The timer function of STM32 is very powerful, including TIME1 and TIME8 advanced timers, TIME2~TIME5 general timers, TIME6 and
TIME7 basic timers.
The general TIMx (TIM2, TIM3, TIM4 and TIM5) timer functions of STM32 include:
1) 16-bit up, down, up/down auto-load counter (TIMx_CNT).
2) 16-bit programmable (can be modified in real time) prescaler (TIMx_PSC), the counter clock frequency division factor is
any value between 1 and 65535.
3) 4 independent channels (TIMx_CH1~4), these channels can be used as:
A. Input capture
B. Output compare
C. PWM generation (edge or center alignment mode)
D. Single pulse mode output
4) External signal (TIMx_ETR) can be used to control the synchronization circuit of timer and timer interconnection (one timer can be used to control another
timer).
5) Interrupt/DMA is generated when the following events occur:
A. Update: counter overflow/underflow, counter initialization (by software or internal/external trigger)
B. Trigger event (counter starts, stops, initializes or counts by internal/external trigger)
C. Input capture
D. Output comparison
E. Support for incremental (quadrature) encoder and Hall sensor circuits for positioning
F. Trigger input as external clock or cycle-by-cycle current management
Next, the time.c file needs to be written:
#include "timer.h"
#include "led.h"
//General timer 3 interrupt initialization
//The clock here is selected to be twice that of APB1, and APB1 is 36M
//arr: automatically reload value.
//psc: clock pre-division number
//Timer 3 is used here!
void TIM3_Int_Init(u16 arr,u16 psc)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM3, ENABLE); //①Clock TIM3 enable
//Timer TIM3 initialization
TIM_TimeBaseStructure.TIM_Period = arr; //Set the value of the automatic reload register period
TIM_TimeBaseStructure.TIM_Prescaler =psc; //Set the prescaler value of the clock frequency divisor
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1; //Set clock division
199
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up; //TIM counts up
TIM_TimeBaseInit(TIM3, &TIM_TimeBaseStructure); //②Initialize TIM3
TIM_ITConfig(TIM3,TIM_IT_Update,ENABLE); //③Enable update interrupt
//Interrupt priority NVIC setting
NVIC_InitStructure.NVIC_IRQChannel = TIM3_IRQn; //TIM3 interrupt
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; //Preemption priority level 0
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 3; //From priority level 3
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //IRQ channel is enabled
NVIC_Init(&NVIC_InitStructure); //④Initialize NVIC registers
TIM_Cmd(TIM3, ENABLE); //⑤Enable TIM3
}
//Timer 3 interrupt service routine⑥
void TIM3_IRQHandler(void) //TIM3 interrupt
{
if (TIM_GetITStatus(TIM3, TIM_IT_Update) != RESET) // Check whether TIM3 update interrupt occurs
{
TIM_ClearITPendingBit(TIM3, TIM_IT_Update ); //Clear TIM3 update interrupt flag
LED1=!LED1;
}
}
I would like to emphasize here that when I was doing the experiment, the program did not report an error, but after burning it, DS0 and DS1 were always off, so I compared the original program to find the error one by one, and finally found that the interrupt service function was written incorrectly, IRQHandler was written as IRQHanlder. At first I was very puzzled, isn’t the interrupt service function name defined by myself, and there is a problem if I write a wrong letter? In fact, it is not the case. I misunderstood how the interrupt function works from the beginning. . .
The interrupt must be initialized first, and then enabled, which is this sentence:
TIM_ITConfig(TIM3,TIM_IT_Update,ENABLE ); // Enable update interrupt
After enabling, it will automatically find and enter the interrupt service function, which means that the interrupt service function has been defined internally. After I wrote it wrong, the program has entered the interrupt service function, but it is stuck inside and can't get out, causing DS1 and DS0 in the main function to not light up... (Comment out the interrupt enable, the interrupt service function of my mistake remains unchanged, and DS0 in the main function lights up after burning)
Next is the main function:
int main(void)
{
delay_init(); //delay function initialization
NVIC_Configuration(); //Set NVIC interrupt group 2: 2-bit preemption priority, 2-bit response priority
200
uart_init(9600); //Serial port initialization baud rate is 9600
LED_Init(); //LED port initialization
TIM3_Int_Init(4999,7199); //10Khz counting frequency, 500ms when counting to 5000
while(1)
{
LED0=!LED0;
delay_ms(200);
}
}
The final experimental result is that DS0 and DS1 flash alternately, but DS0 is twice as fast as DS1.
Previous article:STM32 drives ILI9341 controller to control TFTLCD display
Next article:STM32 study notes timer configuration
Recommended ReadingLatest update time:2024-11-16 13:04
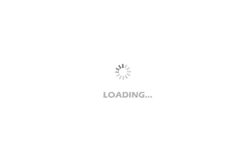
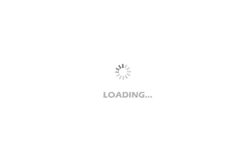
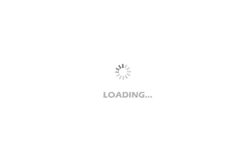
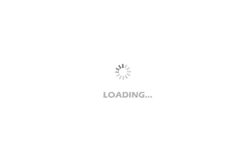
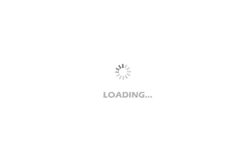
- Popular Resources
- Popular amplifiers
-
The STM32 MCU drives the BMP280 absolute pressure sensor program and has been debugged
-
DigiKey \"Smart Manufacturing, Non-stop Happiness\" Creative Competition - Small Weather Station - STM32F103 Sensor Driver Code
-
LwIP application development practical guide: based on STM32
-
FreeRTOS kernel implementation and application development practical guide: based on STM32
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Undergraduate students from the University of Science and Technology of China designed a processor chip in 9 months! Decoding the story behind it
- The Raspberry Pi discount quota is full. Check out other e-selected products to participate in the event
- Go your own way and let the SI engineer decide
- protues arm2124 simulation snake program
- BlueNRG-1 chip
- Bluetooth 5 New Features and Applications
- GD32F130 will immediately enter the serial port interrupt after the serial port idle interrupt is turned on
- LIS2MDL array PCB board for magnetic nail navigation AGV car
- Filter Design——FilterSolutions
- Android driver learning 1-driver development process (Android.mk)