AVR analog comparator example - AVR analog comparator module can be used to compare the voltage between AIN0 (mega16 PB2 second function) and AIN1 (PB3).
Program operation flow: Initialization >> Open interrupt >> Interrupt service program judgment, the comparison result will be synchronized to
the fifth bit ACO of the analog comparator control and status register - ACSR, and the comparison result can be obtained by detecting the value of ACO. AIN0
// ICC-AVR
// Target : M16
// Crystal: 7.3728MHz
// AVR Analog Comparator Usage Example
#include
#include
#include "delay.h"
//Pin Definition
#define LED0 0 //PB0
#define AIN_P 2 //PB2(AIN0)
#define AIN_N 3 //PB3(AIN1)
//Macro Definition
#define LED0_ON() PORTB|= (1<
//Constant Definition
/*
The positive input of the analog comparator is determined by the ACBG bit, =0 selects the AIN0 pin, =1 selects the 1.23V internal bandgap reference source
Analog comparator multiplexed input (not commonly used, because the ADC will not be able to be used)
can select ADC7..0
The ADC multiplexer can be used to replace the negative input of the analog comparator.
Of course, in order to use this function, the ADC must be turned off first.
If the analog comparator multiplexer enable bit (ACME in SFIOR) is set and the ADC is turned off (ADEN in the ADCSRA register is 0),
the pin that replaces the negative input of the analog comparator can be selected through MUX2..0 in the ADMUX register.
If ACME is cleared or ADEN is set, the negative input of the analog comparator is AIN1.
*/
#define AC_ADC0 0x00 //ADC0
#define AC_ADC1 0x01 //ADC1
#define AC_ADC2 0x02 //ADC2
#define AC_ADC3 0x03 //ADC3
#define AC_ADC4 0x04 //ADC4
#define AC_ADC5 0x05 //ADC5
#define AC_ADC6 0x06 //ADC6
#define AC_ADC7 0x07 //ADC7
void port_init(void)
{
PORTA = 0x00;
DDRA = 0x00;
PORTB = ~((1<
DDRC = 0x00;
PORTD = 0x00;
DDRD = 0x00;
}
//Initialization steps, turn off interrupt, change the value of ACSR, configure analog comparator, turn on interrupt.
//Comparator initialize
// trigger on: Output toggle
void comparator_init(void)
{
ACSR = ACSR & 0xF7; //ensure interrupt is off before changing
//The above sentence will make ACIE zero, and interrupts are not allowed
ACSR=(1<
}
#pragma interrupt_handler ana_comp_isr:17
void ana_comp_isr(void)
{
//analog comparator compare event
//Hardware automatically clears the ACI flag
delay_us(10);
if ((ACSR&(1<
LED0_ON(); //If AIN0
LED0_OFF(); //Otherwise LED is off
delay_ms(200); //When the voltage difference is close to 0V, the analog comparator will produce critical jitter, so delay 200ms to make it visible to the naked eye
}
//call this routine to initialize all peripherals
void init_dev IC es(void)
{
//stop errant interrupts until set up
CLI(); //d ISA ble all interrupts
port_init();
comparator_init();
MCUCR = 0x00;
GICR = 0x00;
TIMSK = 0x00; //timer interrupt sources
SEI(); //re-enable interrupts
//all peripherals are now initialized
}
void main(void)
{
init_devices();
while(1)
;
}
--------------------------------------------------------------------------------
/*
Program test:
VCC VCC
| |
| | | |
AIN0--|W| |W|--AIN1 PB0---R---LED--
| | | | |
| | |
GND GND GND
Two potentiometers, one end connected to VCC, the other end to ground, constitute a potentiometer voltage divider circuit
. AIN0 and AIN1 are connected to the center tap of the potentiometer respectively.
PBO output series resistor drives LED, high level is valid.
Then rotate the potentiometer respectively to increase or decrease the tap voltage, and you will find that the output of PB0 (LED0) will change according to the voltage relationship of AIN0/AIN1
Due to the influence of power supply ripple, IO current and external interference, when the voltage difference is close to 0V, the analog comparator will produce critical jitter. Connecting a small capacitor to the ground of AIN0/AIN1 can improve this situation.
When there is only one potentiometer, you can change it.
1 You can enable ACBG and use the 1.23V internal bandgap reference source instead of AIN0 as the positive input of the analog comparator.
ACSR=(1<
ADCSRA=(1<
Analog Comparator Control and Status Register - ACSR
Bit 7 - ACD: Analog Comparator Disable The
analog comparator is already working by default when it powers up, which is different from other modules
. When ACD is set, the power supply of the analog comparator is cut off. You can set this bit at any time to turn off the analog comparator.
This can reduce the power consumption of the device in working mode and idle mode.
When changing the ACD bit, you must clear the ACIE bit in the ACSR register to disable the analog comparator interrupt. Otherwise, an interrupt may be generated when ACD changes.
Bit 6 – ACBG: Bandgap Reference Source Selection for Analog Comparator
When ACBG is set, the positive input of the analog comparator is replaced by the 1.23V bandgap reference source. Otherwise, AIN0 is connected to the positive input of the analog comparator.
Bit 5 – ACO: Analog Comparator Output
The output of the analog comparator is directly connected to ACO after synchronization. The synchronization mechanism introduces a delay of 1-2 clock cycles
. Bit 4 – ACI: Analog Comparator Interrupt Flag
When the output event of the comparator triggers the interrupt mode defined by ACIS1 and ACIS0, ACI is set.
If the global interrupt flag I in the ACIE and SREG registers is also set, the analog comparator interrupt service routine is executed and ACI is cleared by hardware.
ACI can also be cleared by writing "1".
Bit 3 – ACIE: Analog Comparator Interrupt Enable
When the ACIE bit is set to "1" and the global interrupt flag I in the status register is also set, the analog comparator interrupt is activated.
Otherwise the interrupt is disabled.
Bit2 – ACIC: Analog Comparator Input Capture Enable
This function is used to detect some weak trigger signal sources, saving an external op amp.
When ACIC is set, it allows the analog comparator to trigger the input capture function of the Timer/C1.
At this time, the output of the comparator is directly connected to the front-end logic of the input capture, so that the comparator can take advantage of the noise suppressor and trigger edge selection function of the Timer/C1 input capture interrupt logic.
In order for the comparator to trigger the input capture interrupt of the Timer/C1, TICIE1 of the Timer Interrupt Mask Register TIMSK must be set.
When ACIC is "0", there is no connection between the analog comparator and the input capture function.
Bits 1, 0 – ACIS1, ACIS0: Analog Comparator Interrupt Mode Select
These two bits determine the event that triggers the analog comparator interrupt.
ACIS1 ACIS0 Interrupt mode
0 0 Changes in comparator output can trigger an interrupt
0 1 Reserved
1 0 The falling edge of the comparator output generates an interrupt
1 1 The rising edge of the comparator output generates an interrupt
When changing ACIS1/ACIS0, the interrupt enable bit of the ACSR register must be cleared to disable the analog comparator interrupt. Otherwise, an interrupt may be generated when changing these two bits.
*/
Previous article:ATMEGA8 single chip frequency meter program and circuit diagram
Next article:AVR Thermometer DS18B20
Recommended ReadingLatest update time:2024-11-17 03:55
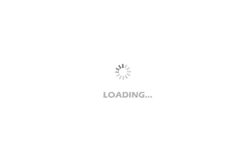
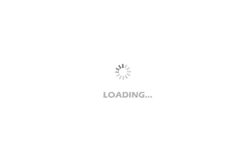
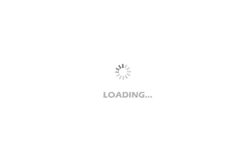
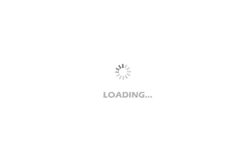
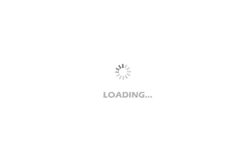
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Metronom Real-Time Operating System RTOS for AVR microcontrollers
-
Learn C language for AVR microcontrollers easily (with video tutorial) (Yan Yu, Li Jia, Qin Wenhai)
-
ATmega16 MCU C language programming classic example (Chen Zhongping)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- [RISC-V MCU CH32V103 Review] + ADC analog watchdog
- I2C Range Extension Reference Design: I2C to CAN
- Application of Aigtek power amplifier in performance study of electrokinetic migration-capture-release of Pb-contaminated municipal sludge
- Recently, the project department has taken on a new project, which requires the performance indicators of IC to meet industrial requirements. Can someone recommend...
- I would like to ask for advice from all the experts. How can a fully enclosed metal shell increase the signal strength of NB?
- About the problem of small current driving large current load
- The power supply cannot be completely turned off. There is a leakage of 4~5MA. I hope an expert can give me some advice.
- The working principle and function of oscilloscope triggering
- Gigabit Network Contactless Connector-SK202 Evaluation Report 2: Research on ST-60
- EEWORLD University Hall----Live Replay: When intelligence meets industry, how can technology be implemented?