;***************************************************
;* Delay controller made of single-chip microcomputer 89C2051 *
;* T0 and TT0 are combined to form a second pulse generator *
;* TSBIN is a second counter (binary, 0~60); 21H unit *
;* TMBIN is a minute counter (binary, 0~60); 22H unit *
;* THBIN is a hour counter (binary, 0~24); 23H unit *
;* KEYCODE is the keyboard value; 29H unit *
;* KEYPRE is the key mark word; PSW.5 *
;* When the countdown reaches 00H00M00S, P3.0, P3.1 terminals output *
;* inverted signals. *
;* S1----Time button; S2-Minute button; *
;* S3-Second button; S4-Exit time synchronization *
;****************************************************
TMSBIN EQU 20H
TSBIN EQU 21H
TMBIN EQU 22H
THBIN EQU 23H
KEYCODE EQU 29H
TT0 EQU 2BH
OUT EQU 2DH
KEYPRE BIT PSW.5
DPS1 DATA 11001111B ;S4
DPM0 DATA 11010111B ;S3
DPM1 DATA 11011111B ;S2
DPH0 DATA 11100111B ;S1
DPH1 DATA 11101111B
HIDE DATA 11111111B ;Blanking word
ORG 0000H
AJMP MAIN
ORG 000BH
SJMP I_T0
ORG 001BH
I_T1: AJMP MAIN1
RETI
;*********** T0 interrupt service ***********
ORG 0030H
I_T0: PUSH A CC
PUSH PSW
MOV TH0,#0DCH ;0.01s time, constant factor is DC00H
;MOV TL0,#00H
CLR C
MOV A,TMSBIN
SUBB A,#01H
MOV TMSBIN,A
MOV R3,TT0
DJNZ R3,I_T01 ;Interrupt times <100 (0.01S per interrupt), transfer out
CPL KEYPRE
MOV R3,#100 ;Interrupt times = 100 times, restart the next count
MOV TMSBIN,#100
CLR C
MOV A,TSBIN ;Second counter plus 1
SUBB A,#01H
MOV TSBIN,A
;SUBB A,#60 ;Second counter>59? Y, second counter = 00
JNC I_T01
MOV A,TMBIN
ADD A,THBIN
JNZ M1
MOV OUT,#11111101B
CLR TR0
MOV TSBIN,#00H
SJMP I_T01
M1: MOV TSBIN,#59
CLR C
MOV A,TMBIN ;Minute counter plus 1
SUBB A,#01H
MOV TMBIN,A
JNC I_T01
MOV A,THBIN
JZ I_T01
MOV TMBIN,#59
CLR C
MOV A,THBIN ;Hour counter plus 1
SUBB A,#01H
MOV THBIN,A
;SUBB A,#24 ;Hour counter>23? Y, hour counter=00
JNC I_T01
MOV THBIN,#00H
I_T01: MOV TT0,R3
POP PSW
POP ACC
RETI
ORG 00F0H
MAIN: MOV P1,#0FFH
MOV OUT,#11111110B ;P3.0 outputs low level
MOV P3,OUT
MOV SP,#40H ;Initialize stack pointer
MOV TMOD,#11H ;T0, T1 work as 16-bit timer
MOV TT0,#100
MOV TH0,#0DCH ;T0 initial value
MOV TL0,#00H
MOV TH1,#00H ;T1 initial value
MOV TL1,#00H
MOV TSBIN,#59
MOV TMBIN,#59
MOV THBIN,#23
CLR EX0
CLR EX1
CLR ES
SETB ET0 ;Open T0, T1 interrupt
SETB ET1
SETB TR0
SETB TR1
SETB EA
MAIN1: MOV R6,#80H ;Main processing
DISP: MOV KEYCODE,#00H
MOV TH1,#00H
MOV TL1,#00H
ACALL DISPLY ;Call display subroutine
DJNZ R6,DISP
MOV DPTR,#KEY
MOV A,KEYCODE ;Read keyboard value
JMP @A+DPTR ;Press key value to jump to corresponding processing program
KEY: SJMP MAIN1
SJMP KEY1 ; In sequence: KEY value + 2, 4, 6, 8, because SJMP occupies 2 bytes
SJMP KEY2 ; S1's KEYCODE = 02H, S2: KEYCODE = 04H
SJMP KEY3 ; S3: KEYCODE = 06H, S4: KEYCODE = 08H
SJMP KEY4
KEY1: CLR TR0 ; Hour counter plus 1
MOV A, THBIN
CLR C
ADDC A, #01H
MOV THBIN, A
SUBB A, #24 ; 24D
JC KEY11
MOV THBIN, #00H
KEY11: SJMP MAIN1
NOP
KEY2: CLR TR0 ; Minute counter plus 1
MOV A, TMBIN
CLR C
ADDC A, #01H
MOV TMBIN, A
SUBB A, #60 ; 60D
JC KEY21
MOV TMBIN,#00H
KEY21: SJMP MAIN1
NOP
KEY3: CLR TR0 ;Increase the second counter by 1
MOV A,TSBIN
CLR C
ADDC A,#01H
MOV TSBIN,A
SUBB A,#60 ;60D
JC KEY31
MOV TSBIN,#00H
KEY31: SJMP MAIN1
NOP
KEY4: MOV TH0,#0DCH ;Exit the time calibration state
MOV TL0,#00H
SETB TR0
SJMP MAIN1
; ********** Display subroutine **************
ORG 0200H
DISPLY: MOV A,TSBIN ; Transfer the second counter into A
MOV B,#0AH
DIV AB ; Count the second value ÷ 10 (the result is stored in A for tens and in B for ones)
SWAP A
ORL A,#07H ; Display the tens digit of the second
MOV P1,A
MOV A,#DPS1
ANL A,OUT
MOV P3,A
ACALL DSP DEL ; Delay (when displaying) 0.5ms
MOV C,P3.7 ; Read the status of S4 key
MOV A,#HIDE
ANL A,OUT
MOV P3,A
JC NP1
MOV KEYCODE,#08H ; S4 is pressed, keyboard value = 8
NP1: MOV P1,#0F7H ; Blanking
NOP
NOP
NOP
MOV A,B ; Display the ones digit of the second
SWAP A
ORL A,#07H
MOV P1,A
MOV A,#DPS0
ANL A,OUT
MOV P3,A
ACALL DSPDEL
MOV A,#HIDE
ANL A,OUT
MOV P3,A
NOP
NOP
NOP
MOV A,TMBIN ;
MOV B,#0AH
DIV AB ;
SWAP A ;Display the tens digit of
minutesORL A,#07H
MOV P1,A
MOV A,#DPM1
ANL A,OUT
MOV P3,A
ACALL DSPDEL
MOV C,P3.7 ;Check if S2 is pressedMOV
A,#HIDE
ANL A,OUT
MOV P3,A
JC NP2
MOV KEYCODE,#04H ;S2 is pressed, keyboard value=4
NOP
NP2: MOV P1,#0F7H ;Hide
NOP
NOP
MOV A,B
SWAP A ;Display the ones digit of
minutesORL A,#07H
MOV P1,A
;MOV C,KEYPRE
;MOV P1.3,C
SETB P1.3
MOV A,#DPM0
ANL A,OUT
MOV P3,A
ACALL DSPDEL
MOV C,P3.7 ;Judge whether S3 is pressed
MOV A,#HIDE
ANL A,OUT
MOV P3,A
JC NP3
MOV KEYCODE,#06H ;If S3 is pressed, the keyboard value = 6
NOP
NP3: MOV P1,#0F7H ;Hide
NOP
NOP
MOV A,THBIN ;
MOV B,#0AH
DIV AB ;
SWAP A ;Tens digit when displayed
JNZ DISPLY1
MOV A,#0F7H
DISPLY1:ORL A,#07H
MOV P1,A
MOV A,#DPH1
ANL A,OUT
MOV P3,A
ACALL DSP DEL
MOV A,#HIDE
ANL A,OUT
MOV P3,A
NOP
MOV A,B
SWAP A ;Ones digit when displayed
ORL A,#07H
ANL A,OUT
MOV P1,A
SETB P1.3
MOV A,#DPH0
ANL A,OUT
MOV P3,A
ACALL DSPDEL
MOV C,P3.7 ;Judge whether S1 is pressed
MOV A,#HIDE
ANL A,OUT
MOV P3,A
JC NP4
MOV KEYCODE,#02H ;S1 is pressed, keyboard value = 2
NOP
NP4: MOV P1,#0F7H ;Hide
NOP
NOP
NOP
RET
;********** Delay subroutine **************8
DSPDEL: MOV R7,#0FFH ; Delay program 0.5ms
DJNZ R7,$
RET
END
Previous article:How to display 8 digits in decimal on a seven segment display?
Next article:DS18B20 assembly program and C program
Recommended ReadingLatest update time:2024-11-16 14:29
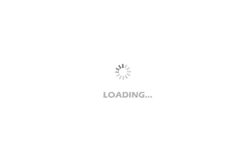
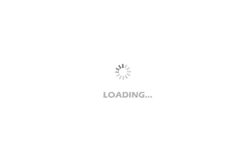
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- CH549EVT Development Board Test——Evaluation Summary
- Familiar Development Tools Support SoC FPGAs
- ADI Analog Dialogue magazine, if you are interested, come and get it~
- Notes and lessons about IAR installation
- [Open Source] Bluetooth module BT401 full set of information based on Bluetooth headset chip development ultra-low cost
- EEWORLD University Hall----Live Replay: ADI's Vital Signs Monitoring Solutions in Wearable Products
- Arrow Electronics' award-winning live broadcast starts at 10:00 this morning: Intel FPGA Deep Learning Acceleration Technology
- stm32f030R8 Chinese reference material
- Recruitment: Executive Vice President of Automotive Electronics (Executive Vice President of New Energy)
- ATmega4809 Curiosity Nano Review + Unboxing and Powering on