#ELSE
#ELIF
#ENDIF
Syntax: #if expr
code
#elif expr
code
#else
code
#endif
expr is a constant expression, a standard operator or a preprocessor identifier;
Code is any standard C source program.
Purpose: The preprocessor evaluates the constant expression and, if the value is non-zero, processes all lines above the optional #ELSE or #ENDIF.
NOTE: You cannot use C variables in #IF, only those created by the preprocessor via #define.
The preprocessor expression DEFINED(id) can be used to return 1 if id is defined, and 0 if id is not defined.
Example: #if MAX_VALUE>255
long value; //If MAX_VALUE>255, define value as a long integer variable
#else
int value; //If MAX_VALUE is not greater than 255, define value as an integer variable
#endif
Example file: ex_extee.c
File: ex_extee.c is as follows:
#if defined(__PCB__) //If the PCB compiler is used, the return value of defined(__PCB__) is 1
#include <16c56.h> //Include 16c56.h header file
#fuses HS, NOWDT, NOPROTECT //HS: high speed crystal/resonator, NOWDT: do not use WDT
// NOPROTECT: Program memory code is not protected
#use delay(clock=20000000) // Enable the built-in functions: delay_ms() and delay_us()
//#USE DELAY() must appear before #use rs232().
#use rs232(baud=9600, xmit=PIN_A3, rcv=PIN_A2)
//Use the baud rate as 9600,
//The sending pin is PIN_A3
//The receiving pin is PIN_A2
//Enable built-in functions: GETC, PUTC and PRINTF, kbhit();
#elif defined(__PCM__)
#include <16F877.h>
#fuses HS,NOWDT,NOPROTECT,NOLVP
#use delay(clock=20000000)
#use rs232(baud=9600, xmit=PIN_C6, rcv=PIN_C7) // Jumpers: 8 to 11, 7 to 12
#elif defined(__PCH__)
#include <18F452.h>
#fuses HS,NOWDT,NOPROTECT,NOLVP
#use delay(clock=20000000)
#use rs232(baud=9600, xmit=PIN_C6, rcv=PIN_C7) // Jumpers: 8 to 11, 7 to 12
#endif //End if definition
#include
#include <2416.c> //Include 2416.c header file
void main() {
BYTE value, cmd; //Declare byte variables value, cmd
EEPROM_ADDRESS address; //Use EEPROM_ADDRESS instead of long int, which is 16 bits
init_ext_eeprom(); //Initialize the I/O pins connected to eeprom
do {
do {
printf("\r\nRead or Write: ");
cmd=getc(); //Read a byte from RS232 port
cmd=toupper(cmd); //Convert lowercase letters in cmd into uppercase letters and send them to cmd
putc(cmd);
} while ( (cmd!='R') && (cmd!='W') ); //Until R or W is entered
printf("\n\rLocation: ");
#if sizeof(EEPROM_ADDRESS)==1
//If EEPROM_ADDRESS is defined as 1 byte, sizeof(EEPROM_ADDRESS)==1 returns 1
address = gethex();
#else //If EEPROM_ADDRESS is defined to be greater than 1 byte
#if EEPROM_SIZE>0xfff
address = gethex();
#else // EEPROM_SIZE is less than 0xfff
address = gethex1(); //Read a byte from the RS232 port and store the high byte address for eeprom
#endif //End if definition
address = (address<<8)+gethex(); //Read 2 bytes from RS232 port and store low byte address for eeprom
#endif //End if definition
if(cmd=='R') //If you enter R, execute the following
printf("\r\nValue: %X\r\n",READ_EXT_EEPROM( address ) );
if(cmd=='W') {
printf("\r\nNew value: ");
value = gethex(); //Input from RS232, prepare for writing eeprom value
printf("\n\r");
WRITE_EXT_EEPROM( address, value );
}
} while (TRUE);
}
File: input.c is as follows:
#include
BYTE gethex1() {
char digit; //declare byte variable digit
digit = getc(); //Read a byte from the RS232 port
putc(digit); //Write a byte to the RS232 port
if(digit<='9') //Return the read byte in hexadecimal
return(digit-'0'); //If the read ASCII code is less than or equal to 39, subtract 30 and return it in hexadecimal
else
return((toupper(digit)-'A')+10); //If the read ASCII code is greater than 39, subtract 41 from it, add 10 and return
}
BYTE gethex() {
int lo, hi; //declare integer variables lo, hi
hi = gethex1(); //Read a byte from the RS232 port and store it in hi
lo = gethex1(); //Read a byte from the RS232 port and store it in lo
if(lo==0xdd)
return(hi);
else
return( hi*16+lo );
}
void get_string(char* s, int max) {
int len; //declare integer variable len
char c; //declare byte type variable c
--max; //Initialize max value
len=0; //Initialize len value
do {
c=getc(); //Read a byte from the RS232 port and store it in c
if(c==8) { // If Backspace is the space key
if(len>0) {
only--;
putc(c); //Write c to RS232
putc(' ');
putc(c);
}
} else if ((c>=' ')&&(c<='~'))
if(len s[len++]=c; putc(c); } } while(c!=13); s[len]=0; } // stdlib.h is required for the ato_ conversions // in the following functions #ifdef _STDLIB //If _STDLIB is defined, execute the following signed int get_int() { char s[5]; //declare character array s[5] signed int i; //Declare a signed integer variable i get_string(s, 5); //Read 5 bytes from the RS232 port and store them in the s array i=atoi(s); //Convert the string in array s[] into an integer and send it to i return(i); } signed long get_long() { char s[7]; //declare character array s[7] signed long l; //Declare a signed long integer variable l get_string(s, 7); //Read 7 bytes from the RS232 port and store them in the s array l=atol(s); //Convert the string in array s[] into a long integer and send it to l return(l); } float get_float() { char s[20]; //declare character array s[7] float f; //declaration of dot-type variable l get_string(s, 20); //Read 20 bytes from the RS232 port and store them in the s array f = atof(s); //Convert the string in array s[] into a symbol number and send it to f return(f); } #endif //End if definition File: 2416.c is as follows: //// Library for a MicroChip 24LC16B //// //// init_ext_eeprom(); Call before the other functions are used //// //// write_ext_eeprom(a, d); Write the byte d to the address a //// //// d = read_ext_eeprom(a); Read the byte d from the address a //// //// b = ext_eeprom_ready(); Returns TRUE if the eeprom is ready //// //// to receive opcodes //// //// The main program may define EEPROM_SDA //// //// and EEPROM_SCL to override the defaults below. //// //// Pin Layout //// //// ----------------------------------------------------------- //// //// | | //// //// | 1: NC Not Connected | 8: VCC +5V | //// //// | 2: NC Not Connected | 7: WP GND | //// //// | 3: NC Not Connected | 6: SCL EEPROM_SCL and Pull-Up | //// //// | 4: VSS GND | 5: SDA EEPROM_SDA and Pull-Up | //// //// ----------------------------------------------------------- //// #ifndef EEPROM_SDA //If EEPROM_SDA is not defined, execute the following #define EEPROM_SDA PIN_C4 //用EEPROM_SDA replace PIN_C4 #define EEPROM_SCL PIN_C3 //用EEPROM_SCL代替PIN_C3 #endif //End if definition #use i2c(master, sda=EEPROM_SDA, scl=EEPROM_SCL) // Set the master to host mode //Unless FORCE_HW is specified, a software function emulating I2C will be generated. // Enable I2C_START, I2C_STOP until the next #USE I2C appears. // Enable I2C_READ, I2C_WRITE until the next #USE I2C occurs. // Enable I2C_POLL until the next #USE I2C occurs. //Specify the sda pin as EEPROM_SDA, and the scl pin as EEPROM_SCL #define EEPROM_ADDRESS long int //用EEPROM_ADDRESS代替long int #define EEPROM_SIZE 2048 //Replace 2048 with EEPROM_SIZE void init_ext_eeprom() { output_float(EEPROM_SCL); //Set the EEPROM_SCL pin as input, open collector connection output_float(EEPROM_SDA); //Set the EEPROM_SDA pin as input, open collector connection } BOOLEAN ext_eeprom_ready() { int1 ack; //declare bit variable ack i2c_start(); //Send start condition ack = i2c_write(0xa0); //Send slave address 0xa0; if ack=0, it means slave responds (ACK); //If ack=1, it means the slave does not respond (NO ACK); i2c_stop(); //Send stop condition return !ack; } // ext_eeprom_ready() function, if it returns 1, it means the slave is ready; if it returns 0, it means the slave is busy or the eeprom is broken void write_ext_eeprom(long int address, BYTE data) { while(!ext_eeprom_ready()); //If the slave is busy, the host waits i2c_start(); //Send start condition i2c_write( (0xa0|(BYTE)(address>>7))&0xfe); //Send the high byte of the command word (send write command) i2c_write(address); //Send the low byte of the command word i2c_write(data); //Send data i2c_stop(); //Send stop condition } BYTE read_ext_eeprom(long int address) { BYTE data; //Declare byte variable data while(!ext_eeprom_ready()); //Send the device address first, if the slave is busy, the host waits i2c_start(); //Here is: send restart condition i2c_write( (0xa0|(BYTE)(address>>7))&0xfe); //Send the high byte of the command word (send write command) i2c_write(address); //Send the low byte of the command word i2c_start(); //Send start condition i2c_write( (0xa0|(BYTE)(address>>7))|1); //Send the high byte of the command word (send a read command) data=i2c_read(0); //Read I2C data, then send ack=0 (no need for slave response) i2c_stop(); //Send stop condition return(data); //Return the read I2C data } The above example is mainly used to read and write 24C16, and verify it through PC RS232
Previous article:PIC microcontroller CCS C language (#IFDEF, #ENDIF usage)
Next article:PIC microcontroller CCS C language (#FUSES usage)
Recommended ReadingLatest update time:2024-11-16 15:27
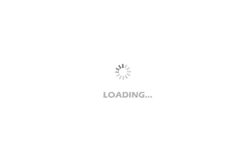
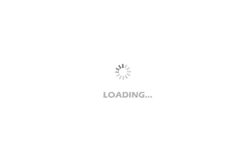
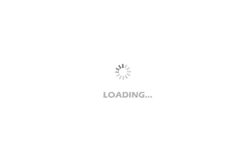
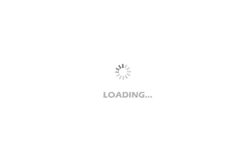
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- STM32F103 brushless DC motor control program
- [GD32E231 DIY Contest] Part 2: Dynamic QR Code Display
- EEWORLD University ----PI power chip: Learn about BridgeSwitch in one minute
- CCS compilation optimization and volatile
- cc3200 GPIO input mode error
- Excellent materials, free of credits: Album of routines for common functional modules in the National Undergraduate Electronic Design Competition
- [Popular Science] IGBT - Starting from the name
- Relay protection device tester verification
- The circuit board cannot start normally after plugging in a power bank
- FPGA beginners ask for help with Verilog test code