#include
#define OP_READ 0xa1 //Device address and read operation, 0xa1 is 1010 0001B
#define OP_WRITE 0xa0 //Device address and write operation, 0xa1 is 1010 0000B
sbit SDA=P3^4; //Define the serial data bus SDA bit as the P3.4 pin
sbit SCL=P3^3; //Define the serial clock bus SDA bit as the P3.3 pin
//Function: Delay 1ms (3j+2)*i=(3×33+2)×10=1010 (microseconds), which can be considered as 1 millisecond
void delay1ms()
{
unsigned char i,j;
for(i=0;i<10;i++)
for(j=0;j<33;j++)
;
}
//Function: delay for several milliseconds
void delaynms(unsigned char n)
{
unsigned char i;
for(i=0;i
}
//Function: start data transmission
void start()
{
SDA = 1; //SDA is initialized to high level "1"
SCL = 1; //When starting data transmission, SCL is required to be high level "1"
_nop_(); //Wait for one machine cycle
_nop_(
); //Wait for one machine cycle
_nop_(); //Wait for one machine cycle
SDA = 0; //The falling edge of SDA is considered to be the start signal
_nop_(); //Wait for one machine cycle
_nop_
(); //Wait for one machine cycle _nop_
(); //Wait for one machine cycle
SCL = 0; //When SCL is low, the data on SDA is allowed to change (that is, subsequent data transmission is allowed)
}
//Function: End data transmission
void stop()
// Stop bit
{
SDA = 0; //SDA is initialized to low level "0" _n
SCL = 1; //When ending data transmission, SCL is required to be high level "1"
_nop_(); //Wait for a machine cycle_nop_
(); //Wait for a machine cycle_nop_
(); //Wait for a machine cycle_nop_
(); //Wait for a machine cycleSDA
= 1; //The rising edge of SDA is considered the end signal_nop_
(); //Wait for a machine cycle_nop_
(); //Wait for a machine cycle_nop_
(); //Wait for a machine cycle_nop_
(); //Wait for a machine cycleSDA
=0;
SCL=0;
}
//Function: Read a byte from AT24Cxx
unsigned char ReadData()
// Move data from AT24Cxx to MCU
{
unsigned char i;
unsigned char x; //Store data read from AT24Cxx
for(i = 0; i < 8; i++)
{
SCL = 1; //Set SCL to high level
x<<=1; //Shift each binary bit in x one position to the left
x|=(unsigned char)SDA; //Store the data on SDA into x through bitwise "OR" operation
SCL = 0; //Read data on the falling edge of SCL
}
return(x); //Return the read data
}
//Answer check
bit ack()
{
bit ack_bit;
SDA = 1; // The sending device (host) should release the SDA line during the high level period of the clock pulse (SCL=1),
//so that the SDA line can be controlled by the receiving device (AT24Cxx)
_nop_(); //Wait for a machine cycle
_nop_(); //Wait for a machine cycle
SCL = 1; //According to the above regulations, SCL should be high
_nop_(); //Wait for a machine cycle _nop_(); //Wait for a
machine cycle _nop_
(); //Wait for a machine cycle
_nop_(); //Wait for a machine cycle
ack_bit = SDA; //The receiving device (AT24Cxx) sends a low level to SDA, indicating that a byte has been received.
//If a high level is sent, it means that it has not been received and the transmission is abnormal
SCL = 0; //When SCL is low, the data on SDA is allowed to change (that is, subsequent data transmission is allowed)
return ack_bit;
}
//Write a byte
//Before calling this data write function, you need to call the start function start() first, so SCL=0
void WriteCurrent(unsigned char y)
{
unsigned char i;
for(i = 0; i < 8; i++) // Shift in 8 bits in a loop
{
SDA = (bit)(y&0x80); //Send the highest bit of data to S through the bitwise "AND" operation
//Because the high bit is in front and the low bit is in the back during transmission
_nop_(); //Wait for one machine cycle
SCL = 1; //Write data to AT24Cxx on the rising edge of SCL
_nop_(); //Wait for one machine cycle
_nop_(); //Wait for one machine cycle
SCL = 0; //Reset SCL to low level to form the 8 pulses required to transmit data on the SCL line
y <<= 1; //Shift each binary bit in y one bit to the left
}
//Return AT24Cxx acknowledgement bit
}
/***************************************************
Function: Main function
************************************************/
main(void)
{
//Data writing process:
//Start-write chip addressing-response-write data first address-response-write data-response-termination signalstart
(); //Start data transferWriteCurrent
(0xa0); //Select the AT24Cxx chip to be operated, and inform it to write dataack
();
WriteCurrent(0x36); //Write to the specified address 0x36
ack();
WriteCurrent(0x00); //Write data 0x00 to the current address (the address specified above)
ack();
stop(); //Stop data transfer
delaynms(4);
//Read data flow:
//Start-write chip addressing-response-write data first address-response-restart-write chip addressing-response-read datastart
();
WriteCurrent(0xa0); //Select the AT24Cxx chip to be operated, and inform it to write dataack
();
WriteCurrent(0x36); //Read data addressack
();
start();
WriteCurrent(0xa1); //Select the AT24Cxx chip to be operated and tell it to read its data
ack();
P1=ReadData(); //Store the read data into P1
stop(); //Stop data transmission
}
Previous article:C51 timer to measure pulse width
Next article:c51: Inspection DS18B20
Recommended ReadingLatest update time:2024-11-16 14:28
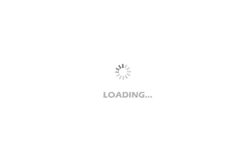
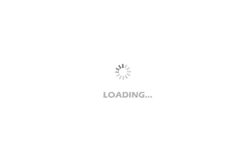
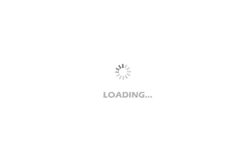
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
Plant growth monitoring system source code based on Raspberry Pi 5
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Lifting the veil on electromagnetic compatibility of PCB boards
- The difference between various debugging interfaces (SWD, JTAG, Jlink, Ulink, STlink)
- [SAMR21 new gameplay] 35. HCSR04 ultrasonic sensor
- The evaluation plan for the current probe and signal generator winners is open! The instruments have been distributed, and we look forward to the sharing of the 6 little ones
- How to use stm to make lcd12864 display
- Thank you + my son, my daughter, and my wife
- How to set breakpoints when debugging a program in keil4?
- 【Qinheng Trial】3. System clock and TIMER0
- [SAMR21 New Gameplay] 32. CPU-related functions
- C2000 Power-on Boot Mode Analysis