1 The relationship between CPU storage structure and variables
Variables need storage space. Different storage spaces lead to different working efficiency when using variables.
The typical operating environment of standard C is the 8086 (including IA-32 series) kernel. Its storage structure is that there are registers inside the CPU and memory outside. The access speed of registers is much higher than that of memory. In standard C, variables without special definitions are placed in memory. Using register can force variables to be stored in registers. For variables that are used frequently and in small quantities, this storage mode can be selected to obtain higher working efficiency.
In contrast, the storage structure of the 51 core microcontroller is a bit strange. It has 3 storage spaces: program memory space (64 KB including on-chip and off-chip), off-chip data memory space (64KB), on-chip data memory and special function register space. It does not have registers in the true sense. Its registers are actually part of the on-chip data memory (such as R0~R7) and special function registers (such as A, B, etc.). Therefore, using variables in Keil C51 is very different from standard C.
2 Keil C51 variable analysis
Keil C51 supports most of the original variable types of standard C, but adds a variety of storage types for these variables, and also adds some variables that are not in standard C.
2.1 New variable storage types added by Keil C51
The format of defining variables in Keil C51 is as follows:
[Storage type] Data type [Storage type] Variable name table;
Among them, [Storage type] is not in standard C. There are 6 types of [Storage type], which are introduced as follows:
①data
②bdata. Store the variable in the on-chip bit-addressable data memory. In the target code, the variable can be easily bit-processed, and it is the
③idata. Store the variable in the on-chip indirectly addressable data memory. In the 52 core, when the on-chip direct addressing data memory is not enough, the 128-byte indirect addressing data memory can be used. The access speed is generally
④xdata. Store the variable in the off-chip data memory. In the target code, only the "MOVX A, @DPTR" and "MOVX@DPTR, A" instructions can be used to access the variable. The access speed is the slowest, but the storage space is the largest (64KB).
⑤pdata. Store the variable in the first page (00H~FFH) of the off-chip data memory. In the target code, the "MOVX A, @Ri" and "MOVX@Ri, A" instructions can be used to access the variable. The access speed is the same as xdata, and the storage space is 256 bytes.
⑥code
2.2 Keil C51's new pointer variable storage type
The pointer variable format in Keil C51 is as follows:
data type [data storage type] * [pointer storage type] identifier;
where [data storage type] and [pointer storage type] are not in standard C. [Data storage type] defines the space for data (i.e., addressable object) storage, and [pointer storage type] defines the space for pointer storage itself. If [data storage type] is not used, the pointer is a general pointer, occupying 3 bytes; if [data storage type] is used, the pointer is a memory-based pointer, occupying 1 to 2 bytes.
2.3 Keil C51's new variable type
bit: bit variable. Stored in a bit-addressable byte (20H to 2FH) of the on-chip data memory, this variable has high practical value in real-time control.
sfr: special function register variable. Stored in the on-chip special function register, used to read and write special function registers.
sbit: special function register bit variable. Stored in a certain bit of the bit-addressable byte (address divisible by 8) of the on-chip special function register, used to read and write the bit-addressable bit of the special function register.
sbitl6: 16-bit special function register variable. Stored in the low address of 2 consecutive bytes of the on-chip special function register, this variable type is rarely used.
The above new variable types in Keil C51 do not support array and pointer operations.
3 The necessity of using variable storage mode in Keil C51
In Keil C51, the storage mode of variables is an option. If this option is not used, Keil C51 will automatically perform the optimal allocation during compilation. However, this processing method has the following disadvantages:
① The system does not know the usage frequency of various variables. It is possible that the variables with high usage frequency use the off-chip storage method with slow access speed, while the variables with low usage frequency use the on-chip storage method, which reduces the running efficiency of the program;
② When using pointer addressing, because the storage method of the addressing object is unknown, general pointers have to be used. In Keil C51, general pointers take up 1 to 2 bytes more, and the storage method must be judged when used, which increases the addressing operation time.
If the storage type can be defined when defining the variable, the storage space of the 51 core microcontroller can be used efficiently to obtain high-quality target code.
4 How to use Keil C51 variables
4.1 Global variables and static local variables
Global variables are generally used in multiple functions and are valid during the entire program. Although static local variables are only used in one function, they are also valid during the entire program. For these variables, you should try to choose the data
unsigned int data
4.2 Arrays (including global and local)
Arrays are generally defined using the idata storage type, and the "MOV@Ri" instruction is used in the target code for indirect addressing. If an error is reported during compilation due to too many array elements, you can use the pdata and xdata storage types instead. It is not very meaningful
to define an array as a data
4.3 Data for table lookup
The characteristics of this type of data are that it needs to remain unchanged and is read-only when used, so it should be defined as code
global and local code type variables.
4.4 Non-static local variables
Non-static local variables are only used within a function, and the variables are released when the function is exited.
If the system uses the small storage mode, these variables can be stored without description, and the compiler software will decide according to the optimal principle. Because non-static local variables used only in functions may use working registers R0~R7, which will be faster and save more storage space. For example:
unsigned char i, j; // The system will use R0~R7 to store i and j as much as possible.
If the system uses the compact or large storage mode, these variables should be defined as data storage mode to prevent the system from defining them as pdagta or xdata mode when deciding on its own, which will reduce work efficiency.
4.5 Pointer
As mentioned above, there are two storage types when defining pointer variables: data storage type, which describes the storage type of the addressed object; pointer storage type, which describes the storage type of the pointer itself. When the data storage type is xdata, the pointer itself occupies 2 bytes; when the data storage type is pdata and idata, etc., the pointer itself occupies 1 byte; if the data storage type is not specified, the pointer itself will occupy 3 bytes. Therefore, when using pointers in KeilC51, you should try to define the data storage type, but pay special attention to the data storage type in the pointer must be consistent with the storage type of the addressed object. Pointers are frequently used, and they need to be constantly set, modified and used, so their own storage type should be selected as data type. For example, when defining an array, its storage type is defined at the same time. When addressing it with a pointer later, the storage type of the array is added to the data type of the pointer. The method is as follows:
4.6 Ambiguous variables
In standard C, if you want to use an ambiguous variable, you can only use an enumeration type. For example:
When the above program is used in Keil C51, although the variable t has only two states, 0 and 1, it still occupies one byte in the target code. This processing method not only wastes storage resources, but also prolongs the processing time. This is not a big problem for the 8086 core, but it cannot be ignored in the 51 core with limited resources and low running speed. The following method can be used in Keil C51:
The effects of these two methods are exactly the same, but in the target code, variable t only occupies 1 bit (i.e. 1/8 byte), and because there are bit processing instructions in the 51 core microcontroller instruction system, the generated target code occupies less memory and runs faster.
4.7 Special function register variables (including bit variables)
Among the special function registers, accumulator A, register B, stack pointer SP and data pointer DPTR are used by the system and are not provided to users in C51. Other special function registers can be defined as variables using sfr, and the bits whose addresses are divisible by 8 can also be defined as bit variables using bsfr. By accessing these variables, you can read and write the special function registers and their bit-addressable bits, so as to achieve the purpose of operating the internal hardware of the microcontroller. For standard 51 core microcontrollers, these special function register variables have been defined in the header files reg51.h, reg52.h or other header files. Users can use #include to include this header file and then use it. Now many 51 core compatible microcontrollers have expanded more special function registers, which need to be defined by users. For specific methods, please refer to the device instructions.
4.8 External data memory variables
If the storage type is set to pdata and xdata, the variables will be stored in the external data memory. These two storage types have the slowest access speed and should not be used unless absolutely necessary. When using these two storage types, pay attention to using them to save only the original data or final results, minimize the number of accesses to them, and do not use them for intermediate results that need to be accessed frequently.
4.9 Other hardware extended with external data memory addresses
Other hardware extended outside the microcontroller generally borrows the external data memory address and is expressed in the form of external data memory units. For these hardware, pointers can be used for read and write operations. For example:
5 Summary
The variables in Keil C51 have added storage types, which makes them slightly more complicated to use than standard C. In Keil C51, different storage types of variables require different access times. Since the C51 core microcontroller has few resources and slow speed, the impact of variable storage types on system operating speed cannot be ignored. Based on the understanding of the relationship between variables and microcontroller storage structures, according to the program's requirements for the use of variables, reasonably selecting the variable storage type can achieve higher work efficiency on the same hardware.
Previous article:C51 delay program re-throws the original
Next article:KEIL C51 error solution
Recommended ReadingLatest update time:2024-11-16 22:27
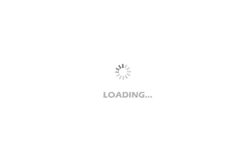
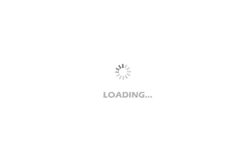
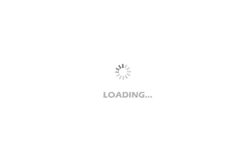
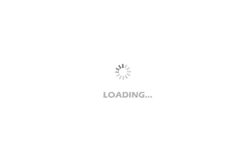
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- NXP Rapid IoT Review】+④NXP Rapid IoT Operation Time Synchronization
- [Evaluation of EVAL-M3-TS6-665PN development board] 1. Hardware circuit
- AM335X GPIO Register Operation
- Overview of IoT Communication Technologies
- ESD Request Assistance
- How to compensate for the unbalanced output of a strain gauge bridge?
- MSP430G2553 State Machine Modeling
- The variable occasionally has a very large value
- [NXP Rapid IoT Review] - NXP Rapid IOT APP Mobile Bluetooth Networking
- USB matching resistor