Note: There may be many wrong ideas in it. I hope everyone will correct them in time after discovering them.
First, let's learn about the dual stack. The following picture is from the "Cortex-M3 Definitive Guide". It's a bit boring, but you still need to read it.
Summarize:
1. After the system is reset, the default is MSP. The state after reset is the privileged thread state. In this state, the register CONTROL[1] can be modified (see the picture above). After entering the user privilege state, these registers cannot be modified.
2. MSP or PSP can be used in user privileged mode (that is, non-interrupt service routines established by users), and only MSP can be used in privileged mode (interrupt service routines).
3. Another very important point is that if PSP is used in user mode, the value of the register is saved in the task stack space. After entering the interrupt program, MSP is used. If there is a high-priority interrupt, MSP will continue to be used. When the program exits the last level of interrupt, the user stack is used to restore the register.
The following uses uCOS-II as an example to illustrate:
First, create a stack OS_STK AppTaskStartStk[1024] //32 bits
STM32 is a full stack that grows downward. After the stack is initialized (before PSP is used, MSP is always used).
| .... | AppTaskStartStk[0]
|-----------------|
| .... | AppTaskStartStk[1]
|-----------------|
| .... |
|-----------------| |---- PSP
Low Memory during Task Switching | .... | |
|-----------------| | |---------------| |----------------|
^ | R4 | <----|----|--OSTCBStkPtr |<-----| (OS_TCB *) |
^ |-----------------| |---------------| |----------------|
^ | R5 | | | OSTCBHighRdy
| |-----------------| |---------------|
| | R6 | | |
| |-----------------| |---------------|
| | R7 | | |
| |-----------------| |---------------|
| | R8 | Task's
| |-----------------| OS_TCB
| | R9 |
| |-----------------|
| | R10 |
Stack |-----------------|
Growth | R11 |
= 1 |-----------------|
| | R0 = p_arg | <-------- PSP at exception (full stack growing downward)
| |-----------------|
| | R1 |
| |-----------------|
| | R2 |
| |-----------------|
| | R3 |
| |-----------------|
| | R12 |
| |-----------------|
| | LR |
| |-----------------|
| | SP = task | AppTaskStartStk[1022]
| |-----------------|
| | xPSR | AppTaskStartStk[1023]
High Memory |-----------------|
Before the first execution of the PendSV interrupt, PSP = 0 has been initialized. MSP is used before entering the interrupt, so the values of the registers automatically pushed onto the stack are saved in the system stack. Since it is the first execution, there is no need to manually save PSP and {R4-R11} into the task stack, and then fetch data from the task stack space into registers {R4-R11}. When exiting the interrupt, set bit 2 of LR to ensure that PSP is used when exiting the interrupt, restore the values of the remaining registers (the values of these registers are automatically pushed onto the stack), and finally enter the task. When executing the task program, PSP is used, and any numbers that need to be pushed onto the stack will be entered into the task stack. Now consider two situations.
(1) If there is a high-priority interrupt to be executed, the register values automatically pushed into the stack will be saved in the stack of the current task, and the MSP will be used after entering the interrupt service routine (if the remaining registers need to be saved, the compiler will automatically generate the corresponding assembly code and save it to the system stack instead of the task stack). If there are higher-priority interrupts later, the MSP will continue to be used.
(2) If there is a high-priority task that needs to be executed at this time, then xPSR, PC, LR, R12, R0-R3 are automatically saved to the stack of the current task by hardware, and then PSP and {R4-R11} need to be pushed into the stack manually.
If two data are pushed onto the stack during the execution of a low-priority task, the result of saving the registers after entering the PendSV interrupt is as follows:
| .... | AppTaskStartStk[0]
|-----------------|
| .... | AppTaskStartStk[1]
|-----------------|
| .... |
|-----------------| |---- PSP
Low Memory during Task Switching | .... | |
|-----------------| | |---------------| |----------------|
^ | R4 | <----|----|--OSTCBStkPtr |<-----| (OS_TCB *) |
^ |-----------------| |---------------| |----------------|
^ | R5 | | | OSTCBHighRdy
| |-----------------| |---------------|
| | R6 | | |
| |-----------------| |---------------|
| | R7 | | |
| |-----------------| |---------------|
| | R8 | Task's
| |-----------------| OS_TCB
| | R9 |
| |-----------------|
| | R10 |
Stack |-----------------|
Growth | R11 |
= 1 |-----------------|
| | R0 = p_arg | <-------- PSP on exception (full stack growing downwards)
| |-----------------|
| | R1 |
| |-----------------|
| | R2 |
| |-----------------|
| | R3 |
| |-----------------|
| | R12 |
| |-----------------|
| | LR |
| |-----------------|
| | SP = task |
| |------------------|
| | xPSR |
| |-----------------|
| | 0x11111111 |
| |------------------|
| | 0x22222222 |
High Memory |-----------------|
Previous article:About assert_param() in STM32
Next article:STM32 interrupts and exceptions
Recommended ReadingLatest update time:2024-11-16 16:56
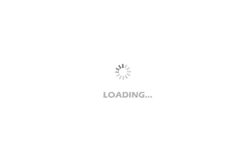
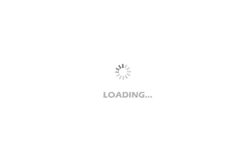
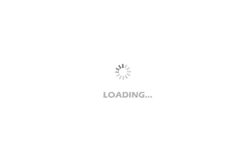
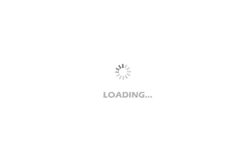
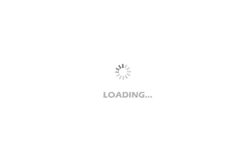
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- EEWORLD University ---- Top 10 Arduino Projects 2020
- Designing Building Security Systems Using the Sub-1 GHz Linux Gateway Software Development Kit
- [STM32]NUCLEO-G071RB Review
- 【Recruitment】RF Engineer
- Bluetooth Technology Principle and Test
- From getting started with the development board evaluation to being ready to give up
- What is the principle of constant current source composed of operational amplifier and MOS?
- Talk about the high and low temperature test problems of CC2640 CC1310
- Professional standards for engineers in integrated circuits, artificial intelligence, the Internet of Things, etc. have been released. Come and see if you are qualified?
- EEWORLD University ---- Networking Technology