//File touchbutton.h #ifndef _TOUCHBUTTON_H_ #define _TOUCHBUTTON_H_ //=========Macro definition============================================ #define DEF_WATCH_TKREFER //Watch reference - for testing //=========================================================== #define V_REFER 1 //AD value exceeds this value, update reference value #define V_PRESS 7 //AD value - reference. If it is greater than this value, it is considered that the button is pressed - button sensitivity #define VT_KTc 30 //The number of times to update the reference value //--------button position definition----------- #define K_TKON SETB0 //ON/OFF #define K_TKBL SETB1 //Boiling #define K_TKPU SETB2 //Water pump #define K_TKST SETB3 // Constant temperature setting //#define V_TKQK1 (uint8)5 //Fast addition and subtraction time value typedef struct TCHBUT{ uint8 KTc1; //Update reference value confirmation time uint8 KRefer1 ; //Key 1 reference voltage uint8 KTc2 ; //Update reference value confirmation time uint8 KRefer2 ; //Key 2 reference voltage /* uint8 KTc3 ; //Update reference value confirmation time uint8 KRefer3 ; //Key 2 reference voltage uint8 KTc4 ; //Update reference value confirmation time uint8 KRefer4 ; //Key 2 reference voltage uint8 KTc5 ; //Update reference value confirmation time uint8 KRefer5 ; //Key 2 reference voltage uint8 KTc6 ; //Update reference value confirmation time uint8 KRefer6 ; //Key 2 reference voltage uint8 KTc7 ; //Update reference value confirmation time uint8 KRefer7 ; //Key 2 reference voltage // uint8 KTc8 ; // Update reference value confirmation time // uint8 KRefer8 ; //Key 2 reference voltage */ uint8 KTVal1 ; uint8 KTVal2 ; // uint8 KTVal3 ; // uint8 KTVal4 ; }tTCHBut ; extern xdata tTCHBut tCHBut ; #define KTc1 tCHBut.KTc1 #define KRefer1 tCHBut.KRefer1 #define KTc2 tCHBut.KTc2 #define KRefer2 tCHBut.KRefer2 #define KTc3 tCHBut.KTc3 #define KRefer3 tCHBut.KRefer3 #define KTc4 tCHBut.KTc4 #define KRefer4 tCHBut.KRefer4 #define KTc5 tCHBut.KTc5 #define KRefer5 tCHBut.KRefer5 #define KTc6 tCHBut.KTc6 #define KRefer6 tCHBut.KRefer6 #define KTc7 tCHBut.KTc7 #define KRefer7 tCHBut.KRefer7 //#define KTc8 tCHBut.KTc8 //#define KRefer8 tCHBut.KRefer8 #define KTVal1 tCHBut.KTVal1 #define KTVal2 tCHBut.KTVal2 #define KTVal3 tCHBut.KTVal3 #define KTVal4 tCHBut.KTVal4 //=======Key detection============== typedef struct BUTTON2{ uint8 TKNOW ; //The current status of the keys uint8 TCLST ; // uint8 TKLST1 ; // uint8 TKLST2 ; // uint8 TKLST3 ; uint8 TKSTB ; //button status after debounce uint8 TKSTBL ; //button status after last debounce uint8 TKAVL ; //falling edge valid value uint8 TKAVLP ; //Rising edge valid value uint8 TKeyStatu ; //Read the current value of the key uint8 TKLONG ; //Long key press count // uint8 TTconQK ; // fast adjustment count 111 555 10 10 10 }tButton2 ; extern tButton2 tKey2 ; #define TKNOW tKey2.TKNOW #define TKLST tKey2.TKLST #define TKLST1 tKey2.TKLST1 //#define KLST2 tKey.TKLST2 //#define KLST3 tKey.TKLST3 #define TKSTB tKey2.TKSTB #define TKSTBL tKey2.TKSTBL #define TKAVL tKey2.TKAVL #define TKAVLP tKey2.TKAVLP #define TKeyStatu tKey2.TKeyStatu #define TKLONG tKey2.TKLONG #define TTconQK tKey2.TTconQK #define V_TKLOV1 200 //1S confirms long press #define V_TKLOV2 20//15 //150MS to set the key flag //========================================= typedef struct KFlag2 //Key processing flag definition { uint8 Flg1: 1 ; uint8 Flg2: 1 ; uint8 Flg3: 1 ; uint8 Flg4: 1 ; uint8 Flg5: 1 ; uint8 Flg6: 1 ; uint8 Flg7: 1 ; uint8 Flg8: 1 ; }tKFlg2 ; external tKFlg2 KF2 ; //#define F_TRdKeyOK KF2.Flg1 //Read key is valid #define F_TKLO KF2.Flg2 //Long press is effective //#define F_TKQK1 KF2.Flg4 //#define F_TKQK2 KF2.Flg5 #define F_TKEYOK KF2.Flg6 //=========================================== //================================ extern void ReadTKRefer(uint8 CH_AD,uint8 *a) ; extern void CheckTouch1(void) ; extern void CheckTouch2(void) ; extern void CheckTKey(void) ; #endif //File touchbutton.c #include "global.h" #include "touchbutton.h" //#include "touchbutton.h" /*********************************************** Touch PWM 500K When the button is pressed, the voltage value increases ***********************************************/ tButton2 tKey2 ; tKFlg2 KF2 ; xdata tTCHBut tCHBut ; //#define LKEY_ENB //Long key enable //*************************************** // Function name: ReadTKRefer // Function: Read touch button reference // Input parameters: CH_AD button AD channel // Export parameter: *a corresponds to the AD reference value of the key //*************************************** void ReadTKRefer(uint8 CH_AD,uint8 *a) { uint8 R_Save[3]; uint8 R_Save2[3]; uint16 R_SaveSum = 0 ; uint8 Tcon = 0 ; uint8 Tmp1 ; uint8 i ; for(i=40;i>0;i--) { AdcSwitch(CH_AD); //Read button 1 voltage Tmp1 = ADDH ; RMov3Data_Byte(R_Save,Tmp1) ; Tmp1 = C3MidVal(R_Save); //The maximum number of digits to be processed is 3, and the median value is taken for processing RMov3Data_Byte(R_Save2,Tmp1) ; Tmp1 = C3MidVal(R_Save2); //The maximum number of digits to be processed is 3, and the median value is taken for processing R_SaveSum += Tmp1 ; Tcon++ ; if(Tcon >= (1<<3)) { *a = R_SaveSum>>3 ; Tcon = 0 ; R_SaveSum = 0 ; } } } //*************************************** // Function name: CheckTouch1 // Function: Detect touch buttons // Entry parameters: None //Export parameters: None //*************************************** void CheckTouch1(void) { uint8 a[3]; uint8 Tmp1 ; uint8 TmpAD ; //temporary reference value AdcSwitch(V_ADCH_tch1); //Read button voltage a[0] = ADDH ; _nop_();_nop_();_nop_();_nop_();_nop_(); AdcSwitch(V_ADCH_tch1); //Read button voltage a[1] = ADDH ; _nop_();_nop_();_nop_();_nop_();_nop_(); AdcSwitch(V_ADCH_tch1); //Read button voltage a[2] = ADDH ; //----The following is the middle value of three AD values----- TmpAD = C3MidVal(a) ; #ifdef DEF_WATCH_TKREFER KTVal1 = TmpAD ; //Current key AD value -- used for testing ^^^^^^^^^^^^^^^^^^^^^^ #endif if(!(TKSTB & SETB0)) //This button is in the lifted state at this time --Change the reference value { if(TmpAD < KRefer1) { Tmp1 = KRefer1 - TmpAD ; } else { Tmp1 = TmpAD - KRefer1 ; } if(Tmp1 >= V_REFER) //Update reference threshold value { KTc1 -- ; if(KTc1 == 0) { KTc1 = VT_KTc ; if(KRefer1 == TmpAD) //KRefer1 = TmpAD ; {} else if(KRefer1 < TmpAD) { KRefer1 ++; //Update reference, add or subtract 1 each time } else { KRefer1 -- ; } } } else { KTc1 = VT_KTc ; } } else //if(TKSTB & SETB0) //This button is currently pressed { KTc1 = VT_KTc ; } Tmp1 = KRefer1 + V_PRESS ; if(TmpAD >= Tmp1) // greater than the reference value, it is considered pressed { TKeyStatu |= SETB0 ; } else { TKeyStatu &= ~SETB0 ; } } //*************************************** // Function name: CheckTouch2 // Function: Detect touch buttons // Entry parameters: None //Export parameters: None //*************************************** void CheckTouch2(void) { uint8 a[3]; uint8 Tmp1 ; uint8 TmpAD ; //temporary reference value AdcSwitch(V_ADCH_tch2); //Read button voltage a[0] = ADDH ; _nop_();_nop_();_nop_();_nop_();_nop_(); AdcSwitch(V_ADCH_tch2); //Read button voltage a[1] = ADDH ; _nop_();_nop_();_nop_();_nop_();_nop_(); AdcSwitch(V_ADCH_tch2); //Read button voltage a[2] = ADDH ; //----The following is the middle value of three AD values----- TmpAD = C3MidVal(a) ; #ifdef DEF_WATCH_TKREFER KTVal2 = TmpAD ; //Current key AD value -- used for testing ^^^^^^^^^^^^^^^^^^^^^^ #endif if(!(TKSTB & SETB1)) //This button is in the lifted state at this time --Change the reference value { if(TmpAD < KRefer2) { Tmp1 = KRefer2 - TmpAD ; } else { Tmp1 = TmpAD - KRefer2 ; } if(Tmp1 >= V_REFER) //Update reference threshold value { KTc2 -- ; if(KTc2 == 0) { KTc2 = VT_KTc ; if(KRefer2 == TmpAD) //KRefer1 = TmpAD ; {} else if(KRefer2 < TmpAD) { KRefer2 ++ ; // Update reference, add or subtract 1 each time } else { KRefer2 -- ; } } } else { KTc2 = VT_KTc ; } } else //if(TKSTB & SETB0) //This button is currently pressed { KTc2 = VT_KTc ; } Tmp1 = KRefer2 + V_PRESS ; if(TmpAD >= Tmp1) // greater than the reference value, it is considered pressed { TKeyStatu |= SETB1 ; } else { TKeyStatu &= ~SETB1 ; } } //File maic.c //======Simple Routine============ //************************************* // Function name: Init_TKey // Function: Read the reference value of the touch button // Entry parameters: None //Export parameters: None //*************************************** void Init_TKey(void) // { uint8 i ; for(i=250;i>0;i--) //Wait for about 250MS to let the touch voltage stabilize { Nopt(250) ; Nopt(250) ; Nopt(250) ; Nopt(250) ; Nopt(250) ; } ReadTKRefer(V_ADCH_tch1,&KRefer1) ; ReadTKRefer(V_ADCH_tch2,&KRefer2) ; ReadTKRefer(V_ADCH_tch3,&KRefer3) ; ReadTKRefer(V_ADCH_tch4,&KRefer4) ; } //-------------------- void CmdTK1(void) { if(!(TKAVL & K_TKON)) return ; TKAVL &= ~K_TKON ; //Processing button 1 pressing program } //-------------------- void CmdTK2(void) { if(!(TKAVL & K_TKBL)) return ; TKAVL &= ~K_TKBL ; //Processing button 2 pressing program } //-------------------------------------- void main(void) { Init_TKey() ; //Read the reference value of the touch key after power on while(1) { if(F_20MS) //20MS runs once { F_20MS = 0 ; CheckTouch1(); //Detect key 1 and update reference value CheckTouch2(); //Detect key 2 and update reference value CheckTKey() ; CmdTK1(); //Process key 1 CmdTK2(); //Process key 2 } } }
//The circuit reference is as follows. PAN2 is the AD detection port. Take this figure as an example. When a button is pressed, the voltage of the PAN2 port will increase.
Previous article:Summary of low power consumption design of single chip microcomputer
Next article:How does MCU cooperate with microcontroller for simulation?
Recommended ReadingLatest update time:2024-11-17 02:00
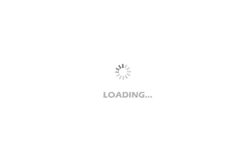
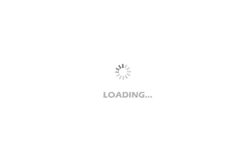
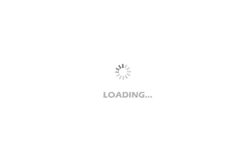
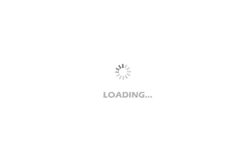
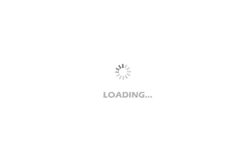
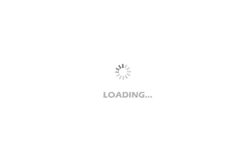
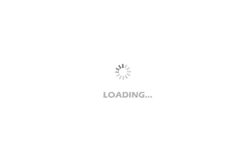
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- TI DBV package silkscreen details
- KiCAD released a new version - 5.1.10
- 2020 National College Student Electronics Contest Test Questions
- High voltage and small size: the latest technology trends of Nichicon's drive film capacitors
- [Chip] Interesting chips, interesting engineer souls
- Serial communication
- TMS320C6678DSP SRIO channel and frequency settings
- Development troubles, what to do when you encounter bad teammates
- Should 5G be blamed for cheating in the college entrance examination?
- How to use DRV8703-Q1