①How to implement UART output? The registers used by UART are as follows: (1) UART linear control register ULCONn, (2) UART control register UCONn, (3) UART FIFO control register UFCONn, (4) UART MODEM control register UMCONn, (5) UART receive and transmit status register UTRSTATn, (6) UART error status register UERSTATn, (7) UART FIFO status register UFSTATn, (8) UART MODEM status register UMSTATn, (9) UART transmit buffer register UTXHn, (10) UART receive buffer register URXHn, (11) UART baud rate divisor register UBRDIVn.
1. The clock frequency must be configured first. If the baud rate is not correct, communication will not work. A clock configuration template will be given later. First, the UART communication port has 6 wires, two power wires, and the four wires nCTS/nRTS/TXD/RXD correspond to PH0-PH3. First, set them to the second function mode through GPHCON. Use "mpll_val = (92<<12)|(1<<4)|(1);" to set the system frequency = 400MHz through MPLLCON. In addition, through the CLKDIVN clock divider control register, UCLK selection register = 0 means that the UPLL clock is set to 48MHz, which is equal to the 48MHz provided by UCLK to USB, HDIVN = 10, and CAMDIVN[9] = 0, indicating HCLK = FCL/4, PDIVN = 1, indicating PCLK = HCLK/2. OK, the frequencies provided by the system now are: FCLK = 400MHz, HCLK = 100MHz, PCLK = 50MHz, UCLK = 48MHz.
Where CAMDIVN is the camera clock divider register, DIVN_UPLL[12]=0, where FCLK=MPLLclock, HDIVN=FCLK/4=100MHz.
2. Next, initialize UART, set UART FIFO control register, set the lowest authority and close FIFO, turn off UMCON mode 0 and mode 1, and select 8 bits of data per frame by setting ULCON linear control register. Select read and write UART data buffer register interrupt request or query mode through UCON control register rUCON0 = 0x245, and enable UART to interrupt on exception. When the transmit buffer becomes empty in non-FIFO mode or the transmit buffer reaches the transmit FIFO trigger level in FIFO mode, an interrupt request is made.
3. Set the baud rate. Use UBRDIV0/1/2 to set the baud rate respectively.
4. Select the serial port number to be read. In fact, the program selects the serial port number to be read.
5. To write data, use the calling method of WrUTXH0('\r'); WrUTXH0(data); to write data.
6. The program receives and uses the interrupt flag to wait for receiving data. UTRSTAT0/1/2, shift register empty interrupt, and then reads the data in the buffer, and sends the acquired data to the hyperterminal, so that the written data can be seen on the terminal.
②How to achieve PWM modulation output?
The buzzer is controlled by GPB0, and the pin multiplexing function TOUT0 is changed to PWM output.
1. Configure the clock frequency division as above. If the frequency is too fast, you can’t hear the sound from the buzzer!
2. Set TOUT0 output, that is, set GPB0 of GPBCON to 10; then use TCFG0/TCFG1/TCNTB0/TCMPB0 to configure the PWM output frequency.
rTCFG0 &= ~0xff; //These two statements set the calibration value of timer 0 and 1, and the initial value of the cycle count
rTCFG0 |= 15; //prescaler = 15+1
rTCFG1 &= ~0xf; //This is to select the 8-frequency division when the MUX input is input to the PWM timer count
rTCFG1 |= 2; //mux = 1/8
rTCNTB0 = (PCLK>>7)/freq; //Timer 0 count buffer register, used to load the initial value of the down count, anyway, the timer is down counting
rTCMPB0 = rTCNTB0>>1; // 50% is used to compare with the value in the counter, which is also used for pulse width modulation
rTCON &= ~0x1f; //Timer control register, turn off dead zone, auto reload, turn off inverter, update TCNTB0/TCMPB0 initial value, turn on TIMER0
rTCON |= 0xb; //disable deadzone, auto-reload, inv-off, update TCNTB0&TCMPB0, start timer 0
rTCON &= ~2; //clear manual update bit and no longer update TCNTB0/TCMPB0.
In fact, the TCNT0 counter, which actually works the hardest, records the 8-frequency pulse from PCLK. It outputs a high level when TCNT0≤TCMPn and outputs a low level when TCNT0>TCMPn.
So what is the current cycle? Timer output clock frequency = PCLK/{prescaler value+1}/{divider value}, PCLK=50MHz, then divider value is the 8-frequency division, after the prescaler, prescaler=15, so frequency = 50MHz/16/8=390.625KHz. And what is the count value, (PCLK>>7)/freq=390625/freq, here let freq=10, that is, T=(1/390625)*39062.5=0.1S, I don't know why the TCNTB value is useless when it reaches a certain level. I forgot that this register is 16 bits and has a valid value of 65536. It is useless to increase it. It is more effective to change the previous timer output frequency.
③How to realize AD sampling?
First, select the AD channel through the ADCCON register, enable the AD conversion prescaler, write the AD conversion pre-calibration value (equivalent to these two items setting the frequency of AD conversion), and through AN0-AN7/YM/YP/XM/XP, the strange AD conversion, after the AD conversion starts, the AD start bit will change from 1->0, after the AD conversion ends, the AD end mark bit will change from 0->1, and the AD conversion result will be stored in the ADCDATA0 register. The AD of S3C2440 is 10, which can be directly taken out from ADCDAT0. Then the program involves ADCCON and ADCDATA0, the two main registers. The program directly inputs a channel and returns an AD value. The other is the AD clock. When configuring the system clock, choose to assign a frequency reference. IICCON is the I2C bus control register.
④How to implement IIC reading and writing?
Whether it is hardware or software processing, I2C is to build an I2C timing to meet hardware communication and finally realize communication. Although it is simple, it is still analyzed from the register and timing. The IICDS register is used. It is the first address of the IIC device to be written or read, but it must be assigned before starting the IIC transmission; the IICSTAT register is used to start IIC communication, and then the IIC control bus is enabled through IICCON, the ACK function of the IIC bus is enabled, the Tx/Rx interrupt is enabled, and the IICCLK frequency is set, rIICCON = 0xaf;. At present, this program uses a register to mark the status. After reading or writing, the program will fall into while waiting. Then the specific status can only be checked through the interrupt, and the value of that register is updated to make the program jump out of while.
⑤How to create a project in ADS, how to create a new program, how to compile and how to simulate?
ADS is actually software launched by ARM. ADS consists of command line development tools, ARM runtime libraries, GUI development environment (Code Warrior and AXD), and utility support software. The command line development tool completes the source code compilation and linking into executable code. The ARM runtime library is mainly used to support compiled C and C++ code. CodeWarrior for ARM is a complete set of integrated development tools that fully utilizes the advantages of ARM RISC. The tool is designed for ARM RISC-based processors. AXD is an ARM extended debugger.
⑥ "C language" variable number of variables? ?
The macros va_arg(), va_start(), and va_end() are used together to allow a function to take a variable number of arguments. A typical example of a function that takes a variable number of arguments is printf(). The type va_list is defined in
Previous article:S3C2440 Study Notes 5 (Analysis of 2440slib.s source program)
Next article:S3C2440 Learning 4 (How to use Jlink)
Recommended ReadingLatest update time:2024-11-16 15:36
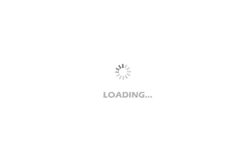
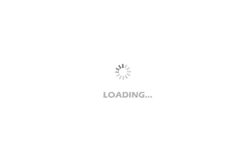
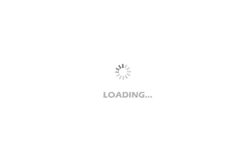
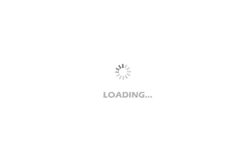
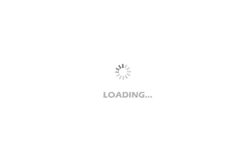
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications