After using PLL, the CPU running speed is greatly increased, but the power consumption will also increase significantly. Therefore, the use of PLL should be limited in low-power applications.
Main function:
#include "LED.H"
#include
#include
#include
#include
#include
#include
// Define longer identifiers as shorter forms
#define SysCtlPeriEnable SysCtlPeripheralEnable
#define SysCtlPeriDisable SysCtlPeripheralDisable
#define GPIOPinTypeIn GPIOPinTypeGPIOInput
#define GPIOPinTypeOut GPIOPinTypeGPIOOutput
// 定义KEY
#define KEY_PERIPH SYSCTL_PERIPH_GPIOG
#define KEY_PORT GPIO_PORTG_BASE
#define KEY_PIN GPIO_PIN_5
// Prevent JTAG from failing
void JTAG_Wait(void)
{
SysCtlPeriEnable(KEY_PERIPH); // Enable the GPIO port where KEY is located
GPIOPinTypeIn(KEY_PORT, KEY_PIN); // Set the pin where KEY is located to input
if ( GPIOPinRead(KEY_PORT , KEY_PIN) == 0x00 ) // If KEY is pressed during reset, enter
{
for (;;); // Infinite loop to wait for JTAG connection
}
SysCtlPeriDisable(KEY_PERIPH); // Disable the GPIO port where KEY is located
}
// Define the global system clock variable
unsigned long TheSysClock = 12000000UL;
// 延时
void Delay(unsigned long ulVal)
{
while ( --ulVal != 0 );
}
// System initialization
void SystemInit(void)
{
SysCtlLDOSet(SYSCTL_LDO_2_75V); // Set the LDO voltage to 2.75V before configuring PLL
SysCtlClockSet(SYSCTL_USE_PLL | // System clock setting, using PLL
SYSCTL_OSC_MAIN | // Main oscillator
SYSCTL_XTAL_6MHZ | // External 6MHz crystal oscillator
SYSCTL_SYSDIV_4); // The frequency division result is 50MHz
TheSysClock = SysCtlClockGet(); // Get the system clock, unit: Hz
LED_Init(LED2 | LED3); //LED initialization
}
int main(void)
{
JTAG_Wait(); // Prevent JTAG failure, important!
SystemInit(); // System initialization
LED_On(LED2); // Turn on LED2
LED_Off(LED3); // Turn off LED3
for (;;)
{
LED_Toggle(LED2 | LED3);
Delay(150 * (TheSysClock / 4000)); // Delay about 150ms
}
}
Previous article:LM3S1138 Getting Started 8, Soft Reset
Next article:LM3S1138 Getting Started 6, System Clock Settings
Recommended ReadingLatest update time:2024-11-17 00:07
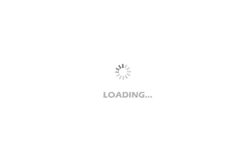
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- 【GD32L233C-START Review】-V. GD32 transplants atomic serial port debugging components to implement serial port function debugging
- 555 classic circuit diagram: 555 simple delay circuit diagram
- [Sipeed LicheeRV 86 Panel Review] 7. fbviewer and ts_test command test
- HyperLynx High-Speed Circuit Design and Simulation (III) Termination Resistance Experiment
- TUSB9261 -- USB3.0 to SATA interface bridge chip programming guide
- How about the Qinheng RISC-V core CH32V103 microcontroller?
- Transformer Isolation Switch
- Dual Limit Comparator (Window Comparator) Circuit
- If you urinate on a 380V high voltage wire (live electricity), will you be electrocuted? Will urine become a conductor and carry electricity, giving you an electric shock?
- Please help me find the schematic diagram, thank you very much