The detailed code and simulation are as follows: (My hardware system is the min Mega16/32 + JTAG ICE of this site)
- #include "config.h"
- struct _PID
- {
- float PVn; //feedback signal variable
- float SPn; //set value
- float Mn; //PID calculation result
- float Kc; //proportional coefficient
- float Ts; //sampling time (ms)
- float Ti; //Integral time (ms)
- float Td; //differential time (ms)
- float Mx; //Integral item adjustment parameter
- float PVn_1; //previous feedback variable
- float MPn; //result value of the proportional term
- float MIn; //Result value of integral term
- float MDn; //Result value of the differential term
- };
- struct _PID *myPID;
- void init_myPID(void);
- void init_ports(void);
- void init_device(void);
- float MPn_value(struct _PID *PID);
- float MIn_value(struct _PID *PID);
- float MDn_value(struct _PID *PID);
- float Mx_value(struct _PID *PID);
- float Mn_value(struct _PID *PID);
- void main (void)
- {
- init_device();
- init_myPID();
- myPID->SPn = 155.5;
- myPID->Kc = 13.2;
- myPID->Ts = 0.2;
- myPID->If = 600.0;
- myPID->Td = 0.0;
- myPID->PVn = 108.2;
- while(1)
- {
- myPID->MPn = MPn_value(myPID);
- myPID->MDn = MDn_value(myPID);
- myPID->Mx = Mx_value(myPID);
- myPID->MIn = MIn_value(myPID);
- myPID->Mn = Mn_value(myPID);
- myPID->PVn_1 = myPID->PVn;
- }
- }
- /******************************************************************************/
- void init_myPID(void)
- {
- myPID->PVn = 0.0;
- myPID->SPn = 0.0;
- myPID->Mn = 0.0;
- myPID->Kc = 0.0;
- myPID->Ts = 0.0;
- myPID->If = 0.0;
- myPID->Td = 0.0;
- myPID->Mx = 0.0;
- myPID->PVn_1 = 0.0;
- myPID->MPn = 0.0;
- myPID->MIn = 0.0;
- myPID->MDn = 0.0;
- }
- //------------------------------------------------------------------------------
- void init_ports(void)
- {
- PORTA = 0x00; //If ADC Function was be used,the PORTA could`t set bit 1
- DDRA = 0x00; //the port set input mode.
- PORTB = 0x00;
- DDRB = 0x00;
- PORTC = 0x00; //m103 output on
ly - DDRC = 0x00;
- PORTD = 0x00;
- DDRD = 0x00;
- }
- //------------------------------------------------------------------------------
- void init_device(void)
- {
- CLI();
- init_ports();
- MCUCR = 0x00; //Set Power control(State:Close)
- GICR = 0x00; //Set boot guide(State:Close).
- SEI(); //re-enable interrupts
- //all peripherals are now initialized
- }
- // Calculate the value of the proportional term
- //------------------------------------------------------------------------------
- float MPn_value(struct _PID *PID)
- {
- float myMPn = 0.0;
- myMPn = PID->Kc *( PID->SPn - PID->PVn);
- return myMPn;
- }
- // Calculate the value of the integral term
- //------------------------------------------------------------------------------
- float MIn_value(struct _PID *PID)
- {
- float myMIn = 0.0;
- myMIn = PID->Kc*(PID->Ts/PID->Ti)*(PID->SPn - PID->PVn) + PID->Mx;
- return myMIn;
- }
- //Calculate the value of the differential term
- //------------------------------------------------------------------------------
- float MDn_value(struct _PID *PID)
- {
- float myMDn = 0.0;
- myMDn = PID->Kc * (PID->Td/PID->Ts) * (PID->PVn_1 - PID->PVn);
- return myMDn;
- }
- //Calculate the result of PID
- //------------------------------------------------------------------------------
- float Mn_value(struct _PID *PID)
- {
- float myMn = 0.0;
- myMn = PID->MPn + PID->MIn + PID->MDn;
- return myMn;
- }
- //Calculate the adjustment value of the integral term
- //------------------------------------------------------------------------------
- float Mx_value(struct _PID *PID)
- {
- float myMx = 0.0;
- if(PID->Mn > 1.0)
- {
- myMx = 1.0 - (PID->MPn + PID->MDn);
- }
- else if(PID->Mn < 0.0)
- {
- myMx = -(PID->MPn + PID->MDn);
- }
- return myMx;
- }
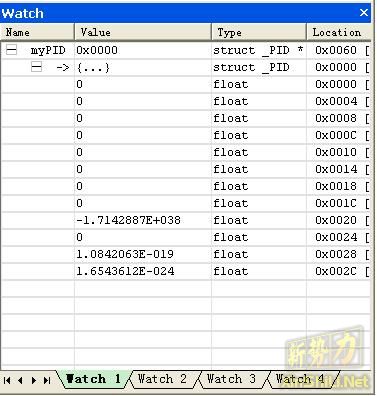
The simulation results when running to the PID initialization function: void init_myPID(void) are as follows: Unable to initialize all to 0
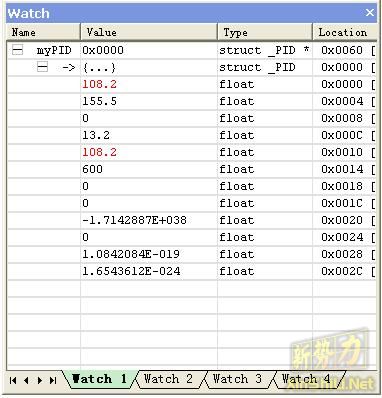
Run to:
myPID->SPn = 155.5;
myPID->Kc = 13.2;
myPID->Ts = 0.2;
myPID->Ti = 600.0;
myPID->Td = 0.0;
myPID->PVn = 108.2;
After reassignment, some parameters Ts are not 0.2
Previous article:How to download AVR ISP download line in AVR Studio environment
Next article:AVR interrupt usage example
Recommended ReadingLatest update time:2024-11-16 19:25
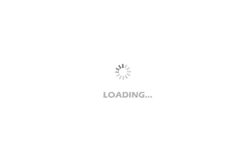
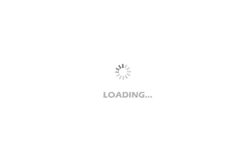
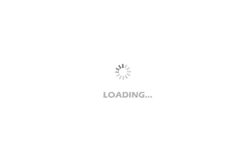
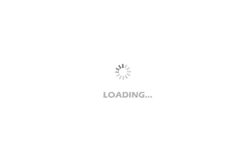
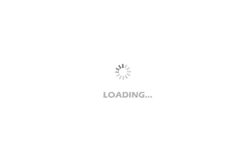
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- How to select and calculate the inductor in a DC-DC power supply
- Moon Phase Display
- Lightning protection for DC power ports
- Purgatory Legend-RAM War
- ATX-100 Series Cable Harness Tester Revealed! (Demo + Features)
- Reasons why the automatic function of a digital oscilloscope cannot be triggered when measuring low-frequency signals
- Running helloworld-porting on iTOP-4418 development board
- Capacitor selection and installation considerations
- Millimeter wave sensor technology for detecting passengers in moving vehicles
- How to detect whether a POE switch is a single-chip microcomputer