/*
Keywords:STM32 UART5
Reference address:STM32 UART5 interrupt receiving program
This function is rewritten based on the online program. Serial port 5 uses interrupt reception. When 20 characters are received, 20 numbers are returned to the PC. For details, see the main function and interrupt function.
*/
#include "stm32f10x_lib.h"
unsigned char TxBuffer5[100] ;
unsigned int i;
unsigned int flag;
/***************************************************
* Function name: void RCC_Configuration()
* Functional description: Reset and clock control configuration
* Parameters: None
* Return value: None
* Global variables: None
* Global static variables: None
* Local static variables: None
***********************************************************/
void RCC_Configuration()
{
ErrorStatus HSEStartUpStatus; //Define the external high-speed crystal oscillator startup status enumeration variable
RCC_DeInit(); //Reset RCC external registers to default values
RCC_HSEConfig(RCC_HSE_ON); //Turn on the external high-speed crystal oscillator
HSEStartUpStatus=RCC_WaitForHSEStartUp(); //Wait for the external high-speed clock to be ready
if(HSEStartUpStatus==SUCCESS){ //External high-speed clock is ready
FLASH_PrefetchBufferCmd(FLASH_PrefetchBuffer_Enable); /* Enable the FLASH pre-read buffer function to speed up the reading of FLASH.
Required usage in all programs, location: in the RCC initialization subroutine, after the clock starts oscillating*/
FLASH_SetLatency(FLASH_Latency_2); //FLASH timing delays several cycles to wait for bus synchronization operation. It is recommended to set the operating frequency of the MCU system according to the following: 0-24MHz, take //Latency=0; 24-48MHz, take Latency=1; 48~72MHz, take Latency=2.
RCC_HCLKConfig(RCC_SYSCLK_Div1); //Configure AHB(HCLK)==system clock/1
RCC_PCLK2Config(RCC_HCLK_Div1); //Configure APB2 (high speed) (PCLK2) == system clock/1
RCC_PCLK1Config(RCC_HCLK_Div2); //Configure APB1 (low speed) (PCLK1) == system clock/2
//Note: AHB is mainly responsible for external memory clock. APB2 is responsible for AD, I/O, advanced TIM, serial port 1. APB1 is responsible for DA, USB, SPI, I2C, CAN, serial port 2345, and ordinary TIM.
RCC_PLLConfig(RCC_PLLSource_HSE_Div1,RCC_PLLMul_9); //Configure PLL clock == (external high-speed crystal clock/1) * 9 ==72MHz
RCC_PLLCmd(ENABLE); //Enable PLL clock
while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY)==RESET); //Wait for PLL clock to be ready
RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK); //Configure system clock == PLL clock
while(RCC_GetSYSCLKSource()!=0x08); //Wait for the system clock source to start
}
//------------------------The following is the operation of turning on the peripheral clock-----------------------//
//RCC_AHBPeriphClockCmd (ABP2 device 1 | ABP2 device 2, ENABLE); //Start AHB device
//RCC_APB2PeriphClockCmd(ABP2 device 1 | ABP2 device 2, ENABLE); //Start ABP2 device
//RCC_APB1PeriphClockCmd(ABP2 device 1 | ABP2 device 2, ENABLE); //Start ABP1 device
//RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO | RCC_APB2Periph_USART1 , ENABLE); //Enable APB2 peripherals
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO
|RCC_APB2Periph_GPIOC | RCC_APB2Periph_GPIOD, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_UART5,ENABLE);
}
/*********************************************
* Function name: NVIC_Configuration(void)
* Function description: NVIC (Nested Interrupt Controller) configuration
* Parameters: None
* Return value: None
* Global variables: None
* Global static variables: None
* Local static variables: None
***********************************************/
void NVIC_Configuration( )
{
NVIC_InitTypeDef NVIC_InitStructure; //Define an interrupt structure
// NVIC_SetVectorTable(NVIC_VectTab_FLASH, 0x0); //Set the starting address of the interrupt vector table to 0x08000000
// NVIC_PriorityGroupConfig(NVIC_PriorityGroup_0); //Set NVIC priority grouping method.
//Note: There are 16 priority levels in total, divided into preemptive and responsive. The number of the two priority levels is determined by this code. NVIC_PriorityGroup_x can be 0, 1, 2, 3, 4.
// respectively represent the preemption priority levels of 1, 2, 4, 8, and 16 and the response priority levels of 16, 8, 4, 2, and 1. After specifying the number of two priority levels, all interrupt levels must be selected from them.
//A high preemption level will interrupt other interrupts and give priority to execution, while a high response level will give priority to execution after other interrupts are completed.
NVIC_InitStructure.NVIC_IRQChannel = UART5_IRQChannel; //Channel is set to serial port 1 interrupt
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0; //Interrupt response priority 0
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //Enable interrupt
NVIC_Init(&NVIC_InitStructure); //Initialization
/* Enable the USART1 Interrupt
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);*/
}
/****************************************
* Function name: GPIO_Configuration()
* Function description: GPIO configuration
* Parameters: None
* Return value: None
* Global variables: None
* Global static variables: None
* Local static variables: None
****************************************/
void GPIO_Configuration()
{
GPIO_InitTypeDef GPIO_InitStructure; //Define GPIO initialization structure
//--------Configure UART5 TX as multiplexed push-pull output AF_PP---------------------//
GPIO_InitStructure.GPIO_Pin=GPIO_Pin_12; //Pin position definition, the label can be NONE, ALL, 0 to 15.
GPIO_InitStructure.GPIO_Speed=GPIO_Speed_50MHz; //Output speed 50MHz
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_AF_PP; //Push-pull output mode Out_PP
GPIO_Init(GPIOC,&GPIO_InitStructure); //Group E GPIO initialization
//--------Configure USART1 TX as multiplexed push-pull output AF_PP---------------------//
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_Init(GPIOA, &GPIO_InitStructure);
//--------Configure UART5's RX as multiplexed floating input IN_FLOATING---------------------//
GPIO_InitStructure.GPIO_Pin=GPIO_Pin_2; //Pin location definition
//Configuring output speed in input mode is meaningless
//GPIO_InitStructure.GPIO_Speed=GPIO_Speed_2MHz; //Output speed 2MHz
GPIO_InitStructure.GPIO_Mode=GPIO_Mode_IN_FLOATING; //Floating input IN_FLOATING
GPIO_Init(GPIOD,&GPIO_InitStructure); //Group C GPIO initialization
//--------Configure USART1's RX as multiplexed floating input IN_FLOATING---------------------//
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
/****************************************************
* Function name: USART1_Configuration()
* Function description: Configure USART1 data format, baud rate and other parameters
* Parameters: None
* Return value: None
* Global variables: None
* Global static variables: None
* Local static variables: None
*******************************************************/
void UART5_Configuration( )
{
USART_InitTypeDef USART_InitStructure; //Serial port settings restore default parameters
USART_InitStructure.USART_BaudRate = 115200; //Baud rate 115200
USART_InitStructure.USART_WordLength = USART_WordLength_8b; //Word length 8 bits
USART_InitStructure.USART_StopBits = USART_StopBits_1; //1-bit stop byte
USART_InitStructure.USART_Parity = USART_Parity_No; //No parity check
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None; //无流控制
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; //Turn on Rx receiving and Tx sending functions
/* Configure USART1 */
//USART_Init(USART1, &USART_InitStructure);
/* Configure UART5 */
USART_Init(UART5, &USART_InitStructure); //Initialization
/* Enable USART1 Receive and Transmit interrupts */
// USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
// USART_ITConfig(USART1, USART_IT_TXE, ENABLE);
/* Enable UART5 Receive and Transmit interrupts */
USART_ITConfig(UART5, USART_IT_RXNE, ENABLE); // If the receive data register is full, an interrupt is generated
// USART_ITConfig(UART5, USART_IT_TXE, ENABLE);
/* Enable the USART1 */
// USART_Cmd(USART1, ENABLE);
/* Enable the UART5 */
USART_Cmd(UART5, ENABLE); //Start the serial port
//-----The following statement solves the problem that the first byte cannot be sent correctly-----//
USART_ClearFlag(UART5, USART_FLAG_TC); // Clear flag
// USART_ClearFlag(USART1, USART_FLAG_TC);
}
/********This is the interrupt service subroutine, in stm32f10x_it.c**************************** */
void UART5_IRQHandler(void)
{
if(USART_GetITStatus(UART5, USART_IT_RXNE) != RESET) //If the receive data register is full
{
TxBuffer5[i]=USART_ReceiveData(UART5);
i++;
}
if(i==20)
{ flag=1;
i=0;}
// USART_SendData(UART5, USART_ReceiveData(UART5)); //Send back to PC
// while(USART_GetFlagStatus(UART5, USART_IT_TXE)==RESET);//Wait for sending to complete
}
/*
void delay(void)
{
unsigned int a,b;
for(a=0;a<1000;a++)
for(b=0;b<200;b++)
} */
/**************************************************
* Function name: main()
* Function description: Main function
* Parameters: None
* Return value: None
* Global variables: None
* Global static variables: None
* Local static variables: None
****************************************************/
int main()
{
unsigned int j;
RCC_Configuration();
GPIO_Configuration();
NVIC_Configuration( );
UART5_Configuration();
while(1){
if(flag)
{
for(j=0;j<20;j++)
{
USART_SendData(UART5,j); //Send back to PC
while(USART_GetFlagStatus(UART5, USART_FLAG_TXE)==RESET);//Wait for USART_IT_TXE to be sent
}
flag=0;
}
}
}
Previous article:STM32 TIMx configuration
Next article:Learning experience of STM32 CAN filter
Recommended ReadingLatest update time:2024-11-17 01:39
Issues that should be noted when using hardware simulation serial port interrupt processing function in STM32
When we use jlink or other emulators to simulate the data reception of the serial port interrupt processing function, if a breakpoint is set in the interrupt function, we will send data to the serial port byte by byte instead of sending multiple bytes at a time. Of course, in a broad sense, if you send so many bytes a
[Microcontroller]
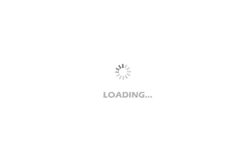
STM32 communication interface (I) serial port
What is a Serial Port There is no doubt that the serial port is the first communication interface we come into contact with. Whether it is serial port debugging or communication with peripherals, the serial port has a wide range of uses. Regarding synchronous and asynchronous, the easiest way to distinguish is to se
[Microcontroller]
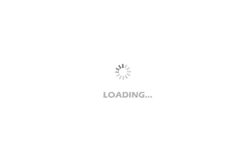
STM32 system ticking and the delay techniques you need to know
I think every microcontroller enthusiast and engineering development designer has had the experience of lighting a lamp. Flowing lights are good things, especially in an environment with limited debugging resources, which can sometimes be of great help.
However, when we first get started, how can we make these sma
[Microcontroller]
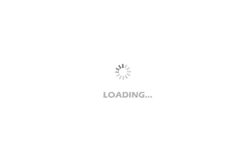
[STM32] Overview of external interrupts, registers, library functions (EXTI general steps)
STM32F1xx official information: "STM32 Chinese Reference Manual V10" - Chapter 9 Interrupts and Events External Interrupt Overview External interrupt (EXTI) is different from the external interrupt of CM3 core described in NVIC interrupt priority management (interrupt vector table). Specifically refers to the
[Microcontroller]
![[STM32] Overview of external interrupts, registers, library functions (EXTI general steps)](https://6.eewimg.cn/news/statics/images/loading.gif)
STM32 basic knowledge learning - system architecture and clock
1. STM32 system architecture stm32 is mainly composed of two master modules and four slave modules 1 Two main modules: Cortex-M0 core and Advanced High-Performance Bus (AHB bus) 通用DMA ( GP-DMA – general-purpose DMA) 2 Four slave modules: Internal SRAM Internal Flash Memory AHB to APB bridge, all peripherals
[Microcontroller]
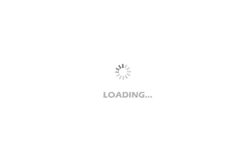
【STM32】NVIC register set
Three structures are defined in the STM32 firmware library to correspond to these three register groups. The correspondence between these three structures and the registers in the ARM manual is as follows:
1. NVIC Register Group
The STM32 firmware library has the following definitions:
typedef struct
{
vu32 IS
[Microcontroller]
STM32 basic timer delay function
Note: The chip used in this practice is the STM32F103VET6 of the cortex-m3 series, and the content involved in the article covers the entire STM32F1 series M3 microcontroller. This article is suitable for beginners who are learning STM32. The STM32 timer includes basic timer, general timer and advanced timer. TIM6 and
[Microcontroller]
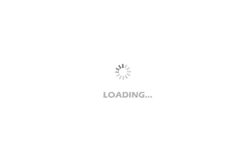
[STM32] Basic principles of general timers (Example: timer interrupt)
STM32F1xx official information: "STM32 Chinese Reference Manual V10" - Chapter 14 General Timer STM32 timer: STM32F103ZET6 has a total of 8 timers, which are: Advanced timers (TIM1, TIM8); general timers (TIM2, TIM3, TIM4, TIM5); basic timers (TIM6, TIM7). STM32 general timer General timer function features de
[Microcontroller]
![[STM32] Basic principles of general timers (Example: timer interrupt)](https://6.eewimg.cn/news/statics/images/loading.gif)
- Popular Resources
- Popular amplifiers
Recommended Content
Latest Microcontroller Articles
He Limin Column
Microcontroller and Embedded Systems Bible
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
MoreSelected Circuit Diagrams
MorePopular Articles
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
MoreDaily News
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- Rambus Launches Industry's First HBM 4 Controller IP: What Are the Technical Details Behind It?
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
Guess you like
- Qorvo helps simplify GaN PA biasing
- Please tell me about the ADS1252 reading data question
- The difference between FPGA and DSP (rough summary)
- gcc cannot find library
- Modification + Portable Ion Air Purifier Disassembly and Modification of Coal Stove Lighter
- [Smart Kitchen] Unboxing - STM32F750
- Is it feasible to automatically turn off the backlight under the key switch?
- 4~20mA current loop acquisition circuit
- Can camera monitoring systems really expand drivers' fields of vision and avoid traffic accidents?
- Several common communication protocols for microcontrollers