There are many exams recently, so I stopped writing the textbook. I wrote a lot before, but I feel that each chapter is not particularly complete. I posted some of the content recently. You are welcome to correct me. The content of this article is based on my understanding of the firmware library. It is organized and introduced in an easy-to-understand order. Some of it refers to the instructions of the firmware library, but it is basically restated and rewritten in the order I understand. My purpose is very simple. Many people write tutorials just to tell you how to do it, but not why you do it. I will try my best to make the cause and effect clear. This is my starting point. Due to my limited level, there are inevitably great limitations. You are welcome to correct me for specific deficiencies.
Figure 5‑5 CMSIS standard software architecture
The layer is mainly divided into the following three parts:
(1) Core Peripheral Access Layer (CPAL): This layer is implemented by ARM. It includes the definition of register names and addresses, the definition of access interfaces to core registers, NVIC, and debug subsystems, and the definition of access interfaces to special-purpose registers (e.g., CONTROL, xPSR). Since access to special registers is defined inline, ARM uses a unified method to mask differences for different compilers. The interface functions defined in this layer are all reentrant.
(2) Device Peripheral Access Layer (DPAL): This layer is implemented by the chip manufacturer. The implementation of this layer is similar to that of CPAL, and is responsible for defining the hardware register address and peripheral access interface. This layer can call the interface functions provided by the CPAL layer and expand the exception vector table according to the device characteristics to handle the interrupt requests of the corresponding peripherals.
(3) Access Functions for Peripherals (AFP): This layer is also implemented by the chip manufacturer and mainly provides access functions for accessing on-chip peripherals. This part is optional.
For a Cortex-M microcontroller system, CMSIS is implemented through the above three parts:
l Defines a common method for accessing peripheral registers and exception vectors;
l Defines the register names of the kernel peripherals and the names of the kernel exception vectors;
l Defines device-independent interfaces for the RTOS core, including a debug channel.
This allows chip vendors to focus on differentiating their products’ peripheral features and eliminates their need to differentiate their microcontrollers.
Different, incompatible standard requirements need to be maintained during programming to achieve low-cost development. The specific file structure in CMSIS is shown in Table 5-6.
Table 5-6 CMSIS folder structure
CMSIS | Core | Documentation | CMSIS Documentation | |
CM3 | Startup | arm | MDK ARM compiler startup file | startup_stm32f10x_hd.s: large capacity product startup file startup_stm32f10x_md.s: startup file for medium capacity products startup_stm32f10x_ld.s: small capacity product startup file |
gcc_ride7 | GCC compiler startup file | |||
and | IAR compiler startup file | |||
TrueSTUDIO | TrueSTUDIO compiler startup file | |||
This folder contains the STMF10xxx CMSIS files: Microcontroller Peripheral Access Layer and Kernel Device Access Layer: core_cm3.h: CMSIS Cortex-M3 core device access layer header file core_cm3.c: CMSIS Cortex-M3 core device access layer source file stm32f10x.h: CMSIS Cortex-M3 STM32f10xxx microcontroller peripheral access layer header file system_stm32f10x.h: CMSIS header file for the Cortex-M3 STM32f10xxx microcontroller peripheral access layer system_stm32f10x.c: CMSIS Cortex-M3 STM32f10xxx microcontroller peripheral access layer source file |
In the actual development process, according to the needs of the application, there are two ways to use the standard peripheral library (StdPeriph_Lib):
(1) Use peripheral drivers: In this case, application development is based on the API (application programming interface) of the peripheral driver. The user only needs to configure the file "stm32f10x_conf.h" and use the corresponding file "stm32f10x_ppp.h/.c".
(2) Not using peripheral drivers: In this case, application development is based on the peripheral register structure and bit definition files.
The advantages and disadvantages of these two methods have been specifically introduced in the section "Advantages of using standard peripheral libraries for development". It should be noted here that using standard peripheral libraries for development can greatly reduce the workload of software development, which is also a current trend in embedded system development.
The standard peripheral library (StdPeriph_Lib) supports all members of the STM32F10xxx series: large-capacity, medium-capacity and small-capacity products. As can be seen from Table 5‑6, the startup file has divided different series. In actual development, according to the specific model of the STM32 product used, the user can configure the standard peripheral library (StdPeriph_Lib) through the preprocessing define in the file "stm32f10x.h" or through the global settings in the development environment. One define corresponds to one product series.
The supported product series are listed below
STM32F10x_LD: STM32 small capacity products
STM32F10x_MD: STM32 medium-capacity products
STM32F10x_HD: STM32 high-capacity products
The specific scope of these defines in the library file is:
l Interrupt IRQ definition in the file "stm3210f.h"
l Vector table in the startup file. There is one startup file for each of the small capacity, medium capacity and large capacity products.
l Peripheral memory image and register physical address
l Product settings: external crystal oscillator (HSE) value, etc.
System configuration function
Therefore, through macro definition, the standard peripheral library can be applied to different series of products, which also facilitates software migration between different products and greatly facilitates software development.
1.1.4 Use of STM32F10XXX standard peripheral library
The standard peripheral library contains numerous variable definitions and functions. If you do not understand their naming conventions and usage rules, it will cause great trouble to programming. This section will mainly describe the relevant specifications in the standard peripheral library. By learning these specifications, you can use the firmware library more flexibly, and it will also greatly enhance the standardization and readability of the program. At the same time, this specification in the standard peripheral library is also worth using and learning when we are doing other related development.
1. Definition of Abbreviations
The main peripherals in the standard peripheral library are in the form of abbreviations, through which the corresponding peripherals can be easily identified.
abbreviation | Peripherals/Units |
ADC | Analog-to-digital converter |
BKP | Backup register |
CAN | Controller Area Network Module |
CEC | |
CRC | CRC calculation unit |
DAC | Digital to Analog Converter |
DBGMCU | Debugging support |
DMA | Direct Memory Access Controller |
GET OUT | External interrupt event controller |
FLASH | Flash memory |
FSMC | Flexible static memory controller |
GPIO | General purpose input and output |
I2C | I2C Interface |
IWDG | Independent watchdog |
PWR | Power/power consumption control |
RCC | Reset and Clock Controller |
RTC | Real Time Clock |
SDIO | SDIO interface |
Serial Peripheral Interface | |
TIM | Timer |
USED | Universal Synchronous/Asynchronous Receiver/Transmitter |
WWDG | Window Watchdog |
2. Naming conventions
The standard peripheral library follows the following naming conventions: PPP represents any peripheral abbreviation, for example: ADC. Both source and header file names begin with "stm32f10x_", for example: stm32f10x_conf.h. Constants that are only applied to one file are defined in that file; constants that are applied to multiple files are defined in the corresponding header file. All constants are written in uppercase letters. Registers are treated as constants. Their names are written in uppercase letters. In most cases, they are consistent with the abbreviation specifications. The name of the peripheral function begins with the abbreviation of the peripheral plus an underscore. The first letter of each word is written in uppercase letters, for example: SPI_SendData. In the function name, only one underscore is allowed to separate the peripheral abbreviation from the rest of the function name. For function naming, there are generally the following rules:
l A function named PPP_Init, whose function is to initialize the peripheral PPP according to the parameters specified in PPP_InitTypeDef, such as TIM_Init.
l A function named PPP_DeInit, which resets all registers of the peripheral PPP to default values, such as TIM_DeInit.
l A function named PPP_Init, which defines the function of the peripheral by setting various parameters in the PPP_InitTypeDef structure, for example: USART_Init.
l A function named PPP_Cmd, which enables or disables the peripheral PPP, for example: SPI_Cmd.
l A function named PPP_ITConfig, which is used to enable or disable an interrupt source from a PPP peripheral, for example: RCC_ITConfig.
l A function named PPP_DMAConfig, which enables or disables the DMA interface of the peripheral PPP, for example: TIM1_DMAConfig.
l Functions used to configure peripheral functions always end with the string "Config", such as GPIO_PinRemapConfig.
l A function named PPP_GetFlagStatus, which checks whether a flag of the peripheral PPP is set, for example: I2C_GetFlagStatus.
l A function named PPP_ClearFlag, which clears the peripheral PPP flag, for example: I2C_ClearFlag.
l A function named PPP_GetITStatus, which is used to determine whether an interrupt from the peripheral PPP has occurred, for example: I2C_GetITStatus.
l A function named PPP_ClearITPendingBit, which clears the peripheral PPP interrupt pending flag, for example: I2C_ClearITPendingBit.
This naming method is very convenient for writing and reading programs. Taking the example function in the standard peripheral library as an example, the following is an excerpt from STM32F10x_StdPeriph_Examples\ADC\3ADCs_DMA\mian.c.
1 DMA_InitType Def DMA_InitStructure; 2 3 /* DMA1 channel1 configuration ----------------------------------------------*/ 4 5 DMA_DeInit(DMA1_Channel1); 6 7 DMA_InitStructure.DMA_PeripheralBaseAddr = ADC1_DR_Address; 8 9 DMA_InitStructure.DMA_MemoryBaseAddr = (uint32_t)&ADC1ConvertedValue;10 11 DMA_InitStructure.DMA_DIR = DMA_DIR_PeripheralSRC;12 13 DMA_InitStructure.DMA_BufferSize = 1;14 15 DMA_InitStructure.DMA_PeripheralInc = DMA_PeripheralInc_Disable;16 17 DMA_InitStructure.DMA_MemoryInc = DMA_MemoryInc_Disable;18 19 DMA_InitStructure.DMA_PeripheralDataSize = DMA_PeripheralDataSize_HalfWord;20 21 DMA_InitStructure.DMA_MemoryDataSize = DMA_MemoryDataSize_HalfWord;22 23 DMA_InitStructure.DMA_Mode = DMA_Mode_Circular;24 25 DMA_InitStructure.DMA_Priority = DMA_Priority_High;26 27 DMA_InitStructure.DMA_M2M = DMA_M2M_Disable;28 29 DMA_Init(DMA1_Channel1, &DMA_InitStructure);30 31 /* Enable DMA1 channel1 */32 33 DMA_Cmd(DMA1_Channel1, ENABLE);
This program completes the configuration of DMA1 channel. First, DMA_InitType DMA_InitStructure is defined, and then various parameters of DMA_InitType are configured. The naming method of each parameter also uses the agreed naming method. From the naming, it is easy to see the specific function referred to by each parameter. After the function parameters are configured, use DMA_Init(DMA1_Channel1, &DMA_InitStructure); to complete the initialization of the corresponding peripherals, and finally use DMA_Cmd(DMA1_Channel1, ENABLE) to enable the corresponding peripherals. From this example, it is easy to see the benefits of the standardized naming rules of the standard peripheral library for writing and reading programs.
3. Variable Definition
In the early versions, there are 24 variable definitions. The corresponding definitions can be found in the Keil installation root directory. The path is: Keil\ARM\INC\ST\STM32F10x\stm32f10x_type.h
1 /* Includes ------------------------------------------------------------------*/ 2 3 /* Exported types ------------------------------------------------------------*/ 4 5 typedef signed long s32; 6 7 typedef signed short s16; 8 9 typedef signed char s8; 10 11 typedef signed long const sc32; /* Read Only */ 12 13 typedef signed short const sc16; /* Read Only */ 14 15 typedef signed char const sc8; /* Read Only */ 16 17 typedef volatile signed long vs32; 18 19 typedef volatile signed short vs16; 20 21 typedef volatile signed char vs8; 22 23 typedef volatile signed long const vsc32; /* Read Only */ 24 25 typedef volatile signed short const vsc16; /* Read Only */ 26 27 typedef volatile signed char const vsc8; /* Read Only */ 28 29 typedef unsigned long u32; 30 31 typedef unsigned short u16; 32 33 typedef unsigned char u8; 34 35 typedef unsigned long const uc32; /* Read Only */ 36 37 typedef unsigned short const uc16; /* Read Only */ 38 39 typedef unsigned char const uc8; /* Read Only */ 40 41 typedef volatile unsigned long vu32; 42 43 typedef volatile unsigned short vu16; 44 45 typedef volatile unsigned char vu8; 46 47 typedef volatile unsigned long const vuc32; /* Read Only */ 48 49 typedef volatile unsigned short const vuc16; /* Read Only */ 50 51 typedef volatile unsigned char const vuc8; /* Read Only */
CMSIS data types are used in versions after 3.0, and the definition of variables is different, but for the purpose of compatibility with older versions, the above data types are still compatible. The IO type qualifiers of CMSIS are shown in Table 5-7, and the data type comparison between CMSIS and STM32 firmware libraries is shown in Table 5-8. These data types can be found in STM32F10x_StdPeriph_Lib_V3.4.0\Libraries\CMSIS\CM3\DeviceSupport\ST\STM32F10x\stm32f10x.h. This part is defined as follows.
1 /*!< STM32F10x Standard Peripheral Library old types (maintained for legacy purpose) */
2
3 typedef int32_t s32;
4
5 typedef int16_t s16;
6
7 typedef int8_t s8;
8
9 typedef const int32_t sc32; /*!< Read Only */
10
11 typedef const int16_t sc16; /*!< Read Only */
12
13 typedef const int8_t sc8; /*!< Read Only */
14
15 typedef __IO int32_t vs32;
16
17 typedef __IO int16_t vs16;
18
19 typedef __IO int8_t vs8;
20
21 typedef __I int32_t vsc32; /*!< Read Only */
22
23 typedef __I int16_t vsc16; /*!< Read Only */
24
25 typedef __I int8_t vsc8; /*!< Read Only */
26
27 typedef uint32_t u32;
28
29 typedef uint16_t u16;
30
31 typedef uint8_t u8;
32
33 typedef const uint32_t uc32; /*!< Read Only */
34
35 typedef const uint16_t uc16; /*!< Read Only */
36
37 typedef const uint8_t uc8; /*!< Read Only */
38
39 typedef __IO uint32_t vu32;
40
41 typedef __IO uint16_t vu16;
42
43 typedef __IO uint8_t vu8;
44
45 typedef __I uint32_t vuc32; /*!< Read Only */
46
47 typedef __I uint16_t vuc16; /*!< Read Only */
48
49 typedef __I uint8_t vuc8; /*!< Read Only */
Table 5-7 CMSIS IO type qualifiers
IO Class Qualifiers | #define | describe |
_I | volatile const | Read-only access |
_O | volatile | Write-only access |
_I.O | volatile | Read and write access |
Table 5-8 Comparison of firmware library and CMSIS data types
Firmware library type | CMSIS Type | describe |
s32 | int32_t | Volatile read-only signed 32-bit data |
s16 | int16_t | Volatile read-only signed 16-bit data |
s8 | int8_t | Volatile read-only signed 8-bit data |
sc32 | const int32_t | Read only signed 32-bit data |
sc16 | const int16_t | Read only signed 16-bit data |
sc8 | const int8_t | Read only signed 8-bit data |
vs32 | _IO int32_t | Volatile read and write access to signed 32-bit data |
vs16 | _IO int16_t | Volatile read and write access to signed 16-bit data |
vs8 | _IO int8_t | Volatile read and write access to signed 8-bit data |
vsc32 | _I int32_t | Volatile read-only signed 32-bit data |
vsc16 | _I int16_t | Volatile read-only signed 16-bit data |
vsc8 | _I int8_t | Volatile read-only signed 8-bit data |
u32 | uint32_t | Unsigned 32-bit data |
and 16 | uint16_t | Unsigned 16-bit data |
8 | uint8_t | Unsigned 8-bit data |
uc32 | const uint32_t | Read only unsigned 32-bit data |
uc16 | const uint16_t | Read only unsigned 16-bit data |
uc8 | const uint8_t | Read only unsigned 8-bit data |
vu32 | _IO uint32_t | Volatile read and write access to unsigned 32-bit data |
vu16 | _IO uint16_t | Volatile read and write access to unsigned 16-bit data |
vu8 | _IO uint8_t | Volatile read and write access to unsigned 8-bit data |
vuc32 | _I uint32_t | Volatile read-only unsigned 32-bit data |
vuc16 | _I uint16_t | Volatile read-only unsigned 16-bit data |
drag8 | _I uint8_t | Volatile read-only unsigned 8-bit data |
The stm32f10x.h file also contains commonly used Boolean shape variable definitions, such as:
1 typedef enum {RESET = 0, SET = !RESET} FlagStatus, ITStatus;
2
3 typedef enum {DISABLE = 0, ENABLE = !DISABLE} FunctionalState;
4
5 #define IS_FUNCTIONAL_STATE(STATE) (((STATE) == DISABLE) || ((STATE) == ENABLE))
6
7 typedef enum {ERROR = 0, SUCCESS = !ERROR} ErrorStatus;
Different versions of the standard peripheral library have slightly different variable definitions. For example, version 3.4 does not have the definitions of TRUE and FALSE in previous versions. Users can also define their own Boolean variables according to their needs in the above format. When encountering related definition problems when using the standard peripheral library for development, you should first find the corresponding header file definition.
4. Usage steps
The previous sections have introduced in detail the composition structure of the standard peripheral library and the functional description of some main files. So what descriptions are needed to use the standard peripheral library in development? The following is a brief introduction. The usage method introduced here is independent of the development environment. The operation method may be slightly different in different development environments, but the overall process is the same. The next section will introduce the detailed process of using the standard peripheral library in the MDK ARM development environment.
First, create a new project and set the startup file corresponding to the tool chain. You can use the template provided in the standard peripheral library, or you can create a new one according to your needs. The standard peripheral library has provided files corresponding to different tool chains, which are located in the STM32F10x_StdPeriph_Lib_V3.4.0\Libraries\CMSIS\CM3\DeviceSupport\ST\STM32F10x\startup directory.
Secondly, select the specific startup file according to the specific model of the product to be used and add it to the project. The files are mainly divided according to the capacity of the product to be used, and you can select according to the product capacity. The specific meaning of each file can be found in the "stm32f10x.h" file. The excerpt is as follows:
1 #if !defined (STM32F10X_LD) && !defined (STM32F10X_LD_VL) && !defined (STM32F10X_MD) && !defined (STM32F10X_MD_VL) && !defined (STM32F10X_HD) && !defined (STM32F10X_HD_VL) && !defined (STM32F10X_XL) && !defined (STM32F10X_CL)
2
3 /* #define STM32F10X_LD */ /*!< STM32F10X_LD: STM32 Low density devices */
4
5 /* #define STM32F10X_LD_VL */ /*!< STM32F10X_LD_VL: STM32 Low density Value Line devices */
6
7 /* #define STM32F10X_MD */ /*!< STM32F10X_MD: STM32 Medium density devices */
8
9 /* #define STM32F10X_MD_VL */ /*!< STM32F10X_MD_VL: STM32 Medium density Value Line devices */ /* #define STM32F10X_HD */ /*!< STM32F10X_HD: STM32 High density devices */
10
11 /* #define STM32F10X_HD_VL */ /*!< STM32F10X_HD_VL: STM32 High density value line devices */
12
13 /* #define STM32F10X_XL */ /*!< STM32F10X_XL: STM32 XL-density devices */
14
15 /* #define STM32F10X_CL */ /*!< STM32F10X_CL: STM32 Connectivity line devices */
16
17 #endif
18
19 /* Tip: To avoid modifying this file each time you need to switch between these
20
21 devices, you can define the device in your toolchain compiler preprocessor.
22
23 - Low-density devices are STM32F101xx, STM32F102xx and STM32F103xx microcontrollers
24
25 where the Flash memory density ranges between 16 and 32 Kbytes.
26
27 - Low-density value line devices are STM32F100xx microcontrollers where the Flash
28
29 memory density ranges between 16 and 32 Kbytes.
30
31 - Medium-density devices are STM32F101xx, STM32F102xx and STM32F103xx microcontrollers
32
33 where the Flash memory density ranges between 64 and 128 Kbytes.
34
35 - Medium-density value line devices are STM32F100xx microcontrollers where the
36
37 Flash memory density ranges between 64 and 128 Kbytes.
38
39 - High-density devices are STM32F101xx and STM32F103xx microcontrollers where
40
41 the Flash memory density ranges between 256 and 512 Kbytes.
42
43 - High-density value line devices are STM32F100xx microcontrollers where the
44
45 Flash memory density ranges between 256 and 512 Kbytes.
46
47 - XL-density devices are STM32F101xx and STM32F103xx microcontrollers where
48
49 the Flash memory density ranges between 512 and 1024 Kbytes.
50
51 - Connectivity line devices are STM32F105xx and STM32F107xx microcontrollers.
52
53 */
"stm32f10x.h" is the entry file of the entire standard peripheral library. This file contains the definition of all peripheral registers of the STM32F10x series (base address and layout of registers), bit definition, interrupt vector table, address mapping of storage space, etc. In order to make this file suitable for different series of products, the program uses macro definition to achieve matching of different products. The comments of the above program have given in detail the product series corresponding to each startup file. Correspondingly, this entry file should also be modified accordingly. It is necessary to correctly comment/remove the corresponding comment define according to the product series used. Below this program, there is also a comment program /*#define USE_STDPERIPH_DRIVER*/, which is used to select whether to use the standard peripheral library. If this comment is retained, the user can develop programs based on direct access to the peripheral registers defined in "stm32f10x.h". All operations are completed based on registers. Currently, microcontroller development that does not use firmware libraries, such as 51, AVR, MSP430, etc., actually adopts this method. By defining peripheral registers and other aspects in the header files of the corresponding models, the corresponding function design is completed for the corresponding register operations in the program.
If you remove the comment of /*#define USE_STDPERIPH_DRIVER*/, you will use the standard peripheral library for development. The user needs to select the peripheral to be used in the file "stm32f10x_conf.h". The peripheral is also selected by commenting/removing comments. The sample program is as follows
1 /* Uncomment the line below to enable peripheral header file inclusion */
2
3 #include "stm32f10x_adc.h"
4
5 /* #include "stm32f10x_bkp.h" */
6
7 /* #include "stm32f10x_can.h" */
8
9 /* #include "stm32f10x_cec.h" */
10
11 /* #include "stm32f10x_crc.h" */
12
13 /* #include "stm32f10x_dac.h" */
14
15 /* #include "stm32f10x_dbgmcu.h" */
16
17 #include "stm32f10x_dma.h"
18
19 /* #include "stm32f10x_exti.h" */
20
21 /* #include "stm32f10x_flash.h" */
22
23 /* #include "stm32f10x_fsmc.h" */
24
25 #include "stm32f10x_gpio.h"
26
27 /* #include "stm32f10x_i2c.h" */
28
29 /* #include "stm32f10x_iwdg.h" */
30
31 /* #include "stm32f10x_pwr.h" */
32
33 #include "stm32f10x_rcc.h"
34
35 /* #include "stm32f10x_rtc.h" */
36
37 /* #include "stm32f10x_sdio.h" */
38
39 /* #include "stm32f10x_spi.h" */
40
41 /* #include "stm32f10x_tim.h" */
42
43 /* #include "stm32f10x_usart.h" */
44
45 /* #include "stm32f10x_wwdg.h" */
46
47 #include "misc.h" /* High level functions for NVIC and SysTick (add-on to CMSIS functions) */
The above program is from the AD acquisition program in the routine. The program uses AD and DMA, so remove the corresponding comments. At the same time, almost all applications need to use reset and clock as well as general I/O, so these two items are necessary.
Most programs also need to use NVIC interrupt IRQ settings and SysTick clock source settings, so "misc.h" is also necessary.
The above has been configured specifically for specific product signals and program functions. Next, you need to configure the clock used by the system. The system clock is also configured through comments in "system_stm32f10x.c". The program is as follows:
#if defined (STM32F10X_LD_VL) || (defined STM32F10X_MD_VL) || (defined STM32F10X_HD_VL)
/* #define SYSCLK_FREQ_HSE HSE_VALUE */
#define SYSCLK_FREQ_24MHz 24000000
#else
/* #define SYSCLK_FREQ_HSE HSE_VALUE */
/* #define SYSCLK_FREQ_24MHz 24000000 */
/* #define SYSCLK_FREQ_36MHz 36000000 */
/* #define SYSCLK_FREQ_48MHz 48000000 */
/* #define SYSCLK_FREQ_56MHz 56000000 */
#define SYSCLK_FREQ_72MHz 72000000
#endif
If not explicitly defined here then the HSI clock will be used as the system clock.
So far, the main external parameters of the system have been configured. These parameters are mainly implemented by changing the relevant macro definitions. Some development environments, such as Keil, support adding global macro definitions in software settings, so chip series definitions, whether to use firmware library definitions, etc. can also be implemented by adding software.
After completing the main parameter configuration, you can start program development. For standard peripheral library development, you can use the convenient API functions provided in the standard peripheral library to design corresponding functions. The advantages of development based on the standard peripheral library have been introduced in Section 4.2.2. After the configuration is completed, the configuration of the corresponding registers can still be directly changed in the program. The efficiency of the program can be improved by operating the registers. Therefore, a combination of the standard peripheral library and register operations can be used.
Previous article:stm32AD single channel and multi-channel conversion (DMA)
Next article:USB learning notes about stm32 USB_HW.c
Recommended ReadingLatest update time:2024-11-17 03:58
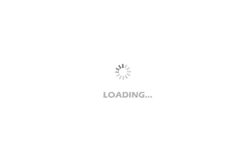
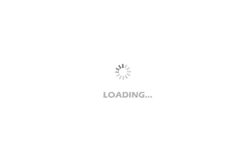
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- All-inclusive OLED
- c0000 28335 Program automatic upgrade problem
- TMS320C62x Boot Mode
- MM32F031 Development Board Review 6: PWM Breathing Light
- Want to learn about switching power supplies? Start by reading this book!
- Practical Application Skills of DC Motors
- Minimalist USB TYPE-C PD3.0/3.1 spoof trigger solution
- [Synopsys IP Resources] Using the ARC SEM130FS processor to meet the safety protection requirements of new automotive SOCs
- 【MicroPython】Using MCO as a clock
- Good news! Owning an ultra-high performance UXR series oscilloscope is no longer a dream!