Proteus simulation circuit diagram
C language program
#include
#include
#define DA0832 XBYTE[0xfffe]
#define uchar unsigned char
#define uint unsigned int
sbit S1 = P1^0;
sbit S2 = P1^1;
//00 sawtooth wave; 01 square wave; 10 triangle wave; 11 sine wave
uchar code sin_tab[] = //sine wave output table
{
0x80,0x83,0x86,0x89,0x8D,0x90,0x93,0x96,0x99,0x9C,0x9F,0xA2,0xA5,0xA8,0xAB,0xAE,
0xB1,0xB4,0xB7,0xBA,0xBC,0xBF,0xC2,0xC5,0xC7,0xCA,0xCC,0xCF,0xD1,0xD4,0xD6,0xD8,
0xDA,0xDD,0xDF,0xE1,0xE3,0xE5,0xE7,0xE9,0xEA,0xEC,0xEE,0xEF,0xF1,0xF2,0xF4,0xF5,
0xF6,0xF7,0xF8,0xF9,0xFA,0xFB,0xFC,0xFD,0xFD,0xFE,0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,
0xFF,0xFF,0xFF,0xFF,0xFF,0xFF,0xFE,0xFD,0xFD,0xFC,0xFB,0xFA,0xF9,0xF8,0xF7,0xF6,
0xF5,0xF4,0xF2,0xF1,0xEF,0xEE,0xEC,0xEA,0xE9,0xE7,0xE5,0xE3,0xE1,0xDF,0xDD,0xDA,
0xD8,0xD6,0xD4,0xD1,0xCF,0xCC,0xCA,0xC7,0xC5,0xC2,0xBF,0xBC,0xBA,0xB7,0xB4,0xB1,
0xAE,0xAB,0xA8,0xA5,0xA2,0x9F,0x9C,0x99,0x96,0x93,0x90,0x8D,0x89,0x86,0x83,0x80,
0x80,0x7C,0x79,0x76,0x72,0x6F,0x6C,0x69,0x66,0x63,0x60,0x5D,0x5A,0x57,0x55,0x51,
0x4E,0x4C,0x48,0x45,0x43,0x40,0x3D,0x3A,0x38,0x35,0x33,0x30,0x2E,0x2B,0x29,0x27,
0x25,0x22,0x20,0x1E,0x1C,0x1A,0x18,0x16,0x15,0x13,0x11,0x10,0x0E,0x0D,0x0B,0x0A,
0x09,0x08,0x07,0x06,0x05,0x04,0x03,0x02,0x02,0x01,0x00,0x00,0x00,0x00,0x00,0x00,
0x00,0x00,0x00,0x00,0x00,0x00,0x01,0x02,0x02,0x03,0x04,0x05,0x06,0x07,0x08,0x09,
0x0A,0x0B,0x0D,0x0E,0x10,0x11,0x13,0x15,0x16,0x18,0x1A,0x1C,0x1E,0x20,0x22,0x25,
0x27,0x29,0x2B,0x2E,0x30,0x33,0x35,0x38,0x3A,0x3D,0x40,0x43,0x45,0x48,0x4C,0x4E,
0x51,0x55,0x57,0x5A,0x5D,0x60,0x63,0x66,0x69,0x6C,0x6F,0x72,0x76,0x79,0x7C,0x7E
};
void Delay_MS(uint);// delay
void stair(uchar AMP);//sawtooth wave
void square(uchar AMP, uchar THL,uchar TLL);//方波
void trian(uchar AMP); //triangle wave
void sin(); //sine wave
void scan(); //scan function
void main()
{
while(1)
{
//Test one by one
//stair(200);
//square(200,10,10);
//trian(200);
//sin();
scan();
}
}
void scan()
{
if((0 == S1) &&(0 == S2))
stair(200);
else if((1 == S1) && (0 == S2))
square(200,10,10);
else if((0 == S1) && (1 == S2))
trian(200);
else
sin();
}
void Delay_MS(uint n)
{
uint k;
for(n; n >0 ;n--)
for(k = 10; k > 0 ;k--);
}
void stair(uchar AMP)
{
flying i;
for(i = 0 ;i < 255; i++)
{
DA0832 = i;
}
}
void square(uchar AMP, uchar THL, uchar TLL)
{
DA0832 = 255 - AMP;
Delay_MS(THL);
DA0832 = 255;
Delay_MS(TLL);
}
void trian(uchar AMP)
{
flying i;
for(i = 255 - AMP ;i < 255; i++)
{
DA0832 = i;
}
for(i-1 ;i > 255 - AMP; i--)
{
DA0832 = i;
}
}
void sin()
{
flying i;
for(i = 0; i < 255; i++)
{
DA0832 = sin_tab[i];
}
}
Output waveform
Previous article:Microcontroller Experiment - Using Timer to Generate Square Wave
Next article:General purpose timer (interrupt function and PWM output)
Recommended ReadingLatest update time:2024-11-16 17:33
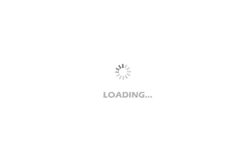
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Inductor and capacitor knowledge points record
- [Anxinke UWB indoor positioning module NodeMCU-BU01] 03. Transplantation preparation: hardware schematic analysis
- What happens when the motor suddenly stops running during operation?
- Vishay Online Library, technical information is waiting for you!
- [National Technology Low Power Series N32L43x Review] 02. Create a template project
- Altium Designer copper patch teardrop setting problem
- [Sipeed LicheeRV 86 Panel Review] 2- Board Resource Introduction and Data Collection
- How much do you know about fast charging? A collection of learning materials on fast charging technology and solutions
- [Xianji HPM6750 Review 1] Experience with two IDE (SES and RS) development platforms
- BlueNRG-x Documentation - Copy and create your own projects