//-----------------------Function declaration, variable definition------------------------
#include
#include
#include
//-----------------------Define pins----------------------------------
sbit PWM=P1^0; //PWM waveform output
sbit DR=P1^1; //Direction control
#define timer_data
#define PWM_T 100 //Define PWM period T as 10ms
unsigned char PWM_t; //PWM_t is pulse width (0~100) time is 0~10ms
unsigned char PWM_count; //Output PWM period count
unsigned char time_count; //Timing count
bit direction; //Direction flag bit
//-----------------------------------------------------------------
// Function name: timer_init
// Function function: Initialize facility timer
//-----------------------------------------------------------------
void timer_init()
{
} //----------------------------------------------------------------- // Function name: setting_PWM // Function function: set PWM pulse width and direction //----------------------------------------------------------------- void setting_PWM() { if(PWM_count==0) //Initial
setting {
PWM_t
=
20; direction=1;
}
}
//
-----------------------------------------------------------------
//
Function
name
:
IntTimer0
//
Function function: timer interrupt handler
//
-----------------------------------------------------------------
void
IntTimer0(
) interrupt 1
{
time_count
++;
DR=direction;
if(time_count>=PWM_T
)
{
time_count
=
0
;
PWM_count++;
setting_PWM(); //Called once for each PWM wave output
}
if(time_count
//=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=·=
DC motor closed loop control Keil c51 source code
//-----------------------Function declaration, variable definition------------------------
#include
sbit INT_0 =P3^2; // Set p3.2 as external interrupt 0
sbit pulse_A=P1^2; // P1.2 is pulse A input
sbit PWM=P1^0; //PWM waveform output
sbit DR=P1^1; //Direction control
//-----------------------Predefined values----------------------------------
#define PWM_T 1800 //Define PWM period T as 18ms
#define Ts 1000 //Define photoelectric encoder sampling time as 10ms
#define timer_data
//-----------------------Preset value----------------------------------
bit direction; //Direction flag bit User setting
unsigned char R; //DC motor speed to be obtained User setting
//-----------------------Actual running status------------------------------
bit real_direction; //Actual running direction of the motor
unsigned char Rr; //Actual speed of the DC motor
//-----------------------Calculated compensation status--------------------------
bit compensate_polarity; //Compensation polarity
unsigned char dR; //Speed compensation
//-----------------------Pulse width obtained after compensation------------------------
unsigned char PWM_t; //PWM_t is the pulse width (320~400) and the time is 3.2~4.0ms
unsigned char PWM_count; //Output PWM cycle count
//-----------------------Each intermediate count value------------------------------
unsigned char pulseB_count; //Pulse count
unsigned char time0_count; //Timer count
unsigned char time1_count; //Timer count
//-----------------------------------------------------------------
// Function name: timer_init
// Function function: Initialize and set the timer
//-----------------------------------------------------------------
void timer_init()
{
TMOD=0x22; /*Timer 1 is working mode 2 (8-bit automatic reload), 0 is mode 2 (8-bit automatic reload) */
PCON=0x00;
TF0=0;
TH0=timer_data
TL0=TH0;
TH1=timer_data
TL1=TH0;
ET0=1; //Timer 0 interrupt is enabled
TR0=1; //Timer 0 starts counting
ET1=1; //Timer 1 interrupt is enabled
TR1=1; //Timer 1 starts counting
EA=1; //Interrupt is enabled
}
//-----------------------------------------------------------------
// Function name: INT0_init()
// Function function: Initialization settings
// Set the working mode of INT0
//-----------------------------------------------------------------
void INT0_init(void )
{
pulseB_count=0; //Pulse counter clears
IT0=1; //Select INT0 as edge trigger mode
EX0=1; //External interrupt enable
EA=1; //System interrupt enable
}
//-----------------------------------------------------------------
// Function name: setting_PWM
// Function function: Set PWM pulse width and set direction
//-----------------------------------------------------------------
void setting_PWM()
{
// direction=1; //Set rotation direction
// R=540; //Set speed
// dR=0; //Speed compensation is zero
// calculate_PWM_t(); //Recalculate pulse width
}
//-----------------------------------------------------------------
// Function name: calculate_PWM_t
// Input parameters: R is the DC motor speed to be obtained, dR is speed compensation
// Export parameters: PWM_t is the pulse width (320~400) and the time is 3.2~4.0ms
// Function function: Calculate pulse width, PWM_t=R/150;
//-----------------------------------------------------------------
void calculate_PWM_t()
{
if(compensate_polarity==1) //Positive compensation
PWM_t=(R+dR)/150;
else
PWM_t=(R-dR)/150; //Negative correction
}
//-----------------------------------------------------------------
// Function name: calculate_Rr
// Input parameter: pulseB_count pulse count
// Output parameter: Rr actual speed of DC motor
// Function function: Calculate the actual speed
//-----------------------------------------------------------------
void calculate_Rr()
{
Rr=pulseB_count/6;
}
//-----------------------------------------------------------------
// Function name: compensate_dR
// Input parameter: Rr actual speed of DC motor
// R required DC motor speed
// Output parameter: dR speed compensation
// Function function: Calculate the actual compensation value and compensation polarity, and redesign according to different compensation algorithms
//-----------------------------------------------------------------
void compensate_Rr()
{
Rr=1;
if(Rr>R)
compensate_polarity=0; //Compensation polarityelse
compensate_polarity
=1;
}
//-----------------------------------------------------------------
// Function name: INT0_intrupt
// Function: External interrupt 0 handler
//-----------------------------------------------------------------
void INT0_intrupt() interrupt 0 using 1
{
pulseB_count++;
if(pulse_A==0)
{
real_direction=1; //If P1.2 is low, the motor is forward, and the value of counter N is increased by 1
}
else //If it is high, the motor is reverse, and the value of counter N is reduced by 1.
{
real_direction=1;
}
}
//-----------------------------------------------------------------
// Function name: IntTimer0
// Function function: timer interrupt handler
//-----------------------------------------------------------------
void IntTimer0() interrupt 1
{
time0_count++;
DR=direction;
if(time0_count>=PWM_T)
{
time0_count=0;
PWM_count++;
setting_PWM(); //Called once for each PWM wave output
}
if(time0_count
else
PWM=0;
}
//-----------------------------------------------------------------
// Function name: IntTimer1
// Function function: timer interrupt handler
//-----------------------------------------------------------------
void IntTimer1() interrupt 3
{
time1_count++;
if(time1_count==1)
{
INT0_init(); //Initialize external interrupt settings
}
if(time1_count>=Ts)
{
time1_count=0; //A compensation cycle ends, the counter is cleared
calculate_Rr(); //Calculate the actual speed
compensate_Rr(); //Calculate the actual compensation value and compensation polarity
calculate_PWM_t(); //Recalculate the pulse width
}
}
//-----------------------------------------------------------------
// Function name: main
// User main function
// Function function: main function
//-----------------------------------------------------------------
void main()
{
direction=1; //Set the rotation direction
R=540; //Set the speed
dR=0; //Speed compensation is zero
calculate_PWM_t(); //Recalculate the pulse width
timer_init();
}
Previous article:Summary of 8051 addressing modes
Next article:Detailed explanation of MCS-51 single-chip microcomputer control word
Recommended ReadingLatest update time:2024-11-16 14:46
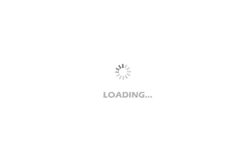
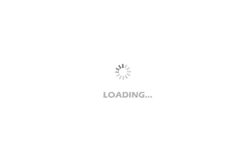
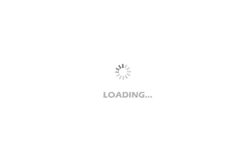
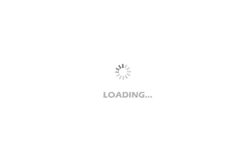
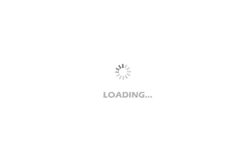
- Popular Resources
- Popular amplifiers
-
西门子S7-12001500 PLC SCL语言编程从入门到精通 (北岛李工)
-
Siemens Motion Control Technology and Engineering Applications (Tongxue, edited by Wu Xiaojun)
-
How to read electrical control circuit diagrams (Classic best-selling books on electronics and electrical engineering) (Zheng Fengyi)
-
MCU C language programming and Proteus simulation technology (Xu Aijun)
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- TouchGFX Design + Make a Rubik's Cube (2)
- Electronic digital alarm clock based on GD32E231
- New version of UFUN learning board information
- 【NXP Rapid IoT Review】 The first test program
- How to Reduce Ripple in a PWM DAC in a Microprocessor
- Interrupt-Based Ambient Light and Environment Sensor Node Reference Design for Sub-1 GHz Networks
- STM32CUbe usage issues
- Low-power MCUs and smart buildings
- STM32F103C8T6 low power consumption issue
- [D-Gesture Recognition Device] D_Gesture Recognition