3.3 STRING.H: String Functions
String functions usually take pointer strings as input values. A string consists of 2 or more characters. The end of a string is represented by a null character. In the functions memcmp, memcpy, memchr, memccpy, memmove, and memset, the string length is explicitly specified by the caller, so that these functions can work in any mode.
Function name: memchr
Prototype: extern void *memchr(void *sl, char val, int len);
Function: memchr sequentially searches for len characters in s1 to find the character val, and returns the pointer to val in s1 if successful.
Pointer, or NULL on failure.
Function name: memcmp
Prototype: extern char memcmp(void *sl, void *s2, int len);
Function: memcmp compares the first len characters of string s1 and s2 character by character. Returns 0 if they are equal, and returns 0 if string s1 is larger than s2.
If s2 is greater than or less than s2, a positive or negative number is returned accordingly.
Function name: memcpy
Prototype: extern void *memcpy(void *dest, void *src, int len);
Function: memcpy copies len characters from the memory pointed to by src to dest, and returns the last character in dest.
If src and dest overlap, the results are unpredictable.
Function name: memccpy
Prototype: extern void *memccpy(void *dest, void *src, char val, int len);
Function: memccpy copies len characters from src to dest. If len characters are actually copied, it returns NULL.
The copying process stops after copying the character val, and a pointer to the next element in dest is returned.
Function name: memmove
Prototype: extern void *memmove(void *dest, void *src, int len);
Function: memmove works the same way as memcpy, but the copy areas can overlap.
Function name: memset
Prototype: extern void *memset(void *s, char val, int len);
Function: memset fills len units in pointer s with the value val.
Function name: strcat
Prototype: extern char *strcat(char *s1, char *s2);
Function: strcat copies string s2 to the end of string s1. It assumes that the address area defined by s1 is large enough to accommodate two strings.
The back pointer points to the first character of the s1 string.
Function name: strncat
Prototype: extern char *strncat(char *s1, char *s2, int n);
Function: strncat copies n characters from string s2 to the end of string s1. If s2 is shorter than n, only s2 is copied.
Function name: strcmp
Prototype: extern char strcmp(char *s1, char *s2);
Function: strcmp compares strings s1 and s2, returns 0 if they are equal, otherwise returns
A positive number.
Function name: strncmp
Prototype: extern char strncmp(char *s1, char *s2, int n);
Function: strncmp compares the first n characters in strings s1 and s2. The return value is the same as strncmp.
Function name: strcpy
Prototype: extern char *strcpy(char *s1, char *s2);
Function: strcpy copies string s2 including the terminator to s1 and returns a pointer to the first character of s1.
Function name: strncpy
Prototype: extern char *strncpy(char *s1, char *s2, int n);
Function: strncpy is similar to strcpy, but only copies n characters. If the length of s2 is less than n, s1 is replaced with '0'
Pad to length n.
Function name: strlen
Prototype: extern int strlen(char *s1);
Function: strlen returns the number of characters in string s1 (including the end character).
Function name: strchr, strpos
Prototype: extern char *strchr(char *s1, char c);
extern int strpos(char *s1, char c);
Function: strchr searches for the first occurrence of the character 'c' in string s1. If successful, it returns a pointer to the character.
The search also includes the terminator. Searching for a null character returns a pointer to the null character instead of a null pointer.
strpos is similar to strchr, but it returns the position of a character in a string or -1. The first character of string s1 is position 0.
Function name: strrchr, strrpos
Prototype: extern char *strrchr(char *s1, char c);
extern int strrpos(char *s1, char c);
Function: strrchr searches for the last occurrence of the character 'c' in string s1. If successful, it returns a pointer to the character.
A pointer to a character is returned, otherwise NULL is returned. Searching for s1 also returns a pointer to a character instead of a NULL pointer.
strrpos is similar to strrchr, but it returns the position of a character in a string or -1.
Function names: strspn, strcspn, strpbrk, strrpbrk
Prototype: extern int strspn(char *s1, char *set);
extern int strcspn(char *s1, char *set);
extern char *strpbrk(char *s1,char *set);
extern char *strpbrk(char *s1,char *set);
Function: strspn searches for the first character in string s1 that is not included in set, and the return value is the character in s1 that is included in set
The number of characters. If all characters in s1 are contained in set, the length of s1 (including the terminator) is returned. If s1 is an empty string, 0 is returned.
strcspn is similar to strspn, but it searches for the first character in string s1 that is contained in set. strpbrk is very similar to strspn, but it returns a pointer to the character that was searched for, rather than a count, or NULL if it is not found.
strrpbrk is similar to strpbrk, but it returns a pointer in s1 to the last character found in the set character set.
3.4 STDLIB.H: Standard Functions
Function name: atof
Prototype: extern double atof(char *s1);
Function: atof converts the string s1 to a floating point value and returns it. The input string must contain numbers that conform to the floating point value specification.
The C51 compiler treats the data types float and double the same.
Function name: atol
Prototype: extern long atol(char *s1);
Function: atol converts the s1 string into a long integer value and returns it. The input string must contain the same
The number of symbols.
Function name: atoi
Prototype: extern int atoi(char *s1);
Function: atoi converts the string s1 to an integer and returns it. The input string must contain numbers that conform to the integer specification.
3.5 MATH.H: Mathematical Functions
Function names: abs, cabs, fabs, labs
Prototype: extern int abs(int va1);
extern char cabs(char val);
extern float fabs(float val);
extern long labs(long val);
Function: abs determines the absolute value of the variable val. If val is positive, it is returned unchanged; if it is negative,
Returns the opposite number. These four functions have the same function except that the variables and return values are different.
Function name: exp, log, log10
Prototype: extern float exp(float x);
extern float log(float x);
extern float log10(float x);
Function: exp returns the power of x with base e, log returns the natural number of x (e=2.718282), log10 returns x raised to 10
The number with base .
Function name: sqrt
Prototype: extern float sqrt(float x);
Function: sqrt returns the square root of x.
Function name: rand, srand
Prototype: extern int rand(void);
extern void srand(int n);
Function: rand returns a pseudo-random number between 0 and 32767. srand is used to initialize the random number generator.
A known (or expected) value such that successive calls to rand will produce the same sequence of random numbers.
Function names: cos, sin, tan
Prototype: extern float cos(float x);
extern float sin(float x);
extern flat tan(flat x);
Function: cos returns the cosine of x. Sin returns the sine of x. Tan returns the tangent of x. All function variables
The range is -π/2 to +π/2, and the variable must be between ±65535, otherwise a NaN error will be generated.
Function names: acos, asin, atan, atan2
Prototype: extern float acos(float x);
extern float asin(float x);
extern float atan(float x);
extern float atan(float y,float x);
Function: acos returns the arccosine of x, asin returns the sine of x, and atan returns the arctangent of x.
The range of atan2 is -π/2 to +π/2. atan2 returns the arc tangent of x/y, and its range is -π to +π.
Function names: cosh, sinh, tanh
Prototype: extern float cosh(float x);
extern float sinh(float x);
extern float tanh(float x);
Function: cosh returns the hyperbolic cosine of x; sinh returns the hyperbolic sine of x; tanh returns the hyperbolic tangent of x
value.
Function name: fpsave, fprestore
Prototype: extern void fpsave(struct FPBUF *p);
extern void fprestore (struct FPBUF *p);
Function: fpsave saves the state of a floating-point subroutine. fprestore restores the state of a floating-point subroutine to its original state.
These two functions are useful when performing floating-point operations using interrupt routines.
3.6 ABSACC.H: Absolute Address Access
Function names: CBYTE, DBYTE, PBYTE, XBYTE
Prototype: #define CBYTE((unsigned char *)0x50000L)
#define DBYTE((unsigned char *)0x40000L)
#define PBYTE((unsigned char *)0x30000L)
#define XBYTE((unsigned char *)0x20000L)
Function: The above macro definition is used to access the 8051 address space with an absolute address, so byte addressing is possible.
Addressing CODE area, DBYTE addresses DATA area, PBYTE addresses XDATA area (through MOVX @R0 command), XBYTE addresses XDATA area (through MOVX @DPTR command).
Example: The following instruction accesses address 0x1000 in the external memory area
xval=XBYTE[0x1000];
XBYTE[0X1000]=20;
By using the #define directive, absolute addresses can be defined with symbols, such as the symbol X10 can be equal to the address XBYTE[0x1000]: #define X10 XBYTE[0x1000].
Function Name: CWORD, DWORD, PWORD, XWORD
Prototype: #define CWORD((unsigned int *)0x50000L)
#define DWORD((unsigned int *)0x40000L)
#define PWORD((unsigned int *)0x30000L)
#define XWORD((unsigned int *)0x20000L)
Function: These macros are similar to the above, except that they specify the type as unsigned int. With flexible data types,
All address spaces are accessible.
3.7 INTRINS.H: Intrinsic Functions
Function names: _crol_, _irol_, _lrol_
Prototype: unsigned char _crol_(unsigned char val,unsigned char n);
unsigned int _irol_(unsigned int val,unsigned char n);
unsigned int _lrol_(unsigned int val,unsigned char n);
Functions: _crol_, _irol_, _lrol_ shift val left by n bits in bitwise form. This function is similar to the 8051 "RLA" instruction.
Related, the above functions differ in parameter types.
example:
#include
main()
{
unsigned int y;
y=0x00ff;
y=_irol_(y,4);
}
Function names: _cror_, _iror_, _lror_
Prototype: unsigned char _cror_(unsigned char val,unsigned char n);
unsigned int _iror_(unsigned int val,unsigned char n);
unsigned int _lror_(unsigned int val,unsigned char n);
Functions: _cror_, _iror_, _lror_ shift val right by n bits in bitwise form. This function is similar to the 8051 "RRA" instruction.
Related, the above functions differ in parameter types.
example:
#include
main()
{
unsigned int y;
y=0x0ff00;
y=_iror_(y,4);
}
Function name: _nop_
Prototype: void _nop_(void);
Function: _nop_ generates a NOP instruction. This function can be used for time comparison in C programs. The C51 compiler generates a NOP instruction in the _nop_ function.
No function call is generated during the operation of the number, that is, the NOP instruction is directly executed in the program.
example:
P()=1;
_nop_();
P()=0;
Function name: _testbit_
Prototype: bit_testbit_(bit x);
Function: _testbit_ generates a JBC instruction that tests a bit and returns 1 if it is set, otherwise it returns 0.
If the bit is set to 1, reset it to 0. The 8051's JBC instruction is used for this purpose. _testbit_ can only be used on directly addressable bits; its use in expressions is not allowed.
STDARG.H: Variable parameter table
The C51 compiler allows reentrant function arguments (symbolized by "..."). The header file STDARG.H allows processing of function parameter lists whose length and data type are unknown at compile time. For this purpose, the following macros are defined.
Macro name: va_list
Function: Pointer to parameter.
Macro name: va_stat(va_list pointer,last_argument)
Function: Initialize the pointer to the parameter.
Macro name: type va_arg(va_list pointer,type)
Function: Returns the parameter of type type.
Macro name: va_end(va_list pointer)
Function: Identify the dummy macro at the end of the table.
3.8 SETJMP.H: Jump all the way
The functions in Setjmp.h are used as normal series of number calls and function terminations, which allow direct returns from deep function calls.
Function name: setjmp
Prototype: int setjmp(jmp_buf env);
Function: setjmp stores the status information in env for use by the function longjmp. When setjmp is called directly, the return value is
It is 0 and returns a non-zero value when called by longjmp. setjmp can only be called once in the statement IF or SWITCH.
Function name: long jmp
Prototype: long jmp(jmp_buf env,int val);
Function: longjmp restores the state in env when setjmp is called. The program continues to execute, as if the function setjmp
The value returned by setjmp is the value val passed in the function longjmp, and the values of all automatic variables and variables not defined with volatile in the function called by setjmp are changed.
3.9 REGxxx.H: Accessing SFR and SFR-BIT Addresses
The files REG51.H, REG52.H and REG552.H allow access to the addresses of the SFRs and SFR-bits of the 8051 series. These files contain the #include directive and define all the SFR names required to address the peripheral circuit addresses of the 8051 series. For some other devices in the 8051 series, the user can easily generate a ".h" file with a file editor.
The following example shows access to 8051 PORT0 and PORT1:
#include
main() {
if(p0==0x10) p1=0x50;
}
Previous article:4*4 keyboard matrix
Next article:C51 Delay Program
Recommended ReadingLatest update time:2024-11-16 16:37
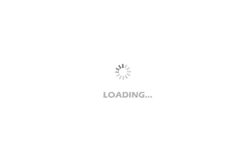
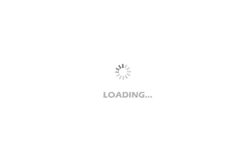
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Playing with Zynq Serial 41——[ex60] Image smoothing processing of OV5640 camera
- Thank you + thank you EEWORLD community
- Medical electronics popular data download collection
- Tailing Micro B91 Development Kit --- Testing Power Consumption in BLE Connections
- Application areas of Bluetooth Low Energy
- GaN Power Devices
- PIN diodes for RF circuits explained in detail
- During the fight against the epidemic, we will teach you how to have instruments in your mind without having any in your hands.
- Migrating between TMS320F28004x and TMS320F28002x
- K210 performance test again