//The program does not perform key debounce, nor does it consider the situation where multiple keys are pressed at the same time.
//The lowest two digital tubes display the corresponding keys (e.g., if S10 is pressed, 10 is displayed; if S25 is pressed, 25 is displayed)
//FF is displayed when no key is pressed
//The key and key scanning results satisfy the following relationship:
// Key Scan Result (result) Key Scan Result
// K10 0XE7 K18 0XB7
// K11 0XEB K19 0XBB
// K12 0XED K20 0XBD
// K13 0XEE K21 0XBE
// K14 0XD7 K22 0X77
// K15 0XDB K23 0X7B
// K16 0XDD K24 0X7D
// K17 0XDE K25 0X7E
//Hardware requirements: All DIP switches S4 are set to ON
// All DIP switches S6 are set to ON, DIP switch S5 positions 5-6 are ON, and other positions are OFF
// Other DIP switches are set to OFF
#include
__CONFIG(0x1832) internal resources of the MCU;
//Chip configuration word, watchdog off, power-on delay on, power-off detection off, low voltage programming off, encryption, 4M crystal HS oscillation
int result;
void delay(); //delay function declaration
void init(); //I/O port initialization function declaration
void scan(); //Key scanning program declaration
void display(int x); //Display function declaration
//---------------------------------------------------
//Main program
void main()
{
while(1) //Loop work
{
init(); //Call initialization subroutine
scan(); //Call key scanning subroutine
display(result); //Call result display subroutine
}
}
//---------------------------------------------------
//Initialization function
void init()
{
ADCON1=0X07; //Set port A as a normal I/O port
TRISA=0X0f; //Set the high 2 bits of port A as output and the low 4 bits as input
TRISC=0XF0; //Set the high 4 bits of port C as input and the low 4 bits as output
TRISD=0X00; //Set port D as output PORTA
=0XFF;
PORTD=0XFF; //Clear all displays first
}
//---------------------------------------------------
//Key scanning program
void scan()
{
PORTC=0XF7; //C3 outputs low level, and the other three bits output high level
asm("nop"); //Insert a certain delay to ensure that the level is stable
result=PORTC; //Read back the high 4 bits of port C
result=result&0xf0; //Clear the low 4 bits
if(result!=0xf0) //Judge whether the high 4 bits are all 1 (all 1 means no button is pressed)?
{
result=result|0x07; //No, add the lower 4 bits 0x07 as the result of the key scan
}
else //Yes, change the lower 4 bits output and re-judge whether a key is pressed
{
PORTC=0XFb; //C2 outputs low level, and the other three bits output high levelasm
("nop"); //Insert a certain delay to ensure that the level is stableresult
=PORTC; //Read back the high 4 bits of port
Cresult=result&0xf0; //Clear the low 4 bitsif
(result!=0xf0) //Judge whether the high 4 bits are all 1 (all 1 means no key is pressed)
{
result=result|0x0b; //No, add the lower 4 bits 0xb as the result of the key scan
}
else //Yes, change the lower 4 bits output and re-scan
{
PORTC=0XFd; //C1 outputs low level, and the other three bits output high levelasm
("nop"); //Insert a certain delay to ensure that the level is stableresult
=PORTC; //Read back the high 4 bits of port C
result=result&0xf0; //Clear the low 4 bitsif
(result!=0xf0) //Judge whether the high 4 bits are all 1 (all 1 means no button is pressed)
{
result=result|0x0d; //No, add the low 4 bits 0x0d as the result of the key scan
}
else //Yes, change the output of the low 4 bits and rescan
{
PORTC=0XFe; //C0 outputs low level, and the other three bits output high levelasm
("nop"); //Insert a certain delay to ensure that the level is stableresult
=PORTC; //Read back the high 4 bits of port C
result=result&0xf0;//Clear the low 4 bitsif
(result!=0xf0) //Judge whether the high 4 bits are all 1 (all 1 means no button is pressed)
{
result=result|0x0e;//No, add the low 4 bits 0x0e as the result of the key scan
}
else //Yes, all key scans are completed, no key is pressed, set the no key pressed flag
{
result=0xff; //The scan result is 0xff, which is used as a sign that no key is pressed
}
}
}
}
}
//------------------------------------------------ ----------
//Display program
void display(int x)
{
switch(result)
{
case 0xe7:
PORTD=0xf9;PORTA=0X2F;delay();PORTD=0xc0;PORTA=0X1F;delay ();break; //K10
case 0xeb:
PORTD=0xf9;PORTA=0X2F;delay();PORTD=0xf9;PORTA=0X1F;delay();break; //K11
case 0xed:
PORTD=0xf9;PORTA=0X2F ;delay();PORTD=0xa4;PORTA=0X1F;delay();break; //K12
case 0xee:
PORTD=0xf9;PORTA=0X2F;delay();PORTD=0xb0;PORTA=0X1F;delay();break ; //K13
case 0xd7:
PORTD=0xf9;PORTA=0X2F;delay();PORTD=0x99;PORTA=0X1F;delay();break; //K14
case 0xdb:
PORTD=0xf9;PORTA=0X2F;delay();PORTD=0x92;PORTA= 0X1F;delay();break; //K15
case 0xdd:
PORTD=0xf9;PORTA=0X2F;delay();PORTD=0X82;PORTA=0X1F;delay();break; //K16
case 0xde:
PORTD=0xf9; PORTA=0X2F;delay();PORTD=0XF8;PORTA=0X1F;delay();break; //K17
case 0xb7:
PORTD=0xf9;PORTA=0X2F;delay();PORTD=0X80;PORTA=0X1F;delay();break; //K18
case 0xbb:
PORTD=0xf9;PORTA=0X2F;delay();PORTD=0X90;PORTA= 0X1F;delay();break; //K19
case 0xbd:
PORTD=0xa4;PORTA=0X2F;delay();PORTD=0xc0;PORTA=0X1F;delay();break; //K20
case 0xbe:
PORTD=0xa4; PORTA=0X2F;delay();PORTD=0xf9;PORTA=0X1F;delay();break; //K21
case 0x77:
PORTD=0xa4;PORTA=0X2F;delay();PORTD=0xa4;PORTA=0X1F;delay();break; //K22
case 0x7b:
PORTD=0xa4;PORTA=0X2F;delay();PORTD=0xb0;PORTA= 0X1F;delay();break; //K23
case 0x7d:
PORTD=0xa4;PORTA=0X2F;delay();PORTD=0x99;PORTA=0X1F;delay();break; //K24
case 0x7e:
PORTD=0xa4; PORTA=0X2F;delay();PORTD=0x92;PORTA=0X1F;delay();break; //K25
case 0xff:
PORTD=0x8e;PORTA=0X2F;delay(); PORTD=0x8e; PORTA=0X1F; delay(); //No button pressed
}
}
//------------------------------------------------------------------
//Delay program
void delay() //Delay program
{
int i; //Define integer variables
for(i=0x100;i--;); //Delay
}
Previous article:PIC16F877A CCP Usage
Next article:PIC16F877A sleep mode
Recommended ReadingLatest update time:2024-11-16 15:37
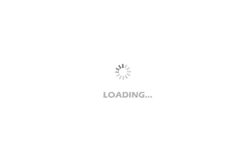
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TI High-Speed Signal Conditioning Product Selection Guide
- STM32L051's ADC conversion is inaccurate after wakeup
- Sharing of experience in single board circuit design 3-selection of pull-up and pull-down resistor parameters
- LVDS Receive
- Create a GD32VF103 project using Visual Studio Code
- Circuit Playground Star Tree
- Principles and Applications of FPGA and Specialized DSP
- nRF52 series is coming, Nordic's low-power Bluetooth solution 52832
- EEWORLD University Hall----Live Replay: Beneng International launches millimeter wave radar module based on Infineon technology, perfectly solving the pain points of PIR market
- [NXP Rapid IoT Review] + Mobile Synchronizer 2