/***************************************************************************************************
Keywords:PIC
Reference address:PIC serial communication program (2) interrupt 1602 echo
*** Function: The serial port debugging assistant sends data to 877, and 877 forwards it back to the serial port assistant after receiving it
*** Experimental content: Send data to 877 through the PC software "Serial Port Debug Assistant" c,
877 sends the received data to the computer
*** Development board connection method: Use a serial cable to connect to the serial port on the microcontroller development board.
The other end is connected to the 9-pin serial port of the computer. If there is no serial port, please purchase a USB to serial cable.
****************************************************************************************************/
#include //Includes the predefined internal resources of the MCU
__CONFIG(0xFF32); //Chip configuration word, watchdog off, power-on delay on, power-off detection off, low voltage programming off, encryption, 4M crystal HS oscillation
#define rs RA5
#define rw RA4
#define and RA3
unsigned char recdata;
void init(); //Declare I/O port initialization function
void lcd_init(); //Declare LCD initialization function
void write(char x); //Declare the function to display 1 byte data
void lcd_enable(); //Declare LCD display setting function
void delay(); //Declare the delay function
void writelcd(char *pt); //Declare LCD write string function
/****************************************************************************
* Name: main()
* Function: Main function
* Entry parameters: None
* Export parameters: None
* Description: None
****************************************************************************/
void main()
{
TRISC=0XFF; //Set the direction of port C to output
SPBRG=0XC; //Set the baud rate to 19200BPS
TXSTA=0X24; //Enable serial port transmission and select high-speed baud rate
RCSTA=0X90; //Enable serial port, continuous reception
RCIE=0X1; //Enable receive interrupt
GIE=0X1; //Open global interrupt
PEIE=0X1; //Enable external interrupt
init(); //Call I/O port initialization function
lcd_init(); //Call LCD initialization function
while(1) //wait for interrupt
{
;
}
}
/****************************************************************************
* Name: usart()
* Function: interrupt function
* Entry parameters:
* Export parameters:
* Note: Note that the keyword interrupt is required. If there are multiple interrupts, in the interrupt service function,
To determine the interrupt source flag
****************************************************************************/
void interrupt usart(void)
{
int i;
if(RCIE&&RCIF) //Judge whether it is a serial port receiving interrupt
{
TXREG=RCREG;
write(TXREG); //Only 3 characters can be displayed, is there a problem? ???
}
}
/****************************************************************************
* Name: init()
* Function: I/O port initialization
****************************************************************************/
void init()
{
ADCON1 = 0X07; //Set port A to a normal I/O port
RA1=1;
TRISA = 0X00; //Set port A to output
TRISD = 0X00; //Set port D to output
}
/****************************************************************************
* Name: lcd_init()
* Function: LCD initialization
****************************************************************************/
void lcd_init()
{
PORTD = 0X1; // Clear display
lcd_enable();
PORTD = 0X38; //8-bit 2-row 5*7 dot matrix
lcd_enable();
PORTD = 0X0e; //Display on, cursor on, blinking
lcd_enable();
PORTD = 0X06; //The text does not move, the cursor moves right
lcd_enable();
PORTD = 0X80; //Company web display address
lcd_enable();
}
/****************************************************************************
* Name: write()
* Function: Write one byte of data to LCD
* Input parameter: char x character
****************************************************************************/
void write(char x)
{
PORTD = x; //Send the data to be displayed to PORTD
rs = 1; //This byte is data, not a command
rw = 0; //This operation is a write, not a read
e = 0; // Pull the enable signal low
delay(); //Keep the enable signal low for a while
e = 1; //Pull up the enable signal to establish the rising edge required for LCD operation
}
/****************************************************************************
* Name: lcd_enable()
* Function: LCD display settings
****************************************************************************/
void lcd_enable()
{
rs = 0; //This byte data is a command, not data
rw = 0; //This operation is a write, not a read
e = 0; // Pull the enable signal low
delay(); //Keep the enable signal low for a while
e = 1; //Pull up the enable signal to establish the rising edge required for LCD operation
}
/****************************************************************************
* Name: delay()
* Function: Delay
****************************************************************************/
void delay()
{
int i;
for (i = 0;i < 5000;i++);
}
Previous article:PIC IIC read and write
Next article:PIC serial communication program (1)
Recommended ReadingLatest update time:2024-11-22 13:03
PIC16-bit microcontroller CAN (8) watchdog
The framework of the program has been completed. After turning on the watchdog, all that remains is to improve the program structure.
According to the documentation, the watchdog is mainly about the configuration bits. Here is my configuration:
_FWDT(WDTPOST_PS4096&WDTPRE_PR32&PLLKEN_OFF&WINDIS_OFF&FWDTEN_ON);
/
[Microcontroller]
Research on Adaptive Control Technology of Stepping Motor Based on PIC Microcontroller
1 Introduction The stepper motor is a discrete motion device, which has a close essential connection with modern digital control technology. The stepper motor is also an actuator that converts electrical pulses into angular displacement or linear displacement. When the stepper motor driver receives a pulse signal, it
[Microcontroller]
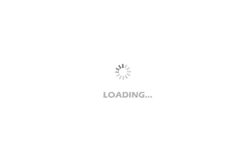
Introduction to 16-bit MCU technology designed with PIC24FJ256DA210
PIC24FJ256DA210 is a 16-bit
MCU with a graphics controller (GFX) module that can interface with LCD displays and up to 96KB of data RAM. The PIC24FJ256DA210 high-performance CPU uses a modified Harvard architecture, with performance up to 16MIPS at 32MHz, an internal oscillator of 8MHz, a 17-bit x 17-bit sin
[Microcontroller]
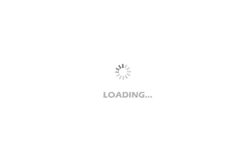
Intelligent humidity measuring instrument circuit based on PIC microcontroller
The circuit of the intelligent humidity meter composed of HM1500/1520 humidity sensor and single chip is shown in the figure below. The meter adopts +5V power supply and is equipped with 4 common cathode LED digital tubes. There are 3 ICs in total in the circuit: IC1 is HM1500/1520 humidity sensor, IC2 is PIC16F874, a
[Microcontroller]
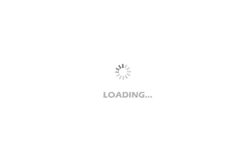
Programming of PIC microcontroller port RB interrupt
In this example, a modular programming method is used, and the program planning, writing, assembling, debugging, modifying,
The program flow is shown in Figures 1 to 7.
Figure 1 Main program flow
Figure 2 Interrupt service subroutine flow
Figure 3 Low-pronunciation subroutine flow
Fi
[Microcontroller]
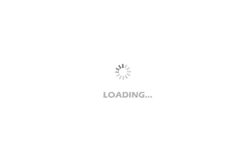
Design of External Oscillator Circuit for PIC Microcontroller
Crystal oscillator design is one of the important links in microcontroller application design, so it is necessary to understand the characteristics and composition of the crystal oscillator circuit and how to select related electronic components.
The PIC microcontroller has four oscillation modes to choose from. The
[Microcontroller]
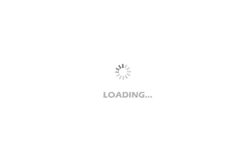
PIC internal EEPROM read and write
Steps to read data from EEPROM:
1. Write the address to the address register EEADR. Note that the address cannot exceed the actual capacity of the internal EEPROM of the PIC1687X microcontroller.
2. Clear the control bit EEPGD to select the EEPROM data memory as the read object.
3. Set the control bit RD to 1 to sta
[Microcontroller]
LCD driver HT1621 PIC microcontroller source code
STATUS EQU 3H
FSR EQU 4H
RB EQU 6H
RC EQU 7H
OPTIONA EQU 81H
TRISB EQU 86H
TRISC EQU 87H
GENR0 EQU 053H
GENR1 EQU 054H
WD_RG EQU 055H
DA_AG0 EQU 056H ;SEG0 SEG1
DA_AG8 EQU 05EH ;SEG16 SEG17
;.............................
C
[Microcontroller]
- Popular Resources
- Popular amplifiers
-
Principles and Applications of Single Chip Microcomputers 3rd Edition (Zhang Yigang)
-
Power Integrated Circuit Technology Theory and Design (Wen Jincai, Chen Keming, Hong Hui, Han Yan)
-
Small Compiler Design Practice (Compiled by Su Mengjin)
-
STM8 C language programming (1) - basic program and startup code analysis
Recommended Content
Latest Microcontroller Articles
- Naxin Micro and Xinxian jointly launched the NS800RT series of real-time control MCUs
- How to learn embedded systems based on ARM platform
- Summary of jffs2_scan_eraseblock issues
- Application of SPCOMM Control in Serial Communication of Delphi7.0
- Using TComm component to realize serial communication in Delphi environment
- Bar chart code for embedded development practices
- Embedded Development Learning (10)
- Embedded Development Learning (8)
- Embedded Development Learning (6)
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Intel promotes AI with multi-dimensional efforts in technology, application, and ecology
- ChinaJoy Qualcomm Snapdragon Theme Pavilion takes you to experience the new changes in digital entertainment in the 5G era
- Infineon's latest generation IGBT technology platform enables precise control of speed and position
- Two test methods for LED lighting life
- Don't Let Lightning Induced Surges Scare You
- Application of brushless motor controller ML4425/4426
- Easy identification of LED power supply quality
- World's first integrated photovoltaic solar system completed in Israel
- Sliding window mean filter for avr microcontroller AD conversion
- What does call mean in the detailed explanation of ABB robot programming instructions?
MoreDaily News
- Infineon Technologies Launches ModusToolbox™ Motor Kit to Simplify Motor Control Development
- Infineon Technologies Launches ModusToolbox™ Motor Kit to Simplify Motor Control Development
- STMicroelectronics IO-Link Actuator Board Brings Turnkey Reference Design to Industrial Monitoring and Equipment Manufacturers
- Melexis uses coreless technology to reduce the size of current sensing devices
- Melexis uses coreless technology to reduce the size of current sensing devices
- Vicor high-performance power modules enable the development of low-altitude avionics and EVTOL
- Chuangshi Technology's first appearance at electronica 2024: accelerating the overseas expansion of domestic distributors
- Chuangshi Technology's first appearance at electronica 2024: accelerating the overseas expansion of domestic distributors
- "Cross-chip" quantum entanglement helps build more powerful quantum computing capabilities
- Ultrasound patch can continuously and noninvasively monitor blood pressure
Guess you like
- EEWORLD University Hall----TI DLP? Technology Innovation and New Applications in Automobiles
- EEWORLD University Hall--Introduction to Digital Filter Design
- Seeking antenna designer/technical assistant
- EEWORLD University ---- TPS65086100: User Programming of Multi-Rail Power Management IC
- Introduction to Common Current Sensors for Electric Vehicle BMS
- Internal Hardware Resources of PIC Microcontroller 16F84 (V)
- A new absolute photoelectric encoder suitable for servo applications
- Memory modules in 103 devices
- RG Roller Guide Rails
- Family Pet Health Maintenance System