The infrared remote control I use uses the NEC protocol, which uses PWM to modulate the information sent.
The NEC protocol has the following characteristics:
1. 8-bit address and 8-bit instruction length;
2. Address and command are transmitted twice (to ensure reliability)
3. PWM pulse position modulation, using the duty cycle of the transmitted infrared carrier to represent "0" and "1";
4. The carrier frequency is 38Khz;
5. The bit time is 1.125ms or 2.25ms;
The bit definition of NEC code: one pulse corresponds to a continuous carrier of 560us, a logic 1 transmission takes 2.25ms (560us pulse + 1680us low level), and a logic 0 transmission takes 1.125ms (560us pulse + 560us low level). The remote control receiver is at a low level when it receives a pulse, and at a high level when there is no pulse. In this way, the signal we receive at the receiving head is: logic 1 should be 560us low + 1680us high, and logic 0 should be 560us low + 560us high.
The data format of NEC remote control command is: synchronization terminal, address code, address inverse code, control code, control inverse code. The synchronization code consists of a 9ms low level and a 4.5ms high level. The address code, address inverse code, control code, and control inverse code are all 8-bit data formats. They are sent in the order of low bit first and high bit last. The inverse code is used to increase the reliability of transmission (can be used for verification).
The connection between the infrared receiving head and stm32 is shown in the figure above. Since it is PWM modulation, it is easy to think of the input capture and output comparison functions of the general timer of stm32. Here, since stm32 receives the information sent by the infrared remote control, the IO port connected to the infrared receiving head should be set to input mode. Because the input always maintains a high level in the idle state, it should be configured as a pull-up input.
RCC->APB2ENR|=1<<3;
GPIOB->CRH&=0xffffff0f; //00: Analog input mode //00: Input mode (state after reset)
GPIOB->CRH|=0x00000080; //10: Pull-up/pull-down input mode
GPIOB->ODR|=1<<9; //ODRy[15:0]: Port output data (y = 0…15)
//These bits are readable and writable and can only be operated in word (16-bit) form.
Because PB.9 is the fourth channel of the general timer, the timer needs to be configured. Um... I haven't used the timer for a long time, and I almost forgot about it. I have to pick it up again.
void time4_init()
{
RCC->APB1ENR|=1<<2;//Turn on the clock of timer 4////?
TIM4->SR=0; //Actually the reset value is 0, so it is unnecessary ////Status register
TIM4->DIER|=1<<4; //Enable capture interrupt of timer four ////1: Enable capture/compare 4 interrupt.
TIM4->PSC=71; //Counting frequency is set to 1M CNT increases by one, the time is 1us
////The clock frequency of the counter CK_CNT is equal to fCK_PSC/(PSC[15:0]+1). //72M/72=1M
TIM4->ARR=10000; //The counter overflows every 10ms
////ARR contains the value that will be transferred to the actual auto-reload register. ////10000*1us=10ms
TIM4->CCMR2|=1<<8; //CC4 channel is configured as input, IC4 is mapped on TI4;
////01: CC4 channel is configured as input, IC4 is mapped on TI4;
TIM4->CCER&=~(1<<13); //Channel 4 is configured as rising edge capture
////0: Non-inverting: Capture occurs on the rising edge of IC1; when used as an external trigger, IC1 is not inverted.
TIM4->CCMR2|=3<<12; //Filtering
////Bit 15:12 IC4F[3:0]: Input capture 4 filter
TIM4->CCER|=1<<12; //Channel 4 capture enable
////1: Capture enable.
TIM4->CR1|=1<<0; //Timer 4 count enable
///1: Enable the counter.
}
Because the first data of the signal received by the infrared receiving head must be a synchronization code, the low level is maintained for 9ms, then a jump occurs, and the high level is maintained for 4.5ms. We judge whether the received data is logic 0 or logic 1, or a synchronization code, based on the duration of the high level. Therefore, we should pay attention to the high level holding time. Therefore, when timer 4 is initialized, it should be configured as rising edge capture. OK, the timer is also set. Next, it is time to set the interrupt processing function of timer 4.
Yes, you need to turn on the TIM4 interrupt in NVIC first
void nvic_init()
{
NVIC->ISER[0]|=1<<30;//The interrupt number of TIM4 is 30/////?
}
void TIM4_IRQHandler(void)
{
if(TIM4->SR&0X10)//Judge whether the interrupt source is caused by channel 4 capture
////This bit is set to '1' by hardware when a capture event occurs, and is cleared to '0' by software or by reading TIMx_CCR1.
////1: The counter value has been captured (copied) to TIMx_CCR1 (an edge with the same polarity as selected was detected on IC1).
{
led1=~led1; //Signal indicator light, which can intuitively determine whether timer 4 generates a capture interrupt
if(CS==1) // rising edge capture occurs. Defined in the header file #define CS PBin(9)
{
TIM4->CNT=0; //Counter clear
////Counter value
TIM4->CCER|=1<<13; //Change the capture interrupt trigger mode to falling edge
////1: Inversion: Capture occurs on the falling edge of IC1; when used as an external trigger, IC1 inverts
TIM4->SR=0; Status flag cleared ////status register
dcb=1; //A data bit must first have a rising edge interrupt and then a falling edge interrupt to record the high level duration
//So for one data bit, two interrupts must appear in pairs////?
}
if(CS==0) //Falling edge capture occurs
{
if(dcb==1)
{
dcb=0;//Close the door after entering, no explanation
TIM4->CCER&=~(1<<13); //Change to rising edge capture
////0: Non-inverting: Capture occurs on the rising edge of IC1; when used as an external trigger, IC1 does not invert
temp=TIM4->CCR4; //CNT count value when falling edge interrupt occurs
////If CC4 channel is configured as input: CCR4 contains the counter value transmitted by the last Input Capture 4 event (IC4).
if(3000
{
OK1=1;
}
if(1000
{
data=data<<1;
data|=1<<0;
ray_flag++;
}
if(300
{
data=data<<1;
data&=~(0<<0);
ray_flag++;
}
if(ray_flag>=32) //NEC protocol sends 32 bits of data at a time
OK2=1;
TIM4->SR=0;
}
}
}
}
The interrupt service routine is configured, and the next step is the middle program
int main()
{
Stm32_Clock_Init(9);
delay_init(72);
gpio_init();
nvic_init();
time4_init();
usart1_init();
while(1)
{
if(OK1==1&&OK2==1)
{
usart1_senddata(temp);
OK1=0;
OK2=0;
ray_flag=0;
}
}
The serial port is used to print data, so the serial port configuration program will not be written.
}
Previous article:Detailed explanation of general timer based on stm32
Next article:STM32 serial port sends data (sends a byte and an array)
Recommended ReadingLatest update time:2024-11-16 23:47
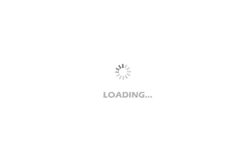
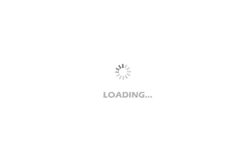
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- A must-know for RF engineers: How to design directional coupler circuits
- DSP Q format operation
- MSP430 Development Considerations and Requirements
- Why Ultra Wideband?
- NUCLEO_G431RB review——by elike
- Raspberry Pi indoor environment monitor Pimoroni Enviro
- Talking about software architecture from a historical perspective (Part 1)
- The bus card chip is broken, please tell me how to fix it
- Clock cycle, machine cycle, instruction cycle
- A small error in the X-NUCLEO-IKS01A3 Quick Start Guide