C language is one of the most efficient, concise and hardware-friendly programming languages that is universally recognized. The transplantation of C language to the single-chip microcomputer MCS-51 began in the mid-to-late 1980s. After nearly 10 years of development, C language has overcome the shortcomings of long code generation and slow running speed. In addition, C language has unparalleled advantages over assembly language in terms of development speed, software quality, structuring, maintainability, etc., and has been increasingly widely used. Keil C51 is a single-chip microcomputer C language compilation system developed by Keil, Germany. The software is fully functional and is one of the most widely used languages by domestic technical developers.
In actual work, it is found that programs written in C language that continuously read the same port often fail to execute after being compiled by Keil C51. Take the 8051 single-chip microcomputer reading 12-bit A/D MAX197 as an example, as shown in Figure 1.
In Figure 1, P1.1 port is used to read the interrupt signal sent by A/D when the conversion is completed, and P1.0 selects to read the high 4 bits or low 8 bits. Now assume that the address of A/D is 8000H, and the starting working word of CH0 port is 40H. To obtain the corresponding high and low conversion data, program in C language as follows.
#include
unsigned char xdata MAX197 _at_ 0x8000;
sbit MAXINT= P1^1;
sbit MAXHBEN= P1^0;
……
void main()
{unsigned char up4, down8; //Set up two variables to receive data
……
MAX197= 0X40; //Start A/D CH0 port for conversion
while(MAXINT) //Wait for conversion to complete
{};
P1.0=0; //Read the lower 8 bits
down8=MAX197;
P1.0=1; //Read the upper 4 bits
up4=MAX197;
}
The above program does not get the high and low bit data as expected. In fact, the data obtained in down8 and up4 are the lower 8 bits. The following is part of the C language code after compilation.
41: //Get the lower 8 bits
42: MAXHBEN=0;
C:0x000C C290 CLR MAXHBEN(0x90.0)
43: down8=MAX197;
C:0x000E 908000 MOV DPTR,#MAX197(0x8000)
C:0x0011 E0 MOVX A,@DPTR
C:0x0012 F509 MOV 0x09,A
44: //Get the upper 4 bits
45: MAXHBEN=1
C:0x0014 D290 SETB MAXHBEN(0x90.0)
46: up4=MAX197;
47:
48:
C:0x0016 F5O8 MOV 0x08,A //0x08 is up4
49: }
By analyzing the above program, we can find that the C compiled program does not read the port again after P1.0 is set to high potential, but directly sends the result of the last read to the high 4-bit variable. If the high bit is read first and the low bit is read later, two high 4-bit data will be obtained. To confirm this, put 4 C language statements that read an external port repeatedly together. After compiling, it is found that only the first statement is compiled and executed. In other words, Keil C51 performs "special" processing when compiling for repeated reading of the same port address, which is very noteworthy. So how to deal with the situation where the same port really needs to be read continuously? The following two methods are introduced for reference.
The first method: add delay.
The delay should not be too long, especially when the conversion speed is required to be high. First, write a delay function:
void delay()
{unsigned char i;
for (i=0, i<=1;i++);
}
Then put the delay program in the middle of the above two reads.
P1.0=0; //Read the lower 8 bits
down8=MAX197:
delay();
P1.0=1; //Read the upper 4 bits
up4=MAX197;
The compiled results are as follows:
49: //Get the lower 8 bits
50: MAXHBEN=0:
C:0x000C C29O CLR MAXHBEN(0x90.0)
51: down8=MAX197;
C:0x000E 908000 MOV DPTR,#MAX197(0x8000)
C:0x0011 E0 MOVX A,@DPTR
C:0x0012 F509 MOV 0x09,A
52: delay();
53: //Get the upper 4 bits
C:0x0014 120029 LCALL delay(C:0029)
54:MAXHBEN = 1;
C:0x0017 D290 SETB MAXHBEN(0x90.0)
55:up4=MAX197;
56:
57:
C:0x0019 E0 MOVX A,@DPTR
C:0x001A F508 MOV 0x08,A
58: }
It can be seen that after P1.0 is set high, the port is read and written again, the program is normal and the upper 4 bits are obtained.
The second method: set another pointer.
void main()
{unsigned char up4,down8; //Set 2 variables for receiving data
unsigned char xdata *pt1;
pt1=0x8000;
……
MAX197=0X40; //Start A/D CH0 port for conversion
while(MAXINT) //Wait for conversion to complete
{};
P1.0=0; //Read the lower 8 bits
down8= MAX197:
P1.0=1; //Read the upper 4 bits
up4=*pt1:
……
The compiled result is as follows:
42: //Get the lower 8 bits
43: MAXHBEN=0;
C:0x0010 C290 CLR MAXHBEN(0x90.0)
44: down8=MAX197;
C:0x0012 908000 MOV DPTR,#MAX197(0x8000)
C:0x0015 E0 M0VX A,@DPTR
C:0x0016 F509 MOV 0x09,A
45: MAXHBEN=1:
46: //Take the upper 4 bits
47:
C:0x0018 D290 SETB MAXHBEN(0x90.0)
48: up4=*pt1:
49:
50:
C:0x001A 8F82 MOV DPL(0x82),R7
C:0x001C 8E83 MOV DPH (0x83),R6
C:0x001E E0 MOVX A,@DPTR
C:0x001F F508 MOV 0x08,A
The above two methods both solve the problem that Keil C51 cannot handle continuous reading and writing of a port, but if the conversion speed requirement is particularly high, it is recommended to use the second method.
Previous article:C51-keil compilation common errors and warnings processing 53
Next article:51 high-precision division program and its use
Recommended ReadingLatest update time:2024-11-16 12:44
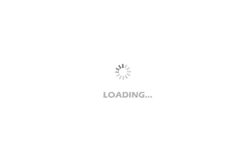
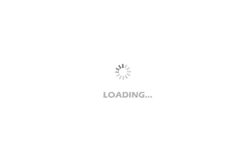
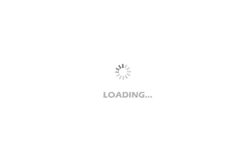
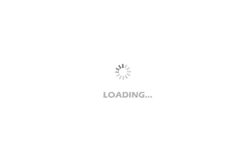
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How to turn off the basic timer of STM32 HAL library
- Reading notes on the good book "Operational Amplifier Parameter Analysis and LTspice Application Simulation"
- AD7190 Issues
- Recruiting MCU sales engineers
- Ask questions about DAF equipment
- VS1053b cannot play music
- HyperLynx High-Speed Circuit Design and Simulation (VIII) High-Speed Board-Level Simulation
- I would like to ask, for NPN tube, does the leakage current ICEO refer to the flow from C to E or from E to C?
- EE Mobile Station Development Board Introduction: DA14580DEVKT-B Evaluation Board
- No doubt! Real-time measurement can also be done with low power consumption