1. Interface within the module:
Use the following identifiers:
#pragma asm
Assembly Statements
#pragma endasm
Note: If you use assembly language in your C51 program, be aware that you need to activate the "Generate Assembler SRC File" and "Assembler SRC File" options in the Properties of the Keil compiler.
Let's take an example:
#i nclude
void main(void)
{
P2=1;
#pragma asm
MOV R7,#10
DEL:MOV R6,#20
DJNZ R6,$
DJNZ R7,DEL
#pragma endasm
P2=0;
}
Other:
1. Add "xx.c" to the project, right-click "xx.c" and select "options for file"xx.c" Select "Generate Assembler SRC File" and "Assemble SRC File" and check the black box to make it effective;
2. According to the selected compilation mode, add the corresponding library file to the project like adding "xx.c" and place it under "xx.c". For example, in smail mode, select "keilc51libc51s.lib" to add it to the project. If floating-point operations are to be performed, also add "keilc51libc51fpl.lib" to the project.
The LIB files in the C51LIB directory under the Keil installation directory are as follows:
C51S.LIB - Small model without floating point operations
C51C.LIB - Compact model without floating point operations
C51L.LIB - Large model without floating point operations
C51FPS.LIB - Small model with floating point operations
C51FPC.LIB - Compact model with floating point operations
C51FPL.LIB - Large model with floating point operations
3. Add optimization to the "xx.c" header file: for example, #pragma OT(4,speed)
4. Add assembly code to "xx.c"
#pragma ASM
;Assembler Code Here
#pragma ENDASM
5. Compile and generate xx.hex
Notice:
If you do not do the first step, you will get the following warning: 'asm/endasm' requires src-control to be active
If you do not do the second step, the following warning will appear: UNRESOLVED EXTERNAL SYMBOL;
REFERENCE MADE TO UNRESOLVED EXTERNAL等
If you do not do step 3, the following warning will appear: UNDEFINED SYMBOL (PASS-2)
2. Interrupt Usage
interrupt xx using y
The xx value after interrupt is the interrupt number, which means the interrupt port number to which this function corresponds. It is usually in 51.
0 External interrupt 0
1 Timer 0
2 External interrupt 1
3 Timer 1
4 Serial interrupt
The others have their own meanings according to the corresponding microcontroller. In fact, when C is compiled, the entry address of your function is placed in the jump address of the corresponding interrupt. Using y means that the register group used by this interrupt function is generally 4 registers r0-r7 in 51. If your terminal function and other programs do not use the same register group, the register group will not be pushed into the stack when entering the interrupt, and will not be popped out when returning, saving code and time.
3. How to use reentrant
The following warning appeared in the program:
*** WARNING L15: MULTIPLE CALL TO SEGMENT
SEGMENT: ?PR?_CRCDATA?PANEL_DISP
CALLER1: ?C_C51STARTUP
CALLER2: ?PR?UART_RECV?PANEL_DISP
*** WARNING L15: MULTIPLE CALL TO SEGMENT
SEGMENT: ?PR?ANALOGALLBECKON?PANEL_DISP
CALLER1: ?C_C51STARTUP
CALLER2: ?PR?UART_RECV?PANEL_DISP
*** WARNING L15: MULTIPLE CALL TO SEGMENT
SEGMENT: ?PR?SWITCHALLBECKON?PANEL_DISP
CALLER1: ?C_C51STARTUP
CALLER2: ?PR?UART_RECV?PANEL_DISP
My program compiled with these 3 warnings, but the program can be downloaded and run normally. But I think these warnings will cause bugs in the program. Literally, it means that the receiving function UART_RECV() in my program calls analogAllBeckon() and switchAllBeckon() multiple times.
Because the ordinary functions of 51 are not reentrant, the variables are placed at fixed addresses. When two functions are run at the same time, the same variable will be modified, resulting in wrong results. So I added void analogAllBeckon()reentrant{//All Analog data beckon after analogAllBeckon() and switchAllBeckon() functions to eliminate the warning in the program. This method shows that the function can be called by multiple tasks without modifying the variable value in the function, so as to achieve the reentrant nature of the function.
There is a detailed discussion on the use of reentrant on the Keil official forum.
Andy Neil (official engineer) suggested
"Are you sure that you really need to make everything reentrant?...A reading of the Keil app notes & knowledgebase articles on this subject showed that it was not necessary. "
Since each time a function declared by reentrant is called, the function parameters and internal variables must be pushed onto the stack, it is easy to cause the stack to overflow. S52 only has a 256-byte data segment. If a simple function has one parameter and three internal variables, more than 4 bytes need to be pushed onto the stack, not including the function call stack. Reentrant is not actually suitable for low-end microcontrollers. Someone on the Keil forum said that reentrant is only suitable for microcontrollers with more than KB of RAM.
4. Variable declaration
The difference between data, idata, xdata, and pdata in the 51 series data: fixedly refers to the first 128 RAMs from 0x00 to 0x7f, which can be directly read and written with acc, with the fastest speed and the smallest generated code. idata: fixedly refers to the first 256 RAMs from 0x00 to 0xff, of which the first 128 are exactly the same as the 128 of data, but the access method is different. idata is accessed in a pointer-like manner similar to that in C. The statement in the assembly is: mox ACC, @Rx. (Unimportant supplement: pointer-style access of idata in C works very well) xdata: external extended RAM, generally refers to the external 0x0000-0xffff space, accessed with DPTR. pdata: the lower 256 bytes of external extended RAM, read and written when the address appears on A0-A7, read and write with movx ACC, @Rx. This is quite special, and C51 seems to have a bug for this, so it is recommended to use it less. But it also has its advantages, and the specific usage belongs to the intermediate problem, so it is not mentioned here.
The role of startup.a51 is the same as that of assembly. The variables and arrays defined in C are initialized in startup.a51. If you define a global variable with a value, such as unsigned char data xxx="100";, then startup.a51 will have a related assignment. If there is no =100, startup.a51 will clear it to 0. (startup.a51==variable initialization). After these initializations are completed, the SP pointer will also be set. There will be no assignment or clearing action for non-variable areas, such as the stack area. Some people like to modify startup.a51 to satisfy their own hobbies. This is unnecessary and may be wrong. For example, when power-off protection is applied, you want to save some variables, but modifying startup.a51 to achieve this is a stupid method. In fact, you can just use the characteristics of the non-variable area and define a pointer variable to point to the lower part of the stack: 0xff. Why do you still need to modify it? It can be said that you don't need to modify startup.a51 at any time if you understand its characteristics.
5. Type-related
A variable can be defined using bit, but not using sbit. sbit can define a port.
Previous article:Choosing the Right 8-bit MCU Communication Interface for IoT Applications
Next article:Design of Intelligent Level Converter Based on Single Chip Microcomputer
Recommended ReadingLatest update time:2024-11-16 07:49
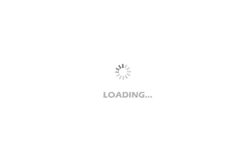
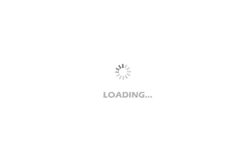
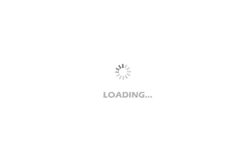
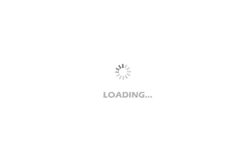
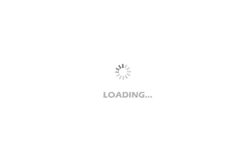
- Popular Resources
- Popular amplifiers
-
Wireless Sensor Network Technology and Applications (Edited by Mou Si, Yin Hong, and Su Xing)
-
Modern Electronic Technology Training Course (Edited by Yao Youfeng)
-
Modern arc welding power supply and its control
-
Small AC Servo Motor Control Circuit Design (by Masaru Ishijima; translated by Xue Liang and Zhu Jianjun, by Masaru Ishijima, Xue Liang, and Zhu Jianjun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Power amplifier application--piezoelectric ceramic impedance test, Antai Electronics Manufacturer
- Indiemicro iND86201 Ernie, who has used it? ---http://www.indiemicro.com/cn/
- Detailed explanation of the technical architecture of the four major mainstream CPU processors
- Porting Ubuntu to A7
- Ground interference problem? (Attached circuit diagram, powered by USB 5V 1A)
- Causes of blistering on PCB copper plating board
- Power supply obstacles + MOSFET switching losses
- GD32 unboxing photos
- IMX6 Linux system compilation manual
- 【Perf-V Evaluation】+ Construction and basic use of development environment (1)