PIC's instruction system
PIC 8-bit microcontrollers have three levels, with corresponding instruction sets. The basic PIC series chip has 33 instructions, each of which is 12 bits long; the intermediate PIC series chip has 35 instructions, each of which is 14 bits long; the advanced PIC series chip has 58 instructions, each of which is 16 bits long. Its instructions are backward compatible.
1. PIC assembly language instruction format The
assembly language instructions of PIC series microcontrollers are the same as those of MCS-51 series single-chip microcomputers. Each assembly language instruction consists of 4 parts, and its writing format is as follows:
label opcode mnemonic operand 1, operand 2; comment
The instruction format is as follows: The 4 parts of the instruction are separated by spaces, which can be 1 or more spaces to ensure that the PC can recognize the instruction during cross-assembly.
1. The label has the same function as the MCS-51 series single-chip microcomputer. The label represents the symbolic address of the instruction. When the program is assembled, the specific value of the instruction memory address has been assigned. The use of symbolic addresses (i.e. labels) in assembly language is convenient for viewing and modification, especially for the representation of instruction transfer addresses. Labels are optional in the instruction format and are only required when they are referenced by other statements. In the absence of labels, one or more spaces must be reserved before the instruction mnemonic before writing the instruction mnemonic. The instruction mnemonic cannot occupy the position of the label, otherwise the mnemonic will be mishandled as a label by the assembler.
When writing a label, the first character must be a letter or a half-width underscore "—", which can be followed by English and numeric characters, colons (:), symbols, etc., and can be combined arbitrarily. In addition, labels cannot be represented by opcode mnemonics and register codes. Labels can also occupy a single line.
2. The opcode mnemonic field is a required option for instructions. This item can be an instruction mnemonic, or it can be composed of pseudo-instructions and macro commands. Its function is to compare the "instruction opcode mnemonics" with the "opcode table" one by one during cross-assembly, and find out their corresponding machine codes one by one.
3. The operand consists of the data value of the operand or the data or address value represented by symbols. If there are two operands, the two operands are separated by a comma (,). When the operand is a constant, the constant can be binary, octal, decimal or hexadecimal. It can also be a defined label, string and ASCII code. When specifically expressed, it is stipulated that the letter "B" is prefixed before the binary number, such as B10011100; the letter "O" is prefixed before the octal number, such as O257; the letter "D" is prefixed before the decimal number, such as D122; and the letter "H" is prefixed before the hexadecimal number, such as H2F. Here, the default system of the PIC 8-bit microcontroller is hexadecimal. Add Ox before the hexadecimal number, such as H2F can be written as Ox2F.
The operand item of the instruction is also optional.
The PIC series, like the MCS-51 series 8-bit microcontroller, has an addressing method, that is, the source or destination of the operand. Because the PIC series microcontroller adopts the reduced instruction set (RISC) structure system, its addressing mode and instructions are both few and simple. Its addressing mode can be divided into four types according to the source of the operand: immediate addressing, direct addressing, register indirect addressing and bit addressing. Therefore, the operands in the instructions of the PIC series microcontroller often have relevant register symbols. Relevant addressing examples can be found later in this article.
4. Comments are used to explain the program and make it easier for people to read the program. Use a semicolon (;) to separate the comments from other parts. When the assembler detects a semicolon, the characters after it will no longer be processed. It is worth noting that when using a subroutine, the entry and exit conditions of the program and the functions and effects that the program should complete should be stated.
II. Clear instructions (4 in total)
1. Register clear instruction
Example: CLRW; register W is cleared
Description: This instruction is very simple, where W is the working register of the PIC microcontroller, equivalent to the accumulator A in the MCS-51 series microcontroller, and CLR is the abbreviation of Clear in English.
2. Watchdog timer clear instruction.
Example: CLRWDT; watchdog timer clears (if assigned, clear the prescaler at the same time)
Description: WDT is the abbreviation of Watchdog Timer in English. CLR sees the above description. Note that these two instructions have no operands.
3. Register f clear instruction. Instruction format: CLRF f
Example: CLRF TMRO; clear TMRO
Description: In the PIC series 8-bit microcontrollers, the symbol F (or f) is often used to represent various registers and the serial addresses of F in the chip. The value of F varies according to different models of the PIC series, generally Ox00~Ox1F/7F/FF. TMRO stands for timer/counter TMRO, so CLRF clears the register and uses direct addressing to directly give the register TMRO to be accessed.
4. Bit clear instruction. Instruction format BCF f, b
Example: BCF REG1, 2; clear the D2 bit of register REG1
Description: BCF is the abbreviation of English Bit Clear F. The F in the instruction format is the same as above; the symbol b represents the bit number (or bit address) of a certain 8-bit data register F in the PIC chip, so the value of b is 0~7 or D0~D7. In the example, REG is the abbreviation of Register. The 2 in the example represents the b=2 in the instruction format, i.e., the D2 bit of register REG1.
Through the above four clear instruction formats and examples, it can be explained that when learning the instructions of the PIC series 8-bit microcontroller, one should first understand the mnemonic meaning (function) of the instruction, and then its expression method. Beginners do not need to memorize the instructions, the important thing is to understand and practice.
III. Instructions for bytes, constants and control operations
1. Transfer immediate value to working register W instruction
Instruction format: MOVLW k; k represents constant, immediate value and label
Description: MOVLW is the abbreviation of Move Literal to w
Example: MOVL 0x1E; constant 30 is sent to W
2. I/O port control register TRIS setting instruction
Instruction format; TRIS f
Description; TRIS f is the abbreviation of Load TRIS Register. Its function is to send the content of working register W to I/O port control register f. When W=0, set the corresponding I/O port as output; W=1, set the I/O port as input.
Example: MOVLW 0x00; send 00H to W
TRIS RA; set PIC RA port as output
MOVLW 0xFF; send FFH to W
TRIS RB; set PIC RB port as input
Description: These are some commonly used instructions in PIC assembly language, that is, statements that set a certain I/O port (here RA port and RB port) as input or output. It can be seen that when reading instructions, one should fully understand the function of the statement format and read it in conjunction with each other.
3. Instruction to send the content of W register to register f (the content of W remains unchanged) Instruction
format: MOVWF f
Description: MOVWF is the abbreviation of Move W to f
Example: MOVLW 0x0B; send 0BH to W
MOVWF 6; send the content of W to RB port
Description: The first instruction 0x0B (constant 11) is sent to the working register W, and the second instruction sends the constant 11 of the content of W to register F6. Looking up the table, F6 is RB port, so PORT_B (B port) = 0BH = D11
4. Register f transfer instruction
Instruction format: MOVF f, d
Description: MOVF is the abbreviation of Move f. F represents a register in PIC. The d in the instruction stipulates: when d=0, the content of f is sent to W; when d=1, the content of f is sent to the register.
Example: MOVF 6,0; the content of port RB is sent to W
MOVWF 8; the content of port RB is sent to f8
Explanation: The 6 in the first instruction represents register f=6. Looking up the register table, f=6 is port RB; 0 represents d=0, which means the selected target is register W. The 8 in the second instruction represents register f=8. So the result of the two instructions is to send the content of port RB to f8. As for the content of f8, it should be added at the beginning of the assembly language, which is omitted here.
5. No operation instruction
Instruction format: NOP
Explanation: NOP is the abbreviation of No Operation in English. NOP has no operand, so it is called a no operation. Executing the NOP instruction only increases the program counter PC by 1, so it takes one machine cycle.
Example: MOVLW 0xOF; send OFH to W
MOVWF PORT_B; write W content to port B
NOP; no operation
MOVF PORT_B, W; read operation
Description: These three instructions are an example of continuous operation on port B of I/O port. The purpose is to ensure that there is a stable time between writing and reading when the content written to port B is to be read out, so the no operation instruction NOP is added.
6. Unconditional jump instruction
Instruction format: GOTO k
Description: When executing this instruction, the instruction is transferred to the specified address (jump). The k in the instruction is often associated with the label in the program. Example: See the jump instruction of 7. Register
content minus 1 in instruction 9 , the result is zero Instruction format: DECFSZ f, d Description: DECFSZ is the abbreviation of Decrement f, Skip of not 0 in English. The meaning of the symbols f and d has been explained above. This instruction means to decrement the contents of the register by 1 and store it in W (d=0) or f (d=1). If the result of the instruction execution is not zero, the instructions are executed in sequence; if it is zero, the next instruction is skipped and then executed (equivalent to executing a null instruction NOP in sequence). In actual instructions, when d=1, this item is often omitted. 8. Register contents plus 1, skip instruction if the result is zero Instruction format: INCFSZ f, d Description: INCFSZ is the abbreviation of Increment f, Skip of 0 in English. The only difference between this instruction and the previous instruction (7) is the "1", that is, when executing this instruction, the contents of register f are incremented by 1. If the result is not zero, the instructions are executed in sequence; if it is zero, the instructions are skipped. The other logical relationships of executing this instruction are the same as the previous one. 9. Subroutine return instruction Instruction format: RETLW k Description: RETLW is the abbreviation of Return Literal to W. This instruction represents the return of the subroutine. Before returning, the 8-bit immediate value is sent to W.
Previous article:PIC16F97+eV1527 decoding source program
Next article:PIC Getting Started 3, SPI Communication and Serial Port Debugging Experiment
Recommended ReadingLatest update time:2024-11-16 12:27
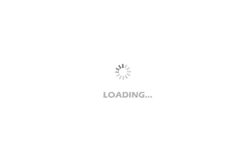
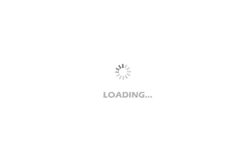
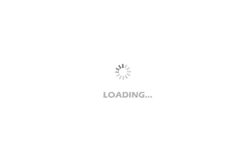
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How is the storage period of components calculated?
- The Definitive Guide to FPGA Rapid System Prototyping (Chinese)
- Temperature problems solved for you (IV) Ambient temperature monitoring
- LGA-12 package soldering issues
- [NXP Rapid IoT Review] +3.rapid-iot-studio example bin summary
- GD32E231 Learning 4: External Interrupt EXTI
- The smallest Bluetooth module in the industry? Now available
- Questions about I2C communication
- Bullshit, playing with a 4.2-inch e-ink screen price tag
- ZigBee (CC2530, ZSTACK) transparent transmission example