ATMega16 has two types of timers, 8-bit and 16-bit. When they overflow is fixed, that is, an interrupt will be generated when the maximum value of their counting range is reached. The maximum counting range of the 8-bit timer is 0~256 (2 to the 8th power), which means it will generate an interrupt when the count reaches 256. The maximum counting range of the 16-bit timer is 0~65536 (2 to the 16th power), which means it will generate an interrupt when the count reaches 65536.
What we call the initial counting value is to set where the timer starts counting. Take an 8-bit timer as an example: the initial value is 100, so the timer starts to accumulate from 100, accumulates 156 times, and generates an interrupt after adding to 256. This is the time consumed in the middle and the instruction cycle is the time we want to set; for example: the initial value is 200, so the timer starts to accumulate from 200, accumulates 56 times, and generates an interrupt after adding to 256. It can be seen that the first timing will only be interrupted after accumulating 156 times, while the second time will be interrupted as long as it accumulates 56 times. Obviously, the time set for the first time is longer than the second time.
Timers can not only set time, but we often use timers when we need precise timing. We can calculate how long it will take for the initial value we set to enter the interrupt.
The following are the registers needed when setting the 8-bit timer:
Experimental platform: ATMega16
Crystal: 11.0592 MHz
Calculation of initial value:
1, 11059200 / 1024 = 10800 Set the frequency to 1024 times, and get how many accumulations are needed per second
2. 10800 / 100 = 108. How many accumulations are needed to get a timing of 10ms?
3, 256 - 108 = 148. Subtract the time to be accumulated from the maximum value of the calculation range to get the initial value, that is, where to start accumulating so that the time is 10ms when overflow occurs.
4, 148 <==> 0x94 Get the hexadecimal value and assign it to TCNT0
Experimental code: Timing 10ms
#include
unsigned char flag = 0;
void timer_init(void)
{
TCCR0 = 0x05; //1024 division
TCNT0 = 0x94; //Assign initial value to count
TIMSK_TOIE0 = 1; //Enable
SREG_I = 1; //Open total interrupt
}
#pragma vector = TIMER0_OVF_vect
__interrupt void time0_normal(void)
{
TCNT0 = 0x94; //Re-assign initial value
flag++;
}
void main(void)
{
timer_init();
DDRB_Bit0 = 1;
while(1)
{
if(flag == 100) //10ms repeated 100 times, that is 1 second
{
PORTB_Bit0 = ~PORTB_Bit0; //Make LED flash
flag = 0;
}
}
}
//++++++++++++++++++++++++++++++++++++++++++++++ +++++++++++++++++++++//
Experimental platform: ATMega16
Crystal: 11.0592
16-bit timer initial value setting:
1, 11059200 / 256 = 43200 Set the frequency division to 256 times to get the number of accumulations required per second
2. 65536 - 43200 = 22336. Subtract the time to be accumulated from the maximum value of the calculation range to get the initial value, that is, where to start accumulating so that the time is 1s when overflow occurs.
3, 22336 <==> 0x57 0x40 Get the hexadecimal value and assign it to TCNT1H and TCNT1L
Experimental code: Timing 1s
#include
unsigned char flag = 0;
void timer_init(void)
{
TCCR1B = 0x04;
TCNT1H = 0x57;
TCNT1L = 0x40;
TIMSK_TOIE1 = 1;
SREG_I = 1;
}
#pragma vector = TIMER1_OVF_vect
__interrupt void time1_normal(void )
{
TCNT1H = 0x57;
TCNT1L = 0x40;
flag++;
}
void main(void)
{
timer_init();
DDRB_Bit0 = 1;
while(1)
{
if(flag == 1)
{
PORTB_Bit0 = ~PORTB_Bit0;
flag = 0;
}
}
}
Previous article:IAR For AVR Serial Port Interrupt Receive
Next article:IAR For AVR Precision Delay
Recommended ReadingLatest update time:2024-11-16 18:09
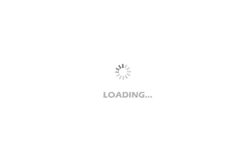
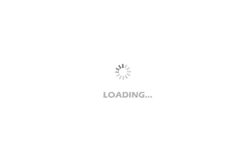
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Dynamic Near Field Communication (NFC) Type 4B Tag
- [GD32E231 Work Submission] Environmental Radiation Dose Monitoring Equipment Design
- [RVB2601 Creative Application Development] 7. IoT Control
- DM6446 DSP end program optimization
- MPXV2202DP connected to IN132 output abnormality, I don't know how to deal with it
- CES 2021 Complement Event Activity Recognition on IMU with Machine Learning Core
- Tektronix Prize-giving Event | Popular Applications of Semiconductor Materials and Device Test Technology
- Internet of Things vs Industrial Internet of Things: 10 Differences That Matter
- The stm32f030c8t6 chip crashes as long as it fetches data during an interrupt
- 【Arduino】168 sensor module series experiments (219) --- INMP441 omnidirectional microphone