Finally, we are talking about pointers. Pointers are the essence of C language. Without pointers, many low-level operations of C language will not be completed. It is also because of the existence of pointers that C language does not look so advanced, because the object of pointer operation is the memory address. If you want to operate pointers skillfully, you must consider hardware-related things such as memory. Of course, you don’t need to know too much. However, the data structure level still needs to be passed. I don’t know much about data structures, so I won’t say much. The relationship between arrays and pointers is so complicated that I have to write this note according to the book.
1. Arrays are not equal to pointers
In C language, array operations are performed in the same way as pointers. However, it is important to remember that arrays are not equal to pointers. For a one-dimensional array a[] and a pointer p=a pointing to an array, the biggest difference between them is that the array method uses the array name a (which is also the first address of the array) to directly access and operate the array, while the pointer method uses the pointer name p to indirectly access and operate the array. In most cases, their operation results are the same, but there are exceptions.
If we define a pointer p outside the file, int *p=a (a is an integer array). When using p in the file, we need to use extern to declare it, indicating that p is an external variable and is defined externally. If we declare it as a pointer extern int * p and then use it, there will be no problem. But if we declare it as an array extern int p[], problems will arise. The compilation system will use array methods to operate on pointers. In other words, when we want to get the value of *p, that is, *a, a[0], because the system treats p as the first address of the array, *p cannot get a[0], but the address value stored in p, that is, the address of a[0]. In this case, the extern declaration must match the definition.
The most fundamental difference between the direct access mode of arrays and the indirect access mode of pointers is that arrays are not equal to pointers. Arrays are usually used to store a fixed number of elements of the same data type. The memory occupied by arrays is implicitly allocated and deleted. Arrays store data, and each element in the array has a unique and clear variable name to identify the data. Using arrays, data can be directly accessed, that is, a[i] simply obtains data with a+i as the address. Pointers are usually used in dynamic data structures. Pointer variables store the address of data (including the address of variables and non-anonymous data). Using pointers to access data adopts an indirect access mode, that is, first obtain the content of the pointer, use it as the address, and then extract data from this address. If the pointer has a subscript, p[i] first gets the content of pointer p, then adds the content of pointer p plus i as the address to extract data. Pointers can point to anonymous data, so you must learn to use pointers to operate anonymous memory. The functions related to memory space in C language are mal LOC (), free().
When defining a pointer, the compiler does not allocate memory space for the object pointed to by the pointer, but only allocates space for the pointer itself, unless a character pointer (which must point to a character type) is defined and initialized with a string constant. In fact, even in this case, it can be regarded as the compiler allocating memory space for this string constant, and then allocating space for the character pointer itself, and making the character pointer point to the first address of the string constant. In ANSI C, the string constant created by the initialized pointer is defined as read-only (? I tried this with turbo C, and it seems that it can be modified). Arrays can also be initialized with string constants, but unlike pointers, arrays initialized with string constants can be modified. The reason is very simple. The array initialized with a string constant is originally a character array, and each character has a certain variable name corresponding to it, so of course a single character can be modified. The string constant created by the initialized pointer is actually anonymous data. If you assign the character pointer pointing to the string constant to another address, this string constant will obviously never be found again.
It can be seen that arrays and pointers are different when processed by the compiler, and their representations at runtime are also different, and different codes may be generated. For the compiler, an array is an address, and a pointer is the address of an address. Therefore, when declaring an external array or defining an array (because the definition of an array must allocate memory space), a pointer cannot be used to replace an array.
Also, a reference to an array cannot be replaced by a pointer to the first element of the array in the following cases:
1. The array name is used as the operand of sizeof(), because the size of the entire array is needed, not the size of the first element pointed to by the pointer;
2. Use the & operator to get the address of the array. The main purpose of the & operator is to implement address-by-address call. The pointer itself is the address, so using & on the pointer is meaningless.
3. The array is initialized by a string constant. This has been mentioned above, so I won't say more. The array initialization by string constant must be done in one go, and cannot be divided into two statements. In other words, string constants can only be used to declare and initialize arrays, and string constants cannot be used to assign values to arrays. This is because in C language, an array can only be initialized when it is declared (there is no reason for this, perhaps because the array name is an immutable left value), and you cannot assign values to the array name, but only to the array elements one by one.
4. The array name is an unmodifiable left value. Its value is the address of the first element of the array and cannot be changed.
2. When are arrays and pointers the same
Most of the time, arrays and pointers are interchangeable.
1. Except for some special cases, the array name in the expression (the array is in use, not in the declaration) is a pointer
Suppose we declare: int a[10], *p, i=2;
You can access a[i] by:
a[i];
*(a+i);
p=a ; p[i] ;
p=a ; *(p+i) ;
p=a+i ; *p ;
In fact, array references such as a[i] are always rewritten by the compiler as *(a+i) at compile time. So, like addition, the operands of the subscript operator can be interchanged (a[i] and i[a] are both correct and equivalent).
The compiler automatically adjusts the step size of the subscript value to the size of the array element. Before performing the addition operation on the starting address, the compiler will be responsible for calculating the step size of each increase. This is why pointers always have type restrictions and each pointer can only point to one type.
2. Array subscript is always the same as the pointer offset
The array subscript is always the same as the pointer offset, so the programmer can use the pointer to access the array, thus bypassing the subscript operator. In this case, the array subscript range check cannot detect all accesses to the array. Therefore, C language does not perform subscript range check. But when we write programs, we must be careful not to cross the boundary.
Pointers are not faster than arrays when dealing with arrays. The fundamental reason why C language rewrites array subscripts as pointer offsets is that pointers and offsets are the basic models used by the underlying hardware.
3. Array names as function parameters are equivalent to pointers
Equating arrays and pointers as formal parameters is done for efficiency reasons. In the declaration of a function parameter, the array name is interpreted by the compiler as a pointer to the first element of the array. The compiler only passes the address of the array to the function, not a copy of the entire array. Implicit conversion means that the following three function definition forms are identical:
fun(int *p){...}
fun(int p[]) {...}
fun(int p[10]) {...}
It is legal to use an array or a pointer to the first element of an array in a function declaration or call.
Note the meaning of the first element here. This first element can be a numeric variable, a character variable, an array, a structure, or a pointer.
Conclusion
An array is not equal to a pointer, but an array can be equivalent to a pointer; a pointer is always a pointer, and a pointer can never be rewritten as an array. You can use subscripts to access pointers, usually when the pointer is a function parameter, usually when the pointer points to an array element.
When declaring and defining externally, arrays cannot be equated with pointers. Except when arrays are used as function parameters, the definition and declaration must match.
Arrays are basically interchangeable with pointers when used. a[i] is always interpreted by the compiler as *(a+i).
When used as function parameters, arrays can be interchanged with pointers. The compiler always modifies the array of function parameters into a pointer to the first element of the array. In fact, what is obtained inside the function is a pointer.
C language does not actually have multidimensional arrays. Only array elements are arrays of arrays. When a multidimensional array is used as a function parameter, the pointer type passed is a pointer to the array.
Previous article:Application of Operators in C51 Programming
Next article:The Relationship between Arrays and Pointers in C51 Programming
Recommended ReadingLatest update time:2024-11-16 14:44
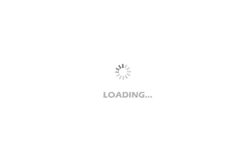
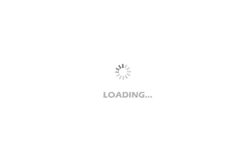
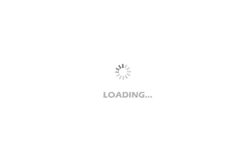
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- I want to know the specific communication process between the external 24C02 EEPROM and the microcontroller. Which forum friend can tell me? Thank you.
- Failed to run Verilog with Cypress PSoC
- The problem of overcurrent capability of two MOS tubes when turned on
- TI Power
- New support for STM32WB55 in MicrPython
- Useful Information|Selected from TI CC3200-LAUNCHXL Evaluation Report
- 100k bridge
- [Zero-knowledge ESP8266 tutorial] Quick Start 6- Make your creations sound
- Remove a chip from a DDR3 1600Mhz 4G memory stick and then put it back together
- 【RT-Thread Reading Notes】7. RT-Thread Study Chapters 8-12 Reading Notes