1. Introduction to basic timers
In STM32, the basic timers include TIM6, TIM7, etc. The basic timer mainly includes a time base unit, which provides 16-bit counting and can count 0~65535. In addition to the counting function, the basic timer can also output a TRGO signal to the DAC module. The basic timer block diagram is as follows:
2. Time base unit introduction
All timers of STM32 have a time base unit. The function of the time base unit is to simply count, that is, to count the number of pulses of the clock source TMxCLK, which comes from the APB1 bus. Advanced and general timers can also use other clock sources for counting, which will be introduced in detail in the advanced timer and general timer. In the basic timer framework, it can be seen that the time base unit consists of the following three parts:
1.ARR automatic reload register
2. CNT counter
3.PSC prescaler
The timing (counting) function of the basic timer is configured as follows:
void TIM6_IRQHandler(void)
{
static int counter = 0;
if(TIM_GetITStatus(TIM6,TIM_IT_Update))
{
//After setting TIM_SelectOnePulseMode(TIM6,TIM_OPMode_Single); interrupt twice
TIM_ClearITPendingBit(TIM6,TIM_IT_Update);
}
}
//Basic timer
void TIM6_Configuration()
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseInitStruct;
NVIC_InitTypeDef NVIC_InitStruct;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM6, ENABLE); //Clock enable
TIM_TimeBaseInitStruct.TIM_Period = 10 -1;
TIM_TimeBaseInitStruct.TIM_Prescaler = 72;
TIM_TimeBaseInitStruct.TIM_ClockDivision = 0;
TIM_TimeBaseInitStruct.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInitStruct.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM6,&TIM_TimeBaseInitStruct);// TIMx->EGR.UG
NVIC_InitStruct.NVIC_IRQChannel = TIM6_IRQn;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_Init(&NVIC_InitStruct);
TIM_ITConfig(TIM6,TIM_IT_Update,ENABLE);
TIM_ClearITPendingBit(TIM6,TIM_IT_Update);
// TIM_SelectOnePulseMode(TIM6,TIM_OPMode_Single); //To configure single pulse mode, open this comment
TIM_ARRPreloadConfig(TIM6,ENABLE);
TIM_Cmd(TIM6,ENABLE);//CEN bit
TIM_ClearITPendingBit(TIM6,TIM_IT_Update);
}
It is worth noting that the basic timer also supports single pulse mode. You can configure the single pulse mode as shown in the code comments. In the single pulse mode, it is important to note that the timer is turned off only after the timer overflows twice, that is, the timer is disabled. In the code, interrupts are configured. In the single pulse mode, it can be clearly seen that the timer interrupt is entered twice.
3. Timer signal output
The signal output of the timer is related to the MMS bit of the control register 2 (TIM6->CR2) in the timer. The signal output by the basic timer can only be used as a trigger for the DAC, while the output signal of the advanced timer and general timer can trigger the timer and DAC. The specific details are not described here. For an example of the timer signal output, please refer to my blog http://blog.csdn.net/quentinecho/article/details/79068001. In this example, the TRGO signal output by TIM6 is used to start the DAC to generate a triangle wave. Of course, other DAC triggering methods can also generate a triangle wave.
#include "stm32f10x.h"
#include "stm32f10x_rcc.h"
#include "system_stm32f10x.h"
#include "stm32f10x_dac.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_tim.h"
/*DAC output = Vref x (DOR/4095)*/
//The two channels of DAC can be configured and used
//Same trigger source/different trigger source
//Simultaneous trigger/independent trigger DAC_DualSoftwareTriggerCmd function sets software simultaneous trigger
//Use waveform generator/Do not use waveform generator
//Use triangle wave generator/Use noise generator/Do not use waveform generator
//Set the same DAC_LFSRUnmask_TriangleAmplitude value/set different DAC_LFSRUnmask_TriangleAmplitude values
//The above situations can be combined arbitrarily without affecting each other.
void DAC_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
DAC_InitTypeDef DAC_InitStruct;
//First step: Enable the clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA,ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_DAC,ENABLE);
//Step 2 Configuration parameters
/*Once the DACx channel is enabled, the corresponding GPIO pin will automatically be connected to the analog output of the DAC. In order to avoid parasitic interference and additional power consumption, pins PA4/PA5 should be set to "analog input" before.
Note that it is "analog input", because there is no analog output in STM32, so although PA4 PA5 outputs analog signals, it can only be set to GPIO_Mode_AIN*/
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_4 | GPIO_Pin_5;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AIN;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA,&GPIO_InitStruct);
GPIO_SetBits(GPIOA,GPIO_Pin_4 | GPIO_Pin_5); //PA.4 PA.5 input high, pull-up input plays an anti-interference role
// /*DAC channel 1 PA4 generates noise*/
// DAC_InitStruct.DAC_WaveGeneration = DAC_WaveGeneration_Noise;
// DAC_InitStruct.DAC_Trigger = DAC_Trigger_T6_TRGO; //DAC_Trigger_T6_TRGO;
// DAC_InitStruct.DAC_OutputBuffer = DAC_OutputBuffer_Disable; //The output buffer can be used to reduce the output impedance and directly drive the external load without an external op amp
// DAC_InitStruct.DAC_LFSRUnmask_TriangleAmplitude = DAC_LFSRUnmask_Bits10_0; //Calculate the LSFR algorithm once each time it is triggered, add the obtained value to the value of DAC_DHRx, remove the overflow bit and write it to the DAC_DORx register to output a specific voltage
// DAC_Init(DAC_Channel_1,&DAC_InitStruct); //The number of bits involved in the LSFR algorithm is determined by DAC_LFSRUnmask_TriangleAmplitude. The value of DAC_LFSRUnmask_Bits10_0 indicates that 10 bits are involved in the LSFR calculation.
/*DAC channel 1 PA4 normal digital-to-analog conversion*/
DAC_InitStruct.DAC_WaveGeneration = DAC_WaveGeneration_None; //Turn off the waveform generator
DAC_InitStruct.DAC_Trigger = DAC_Trigger_T6_TRGO;//DAC_Trigger_Software/DAC_Trigger_Ext_IT9
DAC_InitStruct.DAC_OutputBuffer = DAC_OutputBuffer_Disable; //The output buffer can be used to reduce the output impedance and directly drive the external load without an external op amp
DAC_InitStruct.DAC_LFSRUnmask_TriangleAmplitude = DAC_LFSRUnmask_Bit0; //This parameter is related to the noise/triangle wave generator. For normal DAC conversion, just set it to 0.
DAC_Init(DAC_Channel_1,&DAC_InitStruct);
/*DAC channel 2 PA5 generates triangle wave*/
DAC_InitStruct.DAC_WaveGeneration = DAC_WaveGeneration_Triangle;
DAC_InitStruct.DAC_Trigger = DAC_Trigger_T6_TRGO;
DAC_InitStruct.DAC_OutputBuffer = DAC_OutputBuffer_Disable;
DAC_InitStruct.DAC_LFSRUnmask_TriangleAmplitude = DAC_TriangleAmplitude_4095; //The internal triangle wave counter accumulates 1 each time it is triggered. The value of the counter is added to the value of DAC_DHRx, and the overflow bit is removed before writing to the DAC_DORx register. The output voltage
DAC_Init(DAC_Channel_2,&DAC_InitStruct); //The maximum value of the triangle wave counter is determined by DAC_LFSRUnmask_TriangleAmplitude. When the counter reaches this maximum value, the triangle wave counter starts to decrease.
//Step 3: Enable the device
//DAC_SetDualChannelData(DAC_Align_12b_R,4095,0); Equivalent to DAC_SetChannel1Data(DAC_Align_12b_R, 4095); DAC_SetChannel2Data(DAC_Align_12b_R, 0);
/*DAC channel 1 PA4 enable*/
DAC_SetChannel1Data(DAC_Align_12b_R, 4095); //Set DAC value in 12-bit right-aligned data format. The maximum value is 4095. If it is set to 4096, it will overflow and DORx will be 0.
DAC_Cmd(DAC_Channel_1, ENABLE); //Enable DAC1
/*DAC channel 2 PA5 enable*/
DAC_Cmd(DAC_Channel_2, ENABLE); //Enable DAC1
DAC_SetChannel2Data(DAC_Align_12b_R, 0); //Set DAC value in 12-bit right-aligned data format
}
//Basic timer
void TIM6_Configuration()
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseInitStruct;
//First step: Enable the clock
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM6, ENABLE); //Clock enable
//The second step is to configure the parameters
TIM_TimeBaseInitStruct.TIM_Period = 10 -1;
TIM_TimeBaseInitStruct.TIM_Prescaler = 72;
TIM_TimeBaseInitStruct.TIM_ClockDivision = 0;
TIM_TimeBaseInitStruct.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInitStruct.TIM_RepetitionCounter = 0;
TIM_TimeBaseInit(TIM6,&TIM_TimeBaseInitStruct);// TIMx->EGR.UG
/*TIM6,7 can output 3 types of TRGO signals
#define TIM_TRGOSource_Reset ((uint16_t)0x0000) //Reset UG
#define TIM_TRGOSource_Enable ((uint16_t)0x0010) // Enable CEN
#define TIM_TRGOSource_Update ((uint16_t)0x0020) //Update event
*/
TIM_SelectOutputTrigger(TIM6,TIM_TRGOSource_Update); //Output trigger TRGO signal Here TRGO signal is the update signal generated by timer overflow
//Step 3: Enable the device
TIM_Cmd(TIM6,ENABLE);//CEN bit
}
int main()
{
DAC_Configuration();
TIM6_Configuration();
while(1)
{
}
}
Previous article:stm32 runtime measurement and interval execution
Next article:STM32 timer writes precise delay function
Recommended ReadingLatest update time:2024-11-16 18:05
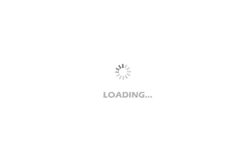
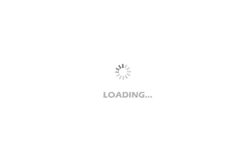
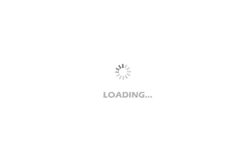
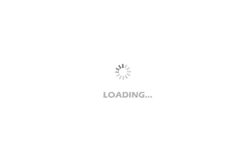
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Introduction to the method of board-level online compilation and downloading of C6000 DSP code
- Working principle of lithium battery equalization circuit
- [SAMR21 new gameplay] 31. Using NVM storage
- [ATmega4809 Curiosity Nano Review] Buttons
- Have you fallen into these customer support "pitfalls"? Read the story, write a comment, grab a post and win a gift!
- About rail-to-rail op amps
- 【TGF4042 signal generator】+ Load capacity test
- EEWORLD University - In-depth understanding of PCI Express 5.0 testing
- Namisoft about virtual voltmeter design and virtual digital multimeter
- Dating Spring - Vegetable Garden and Fruits at Home