STM32 PWM output
Pulse width mode (PWM mode) can be used to generate a signal with a frequency determined by the TIMx_ARR register and a duty cycle determined by the TIMx_CCRx register. In STM32 development, the official library functions provide a relatively complete set of functions, making our development work quite easy. We can even complete our development work and achieve the functions we need without knowing too much about the hardware structure. Here, the author also recommends that you try to familiarize yourself with the functions provided in the firmware library to adjust the frequency and duty cycle of PWM when you are just starting out, and minimize the operation of the underlying registers.
This article uses the STM32F103RB chip, and the output channel is the TIM2_CH2 channel. STM32 has strong portability. If the reader's chip type is different from mine, you can make appropriate modifications to complete your own development.
The library functions used are:
stm32f10x.h: used for system initialization. No matter what development you do, this library must include
stm32f10x_tim.h: TIM timer library function
stm32f10x_rcc.h: clock configuration library function
stm32f10x_gpio.h: GPIO configuration library functions
From the above library functions, we can see that the contents we need to initialize include TIM2 timer, clock enable configuration, and GPIO enable configuration.
void RCC_Config(void);
void GPIO_Config(void);
void TIM_Config(void);
The next step is to write the function body of each function. In these contents, the official has actually given examples. We configure them according to the official function library, and then modify some official variable properties.
RCC_Config function body
void RCC_Config(void)
{
// Enable GPIOA, TIM2
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA,ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2,ENABLE);
}
GPIO_Config function body
void GPIO_Config(void)
{
//GPIO configuration, the official library gives some parameters that need to be configured. If you forget, just refer to it. I configure GPIOA_Pin_1 here.
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin=GPIO_Pin_1;
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed=GPIO_Speed_50MHz;
GPIO_Init(GPIOA,&GPIO_InitStructure);
}
TIM_Config function body
Before configuring the function body, first understand how the duty cycle and frequency of stm32 are calculated
①Frequency: The APB1 clock source we use is 72MHz. We do not do frequency division here. We set the input frequency by configuring related parameters. The calculation method is: Input frequency = APB1 clock / (pre-division coefficient + 1) = 72000000Hz / 360 = 200000Hz
②The TIM_TImeBaseStructure.TIM_Period parameter determines the frequency of the output PWM waveform. The frequency of the output PWM waveform = the input frequency of the timer / TIM_TImeBaseStructure.TIM_Period. In this example, 20000Hz/100=200Hz, that is, one cycle of 5ms
③Configure the duty cycle: Duty cycle = configured duty cycle value / TIM_TImeBaseStructure.TIM_Period. Use this calculation to determine the duty cycle. In this case, the duty cycle is 50/100=50%
④Timer enable
void TIM_Config(void)
{
TIM_TimeBaseInitTypeDef TIM_TImeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
//Configure the clock output frequency of TIM2 and initialize other related parameters
TIM_TImeBaseStructure.TIM_Prescaler=360-1; //Set the frequency of PWM
TIM_TImeBaseStructure.TIM_CounterMode=0;
TIM_TImeBaseStructure.TIM_Period=100;
TIM_TimeBaseInit(TIM2,&TIM_TImeBaseStructure);
//Set the PWM output mode
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM2;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_OutputNState = TIM_OutputNState_Enable;
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OCInitStructure.TIM_OCNPolarity = TIM_OCNPolarity_High;
TIM_OCInitStructure.TIM_OCIdleState = TIM_OCIdleState_Set;
TIM_OCInitStructure.TIM_OCNIdleState = TIM_OCIdleState_Reset;
//Configure the duty cycle
TIM_OCInitStructure.TIM_Pulse=50;
TIM_OC2Init(TIM2,&TIM_OCInitStructure);
TIM_ForcedOC1Config(TIM2,TIM_ForcedAction_Active);
TIM_Cmd(TIM2,ENABLE);
TIM_CtrlPWMOutputs(TIM2,ENABLE);
}
Thus, our entire PWM configuration is completed
Main function
int main()
{
RCC_Config();
GPIO_Config();
TIM_Config();
while(1)
{
;
}
}
Next, let's look at the output frequency and waveform of our GPIOA_Pin_1 pin in Keil.
Keil software simulation
①Configure debugging tools
② Turn on debugging, and set and test the GPIO output pins
③Run at full speed and observe the oscilloscope
STM32-PWM output source code download address
STM32 firmware library version 3.5 user manual Chinese translation version
Previous article:Adjustable PWM output based on stm32 microcontroller
Next article:STM32 study notes: general timer output PWM
Recommended ReadingLatest update time:2024-11-16 20:52
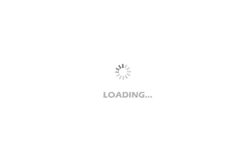
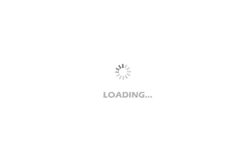
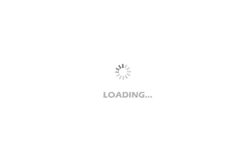
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
-
Intelligent Control Technology of Permanent Magnet Synchronous Motor (Written by Wang Jun)
-
usb_host_device_code
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Questions about the two array forms of LSM6DSO FSM gesture recognition?
- What are the advantages and disadvantages of complementary push-pull H-bridge (2 NMOS + 2 PMOS) and totem pole H-bridge (4 NMOS), and their applications...
- The problem of turning frequency in LC filter circuit design
- Live broadcast at 10 am today [The rapid growth of Renesas RA MCU family members helps build safe and stable industrial control systems]
- Evaluation Weekly Report 20220411: GigaDevice's value-for-money MCU F310 is here, TI's heterogeneous multi-core AM5708 thousand yuan industrial board
- Program space issues
- Buy more time with the Raspberry Pi Pico
- Is this seller a scam?
- RT-Thread device framework learning PIN device
- [Raspberry Pi Pico Review] 4. Connect Pico to the temperature sensor DS18B20 to read the temperature