a small design for practice, a little ugly. Attached is the program and the principle PCB diagram. It is
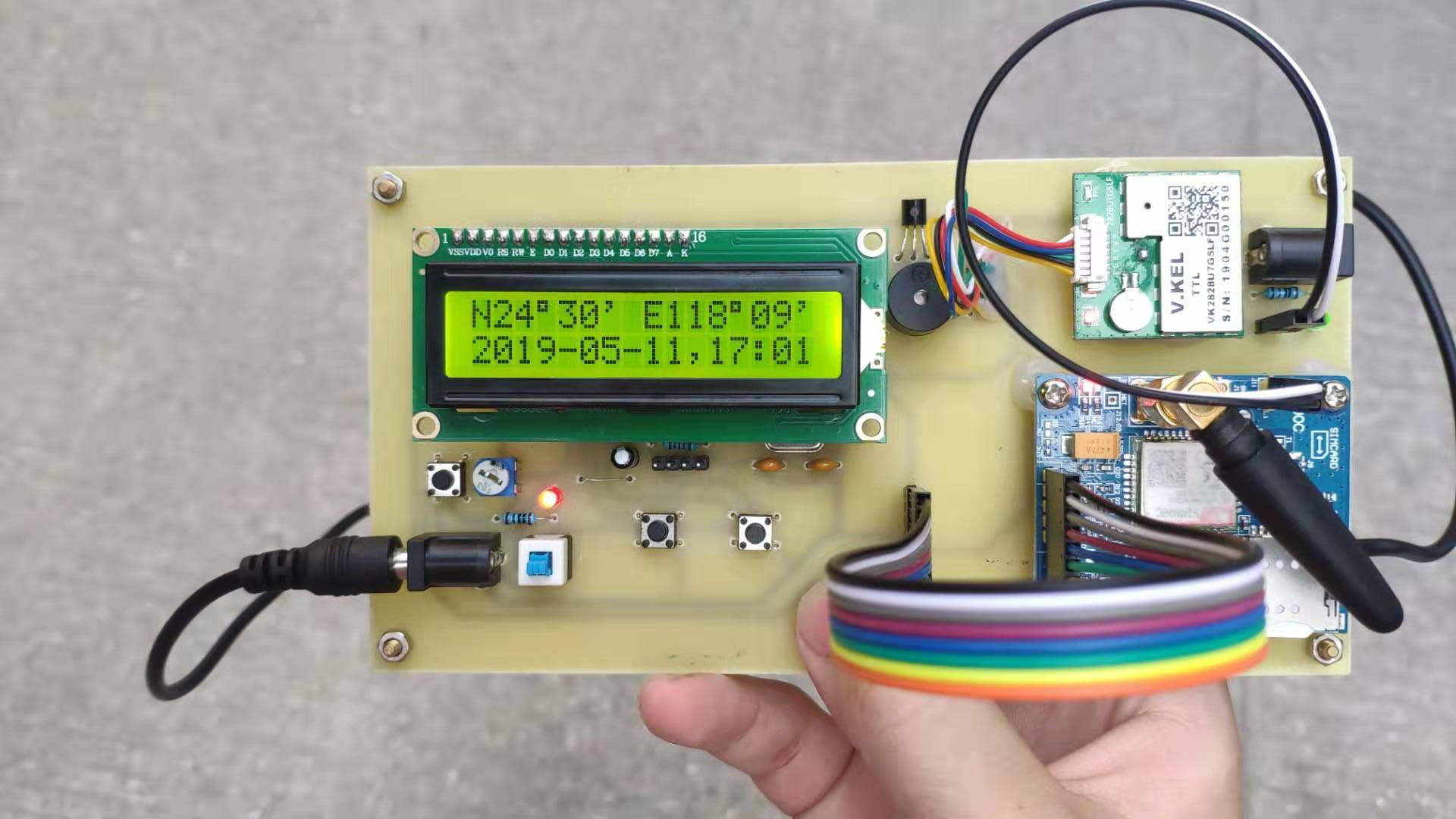
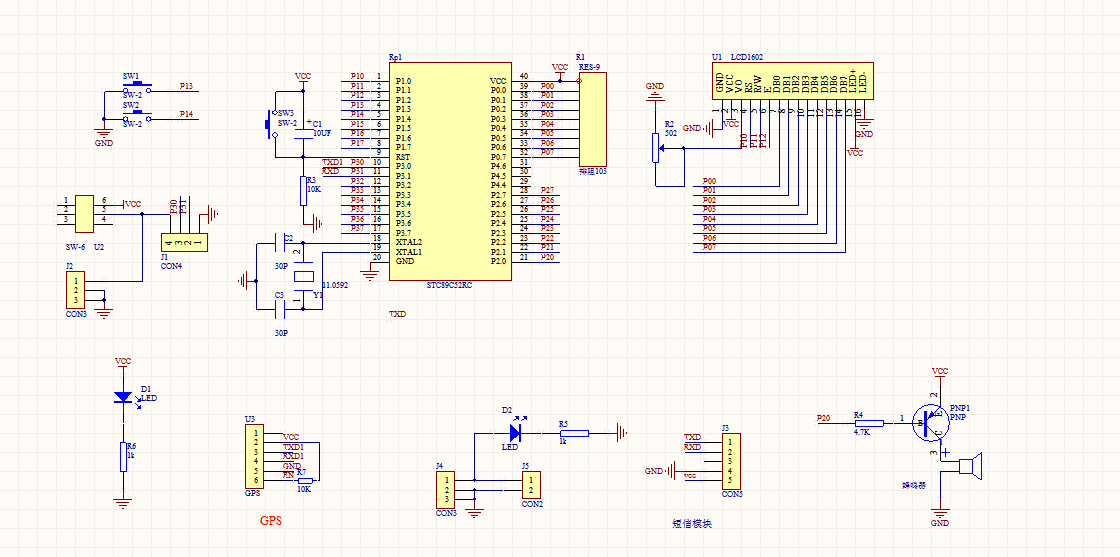
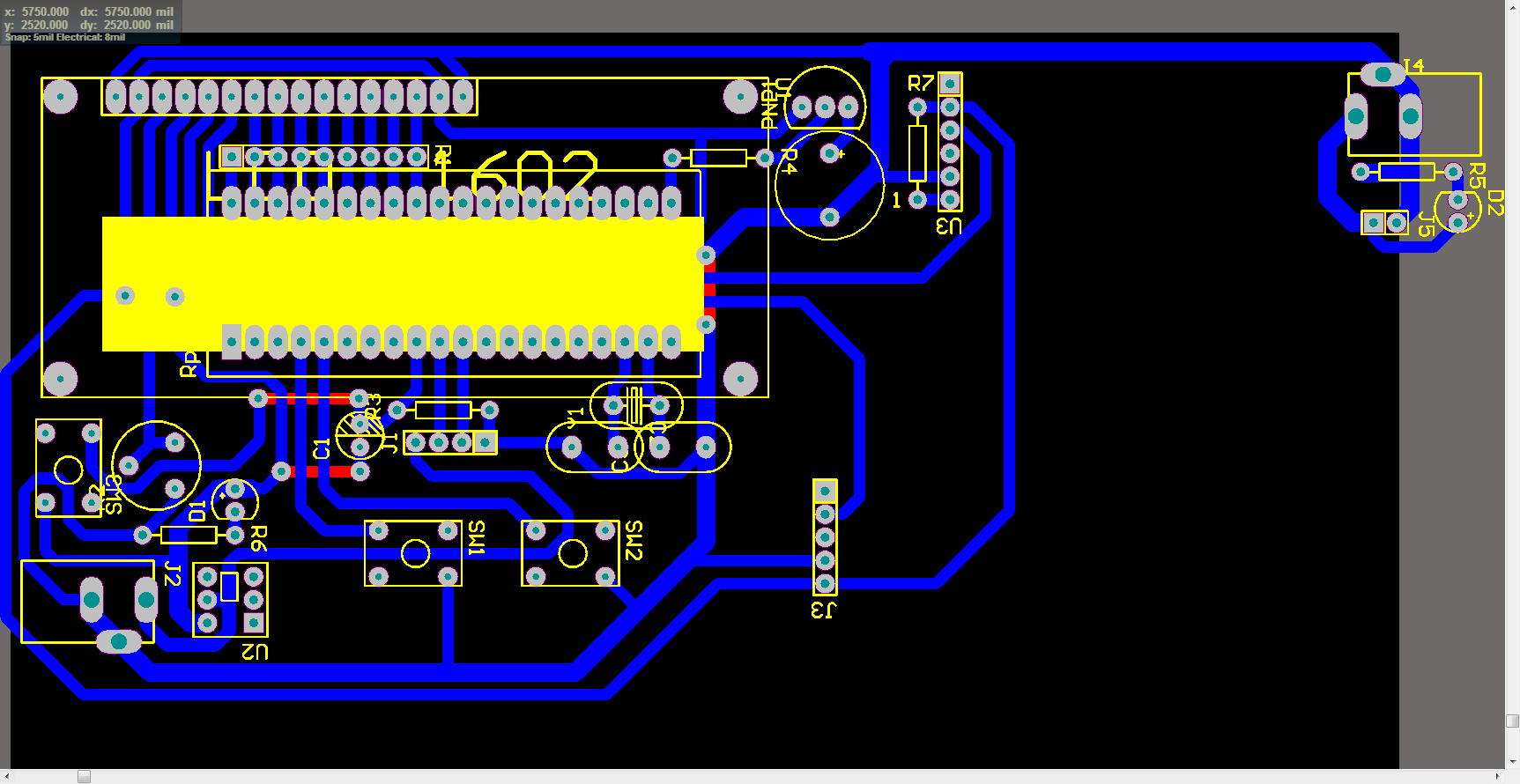
my first post, please forgive me if it is not good.
Button function: one button alarms, buzzer sounds, another button sends a text message to the specified mobile phone, the mobile phone number is written in the program.
The microcontroller source program is as follows:
#include "common.h"
#include "uart.h"
#include "gps.h"
#include "gsm.h"
#include "lcd.h"
#define GPS_STR_LEN 48
fly ess_inc;
//char xdata GPS_SEND_STR[GPS_STR_LEN];
sbit key = P1^3;
sbit key1 = P1^4;
sbit GPS_EN=P3^4;
sbit led1 = P3^3;
#define RMC_YES led1 = 0
#define RMC_NO led1 = 1
GPS_INFO GPS; //GPS information structure
bit GPS_rx_flag = 0;
bit GPS_Have_flag = 0;
#define SEND_NUMBER "AT+CMGS="13420106297"rn"
char xdata send_data[37]="N: ',E: ',2000-00-00,00:00rn";
unsigned char send_len= 0;
bit dis_flag = 1;
bit sendflag=0;
/****************************************
display time
/****************************************/
void GPS_DispTime(void)
{
fly i = 0;
fly ch;
char time[5];
Int_To_Str(GPS.D.year,time);//年
LCD1602_Set_AC(0, 1);
i = 0;
send_len = 18;
while(time[i] != '')
{
ch = time[i++];
LCD1602_write_data(ch);
send_data[send_len++] = ch;
}
LCD1602_write_data('-');
Int_To_Str(GPS.D.month,time);//月
LCD1602_Set_AC(5, 1);
i = 0;
send_len = 23;
while(time[i] != '')
{
ch = time[i++];
LCD1602_write_data(ch);
send_data[send_len++] = ch;
}
LCD1602_write_data('-');
Int_To_Str(GPS.D.day,time);//日
LCD1602_Set_AC(8, 1);
i = 0;
send_len = 26;
while(time[i] != '')
{
ch = time[i++];
LCD1602_write_data(ch);
send_data[send_len++] = ch;
}
LCD1602_write_data(',');
Int_To_Str(GPS.D.hour,time);//time
LCD1602_Set_AC(11, 1);
i = 0;
send_len = 29;
while(time[i] != '')
{
ch = time[i++];
LCD1602_write_data(ch);
send_data[send_len++] = ch;
}
LCD1602_write_data(':');
Int_To_Str(GPS.D.minute,time);//分
LCD1602_Set_AC(14, 1);
i = 0;
send_len = 32;
while(time[i] != '')
{
ch = time[i++];
LCD1602_write_data(ch);
send_data[send_len++] = ch;
}
}
void GPS_DisplayOne(void)
{
flying len,ch, i;
char info[10];
ess_inc = 0;
// memset(GPS_SEND_STR, 0, GPS_STR_LEN);
if (GPS.NS == 'N') //Judge whether it is north latitude or south latitude
{
if(dis_flag)
LCD1602_DisplayChar(0, 0, 'N');
else
LCD1602_DisplayChar(0, 0, ' ');
// GPS_SEND_STR[ess_inc ++] = 'N';
only = 0;
Int_To_Str(GPS.latitude_Degree,info); //Latitude
LCD1602_Set_AC(1, 0);
i = 0;
send_len = 2;
while(info[i] != '')
{
ch = info[i++];
if(dis_flag)
LCD1602_write_data(ch);
else
LCD1602_write_data(' ');
send_data[send_len++] = ch;
// GPS_SEND_STR[ess_inc ++] = ch;
only ++;
}
send_data[send_len++] = 'd';
if(dis_flag)
LCD1602_write_data(0xDF);
else
LCD1602_write_data(' ');
// GPS_SEND_STR[ess_inc ++] = 'd';
only ++;
Int_To_Str(GPS.latitude_Cent,info); //纬分
i = 0;
while(info[i] != '')
{
ch = info[i++];
if(dis_flag)
LCD1602_write_data(ch);
else
LCD1602_write_data(' ');
send_data[send_len++] = ch;
// GPS_SEND_STR[ess_inc ++] = ch;
only ++;
}
if(dis_flag)
LCD1602_write_data(0x27);
else
LCD1602_write_data(' ');
// GPS_SEND_STR[ess_inc ++] = 'm';
Int_To_Str(GPS.latitude_Second,info); //纬秒
i = 0;
while(info[i] != '')
{
ch = info[i++];
// GPS_SEND_STR[ess_inc ++] = ch;
}
// GPS_SEND_STR[ess_inc ++] = 's';
only ++;
while(7 - len)
{
only ++;
LCD1602_write_data(' ');
}
}
// GPS_SEND_STR[ess_inc] = ',';
ess_inc += 1;
if (GPS.EW == 'E') //Judge whether it is east longitude or west longitude
{
if(dis_flag)
LCD1602_DisplayChar(8, 0, 'E');
else
LCD1602_write_data(' ');
// GPS_SEND_STR[ess_inc ++] = 'E';
only = 0;
Int_To_Str(GPS.longitude_Degree,info); //Longitude
LCD1602_Set_AC(9, 0);
i = 0;
send_len = 11;
while(info[i] != '')
{
ch = info[i++];
if(dis_flag)
LCD1602_write_data(ch);
else
LCD1602_write_data(' ');
send_data[send_len++] = ch;
// GPS_SEND_STR[ess_inc ++] = ch;
only ++;
}
send_data[send_len++] = 'd';
if(dis_flag)
LCD1602_write_data(0xDF);
else
LCD1602_write_data(' ');
// GPS_SEND_STR[ess_inc ++] = 'd';
only ++;
Int_To_Str(GPS.longitude_Cent,info); //Longitude cents
i = 0;
while(info[i] != '')
{
ch = info[i++];
if(dis_flag)
LCD1602_write_data(ch);
else
LCD1602_write_data(' ');
send_data[send_len++] = ch;
// GPS_SEND_STR[ess_inc ++] = ch;
only ++;
}
if(dis_flag)
LCD1602_write_data(0x27);
else
LCD1602_write_data(' ');
// GPS_SEND_STR[ess_inc ++] = 'm';
Int_To_Str(GPS.longitude_Second,info); //Longitude seconds
i = 0;
while(info[i] != '')
{
ch = info[i++];
// GPS_SEND_STR[ess_inc ++] = ch;
}
// GPS_SEND_STR[ess_inc ++] = 's';
only ++;
while(7 - len)
{
only ++;
LCD1602_write_data(' ');
}
}
GPS_DispTime();
// GPS_SEND_STR[ess_inc] = ' ';
ess_inc += 1;
if(sendflag==1)
{
sendflag = 0;
gsm_send_englishmsg(SEND_NUMBER,send_data);//Send location SMS
delay_ms(1000);
delay_ms(1000);
LCD1602_DisplayString(0,0," SEND OK ");
delay_ms(1000);
LCD1602_DisplayString(0,0," ");
}
// GPS_DispSpeed(GPS.speed, 10, 1);
// if (GPS.D.second == 0)
// {
// if (GPS_Have_flag)
// {
// gsm_send_englishmsg(phonenum,GPS_SEND_STR); //Send location SMS
// }
// else
// {
// //gsm_send_englishmsg(phonenum,"GPS No Signal");//Send SMS
// }
// }
}
void main(void)
{
fly error_num = 0;
uint count = 0;
GPS_EN = 0;
GPS_rx_flag = 0;
LCD1602_Initialize(); //LCD1602 display initialization
uart_init(UART_B9600);
GPS_EN = 1;
GPS_rx_flag = 1;
BG = 0;
LCD1602_DisplayString_Center(0,"GPS SCAN...");
LCD1602_ClearLine(1);
while(1)
{
if (rev_stop) //If a line is received
{
if (GPS_RMC_Parse(RX1_Buffer, &GPS)) //解析GPRMC
{
RMC_YES;
GPS_DisplayOne(); //Display the first page of GPS information
error_number = 0;
gps_flag = 0;
rev_stop = 0;
GPS_Have_flag = 1;
……………………
Previous article:Simple game Proteus simulation program based on LCD12864 and 51 single chip microcomputer
Next article:Ultra-small wireless transceiver module LT8920 configuration ideas + microcontroller transmission and reception program
Recommended ReadingLatest update time:2024-11-16 17:58
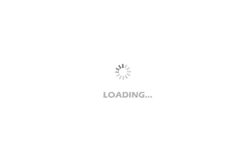
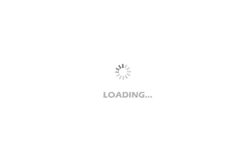
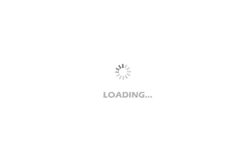
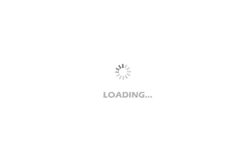
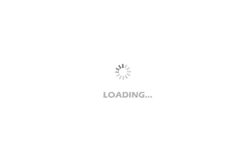
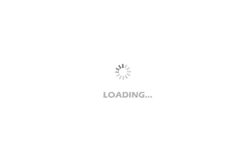
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- [SC8905 EVM Review] + Unboxing test and driver installation
- How to detect inductor saturation | Comment and win a gift!
- Optimizing EMC and Efficiency in High Power DC/DC Converters Part 2
- [McQueen Trial] + Line-following Driving + Ultrasonic Obstacle Avoidance
- Can the freewheeling diode be omitted when the MOS tube controls the solenoid valve?
- STEVAL-MKSBOX1V1 (SensorTile.box) firmware download via USB
- Gallium nitride is a key technology for realizing 5G
- STM32 output 4-20MA or 0-10V circuit sharing
- LIS2MDL array PCB engineering and code information for magnetic nail navigation AGV car
- Live Review: Rochester Electronics Semiconductor Full-Cycle Solutions (including videos, materials, Q&A)