//=========================================
// NAME: main.c
// DESC: TQ2440 serial port UART test program
//=========================================
#include "def.h"
#include "option.h"
#include "2440addr.h"
#include
#include
#include
#include
#include
//======================================================
static volatile int uart_port = 0;
void uart_init(int pclk,int buad,int ch)
{
//UART initialization: port enable, function setting, baud rate, data format setting
rGPHCON = (rGPHCON & ~(0xfff<<4)) | (0xaaa<<4); //Port RX[0:2], TX[0:2] function
rGPHUP = rGPHUP | (0x7<<1); //Port GPH[1:3] disable pull-up
rUFCON0 = 0x0; //Disable FIFO
rUFCON1 = 0x0; //Disable FIFO
rUFCON2 = 0x0; //Disable FIFO
rUMCON0 = 0x0; //Disable AFC
rUMCON1 = 0x0; //Disable AFC
//Normal:No parity:One stop:8-bits interrupt response UART clock: PCLK
rULCON0 = (rULCON0 & ~0xff) | ((0x0<<6)|(0x0<<3)|(0x0<<2)|(0x3));
rUCON0 = (rUCON0 & ~0x3ff) | ((0x1<<9)|(0x1<<6)|(0x1<<2)|(0x1));
rUBRDIV0 = ((int)(pclk/16./buad+0.5)-1);
rULCON1 = (rULCON1 & ~0xff) | ((0x0<<6)|(0x0<<3)|(0x0<<2)|(0x3));
rUCON1 = (rUCON1 & ~0x3ff) | ((0x1<<9)|(0x1<<6)|(0x1<<2)|(0x1));
rUBRDIV1 = ((int)(pclk/16./buad+0.5)-1);
rULCON2 = (rULCON2 & ~0xff) | ((0x0<<6)|(0x0<<3)|(0x0<<2)|(0x3));
rUCON2 = (rUCON2 & ~0x3ff) | ((0x1<<9)|(0x1<<6)|(0x1<<2)|(0x1));
rUBRDIV2 = ((int)(pclk/16./buad+0.5)-1);
uart_port = ch; //Set the serial port number
}
//******************************************************
//Serial port sending function
//******************************************************
//======================================================
void uart_send_byte(int data)
{
if(0 == uart_port)
{
if(data == 'n')
{
while(!(rUTRSTAT0 & 0x2));
rUTXH0 = 'n';
}
while(!(rUTRSTAT0 & 0x2));
rUTXH0 = data;
}
else if(1 == uart_port)
{
if(data == 'n')
{
while(!(rUTRSTAT1 & 0x2));
rUTXH1 = 'n';
}
while(!(rUTRSTAT1 & 0x2));
rUTXH1 = data;
}
else if(2 == uart_port)
{
if(data == 'n')
{
while(!(rUTRSTAT2 & 0x2));
rUTXH2 = 'n';
}
while(!(rUTRSTAT2 & 0x2));
rUTXH2 = data;
}
}
//======================================================
void uart_send_string(char *string)
{
while(*string)
{
uart_send_byte(*string++);
}
}
//======================================================
void uart_printf(char *fmt,...)
{
va_list ap;
char string[256];
va_start(ap,fmt);
vsprintf(string,fmt,ap);
uart_send_string(string);
va_end(ap);
}
//******************************************************
//Serial port receiving function
//******************************************************
//======================================================
void uart_tx_empty(void)
{
if(0 == uart_port)
{
while(!(rUTRSTAT0 & 0x4));//Wait for Tx to be empty
}
if(1 == uart_port)
{
while(!(rUTRSTAT1 & 0x4));//Wait for Tx to be empty
}
if(2 == uart_port)
{
while(!(rUTRSTAT2 & 0x4));//Wait for Tx to be empty
}
}
//======================================================
char uart_get_ch(void)
{
if(0 == uart_port)
{
while(!(rUTRSTAT0 & 0x1));//Wait for Rx to be read
return rURXH0; //Read cache register
}
else if(1 == uart_port)
{
while(!(rUTRSTAT1 & 0x1));//Wait for Rx to be read
return rURXH1; //Read cache register
}
else if(2 == uart_port)
{
while(!(rUTRSTAT2 & 0x1));//Wait for Rx to be read
return rURXH2; //Read cache register
}
return 0;
}
//======================================================
char uart_get_key(void)
{
if(0 == uart_port)
{
if(rUTRSTAT0 & 0x1) //Rx is read
{
return rURXH0; //Read cache register
}
else
{
return 0;
}
}
if(1 == uart_port)
{
if(rUTRSTAT1 & 0x1) //Rx is read
{
return rURXH1; //Read cache register
}
else
{
return 0;
}
}
if(2 == uart_port)
{
if(rUTRSTAT2 & 0x1) //Rx is read
{
return rURXH2; //Read cache register
}
else
{
return 0;
}
}
return 0;
}
//======================================================
void uart_get_string(char *string)
{
char *string1 = string;
char c = 0;
while((c = uart_get_ch())!='r')
{
if(c == 'b')
{
if((int)string1 < (int)string)
{
uart_printf("bb");
string--;
}
}
else
{
*string++ = c;
uart_send_byte(c);
}
}
*string = '�';
uart_send_byte('n');
}
//======================================================
int uart_get_intnum(void)
{
char str[30];
char *string = str;
int base = 10;
int minus = 0;
int result = 0;
int last_index;
int i;
uart_get_string(string);
if(string[0] == '-')
{
minus = 1;
string++;
}
if(string[0] == '0' && (string[1] == 'x' || string[1] == 'X'))
{
base = 16;
string = string + 2;
}
last_index = strlen(string)-1;
if(last_index < 0)
{
return -1;
}
if(string[last_index] == 'h' || string[last_index] == 'H')
{
base = 16;
string[last_index] = 0;
last_index--;
}
if(base == 10)
{
//atoi converts the string into an integer value
result = atoi(string);
result = minus ? (-1*result) : result;
}
else
{
for(i=0;i<=last_index;i++)
{
//Judge whether the character is an English letter. If it is an English letter az or AZ, return a non-zero value, otherwise return zero.
if(isalpha(string[i]))
{
//isupper determines whether the character is an uppercase English letter. When c is an uppercase English letter, it returns a non-zero value, otherwise it returns zero.
if(isupper(string[i]))
{
result = (result<<4) + string[i]-'A'+10;
}
else
{
result = (result<<4) + string[i]-'a'+10;
}
}
else
{
result = (result<<4) + string[i]-'0'+10;
}
}
result = minus ? (-1*result) : result;
}
return result;
}
//======================================================
int uart_get_intnum_gj(void)
{
char string[16];
char *p_string = string;
char c;
int i = 0;
int data = 0;
while((c = uart_get_ch()) != 'r')
{
if(c == 'b')
{
p_string--;
}
else
{
*p_string++ = c;
}
//uart_send_byte(c);
}
*p_string = '�';
i=0;
while(string[i] != '�')
{
] data = data*10;
if(string[i]
{
return -1;
}
data = data +(string[i]-'0');
i++;
}
return data;
}
//******************************************************
void Main(void)
{
uart_init(50000000,115200,0);
while(1)
{
if('-' == uart_get_ch())
{
uart_printf("+n");
}
if('+' == uart_get_key())
{
uart_printf("-n");
}
}
}
Previous article:TQ2440 naked program: serial UART printf test program
Next article:ARM9: How to port linux2.6.38 kernel to TQ2440
Recommended ReadingLatest update time:2024-11-23 03:36
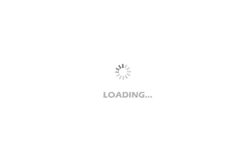
- Popular Resources
- Popular amplifiers
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
- STMicroelectronics discloses its 2027-2028 financial model and path to achieve its 2030 goals
- 2024 China Automotive Charging and Battery Swapping Ecosystem Conference held in Taiyuan
- State-owned enterprises team up to invest in solid-state battery giant
- The evolution of electronic and electrical architecture is accelerating
- The first! National Automotive Chip Quality Inspection Center established
- BYD releases self-developed automotive chip using 4nm process, with a running score of up to 1.15 million
- GEODNET launches GEO-PULSE, a car GPS navigation device
- Should Chinese car companies develop their own high-computing chips?
- Infineon and Siemens combine embedded automotive software platform with microcontrollers to provide the necessary functions for next-generation SDVs
- Continental launches invisible biometric sensor display to monitor passengers' vital signs
- Oscilloscope helps measure harmonic content calculation
- Common Linux commands in the Corelink SinA33 development board virtual machine
- Tkinter study notes——by Changjianze1
- CircuitPython 6.0.0-alpha.3 released
- EEWORLD University Hall ---- Basics of Analog Electronic Technology Du Xiangyu from National University of Defense Technology
- 4412 development board Qt network programming-TCP implementation server and client
- [New Driving Force Technology MM32F031C6 Development Board Review] Summary Post
- What is the relationship between embedded systems and microcontrollers?
- [Automatic clock-in walking timer system based on face recognition] Unboxing (1): SiPEED Maix Bit (K210)
- Learn more about the application of Xilinx FPGA general-purpose IP cores in communications