Hardware equipment: Network card: dm9000 NandFlash: K9F1208U0C 64M
1. Download and decompress the kernel, modify the compiler options of the top-level Makefile:
ARCH ?= arm
CROSS_COMPILE ?= arm-softfloat-linux-gnu-
2. Modify linux-2.6.12/arch/arm/mach-s3c2410/devs.c to support NandFlash and DM9000 network card:
Add header file:
#include
#include
#include
#include
Add support for dm9000 network card device
EXPORT_SYMBOL(s3c_device_usb);
/* NIC DM9000A64 driver,copy from linux-2.6.14. add by guowenxue 2008.08.15 */
/* My board: nETH_CS connects to NGCS3, IRQ_LAN connects to EINT9, CMD connects to A2 */
#ifndef S3C2410_CS3
#define S3C2410_CS3 (0x18000000)
#endif
/* change 0x08000000 to S3C2410_CS3 */
static struct resource s3c_dm9000_resource[] =
{
[0] = {
.start = S3C2410_CS3 + 0x300,
.end = S3C2410_CS3 + 0x300 + 0x03,
.flags = IORESOURCE_MEM
},
[1] = {
.start = S3C2410_CS3 + 0x300 + 0x04,
.end = S3C2410_CS3 + 0x300 + 0x04 + 0x3f, // It's 0x3f, not 0x3
.flags = IORESOURCE_MEM
},
[2] = {
.start = IRQ_EINT9, // My board use EINT9
.end = IRQ_EINT9,
.flags = IORESOURCE_IRQ
}
};
static struct dm9000_plat_data s3c_device_dm9000_platdata =
{
.flags= DM9000_PLATF_16BITONLY
};
struct platform_device s3c_device_dm9000 =
{
.name= "dm9000",
.id= -1,
.num_resources= ARRAY_SIZE(s3c_dm9000_resource),
.resource= s3c_dm9000_resource,
.dev= {
.platform_data = &s3c_device_dm9000_platdata,
}
};
EXPORT_SYMBOL(s3c_device_dm9000);
/**** Add end *****/
/* LCD Controller */
Add NandFlash support:
/* NAND Controller */
static struct resource s3c_nand_resource[] = {
[0] = {
.start = S3C2410_PA_NAND,
.end = S3C2410_PA_NAND + S3C24XX_SZ_NAND,
.flags = IORESOURCE_MEM,
}
};
/******* add by guowenxue 2008.10.28 *********/
static struct mtd_partition partition_info[] =
{
{
name: "mtdblock0 uboot 1M", //mtdblock0 1*1M = 1M
size: 0x00100000,
offset: 0,
}, {
name: "mtdblock1 kernel 3M", //mtdblock1 3*1M = 3M
size: 0x00300000,
offset: 0x00100000,
}, {
name: "mtdblock2 rootfs 6M", //mtdblock2 6*1M = 6M
size: 0x00600000,
offset: 0x00400000,
}, {
name: "mtdblock3 apps 27M", //mtdblock3 27*1M = 27M
size: 0x01B00000,
offset: 0x00A00000,
}, {
name: "mtdblock4 user 27M", //mtdblock4 27*1M = 27M
size: 0x01B00000,
offset: 0x02500000,
}
};
struct s3c2410_nand_set nandset =
{
nr_partitions: 5, /* the number of partitions */
partitions: partition_info, /* partition table */
};
struct s3c2410_platform_nand superlpplatform=
{
tacls:0,
twrph0:30,
twrph1:0,
sets: &nandset,
nr_sets: 1,
};
struct platform_device s3c_device_nand =
{
.name = "s3c2410-nand", /* Device name */
.id = -1, /* Device ID */
.num_resources = ARRAY_SIZE(s3c_nand_resource),
.resource = s3c_nand_resource, /* Nand Flash Controller Registers */
/* Add the Nand Flash device */
.dev = {
.platform_data = &superlpplatform
}
};
/****** add end ********/
3, 修改文件linux-2.6.12/arch/arm/mach-s3c2410/devs.h,导出dm9000设备:
extern struct platform_device s3c_device_sdi;
extern struct platform_device s3c_device_dm9000; //add by guowenxue,2008.11.15
4, 修改文件linux-2.6.12/arch/arm/mach-s3c2410/mach-smdk2410.c, 添加dm9000和nandflash
static struct platform_device *smdk2410_devices[] __initdata = {
&s3c_device_usb,
&s3c_device_lcd,
&s3c_device_wdt,
&s3c_device_i2c,
&s3c_device_iis,
&s3c_device_nand, // this member add by guowenxue 2003.10.28
&s3c_device_dm9000, // this member add by guowenxue 2003.11.15
};
5, 从linux-2.6.14的内核中拷贝driver/net/dm9000.c和dm9000.h到相应目录,同时拷贝
linux-2.6.14/include/linux/dm9000.h到linux-2.6.12的相应目录.修改driver/net/dm9000.c如下:
在文件开始处添加:
#define INTMOD (0x4A000004) //These two line add by guowenxue 2008.11.15
static void *intmod;
After declaring the variable in the static int dm9000_probe(struct device *dev) function, add:
intmod=ioremap_nocache(INTMOD,0x0000004);
writel(0x0,intmod);
Also in this function, comment out the line that reads the MAC address from ROM and replace it with the MAC address we set ourselves:
#if 0 /* Read SROM content */
for (i = 0; i < 64; i++)
((u16 *) db->srom)[i] = read_srom_word(db, i);
/* Set Node Address, the first 6 bytes is the MAC address */
for (i = 0; i < 6; i++)
ndev->dev_addr[i] = db->srom[i];
#endif
add:
#if 1 // add by guowenxue, set MAC address by myself 2008.11.17
unsigned char def_eth_mac_addr[]={0x22,0x44,0x66,0x88,0x44,0x66};
for(i=0; i<6; i++)
ndev->dev_addr[i] = def_eth_mac_addr[i];
#endif
in the function static int dm9000_open(struct net_device *dev) add set_irq_tye():
set_irq_type(dev->irq, IRQT_RISING); // The application type is rising edge interrupt, otherwise the PC cannot ping the development board.
if (request_irq(dev->irq, &dm9000_interrupt, SA_SHIRQ, dev->name, dev))
return -EAGAIN;
6, Modify linux-2.6.12/drivers/serial/s3c2410.c
Modify #define S3C24XX_SERIAL_DEVFS "tts/"
to: #define S3C24XX_SERIAL_DEVFS "ttyS"
Modify the member .dev_name of the structure static struct uart_driver s3c24xx_uart_drv to:
// .dev_name = "s3c2410_serial",
.dev_name = "ttyS",
The previously compiled kernel could not initialize the console, and entered busybox. It turned out that the default serial port name
of s3c2410 was not ttyS0 or tySAC0. In addition, the serial port major device number of S3C2410 is registered as 204, and the minor device number is 64. So we
should pay attention to it in the root file system: #mknod –mode=755 ttyS0 c 204 64
7, Modify the file drivers/net/arm/Kconfig, Add dm9000 driver options:
config DM9000
tristate "DM9000 support"
depends on ARM && NET_ETHERNET
select CRC32
select MII
---help---
Support for DM9000 chipset.
To compile this driver as a module, choose M here and read
called dm9000.
#config MACE
#tristate "MACE (Power Mac ethernet) support"
#depends on NET_ETHERNET && PPC_PMAC && PPC32
8, Modify the file linux-2.6.12/drivers/net/Makefile, add the compilation option of DM9000.
obj-$(CONFIG_SMC91X) += smc91x.o
obj-$(CONFIG_DM9000) += dm9000.o
9, make menuconfig generates the configuration file:
System Type --->
ARM system type (Samsung S3C2410) --->
S3C24XX Implementations --->
[*] SMDK2410/A9M2410
[*] S3C2410 DMA support
[*] Support Thumb user binaries
Boot options --->
(noinitrd root=/dev/mtdblock2 init=/linuxrc console=ttySAC0,115200) Default kernel command
Floating point emulation --->
[*] NWFPE math emulation
Device Drivers --->
Memory Technology Devices (MTD) --->
<*> Memory Technology Device (MTD) support
<*> MTD concatenating support
[*] MTD partitioning support
[*] Command line partition table parsing
<*> Direct char device access to MTD devices
<*> Caching block device access to MTD devices
RAM/ROM/Flash chip drivers --->
<*> Detect flash chips by Common Flash Interface (CFI) probe
<*> Detect non-CFI AMD/JEDEC-compatible flash chips
<*> Support for Intel/Sharp flash chips
<*> Support for AMD/Fujitsu flash chips
<*> Support for RAM chips in bus mapping
NAND Flash Device Drivers --->
<*> NAND Device Support
<*> NAND Flash support for S3C2410 SoC
Networking support --->
[*] Networking support
[*] Network device support
Ethernet (10 or 100Mbit) --->
[*] Ethernet (10 or 100Mbit)
<*> DM9000 support
Character devices --->
Serial drivers --->
<*> Samsung S3C2410 Serial port support
[*] Support for console on S3C2410 serial port
File systems --->
Pseudo filesystems --->
[*] /proc file system support
可选/dev file system support
[*] /dev/pts Extended Attributes
[*] /dev/pts Security Labels
[*] tmpfs Extended Attributes
[*] tmpfs Security Labels
Miscellaneous filesystems --->
<*> Journalling Flash File System v2 (JFFS2) support
(0) JFFS2 debugging verbosity (0 = quiet, 2 = noisy)
[*] JFFS2 support for NAND flash
[*] Advanced compression options for JFFS2
[*] JFFS2 ZLIB compression support
[*] JFFS2 RTIME compression support
<*> Compressed ROM file system support (cramfs)
<*> FreeVxFS file system support (VERITAS VxFS(TM) compatible)
Network File Systems --->
<*> NFS file system support
[*] Provide NFSv3 client support
[*] Provide NFSv4 client support (EXPERIMENTAL)
移植过程中碰到的主要问题及解决方法
1, NAND_ECC_NONE selected by board driver. This is not recommended !!
and Reading data from NAND FLASH without ECC is not recommended
This is because the ECC detection in mtd/nand/s3c2410.c is turned off:
Do not add the following line of code at the end of the function s3c2410_nand_init_chip():
Chip->eccmode = NAND_ECC_NONE;
Without turning off hardware ECC, it is normal to use cramfs and jffs2 file systems, but I haven't tried yaffs2 yet.
2, ftl_cs: FTL header not found.
When making menuconfig:
Device Drivers -?
Memory Technology Devices (MTD) -? Do not select the following three options
<>FTL (Flash Translation Layer) support
<> NFTL (NAND Flash Translation Layer) support
<>INFTL (Inverse NAND Flash Translation Layer) support
3, When starting busybox in linux, it prompts: Can't open /dev/ttyS0: No such file or directoryThis
is because the serial port driver of s3c2410 in the kernel does not register the serial port device name ttyS0, and
the primary and secondary device numbers of the serial port device are not the devices under the /dev directory of the root file system. According to the above method of transplanting the kernel, modify
the serial port driver of s3c2410 (linux-2.6.12/drivers/serial/s3c2410.c) and it will be OK.
4. Several questions about the dm9000 network card driver:
dm9000 Ethernet Driver
dm9000: read wrong id 0x2b2a2928
dm9000: read wrong id 0x2b2a2928
dm9000: wrong id: 0x2b2a2928
dm9000: not found (0).
The main problem is that I followed the method of porting the dm9000 driver from the Internet at the beginning.
I did not set the correct chip select line (NGCS3), interrupt line (INT9) and the CMD connection to A2 in the file linux-2.6.12/arch/arm/mach-s3c2410/devs.c.
dm9000 Ethernet Driver
eth%d: Invalid ethernet MAC address. Please set using ifconfig
eth0: dm9000 at c4862300,c4864304 IRQ 53 MAC: 00:00:00:00:00:00:00In
the source code of the dm9000 driver (driver/net/dm9000.c), the default is to read the MAC address from the ROM, and there is no ROM on my development
board , so I set the MAC address myself in the above driver and it is OK.When
using tftp to download files in busybox, it always prompts timeout. Later, it was found that the development board can ping the PC, but the PC
cannot ping the development board. If the development board pings the PC, the PC can ping the development board.In
the function static int dm9000_open(struct net_device *dev) of the driver file (driver/net/dm9000.c), before applying for
an interrupt, specify the interrupt type to be a rising edge interrupt. For detailed methods, refer to the above code.About
the jffs2 file system:
An error will be reported when using the jffs2.img created by the following command:
On the PC: #mkfs.jffs2 -r jfss2 -o jffs2.imgOn
the development board:
>:echo jffs2.img > /dev/mtdb3
nand_write_ecc: Attempt to write not page aligned data
>: mount -t jffs2 /dev/mtdblock3 /apps/
mtd->read(0x400 bytes from 0x0) returned ECC error
CLEANMARKER node found at 0x00000000 has totlen 0xc != normal 0x0
mtd->read(0x2c bytes from 0xc) returned ECC error
The jffs2.img created by the following command is burned normally:
mkfs.jffs2 -r jffs2 -o jffs2.img -e 0x4000 --pad=0x1B00000 -s 0x200 –n
Explanation of several options of mkfs.jffs2:
(1)-r: Specify the source folder to be made into image.
(2)-o: Specify the file name of the output image file.
(3)-e: The block size of each block to be erased. The default is 64KB. Please note that different flash memory has different block sizes. Mine is Samsung's K9F1208U0B.
(4)--pad (-p): Use hexadecimal to indicate the size of the output file, which is the size of jffs2.img. It is very important that in order not to waste flash space, this value should be consistent with the block size planned by the flash driver. For my board, it is 27MB (0x1B00000).
(5) If a warning similar to "CLEANMARKER node found at 0x0042c000 has totlen 0xc != normal 0x0" appears after mounting, add -n and it will disappear.
Previous article:Nandflash driver in-depth analysis (based on S3C2410)
Next article:s3c2410 processor storage expansion - SDRAM
Recommended ReadingLatest update time:2024-11-17 07:56
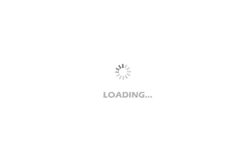
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- MSP430F5529 LAB CODE experimental routine
- Compile and debug ESP32 programs in VSCode
- Questions about generating light painting with allegro16.6
- NUCLEO-G431RB Review -> OPAMP
- CCS8.0
- Advantages of Integrated Current Sensing
- [GD32L233C-START Review] - 8. Driving a 1.5-inch OLED screen using ordinary GPIO
- High-speed acquisition module
- What does USB_OTG_FS and USB_OTG_HS mean?
- I2C Master Mode for TI BLE CC2541