The use of stm32 is different from that of 51 microcontroller. The microcontroller can directly operate the io port by connecting the crystal oscillator and the power supply, but the clock of stm32 is
After being amplified by the frequency multiplier, the phase-locked loop outputs a stable clock frequency.
Doing so brings many benefits. Although the external clock of stm32 is only 8Mhz, after passing through the frequency multiplier, several clock frequencies can be obtained to provide different clock frequencies for different peripherals.
Therefore, STM32 has many buses, the frequencies of these buses are different, and the buses are closed before use. The corresponding buses must be opened before using the peripherals. This is also for the consideration of reducing power consumption for STM32.
All peripherals using stm32 must add their corresponding driver files.
One thing to note about the GPIO port of stm32 is that the GPIO port can be configured into 8 working modes through the GPIO register:
Floating Input
Input with pull-up resistor
Input with pull-down resistor
Analog Input
Open-drain output
Push-pull output
Multiplexed push-pull output
Multiplexed open-drain output
The first four are input states:
With pull-up resistor means that the stm32 has a pull-up resistor inside, and the same applies to pull-down.
Floating input means that there is nothing connected inside the stm32, and you need to connect an external pull-up resistor;
Analog input is used for AD conversion.
The last four are output states:
Open-drain output means that it can output a low level, but if you want to output a high level, you need a pull-up resistor;
Push-pull output means that it can output both high level and low level;
The last two are to open the second function of IO, and they need to be configured to this state when IO port is multiplexed.
This article will implement the LED cyclic flashing on the GPIOA_Pin_4 port.
Operation Register
Each I/O port of stm32 can be freely programmed, and the register of a single I/O port must be accessed as a 32-bit word. Each I/O port of stm32 is controlled by 7 registers:
The two 32-bit port configuration registers CRL (low eight-bit I/O port configuration register) and CRH (high eight-bit I/O port configuration register) in the configuration mode control the mode and output rate of each I/O port.
Description of each bit of CRL output register (same as CRH):
The reset value of this register is 0X4444 4444, which means the port is configured to floating input mode. Each I/O port occupies four configuration bits, the upper two bits are CNF, which sets the input and output mode. The lower two bits are Mode, which sets the output rate.
There are two 32-bit data registers, IDR and ODR, but only the lower 16 bits are used and can only be read in 16-bit form. The ODR register can be used to select the resistor pull-up (corresponding position 1) or pull-down mode for each I/O port in input mode; or to set the output level of each I/O port in output mode;
Description of each bit of ODR output register (same as IRH):
1 32-bit set/reset register BSRR;
1 16-bit reset register BRR;
1 32-bit latch register LCKR;
The clock of the GPIO port is on the APB2 bus. Change the description of each bit of the clock bus register APB2ENR to:
Register operation method code: (sys.h code refers to the stm32 direct operation register development environment configuration )
#include#include "system.h" //LED port definition #define LED0 PAout(4) // PA4 void Gpio_Init(void); int main(void) { Rcc_Init(9); //System clock settings Gpio_Init(); //Initialize the hardware interface connected to the LED while(1) { LED0=0; delay(300000); //delay 300ms LED0=1; delay(300000); } } void Gpio_Init(void) { RCC->APB2ENR|=1<<2; //Enable PORTA clock GPIOA->CRL&=0XFFF0FFFF; GPIOA->CRL|=0X00030000; //PA4 push-pull output GPIOA->ODR|=1<<4; //PA4 output high }
Library function operation
Even if you want to light up an LED, you must first configure the stm32 clock and open the corresponding bus. Before writing the corresponding code, you need to add the peripheral driver files used to the MDK project. You need to add the general io port driver stm32f10x_gpio.c and the clock driver stm32f10x_rcc.c to the project. These two files are under Libraries/src
After adding Keil, it looks like this:
code show as below:
#include "stm32f10x.h" void RCC_Configuration(void); void GPIO_Configuration(void); void delay(vu32 n); //delay function int main(void) { RCC_Configuration(); GPIO_Configuration(); while(1){ GPIO_SetBits(GPIOA,GPIO_Pin_4); //Call library function to set LED_1 to 1 and output high level delay(2000000); GPIO_ResetBits(GPIOA,GPIO_Pin_4); delay(2000000); } } void GPIO_Configuration(void) { GPIO_InitTypeDef GPIO_InitStructure; //Structure initialization GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_Init(GPIOA, &GPIO_InitStructure); } void delay(vu32 n) { while(--n); } void RCC_Configuration(void) { /* Define enumeration type variable HSEStartUpStatus */ ErrorStatus HSEStartUpStatus; /* Reset system clock settings */ RCC_DeInit(); /* Enable HSE*/ RCC_HSEConfig(RCC_HSE_ON); /* Wait for HSE to start oscillating and stabilize*/ HSEStartUpStatus = RCC_WaitForHSEStartUp(); /* Determine whether the HSE is successfully started, if yes, enter if() */ if(HSESTartUpStatus == SUCCESS) { /* Select HCLK (AHB) clock source as SYSCLK divided by 1 */ RCC_HCLKConfig(RCC_SYSCLK_Div1); /* Select PCLK2 clock source as HCLK (AHB) divided by 1 */ RCC_PCLK2Config(RCC_HCLK_Div1); /* Select PCLK1 clock source as HCLK (AHB) divided by 2 */ RCC_PCLK1Config(RCC_HCLK_Div2); /* Set the FLASH delay cycle number to 2 */ //FLASH_SetLatency(FLASH_Latency_2); /* Enable FLASH prefetch cache */ //FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable); /* Select the phase-locked loop (PLL) clock source as HSE 1 division, the multiplier is 9, then the PLL output frequency is 8MHz * 9 = 72MHz */ RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9); /* Enable PLL */ RCC_PLLCmd(ENABLE); /* Wait for PLL output to stabilize */ while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET); /* Select SYSCLK clock source as PLL */ RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK); /* Wait for PLL to become the SYSCLK clock source */ while(RCC_GetSYSCLKSource() != 0x08); } /* Turn on the GPIOA clock on the APB2 bus */ RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE); }
Previous article:The most convenient timer Systick on stm32 [operation register + library function]
Next article:stm32 direct operation register development environment configuration
Recommended ReadingLatest update time:2024-11-17 00:51
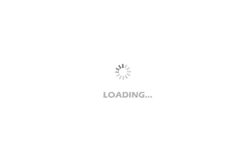
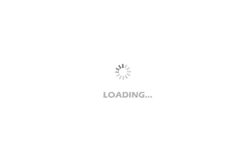
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Pingtouge RVB2601 Review: Light up your LED - GPIO and PWM, and PWM questions
- About the filter circuit of 48V DC after bridge rectification
- 【DFRobot motor driver】+ unboxing
- Detailed explanation of terminal device state switching in TI ZigBee protocol stack
- 6 Common Interface Types in Circuit Design
- GitHub acquires npm
- msp430g2553 hardware IIC
- 6.6kW Totem Pole PFC Reference Design for On-Board Charger
- Does anyone know the manufacturer of this RF IC?
- Oops! The silkscreen is on top of the surface traces