The article http://blog.csdn.net/qq_29344757/article/details/73479924 introduces the clock system of STM32. Now, taking the SetSysClockTo72() function of the STM32 standard peripheral library as an example, we will introduce the programming of RCC.
With the foundation of the previous article, learning RCC is no longer so difficult and boring, at least I think so. The SetSysClockTo72(void) function is the default system clock setting function when we use the peripheral library.
As marked in the figure above, the core function of this function is to set these 5 points.
(1) Set HCLK, HCLK = SYSCLK
(2) Set PCLK2, PCLK2 = HCLK
(3) Set PCLK1, PCLK1 = HCLK / 2
(4) Set the PLL clock source and PLL multiplication factor
(5) Select PLL as the system clock source, that is, PLLCLK = SYSCLK
In general, the system uses the HSE clock source, and then HSE is multiplied by PLL as the system clock. The usual configuration is HSE = 8M, and the multiplication factor of PLL is 9, then the system clock SYSCLK = 8M * 9 = 72MHz, from which it can be deduced that HCLK = PCLK2 = 72MHz, PCLK1 = 36MHz.
PCLK2 is a high-speed bus clock. The high-speed peripherals on the chip are mounted on this bus, such as all GPIO, SPI1, USART1, etc.:
The following code is extracted from the standard peripheral library file system_stm32f10x.c, and the interconnection-related code is deleted. This function directly operates the register. For register operations, please refer to the relevant chapters of "STM32 Chinese Reference Manual_V10.pdf".
The program flow is:
(1) Enable HSE and wait for HSE to stabilize
(2) Set the APB2, APB1, and AHB frequency division coefficients
(3) Set the PLL clock source and PLL multiplication factor
(4) Turn on PLL and wait for PLL to stabilize
(5) Read the clock switching status to ensure that PLLCLK is selected as the system clock
static void SetSysClockTo72(void)
{
__IO uint32_t StartUpCounter = 0, HSEStatus = 0;
//1. Enable HSE
RCC->CR |= ((uint32_t)RCC_CR_HSEON);
//Wait for HSE to stabilize, StartUpCounter is used to judge the timeout processing flag
do
{
HSEStatus = RCC->CR & RCC_CR_HSERDY;
StartUpCounter++;
} while((HSEStatus == 0) && (StartUpCounter != HSE_STARTUP_TIMEOUT));
//If HSE is stable, HSEStatus = 0x01
if ((RCC->CR & RCC_CR_HSERDY) != RESET)
{
HSEStatus = (uint32_t)0x01;
}
else //HSE has timed out and is not stable yet
{
HSEStatus = (uint32_t)0x00;
}
//2. HSE started successfully
if (HSEStatus == (uint32_t)0x01)
{
// Enable flash pre-storage buffer, flash settings related
FLASH->ACR |= FLASH_ACR_PRFTBE;
FLASH->ACR &= (uint32_t)((uint32_t)~FLASH_ACR_LATENCY);
FLASH->ACR |= (uint32_t)FLASH_ACR_LATENCY_2;
//Undivided SYSCLK, HCLK = SYSCLK
RCC->CFGR |= (uint32_t)RCC_CFGR_HPRE_DIV1;
//Undivided HCLK, PCLK2 = HCLK
RCC->CFGR |= (uint32_t)RCC_CFGR_PPRE2_DIV1;
//2-divided HCLK, PCLK1 = HCLK / 2
RCC->CFGR |= (uint32_t)RCC_CFGR_PPRE1_DIV2;
//3. Set the PLL clock source and PLL multiplication factor to 9, that is, PLLCLK is equal to 8M * 9 = 72M
RCC->CFGR &= (uint32_t)((uint32_t)~(RCC_CFGR_PLLSRC | RCC_CFGR_PLLXTPRE |
RCC_CFGR_PLLMULL));
RCC->CFGR |= (uint32_t)(RCC_CFGR_PLLSRC_HSE | RCC_CFGR_PLLMULL9);
//4. Enable PLL
RCC->CR |= RCC_CR_PLLON;
//Wait for PLL to stabilize
while((RCC->CR & RCC_CR_PLLRDY) == 0);
//5. Select PLL as the system clock source
RCC->CFGR &= (uint32_t)((uint32_t)~(RCC_CFGR_SW));
RCC->CFGR |= (uint32_t)RCC_CFGR_SW_PLL;
//Read the clock switching status success flag
while ((RCC->CFGR & (uint32_t)RCC_CFGR_SWS) != (uint32_t)0x08);
}
else
{
//HSE startup failed, the user can program a prompt
}
}
Previous article:STM32 serial USART communication
Next article:STM32 GPIO internal structure and related registers
Recommended ReadingLatest update time:2024-11-16 13:48
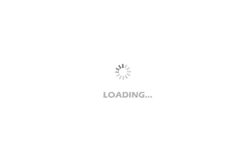
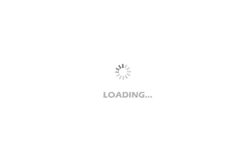
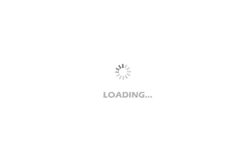
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Selling Zedboard Zynq7020 development board
- I have to say that the old technology I have been exposed to since 2008 was still blocked at that time.
- Calibration of medical devices
- Doesn't high input impedance mean high loss? Why does sampling require high input impedance? Low output impedance, such as running...
- How to set the prohibited routing area when AD wiring
- [Mill Edge AI Computing Box FZ5 Review] Object Detection Demo
- What serial port is UART?
- Read the good book "Operational Amplifier Parameter Analysis and LTspice Application Simulation" 01 LTspice Installation and Example Run
- Power supply circuit or module recommendation (no matter how the positive and negative poles are connected, the power supply can be normal)
- Why signal isolation is important in 48V HEV/EV systems