In MDK, MDK defines the following structure for registers related to NVIC:
- typedef struct
- {
- vu32 ISER[2];
- u32 RESERVED0[30];
- vu32 ICER[2];
- u32 RSERVED1[30];
- vu32 ISPR[2];
- u32 RESERVED2[30];
- vu32 ICPR[2];
- u32 RESERVED3[30];
- vu32 IABR[2];
- u32 RESERVED4[62];
- vu32 IPR[15];
- } NVIC_TypeDef;
The interrupts of STM32 are executed in order under the control of these registers. Only by understanding these interrupt registers can we understand the interrupts of STM32. The following is a brief introduction to these registers:
ISER[2]: ISER stands for Interrupt Set-Enable Registers, which is an interrupt enable register group. As mentioned above, the STM32F103 has only 60 maskable interrupts. Here, two 32-bit registers are used, which can represent a total of 64 interrupts. However, the STM32F103 only uses the first 60 bits. Bits 0 to 31 of ISER[0] correspond to interrupts 0 to 31 respectively. Bits 0 to 27 of ISER[1] correspond to interrupts 32 to 59; in this way, a total of 60 interrupts are corresponding. If you want to enable an interrupt, you must set the corresponding ISER bit to 1 to enable the interrupt (this is just enabling, and it must be combined with interrupt grouping, masking, IO port mapping and other settings to be considered a complete interrupt setting). For specific interrupts corresponding to each bit, please refer to line 36 in stm32f10x_nvic..h.
ICER[2]: The full name is Interrupt Clear-Enable Registers, which is an interrupt disable register group. This register group has the opposite function to ISER, and is used to clear the enable of a certain interrupt. The function of its corresponding bit is the same as ICER. Here, we need to set a special ICER to clear the interrupt bit instead of writing 0 to ISER, because these registers of NVIC are valid when writing 1, and invalid when writing 0.
ISPR[2]: The full name is Interrupt Set-Pending Registers, which is an interrupt pending control register group. The interrupt corresponding to each bit is the same as ISER. By setting 1, the ongoing interrupt can be suspended and the interrupt of the same level or higher level can be executed. Writing 0 is invalid.
ICPR[2]: The full name is Interrupt Clear-Pending Registers, which is an interrupt clear control register group. Its function is opposite to ISPR, and the corresponding bits are the same as ISER. By setting 1, the pending interrupt can be cleared. Writing 0 has no effect.
IABR[2]: The full name is Interrupt Active Bit Registers, which is an interrupt activation flag register group. This is a read-only register that can be used to determine which interrupt is currently being executed. It is automatically cleared by hardware after the interrupt is executed. The interrupt represented by the corresponding bit is the same as ISER. If it is 1, it means that the interrupt corresponding to the bit is being executed.
IPR[15]: The full name is Interrupt Priority Registers, which is a register group for interrupt priority control. This register group is very important! The interrupt grouping of STM32 is closely related to this register group. Since STM32 has more than 60 interrupts, STM32 uses interrupt grouping to determine the interrupt priority. The IPR register group consists of 15 32-bit registers, and each maskable interrupt occupies 8 bits, so a total of 15*4=60 maskable interrupts can be represented. This is exactly the same as the number of maskable interrupts of STM32. IPR[0]'s [31~24], [23~16], [15~8], and [7~0] correspond to interrupts 3~0, and so on, corresponding to a total of 60 external interrupts. However, not all of the 8 bits occupied by each maskable interrupt are used, but only the upper 4 bits are used. These 4 bits are divided into preemption priority and sub-priority. The preemption priority is in front, and the sub-priority is in the back. The number of bits each of these two priorities occupies is determined by the interrupt grouping settings in SCB->AIRCR.
Here is a brief introduction to the interrupt grouping of STM32: STM32 divides interrupts into 5 groups, group 0 to 4. The setting of this grouping is defined by bits 10 to 8 of the SCB->AIRCR register. The specific allocation relationship is shown in the table
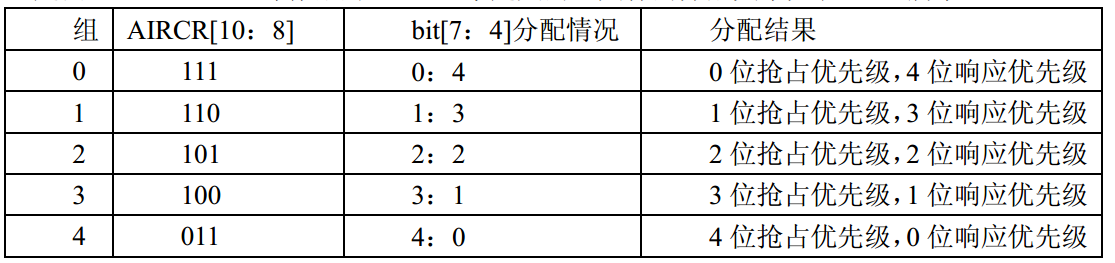
Through this table, we can clearly see the configuration relationship corresponding to groups 0~4. For example, if group is set to 3, then for all 60 interrupts, the highest 3 bits of the upper 4 bits of the interrupt priority register of each interrupt are the preemption priority, and the lower 1 bit is the response priority. For each interrupt, you can set the preemption priority to 0~7 and the response priority to 1 or 0. The preemption priority is higher than the response priority. The smaller the value, the higher the priority.
There are two points to note here: first, if the preemption priority and response priority of two interrupts are the same, the interrupt that occurs first will be executed first; second, the preemption priority with a higher priority can interrupt the ongoing interrupt with a lower preemption priority. For interrupts with the same preemption priority, the response priority with a higher priority cannot interrupt the interrupt with a lower response priority.
Using library functions to implement the above interrupt grouping settings and interrupt priority management simplifies our future interrupt settings. The NVIC interrupt management function is mainly in the misc.c file.
The first is the interrupt priority grouping function NVIC_PriorityGroupConfig. This function is used to group the interrupt priorities. This function can only be called once in the system. Once the grouping is determined, it is best not to change it.
For example, if we set the interrupt priority group value of the entire system to 2 (2-bit preemption priority, 2-bit response priority), the method is: NVIC_PriorityGroupConfig(NVIC_PriorityGroup_2);
After setting up the system interrupt grouping, how do we determine the preemption priority and response priority for each interrupt? Let's look at an important function, the interrupt initialization function NVIC_Init, whose function declaration is:
void NVIC_Init(NVIC_InitTypeDef* NVIC_InitStruct)
NVIC_InitTypeDef is a structure. You can look at the member variables of the structure:
- typedef struct
- {
- uint8_t NVIC_IRQChannel; //Define which interrupt is initialized. We can find the corresponding name of each interrupt in stm32f10x.h, such as USART1_IRQn.
- uint8_t NVIC_IRQChannelPreemptionPriority; //Define the preemption priority level of this interrupt.
- uint8_t NVIC_IRQChannelSubPriority; //Define the sub-priority level of this interrupt.
- FunctionalState NVIC_IRQChannelCmd; //Whether the interrupt is enabled.
- } NVIC_InitTypeDef;
For example, if we want to enable the interrupt of serial port 1, and set the preemption priority to 1 and the sub-priority to 2, the initialization method is:
- USART_InitTypeDef USART_InitStructure;
- NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn; //Serial port 1 interrupt
- NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority=1 ; // preemption priority is 1
- NVIC_InitStructure.NVIC_IRQChannelSubPriority = 2; // Subpriority bit 2
- NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE; //IRQ channel enable
- NVIC_Init(&NVIC_InitStructure);
Previous article:STM32's standby wake-up function
Next article:stm32_file organization structure
Recommended ReadingLatest update time:2024-11-17 00:04
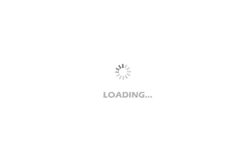
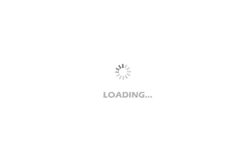
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Op amp output clamping mechanism and avoidance methods
- 【Development and application based on NUCLEO-F746ZG motor】11. Parameter configuration - ADC configuration
- [Solved] Why can’t I change my profile picture?
- The STM32F103VBT6 resets with a button. After releasing the button, it takes three or four seconds for the microcontroller to execute the program.
- [Xianji HPM6750 Review] + Unboxing
- C2000 combines capacitive touch and host controller functions
- 7660 chip common mode inductor manufacturer
- "【TGF4042 Signal Generator】" High-frequency distortion measurement
- Video chip recommendation
- About MSP430 watchdog settings