Serial port byte sending process:
Program the M bit of USARTx_CR1 to define the word length.
Program the STOP bit in USARTx_CR2 to define the number of stop bits.
Program the USARTx_BRR register to determine the baud rate.
Enable the UE bit of USARTx_CR1 to enable USARTx.
If multi-buffer communication is performed, configure the DMA enable (DMAT) of USARTx_CR3.
Enabling the TE bit of USARTx_CR1 enables the transmitter.
Write the data to be sent to the transmit data register TDR.
After writing the last data to the TDR register, wait for the TC position of the status register USARTx_ISR to be set to 1 and the transmission is completed.
Configuration steps 1-6: Configure word length, stop bit, parity bit, baud rate, etc.:
HAL_StatusTypeDef HAL_USART_Init(USART_HandleTypeDef * huart);
The identifier HAL_USART_ENABLE will be referenced inside this function to enable the corresponding serial port.
The routine is as follows:
void uart_init(u32 bound)
{
//UART initialization settings
UART1_Handler.Instance=USART1; //USART1
UART1_Handler.Init.BaudRate=bound; //Baud rate
UART1_Handler.Init.WordLength=UART_WORDLENGTH_8B; //Word length 8 bits
UART1_Handler.Init.StopBits=UART_STOPBITS_1; //One stop bit
UART1_Handler.Init.Parity=UART_PARITY_NONE; //No parity bit
UART1_Handler.Init.HwFlowCtl=UART_HWCONTROL_NONE; //No hardware flow control
UART1_Handler.Init.Mode=UART_MODE_TX_RX; //Transmit and receive mode
HAL_UART_Init(&UART1_Handler); //HAL_UART_Init()会使能UART1
HAL_UART_Receive_IT(&UART1_Handler, (u8 *)aRxBuffer, RXBUFFERSIZE);//This function will enable the receive interrupt;
}
Step 7-8 Send data and wait for sending to complete
HAL_StatusTypeDef HAL_USART_Transmit(USART_HandleTypeDef * huart,uint8_t *pTxdata,uint16_t Size,uint32_t Timeout);
First of all, we need to mention the __weak keyword:
A function with the __weak modifier in front of it is called a weak function. For a weak function, the user can redefine a function with the same name in the user file, and the compiler will eventually choose the user-defined function when compiling. If the user does not define it, the content of the function is the content of the weak function definition.
Benefits of the __weak keyword:
1. For a pre-defined process, we only want to modify a part of the code related to the user in the process. At this time, we can use a weak function to define an empty function, and then let the user define the function. The advantage of this is that we will not make any changes to the existing program flow.
2. The __weak keyword is widely used in the HAL library to modify peripheral callback functions.
3. The peripheral callback function is used by users to write MCU-related programs, which greatly improves the versatility and portability of the program.
The serial port handle is as follows:
We can see that there is a serial port parameter initialization structure in the serial port handle, the specific content is as follows:
Next, we will describe the overall configuration process of the serial port sending program (HAL library):
1. Initialize serial port related parameters and enable serial port: HAL_USRT_Init();
2. Configure serial port related IO ports and multiplex configuration:
call HAL_GPIO_Init() in HAL_UART_MspInit;
3. Send data and wait for data transmission to be completed:
HAL_UART_Transmit();
Previous article:STM32 beginner's guide (day 6) -------Serial communication
Next article:STM32 serial communication sending and receiving
Recommended ReadingLatest update time:2024-11-17 00:48
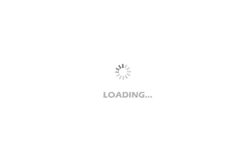
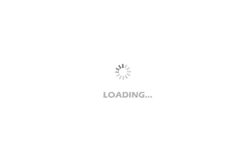
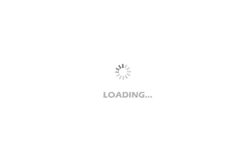
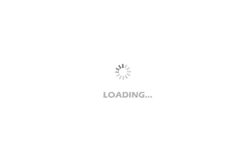
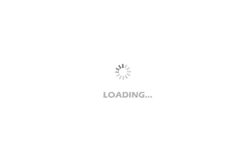
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- High-definition pictures to understand the high-end precision HDI board PCB architecture
- [Perf-V Evaluation] Build and apply Hummingbird open source SOC based on Perf-V development board
- 【GD32307E-START】03- Schematic diagram of expansion board
- Classification of DSP processors
- When BLE meets MEMS——Device Selection
- Oracle laid off employees again, rumored to be N+6 compensation, some people received 1 million compensation
- GPIO pin multiplexing in C6455
- Discussion on the practical ability of silicone pads
- Shenzhen Electronic Hardware Engineer
- "Blink" on the "New Version of Bluesight AB32VG1 RISC-V Development Board"