The stm32 standard peripheral library is the peripheral driver for the full range of stm32 chips. With it, we can greatly accelerate the development of stm32.
First, download the latest stm32 standard peripheral library from the website of st company. The latest version is v3.5.0 when writing this article.
Unzip the zip file and get the following folders and files:
STM32F10x_StdPeriph_Lib_V3.5.0\
_htmresc
Libraries
Project
Utilities
Release_Notes.html
stm32f10x_stdperiph_lib_um.chm
Libraries contains the source code of the library, Project contains examples of using various stm32 peripherals and a project template, Utilities is an example of using the st company's evaluation board, and stm32f10x_stdperiph_lib_um.chm teaches us how to use the standard peripheral library.
Since we are going to use 32-bit microcontrollers, it should be a big project, so we should also plan the project directory. Here I recommend the directory structure
I use. Assuming the project name is template, create a folder called template. There are three fixed folders under this directory: doc, src, and include. doc is used to store project-related data files, src is used to store source code, and each functional module has a folder under src. Include is used to store public header files used by each module. output is used to store compiled output files, which contains two subfolders: obj and list.
template\
doc
src
include
output\obj
\listOrganize
library code
Because many codes in the CMSIS folder under Libraries are related to compilers and chips, there are many folders and they are deep, which is not conducive to project maintenance. In fact, a project often uses fixed compilers and chips, so it is necessary to organize the library.
Create a libstm32 directory under src1
. Copy the contents under Libraries\STM32F10x_StdPeriph_Driver\ to the libstm32 directory2
. Create a cmsis folder under the libstm32 directory, and copy core_cm3.c, core_cm3.h under Libraries\CMSIS\CM3\CoreSupport\; stm32f10x.h, system_stm32f10x.c, system_stm32f10x.h under Libraries\CMSIS\CM3\DeviceSupport\ST\STM32F10x\ to the cmsis folder.
3. Copy the corresponding startup file under Libraries\CMSIS\CM3\DeviceSupport\ST\STM32F10x\startup\arm\ to the cmsis folder according to the chip type you selected. Here I copied startup_stm32f10x_hd.s (the startup file for the large-capacity stm32 chip).
Here is a brief introduction to the library file:
The contents under Libraries\STM32F10x_StdPeriph_Driver\ are the driver codes for various peripheral modules of stm32.
misc.h and misc.c are the driver codes for NVIC and SysTick related to the CM3 core.
What is under Libraries\CMSIS? The full name of cmsis is Cortex Microcontroller Software Interface Standard, which is the hardware abstraction layer of the Cortex series processors and can be understood as the software interface of the cortex core.
core_cm3.c, core_cm3.h,
their directory name is CoreSupport, indicating that these two files are CM3 core support files, and other chips using the CM3 core can also use them, not necessarily stm32. These two files are used to obtain and set the CM3 core and configure some core registers.
stm32f10x.h, system_stm32f10x.c, system_stm32f10x.h and startup_stm32f10x_hd.s are in the DeviceSupport directory, which means these files are related to specific chips, that is, the support files of the stm32 chip. Among them, stm32f10x.h is the entry of the standard peripheral library. The code using the standard peripheral library must include this header file. The two files system_stm32f10x.c and system_stm32f10x.h provide functions to initialize the stm32 chip, configure the PLL, system clock and built-in flash interface. startup_stm32f10x_hd.s is the startup file of the large-capacity stm32 chip.
Create a project
Use keil MDK (I use version 4.12) to create a project in the template directory, and the project name is template. Choose a chip from the stm32 series, it doesn't matter which one (I chose STM32F101RC because my board uses this chip). The next thing to note is that when the pop-up pops up whether to copy the startup code to the project folder, you must select No, because the startup code is already in the standard peripheral library.
Change the top-level directory name in the project window in UV4 to template, and change the first group name to libstm32. Load all .c and .s files in the libstm32 directory into the libstm32 in the project.
Create an init directory under src to place the system initialization code. Copy stm32f10x_it.c under Project\STM32F10x_StdPeriph_Template\ to the init folder, and copy stm32f10x_it.h and stm32f10x_conf.h to the include folder.
stm32f10x_it.c and stm32f10x_it.h are interrupt service program files. stm32f10x_conf.h is the configuration file of the standard peripheral library. For peripherals not needed in the project, you can comment out the included header files. Here I suggest leaving only stm32f10x_gpio.h, stm32f10x_rcc.h, misc.h, and then opening what you need, so that the compilation will be faster. Of course, you can also keep them all.
Use the stm32 standard peripheral library
In fact, the use of the stm32 standard peripheral library is described in the section How to use the Library in stm32f10x_stdperiph_lib_um.chm. Here I list the steps:
1. According to the selected chip, add the startup code in Libraries\CMSIS\CM3\DeviceSupport\ST\STM32F10x\startup\arm to the project. This step has been done above.
2. In lines 66-73 of stm32f10x.h, remove the corresponding comments according to the selected chip type. Here I remove the comments of the STM32F10X_HD line (large-capacity stm32 chip).
3. Remove the USE_STDPERIPH_DRIVER comment on line 105 to enable the stm32 standard peripheral library.
4. In lines 110-115 of system_stm32f10x.c, remove the corresponding comments according to the selected chip frequency. The default SYSCLK_FREQ_72MHz comment has been removed. If your chip frequency is 72MHz, you don’t need to make any changes. My chip is 36MHz, comment SYSCLK_FREQ_72MHz, and remove the SYSCLK_FREQ_36MHz comment.
The marquee program
can now use the stm32 standard peripheral library. The following is an illustration of a simple marquee program.
Create main.c in the init directory as the system entry.
Create a bsp directory under src to place the board-level support code, and create led.c and led.h.
The code is as follows:
led.h
- #ifndef _LED_H_
- #define _LED_H_
- #include
- #define LED_0 0
- #define LED_1 1
- #define LED_2 2
- void led_init(void);
- void led_on(uint32_t n);
- void led_off(uint32_t n);
- #endif
led.c
- #include "stm32f10x.h"
- #include "led.h"
- void led_init(void)
- {
- GPIO_InitTypeDef GPIO_InitStructure;
- RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
- GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7 | GPIO_Pin_8;
- GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
- GPIO_InitStructure.GPIO_Speed = GPIO_Speed_2MHz;
- GPIO_Init(GPIOC, &GPIO_InitStructure);
- }
- void led_on(uint32_t n)
- {
- switch (n)
- {
- case LED_0:
- GPIO_SetBits(GPIOC, GPIO_Pin_6);
- break;
- case LED_1:
- GPIO_SetBits(GPIOC, GPIO_Pin_7);
- break;
- case LED_2:
- GPIO_SetBits(GPIOC, GPIO_Pin_8);
- break;
- default:
- break;
- }
- }
- void led_off(uint32_t n)
- {
- switch (n)
- {
- case LED_0:
- GPIO_ResetBits(GPIOC, GPIO_Pin_6);
- break;
- case LED_1:
- GPIO_ResetBits(GPIOC, GPIO_Pin_7);
- break;
- case LED_2:
- GPIO_ResetBits(GPIOC, GPIO_Pin_8);
- break;
- default:
- break;
- }
- }
The pins in the code need to be modified according to different boards, but the structure is the same.
main.c
- #include "led.h"
- static void delay(uint32_t ms)
- {
- uint32_t count = 8000;
- while (ms--)
- {
- while (count--);
- count = 8000;
- }
- }
- int main(void)
- {
- led_heat();
- for (;;)
- {
- led_on(LED_0);
- led_off(LED_1);
- led_off(LED_2);
- delay(1000);
- led_off(LED_0);
- led_on(LED_1);
- led_off(LED_2);
- delay(1000);
- led_off(LED_0);
- led_off(LED_1);
- led_on(LED_2);
- delay(1000);
- }
- }
Create init and bsp groups in the project and add various codes. In the project Options, add .\include;.\src\libstm32\cmsis;.\src\libstm32\inc;.\src\bsp; to Include Paths in the c/c++ tab. Select .
\output\obj in Select Folder for Objects in the Output tab.
Select .\output\list in Select Folder for Listings in the Listing tab.
Check use ULINK Cortex Debugger, Run to main() in the Debug tab. You can make different choices in this step according to the simulator you have.
Previous article:Detailed explanation of ucosii transplantation on stm32 4
Next article:Detailed explanation of ucosii transplantation on stm32 1
Recommended ReadingLatest update time:2024-11-16 17:59
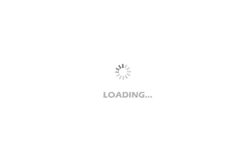
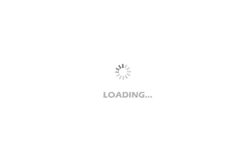
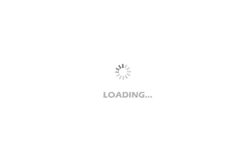
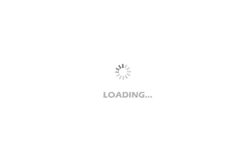
- Popular Resources
- Popular amplifiers
Professor at Beihang University, dedicated to promoting microcontrollers and embedded systems for over 20 years.
- Innolux's intelligent steer-by-wire solution makes cars smarter and safer
- 8051 MCU - Parity Check
- How to efficiently balance the sensitivity of tactile sensing interfaces
- What should I do if the servo motor shakes? What causes the servo motor to shake quickly?
- 【Brushless Motor】Analysis of three-phase BLDC motor and sharing of two popular development boards
- Midea Industrial Technology's subsidiaries Clou Electronics and Hekang New Energy jointly appeared at the Munich Battery Energy Storage Exhibition and Solar Energy Exhibition
- Guoxin Sichen | Application of ferroelectric memory PB85RS2MC in power battery management, with a capacity of 2M
- Analysis of common faults of frequency converter
- In a head-on competition with Qualcomm, what kind of cockpit products has Intel come up with?
- Dalian Rongke's all-vanadium liquid flow battery energy storage equipment industrialization project has entered the sprint stage before production
- Allegro MicroSystems Introduces Advanced Magnetic and Inductive Position Sensing Solutions at Electronica 2024
- Car key in the left hand, liveness detection radar in the right hand, UWB is imperative for cars!
- After a decade of rapid development, domestic CIS has entered the market
- Aegis Dagger Battery + Thor EM-i Super Hybrid, Geely New Energy has thrown out two "king bombs"
- A brief discussion on functional safety - fault, error, and failure
- In the smart car 2.0 cycle, these core industry chains are facing major opportunities!
- The United States and Japan are developing new batteries. CATL faces challenges? How should China's new energy battery industry respond?
- Murata launches high-precision 6-axis inertial sensor for automobiles
- Ford patents pre-charge alarm to help save costs and respond to emergencies
- New real-time microcontroller system from Texas Instruments enables smarter processing in automotive and industrial applications
- Help with UART transmission problem of RL78 G14
- A website that can customize the modules contained in the nodemcu firmware (seemingly lua language version)
- Detailed Explanation of Current Sensing Methods for Switch-Mode Power Supplies
- LIS25BA configuration on STM32F4
- [List Announced] If you were given a blood oximeter, what would you do with it?
- How to deal with the problem of running away during program debugging?
- [NXP Rapid IoT Review] +② NXP Rapid IoT Online Programming Preparation
- Instead of just deleting posts, why not have some scientific pursuit?
- 3.EV_HC32F460_ADC debugging
- Wireless atmosphere light smooth dimming system based on GD32E231