1. Segment names predefined by the assembly system
.text @code segment.data
@initialized data segment.bss
@uninitialized data segment
It should be noted that the .bss segment should be before .text in the source program.
2. Define the entry point
The default entry point of the assembler is the start label, and the user can also use the ENTRY flag in the linker script file to specify other entry points.
.text
.global _start
_start:
3. Word usage
word expression is to put a word value at the current position. This value is expression
. For example,
_rWTCON:
.word 0x15300000
means putting a value of 0x15300000 at the current address, _rWTCON
4. Equ assignment operation, equivalent to the macro definition of C language
.equ MEM_CTRL_BASE, 0x48000000 //Note that the , sign must be added
5. Logical instructions
AND---Logical "AND" operation instruction
ANDS R1,R1,R2; R1=R1&R2, and update the flag bit according to the result of the operation
AND R0,R0,#0x0F; R0=R0&0x0F, take out the lowest 4 bits of data in R0.
Instruction format: ORR {cond} {S} Rd, Rn, operand2 ORR instruction performs bitwise logical "OR" on operand2 and the value of Rn, and stores the result in the destination register Rd. Instruction example:
ORRS R1, R1, R2; R1 = R1 | R2, and updates the flag bit according to the result of the operation
Instruction format:
BIC{cond}{S} Rd,Rn,operand2
cmp R0,R1; compare R0,R1
beq stop; R0=R1 jump to stop
blt less; R0
Stop:...
reference:
http://blog.csdn.net/denlee/article/details/2501182
In embedded development, assembler programs are often used in critical places, such as initialization at system startup, environment preservation and recovery when entering and exiting interrupts, and functions with very demanding performance requirements.
1. Relative jump instructions: b and bl.
The difference is that in addition to jumping, the bl instruction also saves the return address (the address of the next instruction of bl) in the lr register.
Jump range: 32M before and after the current instruction.
They are position-independent instructions.
Example:
b fun1
......
fun1:
bl fun2
......
fun2:
......
2. Data transfer instructions: mov, address read pseudo-instruction: ldr
The mov instruction can assign the value of a register to another register, or assign a constant to a register.
Example:
mov r1, r2
mov r1, #4096
The constant transferred by the mov instruction must be represented by an immediate number.
When you don't know whether a number can be represented by an immediate number, you can use the ldr command to assign a value. ldr is a pseudo instruction, it is not a real instruction, the compiler will expand it into a real instruction: if the constant can be represented by an immediate value, use the mov instruction; otherwise, save the constant in a certain location during compilation, and use the memory read instruction to read it out.
Example:
ldr r1, =4097
The original meaning of ldr is "large-range address read pseudo instruction". The following is to obtain the absolute address of the code:
Example:
ldr r1, =label
label:
......
3. Memory access instructions: ldr, str, ldm, stm
The ldr instruction can be a large-range address read pseudo instruction or a memory access instruction. When there is "=" in front of its second parameter, it indicates a pseudo instruction, otherwise it indicates a memory access instruction. The
ldr instruction reads data from the memory to the register, and the str instruction stores the value of the register to the memory. The data they operate on are all 32 bits.
Example:
ldr r1, [r2, #4] // Read the data of the memory cell at address r2+4 into r1ldr
r1, [r2] // Read the data of the memory cell at address r2 into r1ldr
r1, [r2], #4 // Read the data of the memory cell at address r2 into r1, then r2=r2+4str
r1, [r2, #4] // Save the data of r1 into the memory cell at address r2+4str
r1, [r2] // Save the data of r1 into the memory cell at address r2str
r1, [r2], #4 // Save the data of r1 into the memory cell at address r2, then r2=r2+4ldm
and stm are batch memory access instructions, which can read and write multiple data with only one instruction. The format is:
ldm {cond}
stm {cond}
, where {cond} indicates the execution conditions of the instruction:
Condition code (cond) |
Mnemonics |
meaning |
Conditional flags in cpsr |
0000 |
eq |
equal |
From = 1 |
0001 |
it is |
not equal |
Z = 0 |
0010 |
cs/hs |
Unsigned greater than/equal to |
C = 1 |
0011 |
cc/lo |
Unsigned number is less than |
C = 0 |
0100 |
me |
negative number |
N = 1 |
0101 |
pl |
Non-negative numbers |
N = 0 |
0110 |
vs |
Overflow |
V = 1 |
0111 |
vc |
No overflow |
V = 0 |
1000 |
hi |
Unsigned number greater than |
C = 1 or Z = 0 |
1001 |
ls |
Unsigned number less than or equal to |
C = 0 or Z = 1 |
1010 |
ge |
Signed number greater than or equal to |
N = 1, V = 1 or N = 0, V = 0 |
1011 |
lt |
Signed number is less than |
N = 1, V = 0 or N = 0, V = 1 |
1100 |
gt |
Signed number greater than |
Z = 0 and N = V |
1101 |
the |
Signed less than/equal to |
Z = 1 or N! = V |
1110 |
al |
Unconditional execution |
- |
1111 |
nv |
Never execute |
- |
Most ARM instructions can be executed conditionally, that is, whether to execute the instruction is determined by the conditional flag in the cpsr register: if the condition is not met, the instruction is equivalent to a nop instruction.
Each ARM instruction contains a 4-bit conditional code field, which means that 16 execution conditions can be defined. The
cpsr conditional flags N, Z, C, and V represent Negative, Zero, Carry, and oVerflow, respectively.
ia (Increment After): post-increment mode.
ib (Increment Before): pre-increment mode.
da (Decrement After): post-decrement mode.
db (Decrement Before): pre-decrement mode.
{^} has two meanings:
if there is a pc register in
if there is no pc register in
Example:
HandleIRQ: @Interrupt entry function
sub lr, lr, #4 @Calculate the return address
stmdb sp!, { r0 - r12, lr } @Save the used registers
@r0 - r12, lr are saved in the memory represented by sp
@“!” makes the instruction execute sp = sp - 14 * 4
ldr lr, =int_return @Set the return address after calling the IRQ_Handle function
ldr pc, =IRQ_Handle @Call interrupt distribution function
int_return:
ldmia sp!, { r0 - r12, pc }^ @Interrupt return, “^” means to transfer the value of spsr to cpsr
@So return from irq mode to the interrupted working mode
@“!” makes the instruction execute sp = sp + 14 * 4
4. Addition and subtraction instructions: add , sub
Example:
add r1, r2, #1 // r1 = r2 + 1
sub r1, r2, #1 // r1 = r2 - 1
5. Program status register access instructions: msr, mrs
ARM processor has a program status register (cpsr), which is used to control the processor's working mode and set the interrupt switch.
Example:
msr cpsr, r0 // r0 to cpsr
mrs r0, cpsr // cpsr to r0
6. Other pseudo instructions.extern
: define an external symbol (can be a variable or a function)
.text: indicates that the current statements belong to the code segment.global
: define a program label in this file as global
ARM-THUMB subroutine call rules: ATPCS
In order to enable C language programs and assembly programs to call each other, rules must be formulated for subroutine calls. In ARM processors, this rule is called ATPCS: rules for subroutine calls in ARM programs and THUMB programs. The basic ATPCS rules include register usage rules, data stack usage rules, and parameter passing rules.
1. Register usage rules Subroutines
pass parameters through registers r0 ~ r3, and their aliases a1 ~ a4 can be used at this time. The contents of r0 ~ r3 do not need to be restored before the called subroutine returns.
In a subroutine, r4 ~ r11 are used to save local variables, and their aliases v1 ~ v8 can be used at this time. If some of their registers are used in the subroutine, the values of these registers must be saved when the subroutine enters and restored before returning; for registers not used in the subroutine, these operations are not necessary. In THUMB programs, usually only registers r4 ~ r7 can be used to save local variables.
Register r12 is used as a scratch register between subroutines, and its alias is ip.
Register r13 is used as a data stack pointer, and its alias is sp. Register r13 cannot be used for other purposes in a subroutine. Its value must be the same when entering and exiting a subroutine.
Register r14 is called a link register, and its alias is lr. It is used to save the return address of the subroutine. If the return address is saved in the subroutine (for example, the lr value is saved in the data stack), r14 can be used for other purposes.
Register r15 is the program counter, aliased as pc . It cannot be used for any other purpose.
register |
Aliases |
Rules of Use |
r15 |
pc |
Program Counter |
r14 |
lr |
Connection Register |
r13 |
sp |
Data stack pointer |
r12 |
ip |
The scratch register for the subroutine call |
r11 |
v8 |
ARM state local variable register 8 |
r10 |
v7、s1 |
ARM state local variable register 7. In ATPCS supporting data stack checking, it is the data stack limit pointer |
r9 |
v6、sb |
ARM state local variable register 6, static base address register in ATPCS supporting RWPI |
r8 |
v5 |
ARM state local variable register 5 |
r7 |
v4、wr |
ARM state local variable register 4, THUMB state working register |
r6 |
v3 |
ARM state local variable register 3 |
r5 |
v2 |
ARM state local variable register 2 |
r4 |
v1 |
ARM state local variable register 1 |
r3 |
a4 |
Parameters/results/scratch register 4 |
r2 |
a3 |
Parameters/results/scratch register 3 |
r1 |
a2 |
Parameters/results/scratch register 2 |
r0 |
a1 |
Parameters/results/scratch register 1 |
2. Data stack usage rules
The data stack has two growth directions: when it grows in the direction of decreasing memory address, it is called DESCENDING stack; when it grows in the direction of increasing memory address, it is called ASCENDING stack.
The so-called growth of the data stack is to move the stack pointer. When the stack pointer points to the top element of the stack (the last data pushed into the stack), it is called FULL stack; when the stack pointer points to an empty data unit adjacent to the top element of the stack (the last data pushed into the stack), it is called EMPTY stack.
The data stack can be divided into 4 types:
FD: Full Descending
ED: Empty Descending
FA: Full Ascending
EA: Empty Ascending
ATPCS stipulates that the data stack is of FD type, and the operation on the data stack is 8-byte aligned. Use stmdb / ldmia batch memory access instructions to operate the FD data stack.
When using the stmdb command to save content to the data stack, first decrement the sp pointer and then save the data. When using the ldmia command to restore data from the data stack, first obtain the data and then increment the sp pointer. The sp pointer always points to the top element of the stack, which is exactly the definition of the FD stack.
3. Parameter passing rules
Generally, when the number of parameters does not exceed 4, the four registers r0 ~ r3 are used to pass parameters; if the number of parameters exceeds 4, the remaining parameters are passed through the data stack.
For general return results, r0 ~ r3 are usually used to pass.
Example:
Assume that the CopyCode2SDRAM function is implemented in C language, and its data prototype is as follows:
int CopyCode2SDRAM( unsigned char *buf, unsigned long start_addr, int size )
In the assembly code, use the following code to call it and judge the return value:
ldr r0, =0x30000000 @1. Target address = 0x30000000, which is the starting address of SDRAM
mov r1, #0 @2. Source address = 0
mov r2, #16*1024 @3. Length = 16K
bl CopyCode2SDRAM @Call C function CopyCode2SDRAM
cmp a0, #0 @Judge the return value of the function
Previous article:ARM assembly instructions detailed explanation 1
Next article:ARM assembly instruction learning to implement data block copy
Recommended ReadingLatest update time:2024-11-16 13:50
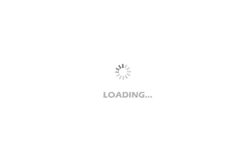
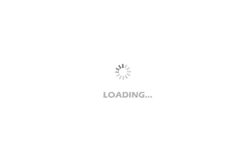
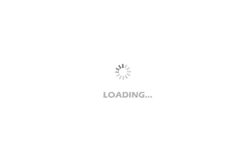
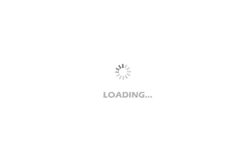
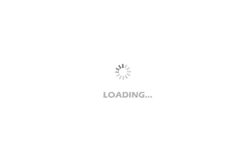
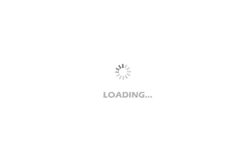
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Android 5.0 BLE low-power Bluetooth slave device application
- JHL HOOD 1969 small class A amplifier board
- Solutions to several difficult problems in the CCS debugging process
- Xunwei iTOP4418 development board Qt system ported 4G-EC20
- How do you calculate the cost of PCB materials?
- [MM32 eMiniBoard Review] Part 7: IIC Read and Write EEPROM
- Altium Designer's dirty tricks to combat piracy
- TMS320F2812 generates PWM waveform process
- TI Power Stage Designer Tool Introduction Documentation
- I would like to ask you, the STM32CUBEMX+SPI+DMA method always sends incorrectly. My chip is STM32F103RC