1. SysTick
Keywords:STM32
Reference address:Application of SysTick in STM32 3.5 firmware library (1)
There is a system timer in the STM32 core, which is a 24-bit down counter. The working principle is that after the system time base timer is set to the initial value and enabled, the count value decreases every time a system clock cycle passes. When the count value decreases to 0, the system timer will automatically reload the initial value and continue the next count. At the same time, the internal COUNTFLAG flag will be set. Trigger an interrupt. In the early firmware library, many functions were provided to set SysTick, but in the 3.5 version of the standard firmware library, the relevant driver functions were removed, and users had to call the functions defined by CMSIS. CMSIS only provided a Systick setting function, which replaced all the original driver functions of STM32. The purpose of doing this may be to simplify the Systick setting, but it reduces the user's controllability of SysTick. The function provided in CMSIS is SysTick_Config(uint32_t ticks); This function sets the value of the automatic reload counter (LOAD), the priority of the SysTick IRQ, resets the value of the counter (VAL), starts counting and turns on the SysTick IRQ interrupt. The SysTick clock uses the system clock by default. This function is defined in Core_cm3.h, and the source code is as follows: static __INLINE uint32_t SysTick_Config(uint32_t ticks) { if (ticks > SysTick_LOAD_RELOAD_Msk) return (1); SysTick->LOAD = (ticks & SysTick_LOAD_RELOAD_Msk) - 1; /* Set initial value*/ NVIC_SetPriority (SysTick_IRQn, (1<<__NVIC_PRIO_BITS) - 1); /* Set interrupt priority */ SysTick->VAL = 0; /* Load the SysTick Counter Value */ SysTick->CTRL = SysTick_CTRL_CLKSOURCE_Msk | SysTick_CTRL_TICKINT_Msk | SysTick_CTRL_ENABLE_Msk; /* Enable Systick interrupt and Systick timer*/ return (0);
} As can be seen from the above function, this function completes the initial value of Systick, interrupt priority, enabling interrupts, and starting the timer, which greatly simplifies the program. The ticks represent the initial value. For example, if the system clock is 72Mhz, then to generate a 1ms time base, we can write it like this. SysTick_Config(SystemCoreClock/1000); Of course, it can also be written as: SysTick_Config(72000); Knowing this, we can use it to make a simple delay function delay_ms(u16 time); code show as below: void delay_ms(u16 time) { nTime=time; /nTime is a global variable and can be set as extern u16 nTime;/ while(nTime); } Add nTime directly to the interrupt function--; Add SysTick_Config(72000) during the main function initialization process;
2. Using library functions and interrupts to achieve delay
/**Under Systick control, PB.5 flashes every 1ms SysTick is configured to interrupt once every 1ms. */ /***Main function***/ #include "stm32f10x.h" __IO uint32_t TimingDelay; void GPIO_Configuration(void); void Delay(__IO uint32_t nTime); int main(void) { GPIO_Configuration(); while (SysTick_Config(72000)); /* Set SysTick timer to generate 1ms interrupt */ //The set value is less than 0x00FFFFFF and the execution continues... while (1) { GPIO_SetBits(GPIOB, GPIO_Pin_5); Delay(1); //Delay 1ms GPIO_ResetBits(GPIOB, GPIO_Pin_5); Delay(1); //Delay 1ms } } void GPIO_Configuration(void) { GPIO_InitTypeDef GPIO_InitStructure; RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE); GPIO_InitStructure.GPIO_Pin = GPIO_Pin_5; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init(GPIOB, &GPIO_InitStructure); } /* Function: Delay nTime ms. */ void Delay(__IO uint32_t nTime) { TimingDelay = nTime; while(TimingDelay); } /***stm32f10x_it.c***/ #include "stm32f10x_it.h" extern __IO uint32_t TimingDelay; void SysTick_Handler(void) { TimingDelay--; }
Previous article:STM32 port clock
Next article:How do structures in STM32 organize similar registers?
Recommended ReadingLatest update time:2024-11-16 12:55
STM32 Program for TLC2543 and TLV5614
Use the two hardware SPIs of STM32 to complete data reading and writing. For details, see the program comments 1 /****************************(C) COPYRIGHT SunHao 2011****************** ************* 2 Name: ADDA.c 3 Function: ADDA related configuration and reading function 6 Version: 1.0 7 Note: Refer to
[Microcontroller]
Simple analysis of STM32 startup file
Simple analysis of STM32 startup file (applicable scope of STM32F10x.s) Timer, model, name in STM32 incomplete manual , all our routines use a startup file called STM32F10x.s, which defines the stack size of STM32 and the names of various interrupts and entry function names, as well as startup-related assembly code.
[Microcontroller]
USB interface microcontroller program design of stm32 based on keil C mdk development environment
First, let's take a look at the working process of USB. When a USB device is connected to the host, the host begins to enumerate the USB device and sends instructions to the USB device to obtain relevant description information of the USB device, including device description (device descriptor ), configuration des
[Microcontroller]
32. FATFS experiment explanation
1. Brief Review 2. FATFS migration steps The most important thing is to configure ffconf.h and write diskio.c, and modify the 6 functions in diskio.c. 3. FATFS development functions After the porting is completed, you can call the API provided by the file system. After all operations are comp
[Microcontroller]
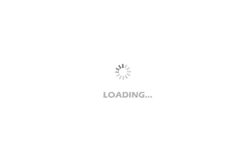
stm32 debugging, enter HardFault_Handler
1. Phenomenon: After entering debugging, the program either enters void HardFault_Handler(void) or void MemManage_Handler(void). 2. Reasons: cstack overflowed. Not enough heap. 3. Modification: stm32f10x_startup.s Stack_Size EQU 0x0001000 AREA STACK, NOINIT, READWRITE, ALIGN=3 S
[Microcontroller]
STM32 example of LED light flashing control and related precautions
In this example, the main purpose is to achieve the flashing of the LED light. First, analyze the driving method of the LED. In this experiment, OpenM3V is used, and the built-in 8 LEDs are all driven by perfusion (low level is bright). If you want to achieve flashing, you need to give the corresponding port a continu
[Microcontroller]
STM32 serial port DMA timeout reception method can greatly save CPU time
This method uses a timer to periodically query the data received by DMA. If it exceeds the set period, it is considered that the data packet has ended, and the data is copied to the buffer and handed over to other programs for processing. It can receive data packets of any size, especially suitable for protocols such a
[Microcontroller]
Brief description of STM32 boot process
Conclusions based on startup mode analysis: 1. The interrupt vector table can be located in the SRAM area through the boot pin setting, that is, the starting address is 0x02000000, and the PC pointer is located at 0x02000000 after reset; 2. The interrupt vector table can be located in the FLASH area through the boot p
[Microcontroller]
- Popular Resources
- Popular amplifiers
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
Guess you like
- Selection of chip beads and chip inductors
- How to put IAP at non-flash memory start address for Ateli AT32F403 microcontroller?
- 【Share】Choice of flyback chip
- Replace the foreign 2SK2837 field effect tube 500V, 24A model in AC-DC switching power supply!
- Program to control ad7708 with MSP430
- Fundamentals of RF/Microwave Switch Test System Design
- Bluetooth is down: BIAS attacks threaten all mainstream Bluetooth chips
- Analog signal isolation GP9303+GP8101 solution
- A heating unit power supply solution with constant and adjustable output power based on TPS61022
- Who remembers a video about the principles of network communication?