/*Ultrasonic distance measurement program, using the external interrupt of 51 MCU. If it is not working well, it is recommended to use Dupont wire to connect the pins directly*/
#include
#include
typedef unsigned char uchar;
typedef unsigned int uint;
/*The ultrasonic module is HC-SR04. The trigger signal is a high level of at least 10us. After that, the module will automatically send 8 40kHz square wave signals.
Once the module receives the echo signal, the Echo pin will output a high level until it stops receiving the signal.
The calculation is based on the duration of the high level of the Echo pin. However, shouldn't the sound wave's journey start when the sound wave is emitted?
For the second calculation, if you want to change it, please change the start time of timer 0. */
sbit Echo = P3^2; //The echo signal output of the ultrasonic module, P3^2 is also the input terminal of the 51 MCU external interrupt 0, using the falling edge to trigger the interrupt
sbit Trig=P1^4; //trigger signal pin
sbit lcdrs = P1^0; //Data command selection terminal, write command when 0, write data when 1
sbit lcdrw = P1^1; // read and write command selection terminal, write data when 0, read data when 1, set to 1 when reading status, and 0 at other times;
sbit lcden=P2^5; //enable terminal
sbit dula=P2^6; //Not useful in this program
sbit wela=P2^7;
sbit STA7=P0^7; //D0~D7 data ports correspond to P0^0~P0^7. When reading LCD data, STA7 corresponds to P0^7. When STA7 is 1, it means the LCD is busy and cannot receive data.
float dis; //distance cache
uchar flag; //interrupt flag
char code table[]="distance:"; //Display characters at specified positions
char code table1[]="cm";
void delayms(uchar x)
{
uint i,j;
for(i=0;i { for(j=0;j<110;j++); } } void nops() //Delay 10.9us, each machine cycle is about 1.09us, when the crystal oscillator is 11.0592MHz { _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); _nop_(); } void distance()//Function to calculate distance { Trig=0; //First pull the trigger low nops(); Trig=1; //Give a high level of at least 10us to start the module nops(); nops(); Trig=0; //The module has been triggered at this time, and the timer count will be started immediately TR0=1; //Turn on timer 0 EX0=1; //Open external interrupt, external interrupt input is P3^2, falling edge is valid, trigger interrupt delayms(1); //Wait a moment. One machine cycle is about 1.09us. If you don't wait, you may miss the calculation of dis and fall into an infinite loop, and you will never get the value. if(flag==1) //If the flag is set to 1, it means that the Echo output is at a falling edge, i.e. the reception is finished { flag=0; } } void wait()//Wait for LCD to be idle { P0=0xff; do { lcdrs=0; //These statements in the loop are the operation timing when reading the status lcdrw=1; lcden=0; lcden=1; }while(STA7==1); //Wait until the LCD is idle before looping lcden=0; //enable to 0 } void write_com(uchar com) //write command program { wait(); lcdrs=0; // Set RS to 0, the data sent will be regarded as a command by the LCD instead of real data, which is the only difference from the data writing program P0=com; lcdrw=0; //Always write, so it is 0 delayms(5); // Make the data stable, so that the LCD has time to read lcden=1; //According to the operation sequence, enable to output a high pulse. This sentence and the following sentence together constitute a high pulse delayms(5); lcden=0; } void write_date(uchar date) //Write data program { wait(); lcdrs=1; //Note that this is writing data P0=date; lcdrw=0; delayms(5); lcden=1; delayms(5); lcden=0; } /* Initialization function */ void init() { wait(); dula=0;//useless wela=0; lcden=0; write_com(0x38); //Set 16*2 display, 5*7 dot matrix, 8-bit data port. This sentence is unchanged write_com(0x0c);//Status word set according to operation sequence. Same as below write_com(0x06); TMOD=0x01; //16-bit counter, there is no need to enable the timer interrupt here, because the timer interrupt has nothing to do, as long as the value in the timer is OK, so there is no need to set TF0 to 1 TH0=0; //Set all to 0 TL0=0; IT0=1; //Set external interrupt falling edge to be effective EA=1; //Open the general interrupt } /*Display function*/ void display(float dis) { uint bai,shi,ge,p1,p2; // respectively, the hundreds place down to two decimal places bai=dis/100; shi=(dis-bai*100)/10; ge=dis-bai*100-shi*10; p1=(dis*10)-bai*1000-shi*100-ge*10; p2=(dis*100)-bai*10000-shi*1000-ge*100-p1*10; write_date(0x30+bai); //Convert numbers to characters, must be +0x30, in addition, 1602 LCD can only receive character data write_date(0x30+shi); write_date(0x30+ge); write_date('.'); write_date(0x30+p1); write_date(0x30+p2); } void main()//main function { uchar i=0; init(); write_com(0x80); //Set the address, display distance in the first row and first column: 0x80 is the starting address of the first row while(table[i]!='\0') { write_date(table[i]); i++; delayms(5); } i=0; write_com(0x80+0x40+6); //Set the address, display cm in the 7th position of the second line, 0x80+0x40 is the starting address of the second line while(table1[i]!='\0') { write_date(table1[i]); i++; delayms(5); } while(1) { distance(); write_com(0x80+0x40); //Set the display address of the data to the start position of the second line, and then refresh the display at this place during the loop display(dis); delayms(60); } } void ex() interrupt 0 //interrupt function of external interrupt { TR0=0; //Once receiving a falling edge, the timer will stop counting immediately dis=(TH0*256+TL0)*1.09/58; //First take out the time value in the timer, then set the timer to 0 flag=1; //Set the flag position to 0 TH0=0; TL0=0; }
Previous article:Electronic clock (51 single chip timer, 1602 LCD)
Next article:51 MCU 1602 LCD mobile display
Recommended ReadingLatest update time:2024-11-16 14:59
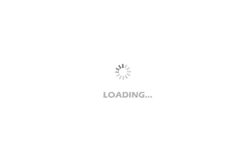
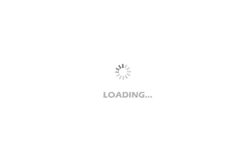
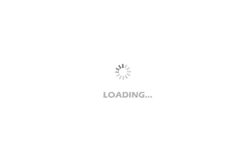
- Popular Resources
- Popular amplifiers
-
Single-chip microcomputer C language programming and simulation
-
100 Examples of Microcontroller C Language Applications (with CD-ROM, 3rd Edition) (Wang Huiliang, Wang Dongfeng, Dong Guanqiang)
-
Principles and Applications of Single Chip Microcomputers and C51 Programming (3rd Edition) (Xie Weicheng, Yang Jiaguo)
-
stm32+lcd1602 example
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- CPU card programming system main frequency setting
- EEWORLD University ---- Automotive eCall Power Solution
- Because he mastered the company's "core technology", the boss hired several strong men to kidnap people on the street after leaving the company
- It's time to test your eyesight
- How to not set a password in AP mode
- LCD Segment Screen Screen Printing Notes
- hfss18 version 3D image setting problem
- The network transformer output does not connect to RJ45, but uses a custom interface!
- EEWORLD University Hall----Live Replay: TI Sitara? Multi-protocol Industrial Communication Optimization Solution, PLC Demo Real-time Demonstration
- [NXP Rapid IoT Review] Reduce the CPU frequency (K64) to save power