According to the schematic diagram of the TQ2440 development board, the buttons are connected to the EINT0, 1, 2, and 4 pins of the S3C2440 that can be used for external interrupts, and GPFCON can set the functions of these pins.
The EXTINT0 register can set the conditions for the interrupts of these four pins. It should be noted that EINT0~2 have their own interrupt vectors, while EINT4~7 share an interrupt vector.
Therefore, when using EINT4, you must also open the corresponding interrupt enable bit in the EINTMASK register, and after entering the EINT4 interrupt, you must also clear the interrupt bit of the EINTPEND register.
The following is the flowchart of the interrupt handling function. The implementation methods of these four buttons are the same:
Here is the procedure:
/*******************************
s3c2440 external interrupt button experiment
LHG
********************************/
#include "2440addr.h"
#include "eint_button.h"
#include "uart.h"
#define U32 unsigned int
int button_result;//Record which button is pressed
int get_button_result(void)//Get the button
{
int b;
switch(button_result)//Switch out the correct button number
{
case 0:b=4;break;
case 1:b=1;break;
case 2:b=3;break;
case 4:b=2;break;
default:b=-1;break;
}
button_result=-1;
return b;//Return button
}
void button_delay(int a,int b)
{
int c ;
if (a==0 || b==0)
return;
while (a--)
for (c=0;c ;
}
void share_eint(int b)//Because the interrupt processing flow is the same, we share a processing function, int b is to select which external interrupt source is
{
if ( (rEXTINT0>>(4*b)&7)==0x1 )//Judge whether it is a high-level interrupt
{
button_result=b;//Notify that a key is pressed
rEXTINT0 &=~( (7<<16)|(7<<8)|(7<<4)|(7) );//Set the four buttons to low level to enter the interrupt
rINTMSK &= ~((U32)0x17);//Enable interrupt eint 0,1,2
rEINTMASK &= ~((U32)0x10);//Enable interrupt eint 4
}
else
{
button_delay(100,100);//Delay debounce
rGPFCON &=~( (3<<8)|0x3f );//Set the pin to inputif
( !(rGPFDAT&(1< {//It is at a low level, confirm that a key is pressedif
(b<4)//If 0,1,2 interrupt
{
rINTMSK |= ((U32)0x17)&(~(1< rEINTMASK |= (U32)0x10;//Shield interrupt eint 4
rEXTINT0 |=(1<<4*b); //Set to high level interrupt
}
else//If it is 4 interrupts
{
//Uart_Printf("int 4/r/n");
rINTMSK |= ((U32)0x17)&(~(1< rEINTMASK &= ~((U32)0x10);//Enable interrupt eint 4
rEXTINT0 |=(1<<4*b); //Set to high level interrupt
}
}
else
{
//It is jitter, do nothing
}
}
rGPFCON &=~( (3<<8)|0x3f );//Set GPIO to external interrupt mode
rGPFCON |= ( (2<<8)|0x2a );
rSRCPND |= 0x17;//clear interrupt flag
rINTPND |= 0x17;
rEINTPEND |= 0x10;
}
void __irq eint0ISR(void) //eint0 interrupt function
{
share_eint(0);
}
void __irq eint1ISR(void) //eint1 interrupt function
{
share_eint(1);
}
void __irq eint2ISR(void) //eint2 interrupt function
{
share_eint(2);
}
void __irq eint4_7ISR(void) //eint4 interrupt function
{
if (rEINTPEND&0x10)
share_eint(4);
rSRCPND |= 0x17;//clear interrupt flag
rINTPND |= 0x17;
rEINTPEND |= 0x10;
}
void init_eint_button(void)//initialization
{
button_result=-1;//No key pressed
//Set interrupt entry
pISR_EINT0=(U32)eint0ISR;
pISR_EINT1=(U32)eint1ISR;
pISR_EINT2=(U32)eint2ISR;
pISR_EINT4_7=(U32)eint4_7ISR;//eint4~7 share an interrupt
rINTMOD &= ~((U32)0x17);//Use IRQ mode
rGPFUP |= 0x17;//No pull-up resistor
rGPFCON &=~( (3<<8)|0x3f );//Set GPIO
rGPFCON |= ( (2<<8)|0x2a );
rEXTINT0 &=~( (7<<16)|(7<<8)|(7<<4)|(7) );//All four buttons enter the interrupt at low level
//Open interrupt
rSRCPND |= 0x17;//Clear interrupt flag
rINTPND |= 0x17;
rEINTPEND |= 0x10;
rINTMSK &= ~((U32)0x17);//Enable interrupt eint 0,1,2
rEINTMASK &= ~((U32)0x10);//Enable interrupt eint 4
// Uart_Printf("button init/n/r");
}
Achievement results:
Previous article:S3C2440 IIC read and write AT24C02A
Next article:Use of S3C2440 timer
Recommended ReadingLatest update time:2024-11-16 14:28
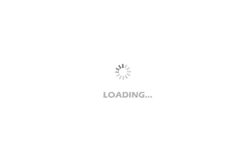
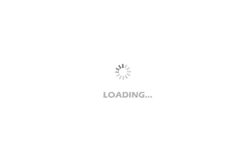
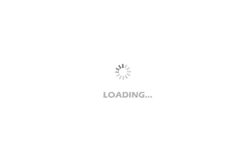
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- quartusii official tutorial
- [Repost] Learn these eight circuit design skills and your professional quality will be greatly improved
- [STM32WB55 Review] +ST's Attack
- MSP430 ADC acquisition filter
- Soil moisture measurement circuit diagram
- I tested the TLC2272 chip and found that the rail-to-rail output is really good.
- [CC1352P Review] BLE Program Framework
- EEWORLD University ---- Robotics
- Do you engineers use Diodes (US-Taiwan) discrete components?
- EEWORLD University - In-depth study of light load high efficiency and low noise power supply reference design for wearable devices and the Internet of Things (TIDA-01566)