM_RepetitionCounter // TIMx,x[1,8,15,16,17] only
7 } TIM_TimeBaseInitTypeDef;
Timer interrupt priority configuration
1 // Interrupt priority configuration
2 void BASIC_TIM_NVIC_Config(void)
3 {
4 NVIC_InitTypeDef NVIC_InitStructure;
5 // Set interrupt group to 0
6 NVIC_PriorityGroupConfig(NVIC_PriorityGroup_0);
7 // Set the interrupt source
8 NVIC_InitStructure.NVIC_IRQChannel = BASIC_TIM_IRQ;
9 // Set the main priority to 0
10 NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
11 // Set the preemption priority to 3
12 NVIC_InitStructure.NVIC_IRQChannelSubPriority = 3;
13 NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
14 NVIC_Init(&NVIC_InitStructure);
15 }
Timer interrupt service routine
1 void BASIC_TIM_IRQHandler (void)
2 {
3 if ( TIM_GetITStatus( BASIC_TIM, TIM_IT_Update) != RESET ) {
4 time++;
5 TIM_ClearITPendingBit(BASIC_TIM, TIM_FLAG_Update);
6 }
7 }
The timer interruption time is 1ms. We define a global variable time. Every time an interruption occurs, let time record the number of interruptions. If we want to implement a 1s timing, we only need to determine whether time is equal to 1000. 1000 1ms equals 1s. Then clear time to 0 and count again. This cycle repeats. At the end of the interrupt service program, the corresponding interrupt flag must be cleared. Remember.
Main function
1 int main(void)
2 {
3 /* led port configuration */
4 LED_GPIO_Config();
5
6 /* Basic timer TIMx,x[6,7] timing configuration*/
7 BASIC_TIM_Config();
8
9 /* Configure the interrupt priority of basic timer TIMx,x[6,7]*/
10 BASIC_TIM_NVIC_Config();
11
12 /* Basic timer TIMx,x[6,7] restarts the clock and starts timing*/
13 BASIC_TIM_APBxClock_FUN(BASIC_TIM_CLK, ENABLE);
14
15 while (1) {
16 if ( time == 1000 ) { /* 1000 * 1 ms = 1s time is up*/
17 time = 0;
18 /* LED1 inversion*/
19 LED1_TOGGLE;
20 }
twenty one }
twenty two }
The function does some necessary initialization, and then continuously judges the value of time in an infinite loop. The value of time changes in the timer interrupt, and each increment means that 1ms has passed. When time is equal to 1000, 1s is up, LED1 flips once, and time is cleared to 0.
4. Thinking
1. Calculate the longest timing time of a basic timer. If you need to use a basic timer to generate a 100s periodic event, how can you achieve it?
2. Modify the experimental program so that LED1 is flipped every 0.5s and LED2 is flipped every 10s.
Previous article:Problems encountered when using STLINK to burn external FLASH in STM32
Next article:STM32 system learning - SPI (read and write serial FLASH)
Recommended ReadingLatest update time:2024-11-16 12:50
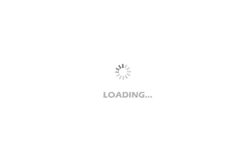
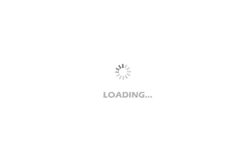
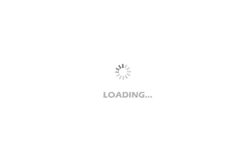
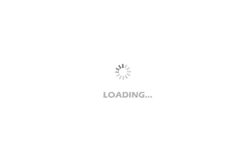
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TPS61040 boost circuit abnormality
- [Atria AT32WB415 series Bluetooth BLE 5.0 MCU] + CAN communication
- What does it mean when a pushpin icon is displayed during STM32CubeMX pin configuration?
- Does TI C6678 support flash partitioning?
- 6678 SRIO: About QMSS, CPPI and SRIO Socket
- 【Top Micro Intelligent Display Module】VI: A comprehensive interface for controls
- RISC-V MCU IDE MRS (MounRiver Studio) development: Setting the engineering encoding character set
- Why are some MCU register addresses 00H and some 0000H? Is there a difference? How many addresses are there from 80H to FFH?
- How can the signal source of Proteus change the phase of the emitted square wave signal?
- [Erha Image Recognition Artificial Intelligence Vision Sensor] Evaluation 2: Built-in 7 functions, face recognition and other tests