PWM generation principle:
The general timer can use the GPIO pin for pulse output. When configured as comparison output and PWM output function, the capture/compare register TIMx_CCR is used as the comparison function, which is referred to as the compare register below. Here is an example to illustrate the PWM output working process of the timer: If the pulse counter TIMx_CNT is configured to count up, and the reload register TIMx_ARR is configured to N, that is, the current count value X of TIMx_CNT is continuously accumulated under the drive of the TIMxCLK clock source. When the value X of TIMx_CNT is greater than N, the value of TIMx_CNT will be reset to 0 and count again. While TIMxCNT is counting, the count value X of TIMxCNT will be compared with the value A pre-stored in the comparison register TIMx_CCR. When the value X of the pulse counter TIMx_CNT is less than the value A of the comparison register TIMx_CCR, a high level (or low level) is output. On the contrary, when the value X of the pulse counter is greater than or equal to the value A of the comparison register, a low level (or high level) is output. In this way, the output pulse period is the value (N+1) stored in the reload register TIMx_ARR multiplied by the clock period of the trigger pulse, and the pulse width is the value A of the comparison register TIMx_CCR multiplied by the clock period of the trigger pulse, that is, the duty cycle of the output PWM is A/(N+1).
STM32 generates PWM configuration method:
1. GPIO port configuration:
When configuring the IO port, you just need to turn on the clock, select the pin, mode, rate, and finally initialize it with a structure. However, on 32, not every IO pin can be used directly for PWM output, because the hardware has specified that certain pins are used to connect to the PWM output port. The following is the pin remapping of the timer, which is actually the multiplexing function selection of the pin:
a. Pin configuration and multiplexing function image of timer 1/8:
According to the above remapping table, we use the channel TIM1__CH2 of timer 1 as the output channel of PWM. If the PA9 pin is selected, it should be configured as follows:
{
GPIO_InitTypeDef GPIO_InitStructure; //Define the structure
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO, ENABLE);
// Enable GPIO peripheral and AFIO multiplexing function module clock
//GPIO_PinRemapConfig(GPIO_FullRemap_TIM1, ENABLE); //Select Tim1 full remapping, that is, use PE11
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; //TIM_CH2
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP; //Multiplex push-pull function
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure); //Initialize pin
}
GPIO configuration to generate six PWM complementary outputs:
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO |RCC_APB2Periph_GPIOA|RCC_APB2Periph_GPIOB,ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_8|GPIO_Pin_9|GPIO_Pin_10; //PA8、9、10
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13|GPIO_Pin_14|GPIO_Pin_15; //PB13、14、15
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
TIM1 settings to generate six PWM complementary outputs:
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure; //Define the time base structure
TIM_OCInitTypeDef TIM_OCInitStructure; //Define output structure
TIM1_BDTRInitTypeDef TIM1_BDTRInitStructure; //Define the dead zone structure
RCC_APB2PeriphClockCmd(RCC_APB1Periph_TIM1, ENABLE); //Enable timer 1 clock
TIM_TimeBaseStructure.TIM_Period = arr; //Automatically reload the value of register ARR
TIM_TimeBaseStructure.TIM_Prescaler =psc; //The value of TIM1 prescaler PSC
TIM_TimeBaseStructure.TIM_ClockDivision = 0; //Clock division, no frequency division
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; //Count up
TIM_TimeBaseInit(TIM1, &TIM_TimeBaseStructure); //Initialize the timer according to the above functions
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1; //Select timer mode, TIM pulse width modulation mode 2
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable; //Comparison output enable
TIM_OCInitStructure.TIM_OutputState = TIM_OutputNState_Enable; //Complementary output enable
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_Low; //Output comparison polarity
TIM_OCInitStructure.TIM_OCNPolarity = TIM_OCNPolarity_Low; // Output complementary polarity
//TIM1_OCInitStructure.TIM1_OCIdleState = TIM1_OCIdleState_Reset; //Output status after dead zone
//TIM1_OCInitStructure.TIM1_OCNIdleState = TIM1_OCIdleState_Reset; //Complementary output status after dead zone
TIM_OCInitStructure.TIM_Pulse = ccr1; //Set channel CH1/CH1N duty cycle
TIM_OC1Init(TIM1, &TIM_OCInitStructure); //Channel CH1/CH1N initialization
TIM_OCInitStructure.TIM_Pulse = ccr2; //Set channel CH2/CH2N duty cycle
TIM_OC2Init(TIM1, &TIM_OCInitStructure); //Channel CH2/CH2N initialization
TIM_OCInitStructure.TIM_Pulse = ccr3; //Set channel CH3/CH3N duty cycle
TIM_OC3Init(TIM1, &TIM_OCInitStructure); //Channel CH3/CH3N initialization
TIM_OC1PreloadConfig(TIM1, TIM_OCPreload_Enable);
TIM_OC2PreloadConfig(TIM1, TIM_OCPreload_Enable);
TIM_OC3PreloadConfig(TIM1, TIM_OCPreload_Enable);
TIM1_BDTRInitStructure.TIM1_OSSRState = TIM1_OSSRState_Enable; //Output selection in operation mode
TIM1_BDTRInitStructure.TIM1_OSSIState = TIM1_OSSIState_Enable; //Output selection in idle mode
TIM1_BDTRInitStructure.TIM1_LOCKLevel = TIM1_LOCKLevel_OFF; //Lock setting
TIM1_BDTRInitStructure.TIM1_DeadTIM1 = 0xF2; //Dead time, 2us
TIM1_BDTRInitStructure.TIM1_Break = TIM1_Break_Enable; //brake function enable
TIM1_BDTRInitStructure.TIM1_BreakPolarity =TIM1_BreakPolarity_High; //Brake input polarity
TIM1_BDTRInitStructure.TIM1_AutomaticOutput =TIM1_AutomaticOutput_Enable; //Automatic output enable
TIM1_BDTRConfig(&TIM1_BDTRInitStructure);
TIM_Cmd(TIM1, ENABLE); //Enable TIM1
TIM1_CtrlPWMOutputs(ENABLE); //Enable the main output of TIM1
}
At this point, the configuration of using TIM1 to generate 6 PWM complementary outputs is completed.
TIM1 output initialization default value:
{
TIM1_OCMode = TIM1_OCMode_Timing;
TIM1_OutputState = TIM1_OutputState_Disable;
TIM1_OutputNState = TIM1_OutputNState_Disable;
TIM1_Pulse = TIM1_Pulse_Reset_Mask;
TIM1_OCPolarity = TIM1_OCPolarity_High;
TIM1_OCNPolarity = TIM1_OCNPolarity_High;
TIM1_OCIdleState = TIM1_OCIdleState_Reset;
TIM1_OCNIdleState = TIM1_OCNIdleState_Reset;
}
TIM1 dead zone initialization default value:
{
TIM1_OSSRState = TIM1_OSSRState_Disable;
TIM1_OSSIState = TIM1_OSSIState_Disable;
TIM1_LOCKLevel = TIM1_LOCKLevel_OFF;
TIM1_DeadTime = TIM1_DeadTime_Reset_Mask;
TIM1_Break = TIM1_Break_Disable;
TIM1_BreakPolarity = TIM1_BreakPolarity_Low;
TIM1_AutomaticOutput = TIM1_AutomaticOutput_Disable;
}
Main tasks completed:
1. I have a deeper understanding of the Hall-less BLDC motor program. I tried to modify the program to change the zero-crossing detection mode of PWM_OFF to PWM_ON, delete the redundant parts of the program, and make detailed comments on the program segment of the TIM advanced timer to deepen my impression.
2. Understand and interpret the Hall BLDC motor program, and basically understand its operating principle and program writing method.
BLDC motor with Hall element:
The difference between the motor without Hall element and the motor without Hall element is that it lacks the startup detection, but has the EXTI external interrupt. The motor rotation program is in this interrupt service subroutine. The detection of Hall element uses three interrupt lines to capture the rotor position.
Its PWM output is the same as that of the BLDC motor without Hall elements. The commutation also uses six-step commutation, which is the Hall six-step commutation, but there is no zero-crossing detection, that is, no ADC peripheral involvement.
Previous article:[STM32 motor vector control] Record 5 - FOC principle
Next article:[STM32 motor square wave] Record 3——TIM1 time base initialization configuration
Recommended ReadingLatest update time:2024-11-16 14:55
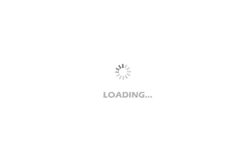
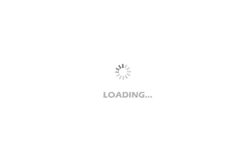
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
-
Intelligent Control Technology of Permanent Magnet Synchronous Motor (Written by Wang Jun)
-
usb_host_device_code
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications